java实现一个wordle猜单词的游戏(使用java swing gui技术)
一.wordle是一个爆火的国外游戏 ,具体来说它有以下几个规则
1.每次输入反馈
2.输入六次没有猜对 输出这个失败的信息 然后提示是否重新猜测或者退出
3.输入六次以内猜对 输出成功信息 然后提示是否重新猜测或者退出
4.输入非字母 输出警告
5.输入少于5个字母 输出警告
6.输入多于六个字母 输出警告
7.随机抽取 从txt或者csv或者excel文档
8.同一个字母 同一个位置 显示绿色 存在该字母不同位置显示黄色 不存在该字母 显示灰色
二.参考图片
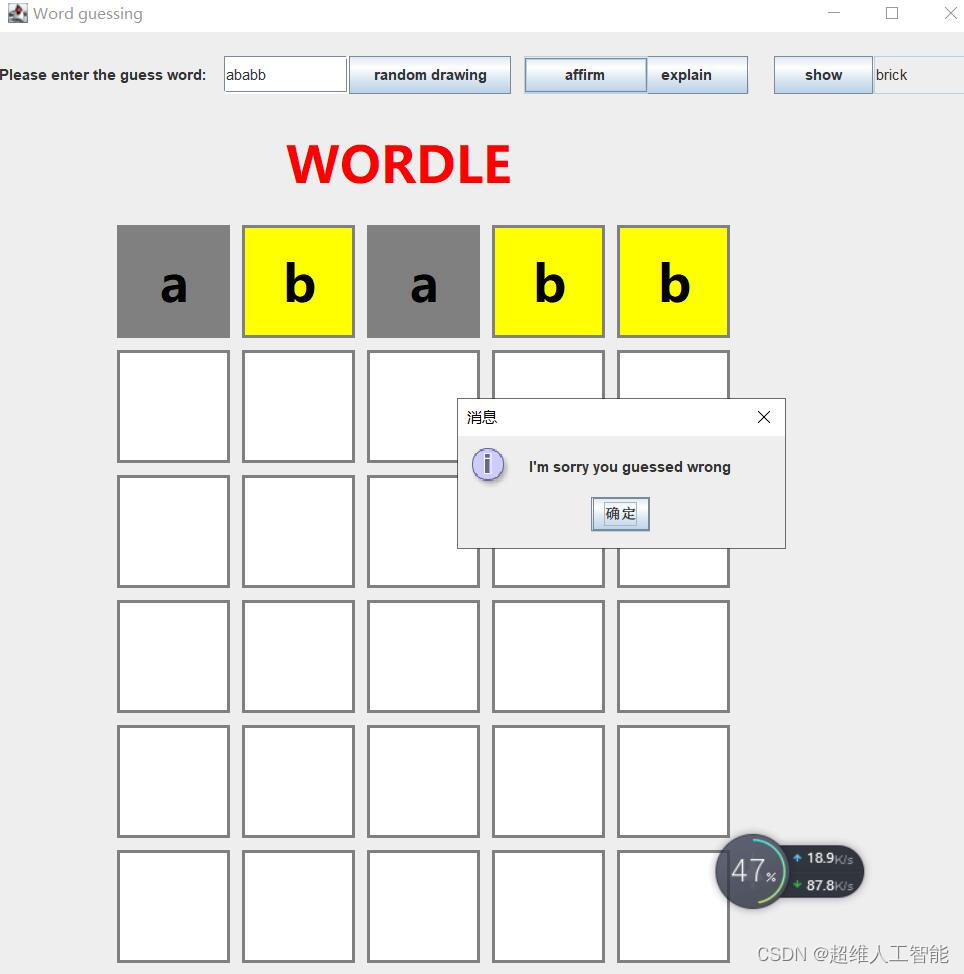
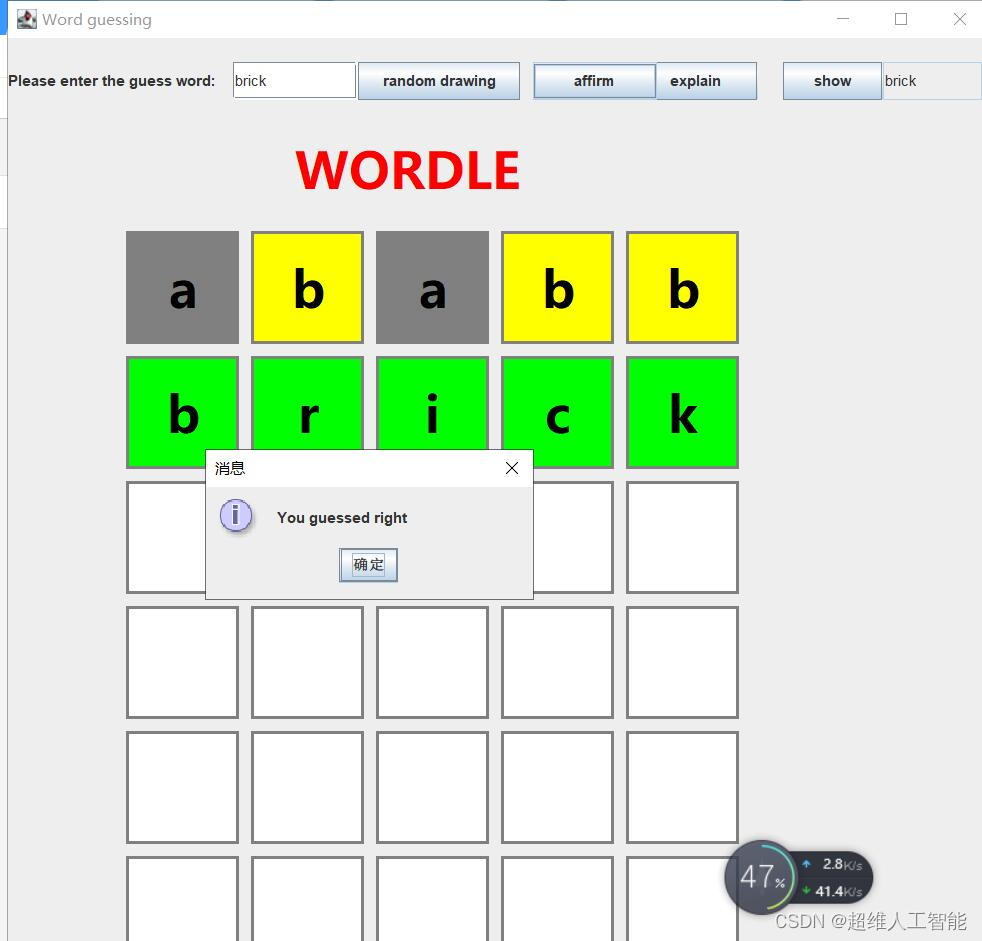
二.参考视频
wordle代码运行和讲解视频链接