1
//1.输入 x 的值,计算出相相应的 y 值。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int x, y;
while (scanf("%d", &x))
{
if (x < 5)
{
y = x;
}else if (5 <= x && x < 15) {
y = x + 6;
}else {
y = x - 6;
}
printf("%d\n", y);
}
}
2
//2. 输入一个小写字母,将其转换为大写字母。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
char a;
while (scanf(" %c",&a))//注意加空格
{
printf("%c\n", a-32);
}
}
3
//3.求一个 3×3 矩阵主对角线元素之和。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int a[3][3];
int i, j,b,sum;
for (i = 0; i < 3; i++)
{
for (j = 0; j < 3; j++)
{
scanf("%d", &b);
a[i][j] = b;
printf("%3d", b);
}
printf("\n");
}
sum = a[0][0] + a[1][1] + a[2][2];
printf("%d\n", sum);
}
4
//4. 求 100~999 之间的水仙花数。
//所谓水仙花数,是指一个 3 位数,它的每位数 字的立方之和等于该数。
//例如,因为 153 = 1 + 125 + 27,所以 153 为水仙花数。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int a;
int b, c, d;//用来存百位,十位,个位
for(a=100;a<=999;a++)
{
b = a / 100;//存百位
c = (a % 100) / 10;//c存十位
d = a % 10;//存个位
if (a == (b*b*b+c*c*c+d*d*d))
{
printf("%d\n", a);
}
}
}
5
//5. 输入百分制成绩,并把它转换成五级分制。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int grade;
while (scanf("%d", &grade))
{
if (90 < grade && grade < 100)
{
printf("A\n");
}else if (80 < grade && grade < 89){
printf("B\n");
}else if (70 < grade && grade < 79) {
printf("C\n");
}
else if (60 < grade && grade < 69) {
printf("D\n");
}
}
}
6
//6. 斐波拉契数列递归实现的方法如下
//请问,如何不使用递归,来实现上述函数?
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int a = 0, b = 1, i = 2 , sum, n;//斐波那契第n项
while (scanf("%d", &n))
{
if (1 == n) printf("斐波那契第%d项=%d\n", n, 0);
if (2 == n) printf("斐波那契第%d项=%d\n", n, 1);
if (n > 2)
{
while (i < n)
{
sum = a + b;
a = b;
b = sum;
i++;
}
printf("斐波那契第%d项=%d\n", n, sum);
}
}
}
7
//7. 编写一个程序,将一个数组中的值按逆序重新存放。
//例如, 原来顺序为8, 6, 5, 4, 1。要求改为 1, 4, 5, 6, 8
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
//数组初始化
int a[5], i = 0, b;
while (i < 5)
{
scanf("%d", &b);
a[i] = b;
i++;
//printf("%3d",a[i]);
//此时打印输出会错误,需要全部初始化完成才可以打印
}
for (i = 0; i < 5; i++)
{
printf("%3d", a[i]);
}
printf("\n");
//逆序
int* p;
p = a+4;
int c[5];
for (i = 0; i < 5 ;i++)
{
c[i] = *(p - i);
}
for (i = 0; i < 5; i++)
{
printf("%3d", c[i]);
}
printf("\n");
}
8
//8. 编写一个程序根据输入的三角形的三条边判断是否能组成三角形,如果可以
//则输出它的面积和三角形类型(等边、等腰、直角三角形)。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int a, b, c;
while (scanf("%d%d%d", &a, &b, &c))
{
if ((a + b) > c && (a + c) > b && (b + c) > a)
{
printf("可以组成三角形\n");
if (a == b && a == c && b == c)
{
printf("等边三角形\n");
}
else if (a == b || a == c || b == c) {
printf("等腰三角形\n");
}
else if (a ^ 2 + b ^ 2 == c ^ 2 || b ^ 2 + c ^ 2 == a ^ 2 || a ^ 2 + c ^ 2 == b ^ 2)
{
printf("直角三角形\n");
}
//求面积 海伦-秦九公式
double s,p;
p = (a + b + c) / 2;
s = sqrt(p * (p - a) * (p - b) * (p - c));
printf("三角形的面积是%.2lf\n", s);
}
else
{
printf("不可以组成三角形\n");
}
}
}
9
//9. 从键盘输入若干个学生成绩,统计并输出最高成绩和最低成绩,当输入负数时结束输入。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int score, high=0, low=1000;
while (scanf("%d", &score))
{
if (score < 0) break;
if (score > high)
{
high = score;
}
if (score < low)
{
low = score;
}
printf("当前最高成绩为%d\n", high);
printf("当前最低成绩为%d\n", low);
}
}
10
//10. 编写函数将化氏温度转换为摄氏温度,公式为 C=(F-32)*5/9;并在主函数中调用。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
