package com.xxx.util;
import org.elasticsearch.action.admin.cluster.state.ClusterStateResponse;
import org.elasticsearch.action.admin.indices.analyze.AnalyzeAction;
import org.elasticsearch.action.admin.indices.analyze.AnalyzeRequestBuilder;
import org.elasticsearch.action.admin.indices.analyze.AnalyzeResponse.AnalyzeToken;
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.action.admin.indices.create.CreateIndexResponse;
import org.elasticsearch.action.admin.indices.delete.DeleteIndexResponse;
import org.elasticsearch.action.admin.indices.exists.indices.IndicesExistsRequest;
import org.elasticsearch.action.admin.indices.exists.indices.IndicesExistsResponse;
import org.elasticsearch.action.bulk.BulkRequestBuilder;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.delete.DeleteRequestBuilder;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.action.search.SearchRequestBuilder;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.action.search.SearchType;
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.client.transport.TransportClient;
import org.elasticsearch.cluster.node.DiscoveryNode;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.transport.InetSocketTransportAddress;
import org.elasticsearch.common.unit.TimeValue;
import org.elasticsearch.index.query.BoolQueryBuilder;
import org.elasticsearch.index.query.QueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.SearchHits;
import org.elasticsearch.search.aggregations.AggregationBuilders;
import org.elasticsearch.search.aggregations.bucket.histogram.DateHistogramAggregationBuilder;
import org.elasticsearch.search.aggregations.bucket.histogram.DateHistogramInterval;
import org.elasticsearch.search.aggregations.bucket.histogram.ExtendedBounds;
import org.elasticsearch.search.aggregations.bucket.histogram.Histogram;
import org.elasticsearch.search.aggregations.bucket.terms.Terms;
import org.elasticsearch.search.aggregations.bucket.terms.TermsAggregationBuilder;
import org.elasticsearch.search.aggregations.metrics.avg.Avg;
import org.elasticsearch.search.aggregations.metrics.avg.AvgAggregationBuilder;
import org.elasticsearch.search.aggregations.metrics.sum.Sum;
import org.elasticsearch.search.aggregations.metrics.sum.SumAggregationBuilder;
import org.elasticsearch.search.sort.SortBuilders;
import org.elasticsearch.transport.client.PreBuiltTransportClient;
import java.net.InetAddress;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.json.JSONException;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.xxx.common.StaticContant;
import com.xxx.util.DateUtil;
/**
* Title: ElasticSearchUtil
* Description: ES工具类
* @author wufei
* @date 2017/12/26 下午2:07:06
*/
public class ElasticSearchUtil {
private static final Logger logger = LoggerFactory.getLogger(ElasticSearchUtil.class);
private static TransportClient client = StaticContant.ESCLIENT;
/**
* 功能描述:服务初始化(本地测试使用,实际开发写为长连接)
* @param clusterName 集群名称
* @param ip 地址
* @param port 端口
*/
@SuppressWarnings({ "resource", "static-access" })
public ElasticSearchUtil (String clusterName, String ip, int port) {
try {
// 通过setting对象指定集群配置信息, 配置集群名称
Settings settings = Settings.builder()
.put("cluster.name", clusterName) //设置集群名
// .put("client.transport.sniff", true) //启动嗅探功能,自动嗅探整个集群的状态,把集群中其他ES节点的ip添加到本地的客户端列表中
// .put("client.transport.ignore_cluster_name", true)//忽略集群名字验证, 打开后集群名字不对也能连接上
// .put("client.transport.nodes_sampler_interval", 5)//报错
// .put("client.transport.ping_timeout", 5) //报错, ping等待时间
.build();
// 创建client,通过setting来创建,若不指定则默认链接的集群名为elasticsearch,链接使用tcp协议即9300
// addTransportAddress此步骤添加IP,至少一个,其实一个就够了,因为添加了自动嗅探配置
this.client = new PreBuiltTransportClient(settings)
.addTransportAddress(new InetSocketTransportAddress(InetAddress.getByName(ip), port));
} catch (Exception e) {
logger.error("es init failed! " + e.getMessage());
}
}
/**
* 查看集群信息
*/
public static void getClusterInfo() {
List<DiscoveryNode> nodes = client.connectedNodes();
for (DiscoveryNode node : nodes) {
System.out.println("HostId:"+node.getHostAddress()+" hostName:"+node.getHostName()+" Address:"+node.getAddress());
}
}
/**
* 功能描述:新建索引
* @param indexName 索引名
*/
public static void createIndex(String indexName) {
try {
if (indexExist(indexName)) {
System.out.println("The index " + indexName + " already exits!");
} else {
CreateIndexResponse cIndexResponse = client.admin().indices()
.create(new CreateIndexRequest(indexName))
.actionGet();
if (cIndexResponse.isAcknowledged()) {
System.out.println("create index successfully!");
} else {
System.out.println("Fail to create index!");
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 功能描述:新建索引
* @param index 索引名
* @param type 类型
*/
public static void createIndex(String index, String type) {
try {
client.prepareIndex(index, type).setSource().get();
} catch (Exception e) {
System.out.println("Fail to create index!");
e.printStackTrace();
}
}
/**
* 功能描述:删除索引
* @param index 索引名
*/
public static void deleteIndex(String index) {
try {
if (indexExist(index)) {
DeleteIndexResponse dResponse = client.admin().indices().prepareDelete(index)
.execute().actionGet();
if (!dResponse.isAcknowledged()) {
logger.info("failed to delete index " + index + "!");
}else {
logger.info("delete index
ElaticSearchUtil工具类封装
最新推荐文章于 2024-08-01 21:02:03 发布
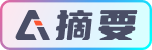