20个Python自动化办公神器:让你的工作与学习更高效!
今天我们将探讨20个Python自动化办公技巧,这些技巧适用于学生和职场人士,可以让你的工作和学习变得更加高效。下面我们一起来看看这些神奇的技巧以及具体的实现代码吧!
1. 批量修改文件名
有时候我们需要批量修改文件名,例如将一堆图片按顺序编号。使用Python可以轻松实现。
import os
folder = "path/to/your/folder" # 文件夹路径
prefix = "Image" # 新文件名前缀
for index, filename in enumerate(os.listdir(folder)):
os.rename(os.path.join(folder, filename), os.path.join(folder, f"{prefix}_{index}.jpg"))
2. 合并PDF文件
当你需要将多个PDF文件合并成一个文件时,可以使用Python的PyPDF2
库。
import PyPDF2
input_files = ["file1.pdf", "file2.pdf"] # 输入文件列表
output_file = "merged.pdf" # 输出文件
pdf_merger = PyPDF2.PdfFileMerger()
for file in input_files:
pdf_merger.append(file)
pdf_merger.write(output_file)
pdf_merger.close()
3. 批量压缩图片
有时候我们需要压缩图片以节省空间,可以使用Python的PIL
库。
from PIL import Image
def compress_image(input_file, output_file, quality):
img = Image.open(input_file)
img.save(output_file, quality=quality)
input_file = "example.jpg"
output_file = "compressed.jpg"
quality = 80 # 质量,1-100之间,数值越低压缩率越高
compress_image(input_file, output_file, quality)
4. 网络爬虫
网络爬虫可以帮助你快速获取网页上的信息。使用Python的requests
和BeautifulSoup
库,你可以轻松实现。
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# 提取网页上的信息,例如提取所有的链接
links = [a.get("href") for a in soup.find_all("a")]
5. 发送邮件
使用Python的smtplib
库可以轻松实现发送邮件的功能。
import smtplib
def send_email(subject, body, to_email, from_email, password):
message = f"Subject: {subject}\n\n{body}"
with smtplib.SMTP_SSL("smtp.gmail.com", 465) as server:
server.login(from_email, password)
server.sendmail(from_email, to_email, message)
subject = "Hello"
body = "This is a test email."
to_email = "recipient@example.com"
from_email = "your_email@example.com"
password = "your_password"
send_email(subject, body, to_email, from_email, password)
6. Excel操作
使用Python的openpyxl
库可以轻松读取和修改Excel文件。
import openpyxl
# 读取Excel文件
workbook = openpyxl.load_workbook("example.xlsx")
sheet = workbook.active
# 获取单元格的值
cell_value = sheet["A1"].value
# 修改单元格的值
sheet["A1"].value = "New Value"
# 保存文件
workbook.save("output.xlsx")
7. Word操作
使用Python的python-docx
库可以轻松读取和修改Word文档。
import docx
# 读取Word文件
doc = docx.Document("example.docx")
# 获取段落文字
paragraphs = [para.text for para in doc.paragraphs]
# 添加段落
doc.add_paragraph("New paragraph")
# 保存文件
doc.save("output.docx")
8. PowerPoint操作
使用Python的python-pptx
库可以轻松读取和修改PowerPoint文件。
from pptx import Presentation
# 读取PPT文件
ppt = Presentation("example.pptx")
# 获取幻灯片数量和标题
slide_count = len(ppt.slides)
titles = [slide.shapes.title.text for slide in ppt.slides]
# 添加新的幻灯片
slide_layout = ppt.slide_layouts[0] # 选择布局
new_slide = ppt.slides.add_slide(slide_layout)
new_slide.shapes.title.text = "New Slide"
# 保存文件
ppt.save("output.pptx")
9. 读写CSV文件
使用Python的csv
库可以轻松读取和写入CSV文件。
import csv
# 读取CSV文件
with open("example.csv", "r") as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
# 写入CSV文件
data = [["Name", "Age"], ["Alice", 30], ["Bob", 25]]
with open("output.csv", "w") as csvfile:
writer = csv.writer(csvfile)
for row in data:
writer.writerow(row)
10. 计划任务
使用Python的schedule
库可以定时执行任务。
import schedule
import time
def job():
print("Task executed!")
