听说jquery ui中dialog和easy ui中的dialog很像,因为没有用过easy ui,但在工作中有用到jquery ui中dialog,于是从官网上将例子看了下,并记录如下。
实例一
这是一个很简单的应用,打开一个对话框。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Dialog - Default functionality</title>
<link rel="stylesheet" href="jquery-ui.css">
<script src="jquery-1.10.2.js"></script>
<script src="jquery-ui.js"></script>
<link rel="stylesheet" href="style.css">
<script>
$(function() {
$( "#dialog" ).dialog();
});
</script>
</head>
<body>
<div id="dialog" title="Basic dialog">
<p>This is the default dialog which is useful for displaying information. The dialog window can be moved, resized and closed with the 'x' icon.</p>
</div>
</body>
</html>
代码$( "#dialog" ).dialog();加载页面的时候弹出一个对话框。效果如下:
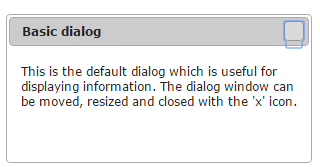
实例二
点击按钮,弹出带动画功能的对话框,定义显示和影藏效果,effect: "blind":打开时时百叶窗效果,从上到下显示,effect: "explode"关闭时是爆发,碎片效果。
这里不是页面加载的时候就显示对话框,而是点击添加账号的时候弹出这个对话框,所以要先定义这个对话框“$( "#dialog" ).dialog({,”,点击添加按钮的时候弹出对话框“dialog.dialog( "open" );”。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Dialog - Animation</title>
<link rel="stylesheet" href="jquery-ui.css">
<script src="jquery-1.10.2.js"></script>
<script src="jquery-ui.js"></script>
<link rel="stylesheet" href="style.css">
<script>
$(function() {
$( "#dialog" ).dialog({
autoOpen: false,
show: {
effect: "blind",
duration: 1000
},
hide: {
effect: "explode",
duration: 1000
}
});
$( "#opener" ).click(function() {
$( "#dialog" ).dialog( "open" );
});
});
</script>
</head>
<body>
<div id="dialog" title="Basic dialog">
<p>This is an animated dialog which is useful for displaying information. The dialog window can be moved, resized and closed with the 'x' icon.</p>
</div>
<button id="opener">Open Dialog</button>
</body>
</html>
运行效果:
实例三
作用是弹出一个模态确认对话框,mode:true,不能操作后面的父页面,除非关闭当前的对话框。在对话框上加了两个按钮,一个确认,一个取消,可以分别做一些操作,其实也不限于这两个按钮,如果需要的话可以在下面添加任意多个按钮,实现任意多的功能。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Dialog - Modal confirmation</title>
<link rel="stylesheet" href="jquery-ui.css">
<script src="jquery-1.10.2.js"></script>
<script src="jquery-ui.js"></script>
<link rel="stylesheet" href="style.css">
<script>
$(function() {
$( "#dialog-confirm" ).dialog({
resizable: false,
height:140,
modal: true,
buttons: {
"Delete all items": function() {
$( this ).dialog( "close" );
},
Cancel: function() {
$( this ).dialog( "close" );
}
}
});
});
</script>
</head>
<body>
<div id="dialog-confirm" title="Empty the recycle bin?">
<p><span class="ui-icon ui-icon-alert" style="float:left; margin:0 7px 20px 0;"></span>These items will be permanently deleted and cannot be recovered. Are you sure?</p>
</div>
<p>Sed vel diam id libero <a href="http://example.com">rutrum convallis</a>. Donec aliquet leo vel magna. Phasellus rhoncus faucibus ante. Etiam bibendum, enim faucibus aliquet rhoncus, arcu felis ultricies neque, sit amet auctor elit eros a lectus.</p>
</body>
</html>
运行效果:
实例四
带form功能的对话框,这个例子有些复杂,可以实现验证,请求等功能。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Dialog - Modal form</title>
<link rel="stylesheet" href="jquery-ui.css">
<script src="jquery-1.10.2.js"></script>
<script src="jquery-ui.js"></script>
<link rel="stylesheet" href="style.css">
<style>
body { font-size: 62.5%; }
label, input { display:block; }
input.text { margin-bottom:12px; width:95%; padding: .4em; }
fieldset { padding:0; border:0; margin-top:25px; }
h1 { font-size: 1.2em; margin: .6em 0; }
div#users-contain { width: 350px; margin: 20px 0; }
div#users-contain table { margin: 1em 0; border-collapse: collapse; width: 100%; }
div#users-contain table td, div#users-contain table th { border: 1px solid #eee; padding: .6em 10px; text-align: left; }
