下载地址:http://zxingnet.codeplex.com/
zxing.net是.net平台下编解条形码和二维码的工具,使用非常方便。
首先下载二进制dll文件,引入工程;
代码:
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- using ZXing.QrCode;
- using ZXing;
- using ZXing.Common;
- using ZXing.Rendering;
- namespace zxingTest
- {
- public partial class Form1 : Form
- {
- EncodingOptions options = null;
- BarcodeWriter writer = null;
- public Form1()
- {
- InitializeComponent();
- options = new QrCodeEncodingOptions
- {
- DisableECI = true,
- CharacterSet = "UTF-8",
- Width = pictureBoxQr.Width,
- Height = pictureBoxQr.Height
- };
- writer = new BarcodeWriter();
- writer.Format = BarcodeFormat.QR_CODE;
- writer.Options = options;
- }
- private void buttonQr_Click(object sender, EventArgs e)
- {
- if (textBoxText.Text == string.Empty)
- {
- MessageBox.Show("输入内容不能为空!");
- return;
- }
- Bitmap bitmap = writer.Write(textBoxText.Text);
- pictureBoxQr.Image = bitmap;
- }
- }
- }
效果:
将字符编码时可以指定字符格式;默认为ISO-8859-1英文字符集,但一般移动设备常用UTF-8字符集编码,
可以通过QrCodeEncodingOptions设置编码方式。
如果要生成其他zxing支持的条形码,只要修改BarcodeWriter.Format就可以了。
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- using ZXing.QrCode;
- using ZXing;
- using ZXing.Common;
- using ZXing.Rendering;
- namespace zxingTest
- {
- public partial class Form1 : Form
- {
- EncodingOptions options = null;
- BarcodeWriter writer = null;
- public Form1()
- {
- InitializeComponent();
- options = new EncodingOptions
- {
- //DisableECI = true,
- //CharacterSet = "UTF-8",
- Width = pictureBoxQr.Width,
- Height = pictureBoxQr.Height
- };
- writer = new BarcodeWriter();
- writer.Format = BarcodeFormat.ITF;
- writer.Options = options;
- }
- private void buttonQr_Click(object sender, EventArgs e)
- {
- if (textBoxText.Text == string.Empty)
- {
- MessageBox.Show("输入内容不能为空!");
- return;
- }
- Bitmap bitmap = writer.Write(textBoxText.Text);
- pictureBoxQr.Image = bitmap;
- }
- }
- }
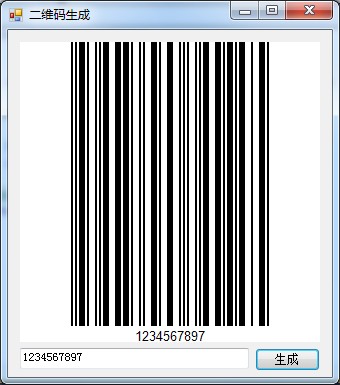
输入字符串需要符合编码的格式,不然会报错。
解码方式:
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- using ZXing.QrCode;
- using ZXing;
- using ZXing.Common;
- using ZXing.Rendering;
- namespace zxingTest
- {
- public partial class Form1 : Form
- {
- BarcodeReader reader = null;
- public Form1()
- {
- InitializeComponent();
- reader = new BarcodeReader();
- }
- private void Form1_DragEnter(object sender, DragEventArgs e)//当拖放进入窗体
- {
- if (e.Data.GetDataPresent(DataFormats.FileDrop))
- e.Effect = DragDropEffects.Copy; //显示拷贝效应
- else
- e.Effect = DragDropEffects.None;
- }
- private void Form1_DragDrop(object sender, DragEventArgs e) //当拖放放在窗体上
- {
- string[] fileNames = (string[])e.Data.GetData(DataFormats.FileDrop, false); //获取文件名
- if (fileNames.Length > 0)
- {
- pictureBoxPic.Load(fileNames[0]); //显示图片
- Result result = reader.Decode((Bitmap)pictureBoxPic.Image); //通过reader解码
- textBoxText.Text = result.Text; //显示解析结果
- }
- }
- }
- }
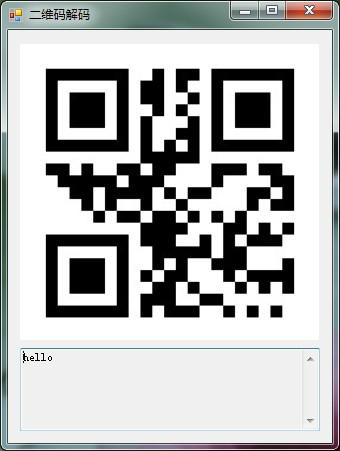