# 每隔10秒执行一次任务
schedule.every(10).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
11. 语音识别
使用Python的speech_recognition
库可以将语音转换为文本。
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Say something...")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio)
print("You said: ", text)
except sr.UnknownValueError:
print("Google Speech Recognition could not understand audio")
except sr.RequestError as e:
print("Could not request results from Google Speech Recognition service; {0}".format(e))
12. 文本翻译
使用Python的googletrans
库可以实现文本翻译。
from googletrans import Translator
translator = Translator()
translated = translator.translate("Hello, world!", dest="zh-CN")
print(translated.text)
13. 字符串模板
使用Python的string
库可以轻松生成动态字符串。
from string import Template
template = Template("Hello, ${name}!")
message = template.substitute(name="John")
print(message)
14. 二维码生成
使用Python的qrcode
库可以生成二维码。
import qrcode
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data("https://example.com")
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="white")
img.save("qrcode.png")
15. 数据可视化
使用Python的matplotlib
库可以绘制图表。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.title("Line Plot")
plt.show()
16. JSON操作
使用Python的json
库可以轻松读写JSON文件。
import json
# 读取JSON文件
with open("example.json", "r") as file:
data = json.load(file)
# 写入JSON文件
new_data = {"name": "John", "age": 30}
with open("output.json", "w") as file:
json.dump(new_data, file)
17. 文件差异比较
使用Python的difflib
库可以比较两个文件的差异。
import difflib
with open("file1.txt", "r") as file:
file1_data = file.readlines()
with open("file2.txt", "r") as file:
file2_data = file.readlines()
differ = difflib.Differ()
diff = list(differ.compare(file1_data, file2_data))
with open("diff.txt", "w") as file:
file.writelines(diff)
18. 自动化测试
使用Python的unittest
库可以实现自动化测试。
import unittest
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual("hello".upper(), "HELLO")
def test_isupper(self):
self.assertTrue("HELLO".isupper())
self.assertFalse("Hello".isupper())
def test_split(self):
s = "hello world"
self.assertEqual(s.split(), ["hello", "world"])
with self.assertRaises(TypeError):
s.split(2)
if __name__ == "__main__":
unittest.main()
19. 文件备份
使用Python可以轻松实现文件备份功能。
import shutil
source_file = "example.txt"
backup_folder = "backup"
shutil.copy(source_file, backup_folder)
20. 自动化打包
使用Python可以自动化打包你的项目。
import os
import zipfile
folder = "path/to/your/folder"
zip_filename = "output.zip"
with zipfile.ZipFile(zip_filename, "w", compression=zipfile.ZIP_DEFLATED) as zip_file:
for root, dirs, files in os.walk(folder):
for file in files:
zip_file.write(os.path.join(root, file))
以上就是20个Python自动化办公技巧,学习以上技巧能大大提高工作效率,成为最早摸鱼的职场小渔夫(不是),成为效率蹭蹭涨的小能手!
关于Python学习指南
学好 Python 不论是就业还是做副业赚钱都不错,但要学会 Python 还是要有一个学习规划。最后给大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
包括:Python激活码+安装包、Python web开发,Python爬虫,Python数据分析,人工智能、自动化办公等学习教程。带你从零基础系统性的学好Python!