.ui-dialog .ui-state-error { padding: .3em; }
.validateTips { border: 1px solid transparent; padding: 0.3em; }
</style>
<script>
$(function() {
var dialog, form,
// From http://www.whatwg.org/specs/web-apps/current-work/multipage/states-of-the-type-attribute.html#e-mail-state-%28type=email%29
emailRegex = /^[a-zA-Z0-9.!#$%&'*+\/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/,
name = $( "#name" ),
email = $( "#email" ),
password = $( "#password" ),
allFields = $( [] ).add( name ).add( email ).add( password ),
tips = $( ".validateTips" );
function updateTips( t ) {
tips
.text( t )
.addClass( "ui-state-highlight" );
setTimeout(function() {
tips.removeClass( "ui-state-highlight", 1500 );
}, 500 );
}
function checkLength( o, n, min, max ) {
if ( o.val().length > max || o.val().length < min ) {
o.addClass( "ui-state-error" );
updateTips( "Length of " + n + " must be between " +
min + " and " + max + "." );
return false;
} else {
return true;
}
}
function checkRegexp( o, regexp, n ) {
if ( !( regexp.test( o.val() ) ) ) {
o.addClass( "ui-state-error" );
updateTips( n );
return false;
} else {
return true;
}
}
function addUser() {
var valid = true;
allFields.removeClass( "ui-state-error" );
valid = valid && checkLength( name, "username", 3, 16 );
valid = valid && checkLength( email, "email", 6, 80 );
valid = valid && checkLength( password, "password", 5, 16 );
valid = valid && checkRegexp( name, /^[a-z]([0-9a-z_\s])+$/i, "Username may consist of a-z, 0-9, underscores, spaces and must begin with a letter." );
valid = valid && checkRegexp( email, emailRegex, "eg. ui@jquery.com" );
valid = valid && checkRegexp( password, /^([0-9a-zA-Z])+$/, "Password field only allow : a-z 0-9" );
if ( valid ) {
$( "#users tbody" ).append( "<tr>" +
"<td>" + name.val() + "</td>" +
"<td>" + email.val() + "</td>" +
"<td>" + password.val() + "</td>" +
"</tr>" );
dialog.dialog( "close" );
}
return valid;
}
dialog = $( "#dialog-form" ).dialog({
autoOpen: false,
height: 300,
width: 350,
modal: true,
buttons: {
"Create an account": addUser,
Cancel: function() {
dialog.dialog( "close" );
}
},
close: function() {
form[ 0 ].reset();
allFields.removeClass( "ui-state-error" );
}
});
form = dialog.find( "form" ).on( "submit", function( event ) {
event.preventDefault();
addUser();
});
$( "#create-user" ).button().on( "click", function() {
dialog.dialog( "open" );
});
});
</script>
</head>
<body>
<div id="dialog-form" title="Create new user">
<p class="validateTips">All form fields are required.</p>
<form>
<fieldset>
<label for="name">Name</label>
<input type="text" name="name" id="name" value="Jane Smith" class="text ui-widget-content ui-corner-all">
<label for="email">Email</label>
<input type="text" name="email" id="email" value="jane@smith.com" class="text ui-widget-content ui-corner-all">
<label for="password">Password</label>
<input type="password" name="password" id="password" value="xxxxxxx" class="text ui-widget-content ui-corner-all">
<!-- Allow form submission with keyboard without duplicating the dialog button -->
<input type="submit" tabindex="-1" style="position:absolute; top:-1000px">
</fieldset>
</form>
</div>
<div id="users-contain" class="ui-widget">
<h1>Existing Users:</h1>
<table id="users" class="ui-widget ui-widget-content">
<thead>
<tr class="ui-widget-header ">
<th>Name</th>
<th>Email</th>
<th>Password</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>john.doe@example.com</td>
<td>johndoe1</td>
</tr>
</tbody>
</table>
</div>
<button id="create-user">Create new user</button>
</body>
</html>
运行效果:
点击添加用户弹出对话框
元素验证失败时
这个带请求功能的对话框,代码很严谨,例子很经典!
