Excel表格(多种样式开发)——JAVA开发
本博客中的样式为多表头样式,下面放入一张成型图
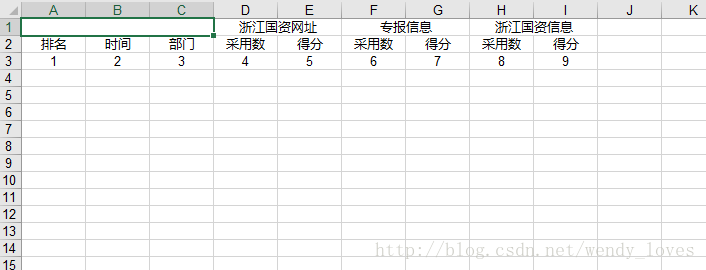
Maven:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.9</version>
</dependency>
代码展示
CellModel.java
public class CellModel {
private String cellName;
private Integer startRow;
private Integer startColumn;
private Integer endRow;
private Integer endColumn;
public String getCellName() {
return cellName;
}
public void setCellName(String cellName) {
this.cellName = cellName;
}
public Integer getStartRow() {
return startRow;
}
public void setStartRow(Integer startRow) {
this.startRow = startRow;
}
public Integer getStartColumn() {
return startColumn;
}
public void setStartColumn(Integer startColumn) {
this.startColumn = startColumn;
}
public Integer getEndRow() {
return endRow;
}
public void setEndRow(Integer endRow) {
this.endRow = endRow;
}
public Integer getEndColumn() {
return endColumn;
}
public void setEndColumn(Integer endColumn) {
this.endColumn = endColumn;
}
}
Util.java
public static File createCSVUtil(String xlsName, List<CellModel> cellNameList, Integer cellRow, List<LinkedHashMap> exportData, LinkedHashMap rowMapper, String outPutPath) throws Exception {
File XlsUtil = null
//表格创建&命名
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet = workbook.createSheet(xlsName)
HSSFCellStyle cellStyle = workbook.createCellStyle()
cellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER)
HSSFRow row = sheet.createRow(cellRow)
for (CellModel cellModel : cellNameList) {
//遍历插入表头
HSSFCell cell = row.createCell(cellModel.getStartColumn())
cell.setCellValue(cellModel.getCellName())
cell.setCellStyle(cellStyle)
}
for (CellModel cellModel : cellNameList) {
sheet.addMergedRegion(new CellRangeAddress(cellModel.getStartRow(), cellModel.getEndRow(), cellModel.getStartColumn(), cellModel.getEndColumn()))
}
cellRow++
HSSFRow row1 = sheet.createRow(cellRow)
Iterator<Map.Entry> iterator = rowMapper.entrySet().iterator()
while (iterator.hasNext()) {
Map.Entry entry = iterator.next()
Integer key = Integer.valueOf(entry.getKey().toString())
String value = entry.getValue().toString()
HSSFCell cell = row1.createCell(key - 1)
cell.setCellValue(value)
cell.setCellStyle(cellStyle)
}
for (LinkedHashMap hashMap : exportData) {
cellRow++
HSSFRow rowValue = sheet.createRow(cellRow)
Iterator<Map.Entry> iteratorRow = hashMap.entrySet().iterator()
while (iteratorRow.hasNext()) {
Map.Entry entryRow = iteratorRow.next()
Integer key = Integer.valueOf(entryRow.getKey().toString())
String value = entryRow.getValue().toString()
HSSFCell cellValue = rowValue.createCell(key - 1)
cellValue.setCellValue(value)
cellValue.setCellStyle(cellStyle)
}
}
FileOutputStream output = new FileOutputStream(outPutPath + xlsName + ".csv")
workbook.write(output)
output.flush()
XlsUtil = new File(outPutPath + xlsName + ".csv")
return XlsUtil
}
public static void main(String[] args) {
String xlsName = "东源欧巴的表格"
List<CellModel> cellNameList = new ArrayList()
CellModel cellModel1 = new CellModel()
Integer cellRow = 0
cellModel1.setCellName("")
cellModel1.setStartRow(0)
cellModel1.setEndRow(0)
cellModel1.setStartColumn(0)
cellModel1.setEndColumn(2)
CellModel cellModel2 = new CellModel()
cellModel2.setCellName("浙江国资网址")
cellModel2.setStartRow(0)
cellModel2.setEndRow(0)
cellModel2.setStartColumn(3)
cellModel2.setEndColumn(4)
CellModel cellModel3 = new CellModel()
cellModel3.setCellName("专报信息")
cellModel3.setStartRow(0)
cellModel3.setEndRow(0)
cellModel3.setStartColumn(5)
cellModel3.setEndColumn(6)
CellModel cellModel4 = new CellModel()
cellModel4.setCellName("浙江国资信息")
cellModel4.setStartRow(0)
cellModel4.setEndRow(0)
cellModel4.setStartColumn(7)
cellModel4.setEndColumn(8)
cellNameList.add(cellModel1)
cellNameList.add(cellModel2)
cellNameList.add(cellModel3)
cellNameList.add(cellModel4)
LinkedHashMap rowMapper = new LinkedHashMap() {
{
put("1", "排名")
put("2", "时间")
put("3", "部门")
put("4", "采用数")
put("5", "得分")
put("6", "采用数")
put("7", "得分")
put("8", "采用数")
put("9", "得分")
}
}
List<LinkedHashMap> exportData = Lists.newArrayList()
LinkedHashMap<String, String> rowPut = Maps.newLinkedHashMap()
rowPut.put("1", "1" + "\t")
rowPut.put("2", "2" + "\t")
rowPut.put("3", "3" + "\t")
rowPut.put("4", "4" + "\t")
rowPut.put("5", "5" + "\t")
rowPut.put("6", "6" + "\t")
rowPut.put("7", "7" + "\t")
rowPut.put("8", "8" + "\t")
rowPut.put("9", "9" + "\t")
exportData.add(rowPut)
try {
createCSVUtil(xlsName,cellNameList,cellRow,exportData,rowMapper,"D://")
} catch (Exception e) {
e.printStackTrace()
}
}
本文只是简单的封装了多表头的Excel工具类,如果你的项目中应用的样式更“花哨”,更头疼,可以从本文中获取新的思路,代码还有优化的必要。希望能给你带来帮助