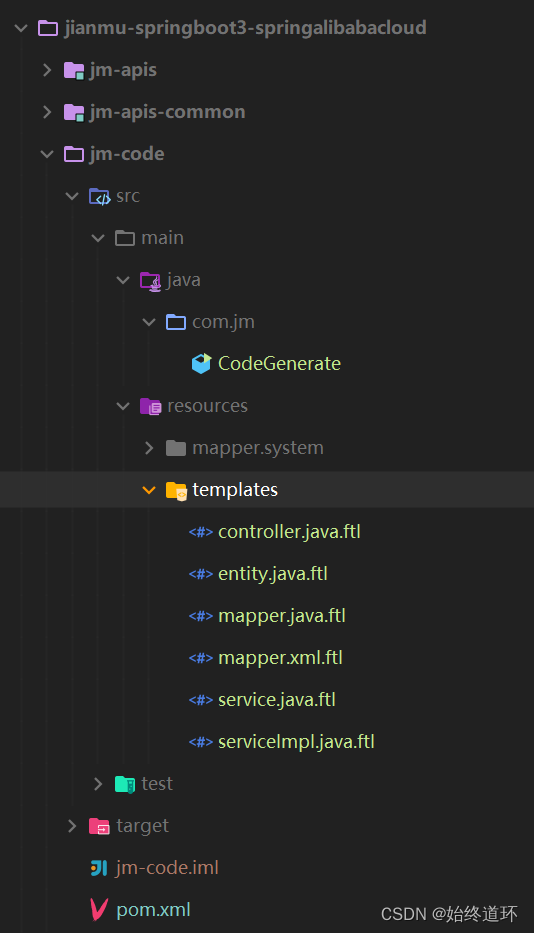
jm-code pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://maven.apache.org/POM/4.0.0"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>jianmu-springboot3-springalibabacloud</artifactId>
<groupId>org.jm</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>jm-code</artifactId>
<properties>
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>17</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.5.1</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.31</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
</dependencies>
</project>
templates controller.java.ftl
package ${package.Controller};
import org.springframework.web.bind.annotation.RequestMapping;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.web.bind.annotation.*;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
import org.springframework.web.bind.annotation.RestController;
<#if superControllerClassPackage??>
import ${superControllerClassPackage};
</#if>
@RestController
@Tag(name = "${table.comment!}管理")
@RequestMapping(SysApiConstant.SYS_API + "${controllerMappingHyphen?replace("-","_")}")
public class ${entity}Api {
private final ${table.serviceName} ${table.entityPath}Service;
@Autowired
public ${entity}Api(${table.serviceName} ${table.entityPath}Service) {
this.${table.entityPath}Service = ${table.entityPath}Service;
}
@PostMapping("/page")
@ResponseResult
@Operation(summary = "${table.comment!}列表分页查询")
public PageVO<${entity}> page(@Validated @RequestBody BasePageParam param)throws IllegalAccessException{
return this.${table.entityPath}Service.page(param);
}
@GetMapping("/get/{id}")
@ResponseResult
@Operation(summary = "${table.comment!}详情查询")
public ${entity} get(@PathVariable("id") String id){
return this.${table.entityPath}Service.get(id);
}
@PostMapping("/save")
@ResponseResult
@Operation(summary = "${table.comment!}保存")
public Boolean save(@RequestBody @Validated(SaveGroup.class) ${entity} ${table.entityPath}){
return this.${table.entityPath}Service.add(${table.entityPath});
}
@PostMapping("/modify")
@ResponseResult
@Operation(summary = "${table.comment!}修改")
public Boolean modify(@RequestBody @Validated(UpdateGroup.class) ${entity} ${table.entityPath}) {
return this.${table.entityPath}Service.modify(${table.entityPath});
}
@PostMapping("/del")
@ResponseResult
@Operation(summary = "${table.comment!}删除")
public Boolean delete(@RequestBody List<String> ids){
return this.${table.entityPath}Service.del(ids);
}
}
templates entity.java.ftl
package ${package.Entity};
<#list table.importPackages as pkg>
import ${pkg};
</#list>
<#if swagger>
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
</#if>
<#if entityLombokModel>
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
</#if>
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Null;
import com.jianmu.bean.validated.SaveGroup;
import com.jianmu.bean.validated.UpdateGroup;
