struts 2
由Apache 软件基金会(ASF)赞助的一个开源项目,基于Java Servlet、JSP以及Custom Tag Library 等技术,实现了mvc设计模式的应用框架。
参考链接 struts2 教程
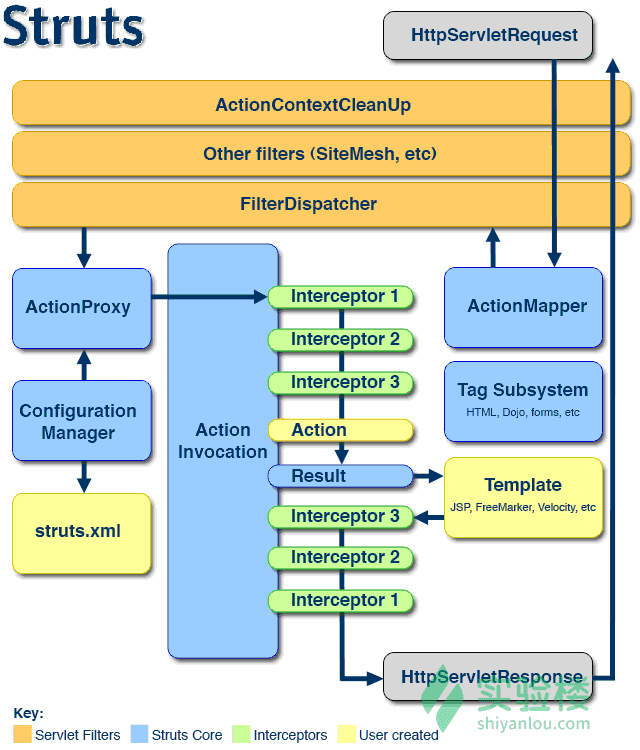
********************* 简单项目实例 ********************
项目结构
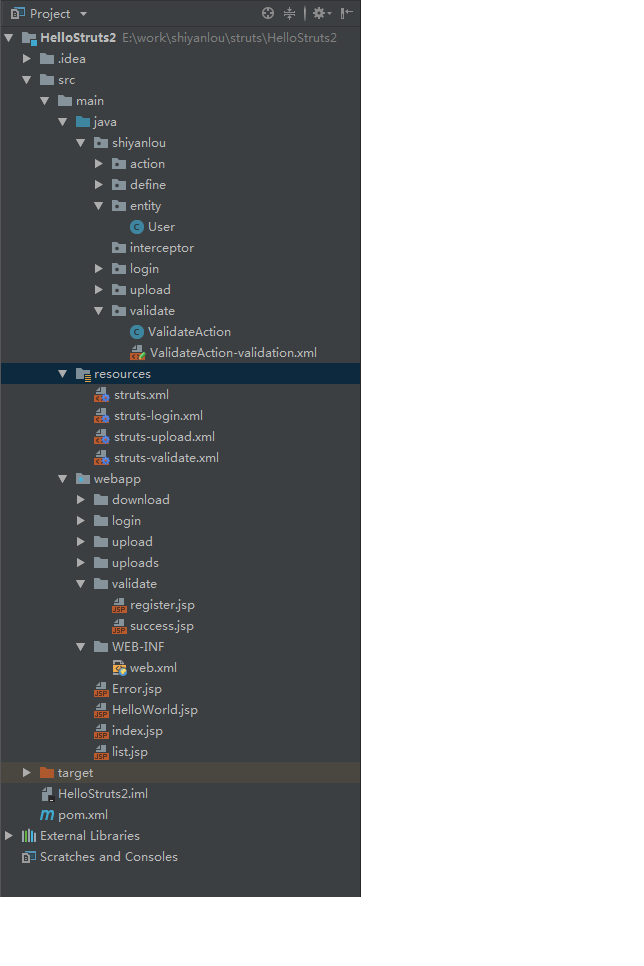
web.xml 简单配置
<display-name>HelloStruts2</display-name> <!-- 项目展示名称 -->
<filter>
<filter-name>struts2</filter-name> <!-- 过滤器名称 -->
<filter-class> <!-- 过滤器完整类路径 -->
org.apache.struts2.dispatcher.filter.StrutsPrepareAndExecuteFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name> <!-- 在 filter 中定义的过滤器 -->
<url-pattern>/*</url-pattern> <!-- 指定过滤器适用路径 -->
</filter-mapping>
<welcome-file-list> <!-- 网站欢迎页/首页,可以不配置,亲测 输入地址127.0.0.1:8080 直接到达 index.jsp -->
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
HelloWorld 简单action
public class HelloWorldAction {
private String name;
public String execute() throws Exception { // action 执行方法,必须返回字串,方法名可自定义
if (getName() == null || getName().equals("")) {
return "error";
}
return "hello";
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
struts.xml 简单配置
<!-- 一些全局配置 -->
<constant name="struts.enable.DynamicMethodInvocation" value="false"/> <!-- 是否开启动态方法代理 -->
<constant name="struts.ognl.allowStaticMethodAccess" value="true"/> <!-- 是否允许jsp访问静态方法 -->
<constant name="struts.devMode" value="true"/> <!-- 是否开启调试模式 -->
<!-- 包,一系列相关action的集合 -->
<!-- name 包名称 -->
<!-- namespace 包命名空间 如 "/first",则访问其包下 "second" action 路径为 /first/second -->
<!-- extends 包继承,默认struts-default,将继承其包内所有内容 -->
<package name="default" namespace="" extends="struts-default">
<default-action-ref name="index"/> <!-- 包默认action -->
<global-results> <!-- 包内返回结果设置(当包内多个action返回相同结果时) -->
<result name="error">/Error.jsp</result>
</global-results>
<global-exception-mappings> <!-- 包内异常捕获映射,指定发生特定异常时返回哪个结果 -->
<exception-mapping exception="java.lang.Exception" result="error"/>
</global-exception-mappings>
<action name="index"> <!-- 这样配置直接返回页面 -->
<result>/index.jsp</result>
</action>
<!-- action 响应处理 -->
<!-- name 包内唯一标识 -->
