1.1 认识 std.http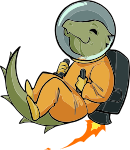
std.http 是 Zig 标准库中用于处理 HTTP 相关操作的类库。以我学习新的编程语言的经历来看,编写web程序是最常见的技术场景,所以熟练掌握 HTTP server/client 服务相关的编程知识是比较重要的。
std.http
主要包含以下API:
Client
: HTTP client implementation.Server
: HTTP server implementation.- protocol:headers parse methods.
Connection
: Connection type (keep_alive
,close
)ContentEncoding
: Content encoding options (compress
,deflate
,gzip
andzstd
)Field
: Common type forname
andvalue
Headers
: HTTP headersMethod
: HTTP methods such asGET
andPOST
Status
: HTTP status codes (not_found = 404
,teapot = 418
, etc.)TransferEncoding
: Form of encoding used to transfer the body (chunked
)Version
: CurrentlyHTTP/1.0
andHTTP/1.1
1.2 编写一个HTTP client程序
先创建一个开工项目:
$ mkdir -p httpz
$ cd httpz
$ zig init
$ ls -ls
total 20
4 -rw-r--r-- 1 xiaods xiaods 3879 Jun 3 11:53 build.zig
4 -rw-r--r-- 1 xiaods xiaods 3080 Jun 3 11:50 build.zig.zon
4 drwxr-xr-x 2 xiaods xiaods 4096 Jun 3 13:33 src
4 drwxr-xr-x 6 xiaods xiaods 4096 Jun 3 11:51 zig-cache
4 drwxr-xr-x 4 xiaods xiaods 4096 Jun 3 11:51 zig-out
编辑 src/main.zig,我们将使用 std.heap.GeneralPurposeAllocator,这是一个安全的分配器,可以防止双重释放(double-free)、使用后释放(use-after-free),并且能够检测内存泄漏。
const std = @import("std");
const print = std.debug.print;
const http = std.http;
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer _ = gpa.deinit();
const allocator = gpa.allocator();
下一步,为了发送一个请求,我们需要几样东西:
- client.open 函数
- 一个从URL解析而来的 std.Uri
下面是我们如何将这些参数组合在一起的方法:
const uri = try std.Uri.parse("http://httpbin.org/headers");
const buf = try allocator.alloc(u8, 1024 * 1024 * 4);
defer allocator.free(buf);
var req = try client.open(.GET, uri, .{
.server_header_buffer = buf,
});
defer req.deinit();
为了真正的发送请求,需要通过send,finish,wait来完成:
try req.send();
try req.finish();
try req.wait();
打印返回的服务器headers 信息:
var iter = req.response.iterateHeaders();
while (iter.next()) |header| {
std.debug.print("Name:{s}, Value:{s}\n", .{ header.name, header.value });
}
try std.testing.expectEqual(req.response.status, .ok);
打印返回的服务端内容:
var rdr = req.reader();
const body = try rdr.readAllAlloc(allocator, 1024 * 1024 * 4);
defer allocator.free(body);
print("Body:\n{s}\n", .{body});
把上面的代码所有内容放在一起,并打印出响应内容:
const std = @import("std");
const print = std.debug.print;
const http = std.http;
pub fn main() !void {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer _ = gpa.deinit();
const allocator = gpa.allocator();
var client = http.Client{ .allocator = allocator };
defer client.deinit();
const uri = try std.Uri.parse("http://httpbin.org/headers");
const buf = try allocator.alloc(u8, 1024 * 1024 * 4);
defer allocator.free(buf);
var req = try client.open(.GET, uri, .{
.server_header_buffer = buf,
});
defer req.deinit();
try req.send();
try req.finish();
try req.wait();
var iter = req.response.iterateHeaders();
while (iter.next()) |header| {
std.debug.print("Name:{s}, Value:{s}\n", .{ header.name, header.value });
}
try std.testing.expectEqual(req.response.status, .ok);
var rdr = req.reader();
const body = try rdr.readAllAlloc(allocator, 1024 * 1024 * 4);
defer allocator.free(body);
print("Body:\n{s}\n", .{body});
}
跑一下:
$ zig build run
Name:Date, Value:Mon, 03 Jun 2024 08:24:19 GMT
Name:Content-Type, Value:application/json
Name:Content-Length, Value:202
Name:Connection, Value:keep-alive
Name:Server, Value:gunicorn/19.9.0
Name:Access-Control-Allow-Origin, Value:*
Name:Access-Control-Allow-Credentials, Value:true
Body:
{
"headers": {
"Accept-Encoding": "gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "zig/0.12.0 (std.http)",
"X-Amzn-Trace-Id": "Root=1-665d7db3-258c846d0fcca0912fadfa8b"
}
}
成功了!我们成功地向服务器发送了一个GET请求并打印出了响应。
GET请求的例子我们看到了,那么如何发起POST请求呢?让我们继续拿例子说话。
准备好发送内容:
const uri = try std.Uri.parse("http://httpbin.org/anything");
const payload =
\\ {
\\ "name": "zig-learning",
\\ "author": "xiaods"
\\ }
;
发送POST 请求:
var buf: [1024]u8 = undefined;
var req = try client.open(.POST, uri, .{ .server_header_buffer = &buf });
defer req.deinit();
req.transfer_encoding = .{ .content_length = payload.len };
try req.send();
var wtr = req.writer();
try wtr.writeAll(payload);
try req.finish();
try req.wait();
try std.testing.expectEqual(req.response.status, .ok);
打印返回内容:
var rdr = req.reader();
const body = try rdr.readAllAlloc(allocator, 1024 * 1024 * 4);
defer allocator.free(body);
print("Body:\n{s}\n", .{body});
完整的Post代码如下:
const std = @import("std");
const print = std.debug.print;
const http = std.http;
pub fn main() !void {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer _ = gpa.deinit();
const allocator = gpa.allocator();
var client = http.Client{ .allocator = allocator };
defer client.deinit();
const uri = try std.Uri.parse("http://httpbin.org/anything");
const payload =
\\ {
\\ "name": "zig-learning",
\\ "author": "xiaods"
\\ }
;
var buf: [1024]u8 = undefined;
var req = try client.open(.POST, uri, .{ .server_header_buffer = &buf });
defer req.deinit();
req.transfer_encoding = .{ .content_length = payload.len };
try req.send();
var wtr = req.writer();
try wtr.writeAll(payload);
try req.finish();
try req.wait();
try std.testing.expectEqual(req.response.status, .ok);
var rdr = req.reader();
const body = try rdr.readAllAlloc(allocator, 1024 * 1024 * 4);
defer allocator.free(body);
print("Body:\n{s}\n", .{body});
}
运行结果:
$ zig run src/http-post.zig
Body:
{
"args": {},
"data": " {\n \"name\": \"zig-learning\",\n \"author\": \"xiaods\"\n }",
"files": {},
"form": {},
"headers": {
"Accept-Encoding": "gzip, deflate",
"Content-Length": "52",
"Host": "httpbin.org",
"User-Agent": "zig/0.12.0 (std.http)",
"X-Amzn-Trace-Id": "Root=1-665d8114-01b0167844d8d101012e6d6a"
},
"json": {
"author": "xiaods",
"name": "zig-learning"
},
"method": "POST",
"origin": "219.133.170.77",
"url": "http://httpbin.org/anything"
}
请消化消化以上代码,别着急,我们后面继续前行,编写web server