<#if entityLombokModel>
@Data()
@Accessors(chain = true)
@EqualsAndHashCode(callSuper = false)
</#if>
<#if table.convert>
@TableName("${schemaName}${table.name}")
</#if>
<#if swagger>
@ApiModel(value = "${entity}对象", description = "${table.comment!}")
</#if>
<#if superEntityClass??>
public class ${entity} extends ${superEntityClass}<#if activeRecord><${entity}></#if> {
<#elseif activeRecord>
public class ${entity} extends Model<${entity}> {
<#elseif entitySerialVersionUID>
public class ${entity} implements Serializable {
<#else>
public class ${entity} {
</#if>
<#if entitySerialVersionUID>
private static final long serialVersionUID = 1L;
</#if>
<#-- ---------- BEGIN 字段循环遍历 ---------->
<#list table.fields as field>
<#if field.keyFlag>
<#assign keyPropertyName="${field.propertyName}"/>
</#if>
<#if field.comment!?length gt 0>
<#if swagger>
@ApiModelProperty("${field.comment}")
<#else>
</#if>
</#if>
<#if field.keyFlag>
<#-- 主键 -->
<#if field.keyIdentityFlag>
@TableId(value = "${field.annotationColumnName}", type = IdType.AUTO)
<#elseif idType??>
@TableId(value = "${field.annotationColumnName}", type = IdType.${idType})
<#elseif field.convert>
@TableId("${field.annotationColumnName}")
</#if>
@NotNull(groups = UpdateGroup.class, message = "id must is not null")
@Null(groups = SaveGroup.class,message = "id must is null")
<#-- 普通字段 -->
<#elseif field.fill??>
<#-- ----- 存在字段填充设置 ----->
<#if field.convert>
@TableField(value = "${field.annotationColumnName}", fill = FieldFill.${field.fill})
<#else>
@TableField(fill = FieldFill.${field.fill})
</#if>
<#elseif field.convert>
@TableField("${field.annotationColumnName}")
</#if>
<#-- 乐观锁注解 -->
<#if field.versionField>
@Version
</#if>
<#-- 逻辑删除注解 -->
<#if field.logicDeleteField>
@TableLogic
</#if>
private ${field.propertyType} ${field.propertyName};
</#list>
<#------------ END 字段循环遍历 ---------->
<#if !entityLombokModel>
<#list table.fields as field>
<#if field.propertyType == "boolean">
<#assign getprefix="is"/>
<#else>
<#assign getprefix="get"/>
</#if>
public ${field.propertyType} ${getprefix}${field.capitalName}() {
return ${field.propertyName};
}
<#if chainModel>
public ${entity} set${field.capitalName}(${field.propertyType} ${field.propertyName}) {
<#else>
public void set${field.capitalName}(${field.propertyType} ${field.propertyName}) {
</#if>
this.${field.propertyName} = ${field.propertyName};
<#if chainModel>
return this;
</#if>
}
</#list>
</#if>
<#if entityColumnConstant>
<#list table.fields as field>
public static final String ${field.name?upper_case} = "${field.name}";
</#list>
</#if>
<#if activeRecord>
@Override
public Serializable pkVal() {
<#if keyPropertyName??>
return this.${keyPropertyName};
<#else>
return null;
</#if>
}
</#if>
<#if !entityLombokModel>
@Override
public String toString() {
return "${entity}{" +
<#list table.fields as field>
<#if field_index==0>
"${field.propertyName}=" + ${field.propertyName} +
