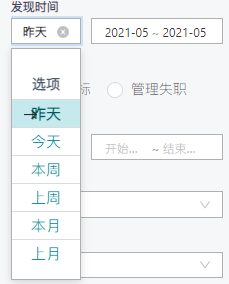
<a-form-item v-if="item.dictObjectControlTypeName === 'date'">
<template slot="label">{{ item.showName }}</template>
<div class="w100 tes-from">
<a-select
@change="changeWeek($event,item)"
allowClear
style="width: 70px;margin-right:10px"
>
<a-select-opt-group>
<div slot="label">选项</div>
<a-select-option
v-for="sfn in dictData[item.inputValueAddress]"
:value="sfn.name"
:key="sfn.id"
>
{{ sfn.name }}
</a-select-option>
</a-select-opt-group>
</a-select>
<a-range-picker
style="flex:1"
v-decorator="[item.attributeCode]"
:showToday="false"
>
</a-range-picker>
</div>
</a-form-item>
定义一个全局变量
var dateRange = {
currentDate: new Date(),
millisecond: 1000 * 60 * 60 * 24,//一天的毫秒数
getThisDay: function() {
//起止日期数组
var startStop = new Array()
var now = new Date()
now.setTime(now.getTime())
var s1 = now.getFullYear()+"-" + (now.getMonth()+1) + "-" + now.getDate()
//添加今天
startStop.push(s1) //今天起始时间
//添加今天
startStop.push(s1) //今天终止时间
//返回
return startStop;
},
getLastDay: function() {
//起止日期数组
var startStop = new Array()
var now = new Date()
now.setTime(now.getTime()-24*60*60*1000)
var s1 = now.getFullYear()+"-" + (now.getMonth()+1) + "-" + now.getDate()
//添加昨天
startStop.push(s1) //昨天起始时间
//添加昨天
startStop.push(s1) //昨天终止时间
//返回
return startStop;
},
getThisWeek: function() {
//起止日期数组
var startStop = new Array();
//返回date是一周中的某一天
var week = dateRange.currentDate.getDay();
//减去的天数
var minusDay = week != 0 ? week - 1 : 6;
//本周 周一
var monday = new Date(dateRange.currentDate.getTime() - (minusDay * dateRange.millisecond));
//本周 周日
var sunday = new Date(monday.getTime() + (6 * dateRange.millisecond));
//添加本周时间
startStop.push(dateRange.formatterDate(monday)); //本周起始时间
//添加本周最后一天时间
startStop.push(dateRange.formatterDate(sunday)); //本周终止时间
//返回
return startStop;
},
/**
* 获得上一周的起止日期
*/
getLastWeek: function() {
//起止日期数组
var startStop = new Array()
//返回date是一周中的某一天
var week = dateRange.currentDate.getDay();
//减去的天数
var minusDay = week != 0 ? week - 1 : 6;
//获得当前周的第一天
var currentWeekDayOne = new Date(dateRange.currentDate.getTime() - (dateRange.millisecond * minusDay));
//上周最后一天即本周开始的前一天
var priorWeekLastDay = new Date(currentWeekDayOne.getTime() - dateRange.millisecond);
//上周的第一天
var priorWeekFirstDay = new Date(priorWeekLastDay.getTime() - (dateRange.millisecond * 6));
startStop.push(dateRange.formatterDate(priorWeekFirstDay));
startStop.push(dateRange.formatterDate(priorWeekLastDay));
//返回
return startStop
},
/**
* 获得这个月的起止日期
*/
getThisMonth: function() {
var startStop = new Array();
//获得当前月份0-11
var currentMonth = dateRange.currentDate.getMonth();
//获得当前年份4位年
var currentYear = dateRange.currentDate.getFullYear();
//求出本月第一天
var firstDay = new Date(currentYear, currentMonth, 1);
//当为12月的时候年份需要加1
//月份需要更新为0 也就是下一年的第一个月
//否则只是月份增加,以便求的下一月的第一天
if (currentMonth == 11) {
currentYear++;
currentMonth = 0;
} else {
currentMonth++;
}
//下月的第一天
var nextMonthDayOne = new Date(currentYear, currentMonth, 1);
//求出上月的最后一天
var lastDay = new Date(nextMonthDayOne.getTime() - dateRange.millisecond);
startStop.push(dateRange.formatterDate(firstDay));
startStop.push(dateRange.formatterDate(lastDay));
//返回
return startStop;
},
/**
* 获得上个月的起止日期
*/
getLastMonth: function() {
var startStop = new Array();
//获得当前月份0-11
var currentMonth = dateRange.currentDate.getMonth();
//获得当前年份4位年
var currentYear = dateRange.currentDate.getFullYear();
var currentDay = new Date(currentYear, currentMonth, 1);
//上个月的第一天
//年份为0代表,是本年的第一月,所以不能减
if (currentMonth == 0) {
currentMonth = 11; //月份为上年的最后月份
currentYear--; //年份减1
}
else {
currentMonth--;
}
var firstDay = new Date(currentYear, currentMonth, 1);
//求出上月的最后一天
var lastDay = new Date(currentDay.getTime() - dateRange.millisecond);
startStop.push(dateRange.formatterDate(firstDay));
startStop.push(dateRange.formatterDate(lastDay));
//返回
return startStop;
},
/**
* 格式化日期(不含时间)
*/
formatterDate : function(date) {
var datetime = date.getFullYear()
+ "-"// "年"
+ ((date.getMonth() + 1) > 10 ? (date.getMonth() + 1) : "0"
+ (date.getMonth() + 1))
+ "-"// "月"
+ (date.getDate() < 10 ? "0" + date.getDate() : date
.getDate());
return datetime;
}
}
select change事件调用
import moment from 'moment'
//改变时间周
changeWeek(value,item){
var dataFrom = 'YYYY/MM/DD'
if(value == '昨天'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getLastDay()[0], dataFrom), moment(dateRange.getLastDay()[1], dataFrom)]}
)
}else if(value == '今天'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getThisDay()[0], dataFrom), moment(dateRange.getThisDay()[1], dataFrom)]}
)
}else if(value == '本周'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getThisWeek()[0], dataFrom), moment(dateRange.getThisWeek()[1], dataFrom)]}
)
}else if(value == '上周'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getLastWeek()[0], dataFrom), moment(dateRange.getLastWeek()[1], dataFrom)]}
)
}else if(value == '本月'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getThisMonth()[0], dataFrom), moment(dateRange.getThisMonth()[1], dataFrom)]}
)
}else if(value == '上月'){
this.form.setFieldsValue(
{ [item.attributeCode] : [moment(dateRange.getLastMonth()[0], dataFrom), moment(dateRange.getLastMonth()[1], dataFrom)]}
)
}
},