<!-- class 类路径,从包开始 -->
<!-- method 执行方法,默认为 execute,可以不写,但如果自定义方法名则必须给出 -->
<action name="hello" class="shiyanlou.action.HelloWorldAction" method="execute">
<!-- result 返回结果 -->
<!-- name 结果名称,对应 java action 类中执行方法的返回字串 -->
<!-- type 返回类型,默认 dispatcher,可不配置;其他类型必须配置-->
<result name="hello" type="dispatcher">/HelloWorld.jsp</result> <!-- 以webapp为根目录 -->
</action>
<action name="list" class="shiyanlou.action.ShowListAction">
<result name="list">/list.jsp</result>
</action>
</package>
<!-- 引入其他配置文件 项目较大时可分模块配置 -->
<!-- <include file="struts-upload.xml"/> -->
index.jsp 欢迎页/首页
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>wellcome</title>
</head>
<body>
<h1>Hello World Struts2</h1>
<form action="hello">
<label for="name">Please enter your name</label><br/>
<input type="text" name="name" id="name"/>
<input type="submit" value="Enter"/>
</form>
</body>
</html>
HelloWorld.jsp 结果页
<%@ page contentType="text/html;charset=UTF-8" language="java" pageEncoding="utf-8" %>
<%@taglib prefix="s" uri="/struts-tags" %> <!-- 引入struts标签 -->
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>hello world</title>
</head>
<body>
Hello, this is my review page of first test.
<br/>
--by <s:property value="name"/>
</body>
</html>
********************* JSP 数据展示 ********************
访问集合
集合大小: collection.size 或 collection.size() eg: list.size
所有元素的某个属性列表:collection.{attr} eg: collection.{name} eg: list.{name}
list [有序集合]
- 单个元素:list[index] eg: list[0]
- 某个元素的属性:list[index].attr 或 list.{attr}[index]
set [无序]
- 单个元素:不能获取特定元素
- 某个元素的属性:不能获取特定元素的属性,可以获得随机元素的属性 set.{attr}[0] eg: set.{name}[0]
map [无序]
- 单个元素:map[key] 或 map.key eg: map['user1'] map.user1
- 某个元素的属性:map[key].attr 或 map.key.attr eg: map['user1'].name map.user1.name
- 所有key 列表:map.keys eg: map.keys
- 所有value列表:map.values eg: map.values
过滤集合
? - 满足条件的所有元素
符合条件的所有元素的属性列表: list.{?#this.age>16}.{name}
某个元素:list.{?#this.age>16}[0]
元素的属性 list.{?#this.age>16}[0].name list.{?#this.age>16}.{name}[0]
^ - 满足条件的第一个元素
$ - 满足条件的最后一个元素