<#else>
", ${field.propertyName}=" + ${field.propertyName} +
</#if>
</#list>
"}";
}
</#if>
}
templates mapper.java.ftl
package ${package.Mapper};
import ${package.Entity}.${entity};
import ${superMapperClassPackage};
<#if mapperAnnotation>
import org.apache.ibatis.annotations.Mapper;
</#if>
import com.jianmu.config.mybatis.EnhancePlusMapper;
<#if mapperAnnotation>
@Mapper
</#if>
<#if kotlin>
interface ${table.mapperName} : ${superMapperClass}<${entity}>
<#else>
public interface ${table.mapperName} extends EnhancePlusMapper<${entity}> {
}
</#if>
templates mapper.xml.ftl
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="${package.Mapper}.${table.mapperName}">
<#if enableCache>
<cache type="${cacheClassName}"/>
</#if>
<#if baseResultMap>
<resultMap id="BaseResultMap" type="${package.Entity}.${entity}">
<#list table.fields as field>
<#if field.keyFlag><#--生成主键排在第一位-->
<id column="${field.name}" property="${field.propertyName}"/>
</#if>
</#list>
<#list table.commonFields as field><#--生成公共字段 -->
<result column="${field.name}" property="${field.propertyName}"/>
</#list>
<#list table.fields as field>
<#if !field.keyFlag><#--生成普通字段 -->
<result column="${field.name}" property="${field.propertyName}"/>
</#if>
</#list>
</resultMap>
</#if>
<#if baseColumnList>
<sql id="Base_Column_List">
<#list table.commonFields as field>
${field.columnName},
</#list>
${table.fieldNames}
</sql>
</#if>
</mapper>
templates service.java.ftl
package ${package.Service};
import ${package.Entity}.${entity};
import ${superServiceClassPackage};
import com.jianmu.bean.base.BasePageParam;
import com.jianmu.bean.base.PageVO;
import com.baomidou.mybatisplus.extension.service.IService;
import com.jianmu.config.mybatis.EnhanceService;
public interface ${table.serviceName} extends EnhanceService<${entity}> {
PageVO<${entity}> page(BasePageParam param);
}
templates serviceImpl.java.ftl
package ${package.ServiceImpl};
import ${package.Entity}.${entity};
import ${package.Mapper}.${table.mapperName};
import ${package.Service}.${table.serviceName};
import ${superServiceImplClassPackage};
import com.jianmu.bean.base.PageVO;
import org.springframework.stereotype.Service;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.jianmu.config.mybatis.EnhanceServiceImpl;
import org.apache.commons.collections4.CollectionUtils;
@Service
public class ${table.serviceImplName} extends EnhanceServiceImpl<${table.mapperName}, ${entity}> implements ${table.serviceName} {
@Autowired
public ${table.serviceImplName}(RedisTemplate<String, Object> redis, RedissonClient redissonClient){
super(redis,redissonClient);
}
@Override
public PageVO<${entity}> page(BasePageParam param){
return PageVO.convert(page(new Page<>(param.getCurrent(), param.getSize()), new LambdaQueryWrapper<>()));
}
}
生成效果
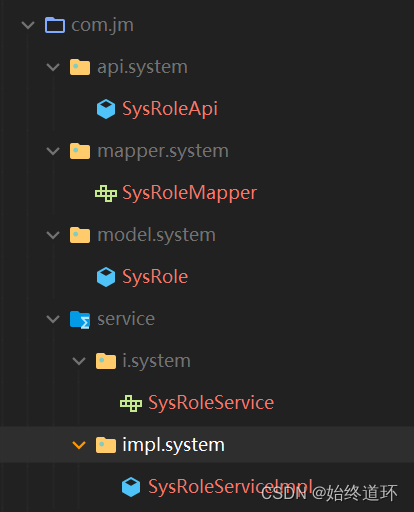
完整代码请看项目git地址
https://gitee.com/sunuping/jianmu-example-jdk17.git