float tmp(float F)
{
float C;
C = (F - 32) * 5 / 9;
printf("摄氏温度为%.2f\n", C);
}
int main()
{
float F;
scanf("%f", &F);
tmp(F);
}
11
//11. 输入一个自然数,输出其各因子的连乘形式,如输入12,则输出12 = 1 * 2 * 2 * 3。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int factor[100] = { 0 };
//因子
int factor_try(int n)
{
int count = 0;
int m = 2;//试验因子
for (m; m <= n;)
{
if (1 == n / m && n % m == 0)
{
break;
}
if (0 == n % m)
{
factor[count] = m;
n = n / m;
++count;
m = 2;
}
else {
m++;
}
}
factor[count] = m;
}
//打印因子
void printf_factor(int n)
{
int index = 0;
printf("%d=1*", n);
while (0 != factor[index])
{
printf("%d", factor[index]);
if (factor[index + 1] == 0)
{
break;
}
printf("*");
++index;
}
}
int main()
{
int n;
scanf("%d", &n);
factor_try(n);
printf_factor(n);
return 0;
}
12
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ARR_SIZE(arr) (sizeof(arr) / sizeof(arr[0]))
/*
N 个整数从小到大排列,输入一个新数插入其中,使 N+1 个整数仍然有序
*/
void Bubble_sort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main(void) {
int nums[5] = { 2, 3, 7, 9, 10 };
int nums1[6] = { 2,3,7,9,10,4 };//插入元素4
Bubble_sort(nums1, 6);//这里选择冒泡排序
for (int i = 0; i < 6; i++) {
printf("%3d", nums1[i]);
}
return 0;
}
13
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ARR_SIZE(arr) (sizeof(arr) / sizeof(arr[0]))
/*
在 100~200 之间找出满足用 3 除余 2,用 5 除余 3 和用 7 除余 2 的所有整数
*/
int main(void) {
for (int n = 100; n <= 200; n++) {
if ((n % 3 == 2) && (n % 5 == 3) && (n % 7 == 2)) {
printf("%3d ", n);
}
}
return 0;
}
14
//14. 输入 10 个同学的成绩,统计 80 分以上和不及格的人数,并输出平均分
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int score[10] = {0};
int ScoreTotal()
{
int n = 9;
int STscore = 0;//学生分数
int total = 0;
while (n>=0)
{
scanf("%d", &STscore);
score[n] = STscore;
total += score[n];
n--;
}
return total;
}
int main()
{
int T=ScoreTotal();
int count1 = 0,count2=0;//count1统计不及格人数
//count2统计80分以上人数
int i = 0;
for (i; i < 10; i++)
{
if (score[i] < 60)
{
count1++;
}
if (score[i] > 80)
{
count2++;
}
}
printf("不及格的人数:%d\n", count1);
printf("80分以上的人数:%d\n", count2);
printf("平均分:%d\n", T / 10);
return 0;
}
15
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define ARR_SIZE(arr) (sizeof(arr) / sizeof(arr[0]))
/*
编写一个函数来检验输入的一个字符是大写字母还是小写字母或不是 26 英文字母。
*/
int main(void) {
char ch;
scanf("%c", &ch);//输入c/A/1验证
if (isalpha(ch)) {
printf("%c 是字母\n", ch);
if (isupper(ch)) {
printf("%c 是大写字母\n", ch);
}else {
printf("%c 是小写字母\n", ch);
}
}else {
printf("%c 不是字母\n", ch);
}
return 0;
}
16
//16. 编写一个程序,从键盘输入半径和高,输出圆柱体的底面积和体积。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
double r, h;
scanf("%lf %lf", &r, &h);
double S = r * r * 3.14;
double V = S * h;
printf("S=%.2lf\n", S);
printf("V=%.2lf\n", V);
}
17
//17. 输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <ctype.h>
/*
fgets 会将输入中的换行符 '\n' 作为字符串的一部分进行读取
fgets 会在读取到换行符时停止读取,并在其后添加字符串结束符 '\0'。
*/
int main(void) {
char str[1000];
int letters = 0, spaces = 0, digits = 0, others = 0;
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) {
letters++;
}
else if (isspace(str[i])) {//isspace 函数遇到‘\n’,会返回非零值(通常是 1)。导致在统计空格时也将换行符计算在内。
spaces++;
}
else if (isdigit(str[i])) {
digits++;
}
else {
others++;
}
}
printf("英文字母: %d\n", letters);
printf("空格: %d\n", spaces);