var dialog, form, emailRegex = /^[a-zA-Z0-9.!#$%&'*+\/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/, name = $( "#name" ), email = $( "#email" ), password = $( "#password" ), allFields = $( [] ).add( name ).add( email ).add( password ), tips = $( ".validateTips" );
定义元素变量,要用到的验证,allFields = $( [] ).add( name ).add( email ).add( password ),这个用来把要用到的变量加到集合里面,用来添加和删除css样式,后面会用到的,很巧妙,$( [] )这个写法可以把多个元素添加到一个集合中。
function updateTips( t ) { tips .text( t ) .addClass( "ui-state-highlight" ); setTimeout(function() { tips.removeClass( "ui-state-highlight", 1500 ); }, 500 ); }
给元素添加文字和css样式,并且在500毫秒内移除这个样式。
function checkLength( o, n, min, max ) { if ( o.val().length > max || o.val().length < min ) { o.addClass( "ui-state-error" ); updateTips( "Length of " + n + " must be between " + min + " and " + max + "." ); return false; } else { return true; } }
检查输入文字的长短,4个参数分别是元素本身,元素名称,最小长度,最大长度。
function checkRegexp( o, regexp, n ) { if ( !( regexp.test( o.val() ) ) ) { o.addClass( "ui-state-error" ); updateTips( n ); return false; } else { return true; } }
用正则表达式检查元素是否符合规则,3个参数,元素本身,正则表达式,错误提示。
function addUser() { var valid = true; allFields.removeClass( "ui-state-error" ); valid = valid && checkLength( name, "username", 3, 16 ); valid = valid && checkLength( email, "email", 6, 80 ); valid = valid && checkLength( password, "password", 5, 16 ); valid = valid && checkRegexp( name, /^[a-z]([0-9a-z_\s])+$/i, "Username may consist of a-z, 0-9, underscores, spaces and must begin with a letter." ); valid = valid && checkRegexp( email, emailRegex, "eg. ui@jquery.com" ); valid = valid && checkRegexp( password, /^([0-9a-zA-Z])+$/, "Password field only allow : a-z 0-9" ); if ( valid ) { $( "#users tbody" ).append( "<tr>" + "<td>" + name.val() + "</td>" + "<td>" + email.val() + "</td>" + "<td>" + password.val() + "</td>" + "</tr>" ); dialog.dialog( "close" ); } return valid; }
添加用户的方法,验证方法valid = valid && 这个用的很巧妙,连续利用了&&操作,这里的写法非常的灵活也很巧妙。在这里可以实现具体的功能,也可以在form里面添加action,form到具体页面中操作。
dialog = $( "#dialog-form" ).dialog({ autoOpen: false, height: 300, width: 350, modal: true, buttons: { "Create an account": addUser, Cancel: function() { dialog.dialog( "close" ); } }, close: function() { form[ 0 ].reset(); allFields.removeClass( "ui-state-error" ); } });
这里是最主要的功能,一个定义一个对话框,其中有个2个按钮,一个是添加一个账号,访问addUser函数,一个是取消,直接定义关闭对话框,最后还定义了默认的关闭功能,form中清除元素的值,清除错误提示。
form = dialog.find( "form" ).on( "submit", function( event ) { event.preventDefault(); addUser(); });
定义form,其实就是页面中的form,还定义了提交时请求动作,首先取消默认事件,然后执行addUser。
$( "#create-user" ).button().on( "click", function() { dialog.dialog( "open" ); });
给添加用户按钮绑定事件,这里绑定事件没有直接.click,也没有live,也没有trigger,on是jquery官方推荐的一个绑定函数方法。注意这里不是页面加载的时候就显示对话框,而是点击添加账号的时候弹出这个对话框,所以要先定义这个对话框“dialog = $( "#dialog-form" ).dialog({”,点击添加按钮的时候弹出对话框“dialog.dialog( "open" );”。
实例五
模态对话框,作用是弹出一个模态对话框,mode:true,其实就是后面不能回到后面的父页面,除非关闭当前的对话框。在对话框后面加了一个OK关闭按钮。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery UI Dialog - Modal message</title>
<link rel="stylesheet" href="jquery-ui.css">
<script src="jquery-1.10.2.js"></script>
<script src="jquery-ui.js"></script>
<link rel="stylesheet" href="style.css">
<script>
$(function() {
$( "#dialog-message" ).dialog({
modal: true,
buttons: {
Ok: function() {
$( this ).dialog( "close" );
}
}
});
});
</script>
</head>
<body>
<div id="dialog-message" title="Download complete">
<p>
<span class="ui-icon ui-icon-circle-check" style="float:left; margin:0 7px 50px 0;"></span>
Your files have downloaded successfully into the My Downloads folder.
</p>
<p>
Currently using <b>36% of your storage space</b>.
</p>
</div>
<p>Sed vel diam id libero <a href="http://example.com">rutrum convallis</a>. Donec aliquet leo vel magna. Phasellus rhoncus faucibus ante. Etiam bibendum, enim faucibus aliquet rhoncus, arcu felis ultricies neque, sit amet auctor elit eros a lectus.</p>
</body>
</html>
运行效果: