public class RecurTheTreeNode {
//非递归遍历
public void preOrderRecur(TreeNode head){
if(head == null) return;
System.out.println(head.val);
preOrderRecur(head.left);
preOrderRecur(head.right);
}
public void inOrderRecur(TreeNode head){
if(head == null) return;
inOrderRecur(head.left);
System.out.println(head.val);
inOrderRecur(head.right);
}
public void posOrderRecur(TreeNode head){
if(head == null) return;
posOrderRecur(head.left);
posOrderRecur(head.right);
System.out.println(head.val);
}
//非递归遍历:对于非递归遍历,一般用栈来实现,因为栈能够实现节点的重复访问多次。
//递归的方法无非就是用函数栈来实现保存信息
public void preOrderUnRecur(TreeNode head){
if(head == null) return;
Stack<TreeNode> stack = new Stack<>();
stack.push(head);
TreeNode cur = null;
while(!stack.isEmpty()){
cur = stack.pop();
System.out.println(cur.val);
if(cur.right != null){
//把最后输出的信息先压栈
stack.push(cur.right);
}
if(cur.left != null){
stack.push(cur.left);
}
}
}
//中序遍历
public void inOrderUnRecur(TreeNode head){
if(head == null){
return;
}
Stack<TreeNode> stack = new Stack<>();
TreeNode cur = null;
while(!stack.isEmpty() || head != null){
if(head != null){
head = head.left;
} else {
cur = stack.pop();
System.out.println(cur.val);
head = cur.right;
}
}
}
//二叉树的后续遍历双栈和单栈遍历
//前序遍历,先遍历中间部分再遍历左后右, 那么用两个栈先遍历中间,先右再左,倒过来就是后续遍历
public void posOrderUnRecur(TreeNode head){
if(head == null) return;
Stack<TreeNode> stack1 = new Stack<>();
Stack<TreeNode> stack2 = new Stack<>();
TreeNode cur = null;
stack1.push(head.left);
while(!stack1.isEmpty()){
cur = stack1.pop();
stack2.push(cur);
if(cur.left != null){
stack1.push(cur.left);
}
if(cur.right != null){
stack1.push(cur.right);
}
}
while(!stack2.isEmpty()){
System.out.println(stack2.pop() + " ");
}
}
//单栈 对于单栈解法要经过一个节点3次
//对于头节点为最后遍历的节点,所以此前已经遍历过左右孩子,遍历的过程会经过两次该节点,
//二我们遍历完孩子向上走的过程一般为设定一个节点为孩子,如果该节点是头节点的子节点则不进入该节点
public void posOrderUnRecur2(TreeNode head){
if(head == null){
return;
}
Stack<TreeNode> stack = new Stack<>();
stack.push(head);
TreeNode cur = null;
while(!stack.isEmpty()){
//该节点要经过好几次,所以以peek的形式弹出
cur = stack.peek();
//保证压入左孩子
if(cur.left != null && cur.left != head && cur.right != head){
stack.push(cur.left);
//保证压入右孩子
} else if(cur.right != null && cur.right != head){
stack.push(cur.right);
} else {
//当左右为空打印左右 或者左右已经弹出过时候打印头节点
System.out.println(cur.val);
head = cur;
}
}
}
}
二次树的前中后序遍历
最新推荐文章于 2022-12-09 00:28:25 发布
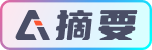