printf("数字: %d\n", digits);
printf("其他字符: %d\n", others);
return 0;
}
18
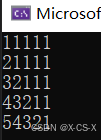
// 18.打印如下图案
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main(void) {
char nums[6][6];
//初始化二维数组
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++) {
nums[i][j] = 1;
}
}
for (int i = 0; i < 5; i++) { //行
int num = i + 1;
for (int k = 0; k < i; k++) { //列
nums[i][k] = num;
num--;
}
}
//打印
for (int m = 0; m < 5; m++)
{
for (int n = 0; n < 5; n++)
{
printf("%d", nums[m][n]);
}
printf("\n");
}
printf("\n");
return 0;
}
21
/*21. 编写一个函数,要求输入年月日时分秒,输出该年月日时分秒的下一秒。如
输入 2004 年 12 月 31 日 23 时 59 分 59 秒,则输出 2005 年 1 月 1 日 0 时 0 分 0
秒。*/
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdbool.h>
// 判断年份是否为闰年
bool is_leap_year(int year) {
// 如果年份能被400整除,则为闰年
// 或者如果年份能被4整除但不能被100整除,也是闰年
return (year % 400 == 0) || (year % 4 == 0 && year % 100 != 0);
}
int main(void) {
printf("请输入年月日时分秒\n");
int year, month, day;
int hour, minute, second;
scanf("%d %d %d", &year, &month, &day);
scanf("%d %d %d", &hour, &minute, &second);
int days_in_month[] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
if (is_leap_year(year)) {
days_in_month[2] = 29;
}
second++;
if (60 == second) {
minute++;
second = 0;
}
if (60 == minute) {
hour++;
minute = 0;
}
if (24 == hour) {
day++;
hour = 0;
}
if (day > days_in_month[month]) {
month++;
day = 1;
}
if (month > 12) {
year++;
month = 1;
}
printf("%d年 %d月 %d日 %d时 %d分 %d秒", year, month, day,hour,minute,second);
}
22
//22. 编写一个程序,交换两个数,不用第三块儿内存。
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int a, b;
scanf("%d%d", &a, &b);
a = a + b;
b = a - b;//此时的b等于原来的a
a = a - b;//此时的a等于原来的b
printf("a=%d,b=%d", a, b);
}
23
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define ARR_SIZE(arr) (sizeof(arr) / sizeof(arr[0]))
/*
有一段文本,统计其中的单词数。例如:
As a technology , "HailStorm" is so new that it is still only known by its code name. 注意:单词间的间隔不一定是一个空格。
*/
int main(void) {
char str[] = "As a technology, \"HailStorm\" is so new that it is still only known by its code name";//用反斜杠(\)转义双引号(\")以包含在字符串中。
char* p = str;
int count = 0;
while (*p) {
if (isalpha(*p)) {
count++;
}
p++;
}
printf("%d", count);
return 0;
}
25
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ARR_SIZE(arr) (sizeof(arr) / sizeof(arr[0]))
/*
25. 有一字符串 a,内容为:My name is Li jilin.,另有字符串 b,内容为:Mr. Zhang Haoling is very happy.
写一函数,将字符串 b 中从第 5 个到第 17个字符复制到字符串 a 中,取代字符串 a 中第 12 个字符以后的字符。输出新的字符串。
*/
int main(void) {
char a[100] = "My name is Li jilin.";//注意定义足够长的字符数组,避免拷贝字符后a数组越界
char b[] = "Mr. Zhang Haoling is very happy.";
char* pa = a;
char* pb = b;
a[12] = '\0';
strncat(a, pb + 4, 13);
/* char *strncat(char *dest, const char *src, size_t n);
找到dest字符串末尾的空字符,然后从该字符开始,将 src 的首个元素复制到 dest末尾,直到:
1.已经复制了 n 个字符。
2.或者复制到达了src字符串的结尾,即遇到了src的空字符串。所以该函数不会把src中的空字符复制到dest中。
最后,strncat函数一定会在dest的末尾添加一个空字符,以确保dest能够表示一个字符串。*/
puts(pa);
return 0;
}
28
//题目:28. 用冒泡法对 10 个整数排序
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
void Bubble_sort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main(void) {
int nums[10] = { 16,1,45,23,99,2,18,67,42,10 };
Bubble_sort(nums, 10);
for (int i = 0; i < 10; i++) {
printf("%3d", nums[i]);
}
}