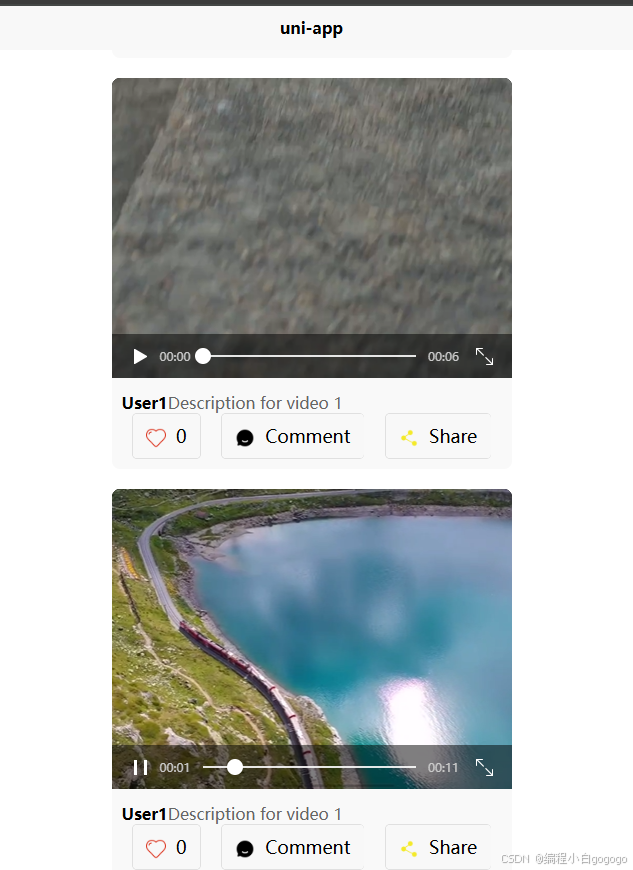
<template>
<view class="container">
<scroll-view scroll-y class="video-list" @scrolltolower="loadMoreVideos">
<view v-for="(video, index) in videos" :key="video.id" class="video-item">
<video
:id="`myVideo-${index}`"
:src="video.url"
controls
object-fit="cover"
class="video-player"
@play="onVideoPlay(index)"
@click="navigateToVideoDetail(video.id)"
></video>
<view class="video-info">
<text class="username">{{ video.username }}</text>
<text class="description">{{ video.description }}</text>
<view class="actions">
<button @click="toggleLike(video)">
<image :src="isLiked(video.id) ? '/static/liked.png' : '/static/like.png'" class="action-icon"></image>
{{ video.likes }}
</button>
<button @click="showComments(video)">
<image src="/static/comment.png" class="action-icon"></image>
Comment
</button>
<button>
<image src="/static/share.png" class="action-icon"></image>
Share
</button>
</view>
</view>
</view>
</scroll-view>
<!-- 评论弹窗 -->
<view v-if="selectedVideo && showCommentModal" class="comment-modal">
<view class="modal-content">
<textarea v-model="newComment" placeholder="写下你的评论..." class="comment-input"></textarea>
<button @click="addComment">提交评论</button>
<view class="comments-list">
<text v-for="comment in selectedVideo.comments" :key="comment.id" class="comment-item">
{{ comment.text }}
</text>
</view>
<button @click="closeCommentModal">关闭</button>
</view>
</view>
</view>
</template>
<script>
export default {
data() {
return {
videos: [
{
id: 1,
url: '/static/1.mp4',
username: 'User1',
description: 'Description for video 1',
likes: 0,
},
{
id: 2,
url: '/static/2.mp4',
username: 'User2',
description: 'Description for video 2',
likes: 0,
},
{
id: 3,
url: '/static/3.mp4',
username: 'User3',
description: 'Description for video 1',
likes: 0,
},
{
id: 4,
url: '/static/4.mp4',
username: 'User4',
description: 'Description for video 1',
likes: 0,
},
{
id: 5,
url: '/static/5.mp4',
username: 'User5',
description: 'Description for video 2',
likes: 0,
},
{
id: 6,
url: '/static/6.mp4',
username: 'User6',
description: 'Description for video 1',
likes: 0,
},
{
id: 7,
url: '/static/7.mp4',
username: 'User7',
description: 'Description for video 1',
likes: 0,
},
{
id: 8,
url: '/static/8.mp4',
username: 'User2',
description: 'Description for video 2',
likes: 0,
},
{
id: 9,
url: '/static/9.mp4',
username: 'User1',
description: 'Description for video 1',
likes: 0,
},
{
id: 10,
url: '/static/10.mp4',
username: 'User1',
description: 'Description for video 1',
likes: 0,
},
// Add more video objects as needed
],
currentIndex: 0,
likedVideos: [], // 追踪已点赞的视频ID
showCommentModal: false, // 控制评论弹窗的显示状态
selectedVideo: null, // 当前选择的视频对象
newComment: ''
};
},
methods: {
toggleLike(video) {
const index = this.likedVideos.indexOf(video.id);
