一.引言
在Android中,不论是实际开发,还是在android面试中,MVC无时无刻都存在我们的身边,由此可见MVC的重要性,所以,我将分模块来对MVC框架做一个完整的剖析,全面认识MVC,能够在项目开发中非常熟练的引用。
二.MVC的基本概念
MVC的全名是: Model View Controller,是模型(Model)-视图(View)-控制器(Controller)的简写,它是一种代码设计典范,通过业务逻辑,数据,视图分离的方式来组织代码的编写,通过MVC,我们可以将不同的操作分离出来,各模块各司其职,专门处理其对应的工作,使得代码达到易维护,重复使用,耦合低等效果。
三.MVC中三个模块的简单描述
(1)模型(Model): 一些业务逻辑可以放在该层来进行处理,比如网络请求数据,对数据库的增删改查的处理,总之,一系列涉及到业务逻辑处理和一些耗时的操作,都可以分离出来,放在Model层来操作;
(2)视图(View): 在android中,该层主要是指xml的布局文件,用来显示界面数据,直接与用户进行交互;
(3)控件器(Controller):充当桥梁的作用,用于协调模型(Model)和视图(View)的处理,控制数据的展示。
四.商品列表案例概叙MVC
这里为了简单起见,数据在本地随机生成,没有从服务器获取,因为主要重点不是网络交互。
首先,看效果图:
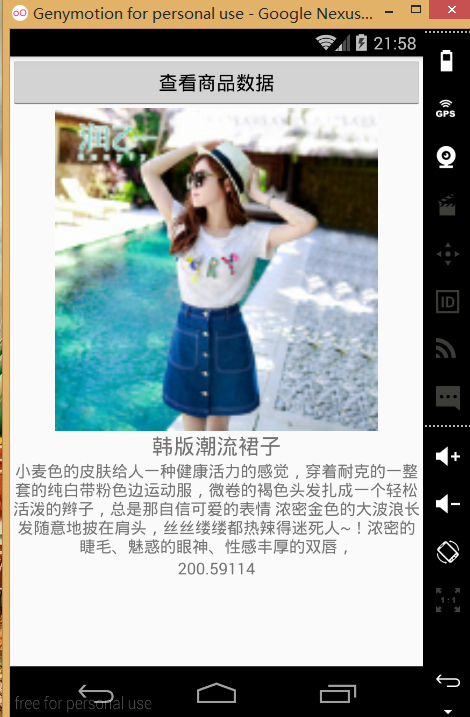
activity:
package mzbemployeeapp.wellke.com.mvcdemo;
import android.os.Bundle;
import android.widget.ImageView;
import android.widget.TextView;
import butterknife.ButterKnife;
import butterknife.InjectView;
import butterknife.OnClick;
import mzbemployeeapp.wellke.com.mvcdemo.activitys.BaseActivity;
import mzbemployeeapp.wellke.com.mvcdemo.entity.Shop;
import mzbemployeeapp.wellke.com.mvcdemo.interfaces.GetData;
import mzbemployeeapp.wellke.com.mvcdemo.listener.GetDataListener;
import mzbemployeeapp.wellke.com.mvcdemo.model.GetDataImplement;
public class MainActivity extends BaseActivity implements GetDataListener {
@InjectView(R.id.shop_name)
TextView shopName;
@InjectView(R.id.shop_desc)
TextView shopDesc;
@InjectView(R.id.shop_price)
TextView shopPrice;
GetData getData;
@InjectView(R.id.shop_image)
ImageView shopImage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.inject(this);
init();
}
private void init() {
getData = new GetDataImplement();
}
@OnClick(R.id.btn)
public void onClick() {
getData.getShopsData(this);
}
@Override
public void onGetDataSuccess(Shop shop) {
showData(shop);
}
@Override
public void onGetDataError(String errmessage) {
}
//控制视图(View)层数据显示的方法
private void showData(Shop shop) {
shopImage.setImageResource(shop.getImage());
shopName.setText(shop.getName());
shopPrice.setText(String.valueOf(shop.getPrice()));
shopDesc.setText(shop.getDesc());
}
}
package mzbemployeeapp.wellke.com.mvcdemo.listener;
import mzbemployeeapp.wellke.com.mvcdemo.entity.Shop;
/**
* Created by wellke on 2016/1/28.