👉Python所有方向的学习路线👈
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。(全套教程文末领取)
👉Python学习视频600合集👈
观看零基础学习视频,看视频学习是最快捷也是最有效果的方式,跟着视频中老师的思路,从基础到深入,还是很容易入门的。
温馨提示:篇幅有限,已打包文件夹,获取方式在:文末
👉Python70个实战练手案例&源码👈
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
👉Python大厂面试资料👈
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
👉Python副业兼职路线&方法👈
学好 Python 不论是就业还是做副业赚钱都不错,但要学会兼职接单还是要有一个学习规划。
👉 这份完整版的Python全套学习资料已经上传,朋友们如果需要可以扫描下方CSDN官方认证二维码或者点击链接免费领取【保证100%免费
】
点击免费领取《CSDN大礼包》:Python入门到进阶资料 & 实战源码 & 兼职接单方法 安全链接免费领取
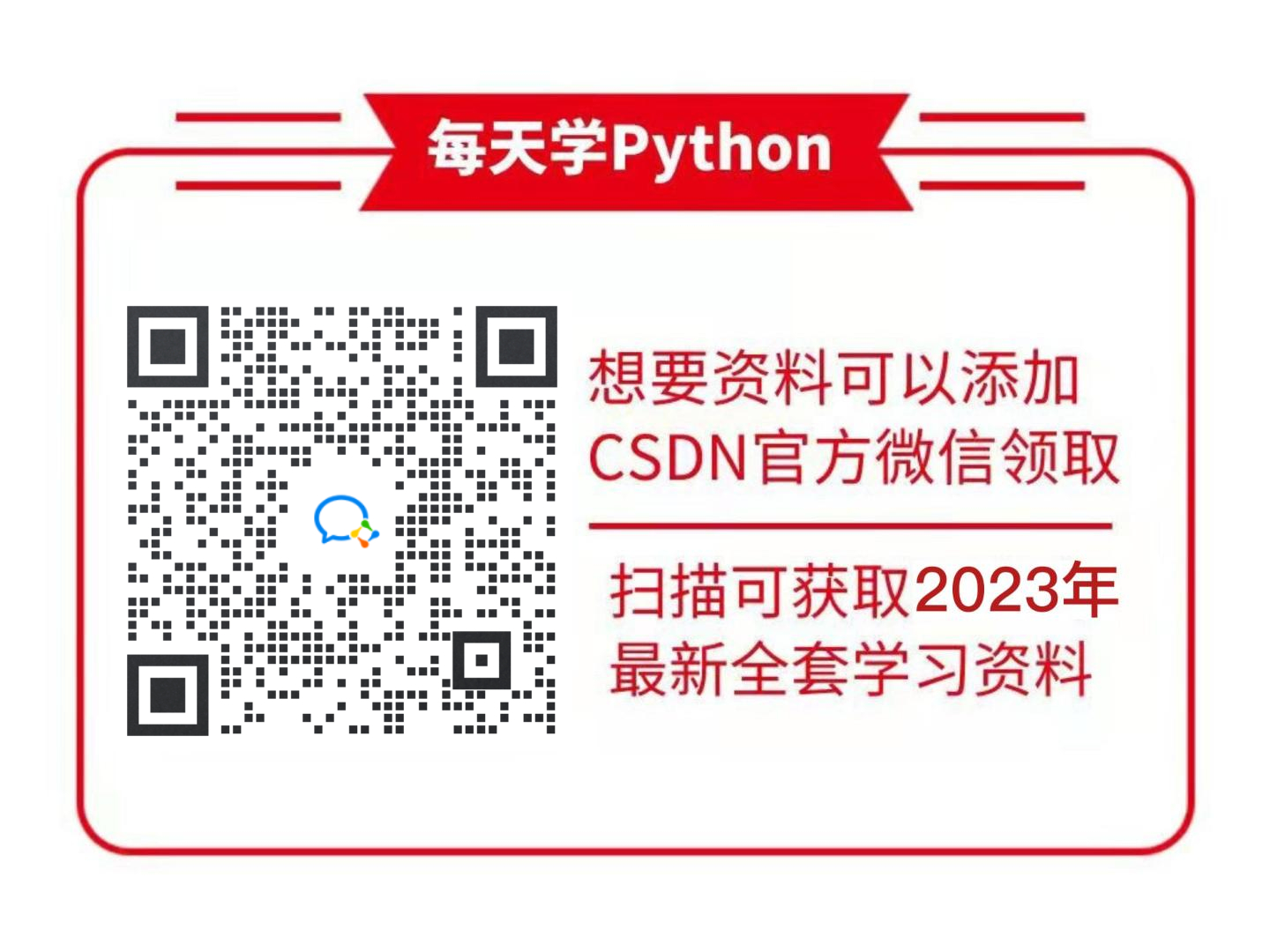