if (index > -1) {
// 如果已经点赞,则取消点赞
this.likedVideos.splice(index, 1);
video.likes--; // 减少点赞数
} else {
// 否则添加到已点赞的列表中
this.likedVideos.push(video.id);
video.likes++; // 增加点赞数
}
},
isLiked(id) {
return this.likedVideos.includes(id);
},
likeVideo(index) {
const video = this.videos[index];
if (this.likedVideos.includes(video.id)) {
this.likedVideos = this.likedVideos.filter(id => id !== video.id);
video.likes--;
} else {
this.likedVideos.push(video.id);
video.likes++;
}
this.saveLikedVideosToLocalStorage();
},
saveLikedVideosToLocalStorage() {
localStorage.setItem('likedVideos', JSON.stringify(this.likedVideos));
},
loadLikedVideosFromLocalStorage() {
const storedLikedVideos = localStorage.getItem('likedVideos');
if (storedLikedVideos) {
this.likedVideos = JSON.parse(storedLikedVideos);
this.videos.forEach(video => {
if (this.likedVideos.includes(video.id)) {
video.likes++;
}
});
}
},
onVideoPlay(index) {
if (this.currentIndex !== index) {
const currentVideoContext = uni.createVideoContext(`myVideo-${this.currentIndex}`);
currentVideoContext.pause();
this.currentIndex = index;
}
},
loadMoreVideos() {
console.log('Loading more videos...');
},
navigateToVideoDetail(id) {
uni.navigateTo({
url: `/pages/videoDetail/videoDetail?id=${id}`,
});
},
showComments(video) {
this.selectedVideo = video;
this.showCommentModal = true;
},
closeCommentModal() {
this.showCommentModal = false;
this.newComment = ''; // 清空输入框
},
addComment() {
if (this.newComment.trim()) {
// 假设每个视频都有一个 comments 数组存储评论
const newCommentItem = { id: Date.now(), text: this.newComment };
this.selectedVideo.comments.push(newCommentItem);
this.newComment = ''; // 清空输入框
}
},
},
mounted() {
this.loadLikedVideosFromLocalStorage();
},
};
</script>
<style>
.comment-modal {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5); /* 半透明背景 */
display: flex;
justify-content: center;
align-items: center;
}
.modal-content {
background-color: white;
padding: 20px;
border-radius: 8px;
width: 80%;
max-width: 500px;
}
.comment-input {
width: 100%;
height: 60px;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 4px;
}
.comments-list {
max-height: 200px;
overflow-y: auto;
margin-bottom: 10px;
}
.comment-item {
padding: 5px 0;
}
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.video-list {
width: 100%;
max-width: 400px;
}
.video-item {
margin-bottom: 20px;
border-radius: 8px;
overflow: hidden;
background-color: #f9f9f9;
}
.video-player {
width: 100%;
height: 300px;
}
.video-info {
padding: 10px;
}
.username {
font-weight: bold;
margin-bottom: 5px;
}
.description {
color: #666;
margin-bottom: 10px;
}
.actions {
display: flex;
justify-content: space-between;
}
.action-icon {
width: 20px;
height: 20px;
vertical-align: middle;
margin-right: 5px;
}
.liked .action-icon {
filter: hue-rotate(345deg); /* 红色色调 */
}
</style>