*/
public interface GetDataListener {
void onGetDataSuccess(Shop shop);
void onGetDataError(String errmessage);
}
package mzbemployeeapp.wellke.com.mvcdemo.interfaces;
import mzbemployeeapp.wellke.com.mvcdemo.listener.GetDataListener;
/**
* Created by wellke on 2016/1/28.
*/
public interface GetData {
void getShopsData(GetDataListener getDataListener);
}
package mzbemployeeapp.wellke.com.mvcdemo.entity;
/**
* Created by wellke on 2016/1/28.
*/
public class Shop {
String name;
int image;
String desc;
float price;
public Shop() {
}
public Shop(String name, int image, float price, String desc) {
this.name = name;
this.image = image;
this.price = price;
this.desc = desc;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getImage() {
return image;
}
public void setImage(int image) {
this.image = image;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
}
package mzbemployeeapp.wellke.com.mvcdemo.model;
import java.util.Random;
import mzbemployeeapp.wellke.com.mvcdemo.R;
import mzbemployeeapp.wellke.com.mvcdemo.entity.Shop;
import mzbemployeeapp.wellke.com.mvcdemo.interfaces.GetData;
import mzbemployeeapp.wellke.com.mvcdemo.listener.GetDataListener;
/**
* Created by wellke on 2016/1/28.
*/
public class GetDataImplement implements GetData {
//这里在实际开发中应该是网络请求数据,这里为了方便直接在本地生成
@Override
public void getShopsData(GetDataListener getDataListener) {
//随机生成一组数据
Shop shop=new Shop("韩版潮流裙子", R.mipmap.skirt_15,new Random().nextFloat()+200.0f,"小麦色的皮肤给人一种健康活力的感觉," +
"穿着耐克的一整套的纯白带粉色边运动服,微卷的褐色头发扎成一个轻松活泼的辫子,总是那自信可爱的表情 浓密金" +
"色的大波浪长发随意地披在肩头," + "丝丝缕缕都热辣得迷死人~!浓密的睫毛、魅惑的眼神、性感丰厚的双唇,");
if (shop != null){
getDataListener.onGetDataSuccess(shop);
}
else {
getDataListener.onGetDataError("数据请求失败");
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="mzbemployeeapp.wellke.com.mvcdemo.MainActivity">
<Button
android:id="@+id/btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="查看商品数据" />
<ImageView
android:layout_width="300dp"
android:layout_height="300dp"
android:layout_gravity="center"
android:id="@+id/shop_image"
/>
<TextView
android:id="@+id/shop_name"
android:gravity="center"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/shop_desc"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="15sp"
android:gravity="center"
/>
<TextView
android:id="@+id/shop_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="15sp"
android:gravity="center"
/>
</LinearLayout>
五.实例简述
这个实例其实非常简单,就是当你点击按钮的时候获取数据,并对其进行了展示,但是他运用MVC框架模式,使得程序代码健壮性提升了几个档次,这里activity充当控制器(Controller)的角色,用于控制数据的展示,布局文件则是视图层,用于直接展示数据,与用户进行交互,GetDataImplement是模型(Model)层,用于获取数据,在activity中,实现了GetDataListener,当点击按钮时,调用GetDataImplement中的getShopsData方法,获取商品数据,在getShopsData方法中,当数据获取获取成功后就调用GetDataListener的onGetDataSuccess方法,否则调用onGetDataError方法,然后再activity实现GetDataListener接口时,会实现onGetDataSuccess和onGetDataError这两个方法。
六.总结
通过MVC,可以大大的简化代码的编写,首先,降低了代码间的耦合性,不会由于修改了某一个地方,而导致其他模块也发生了变化,然后,代码也更易于维护,比如,在另一个界面,我们要从服务器获取数据,我们只需要另外写一个类,去实现GetData接口即可,不用再activity中做大量的修改,这样当我们在添加需求和修改需求时,可以大大降低因修改代码而造成bug出现的情况。