点击上方 "程序员小乐"关注公众号, 星标或置顶一起成长
每天凌晨00点00分, 第一时间与你相约
每日英文
When you have something you really love but it causes you pain, God is just testing you to see if you are strong enough to hold it.
当你真正喜欢一样东西,但它又给你带来伤害的时候,其实这是老天在考验你是否足够坚持。
每日掏心话
“你走的路越多,就知道世界有多大,你看的书越多,就知道自己有多浅,一岁又一岁,评判很多事情的标准都与从前不再一样。人啊,这无解的心理和奇怪的人生,就连自己都是捉摸不透的。”
来自:gdpuzxs | 责编:乐乐
链接:cnblogs.com/gdpuzxs/p/7222221.html
程序员小乐(ID:study_tech)第 657 次推文 图片来自网络
正文
1、pom.xml引入jar包
如下:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、修改项目启动类,增加注解@EnableCaching,开启缓存功能
如下:
package springboot;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
@EnableCaching
public class SpringbootApplication{
public static void main(String[] args) {
SpringApplication.run(SpringbootApplication.class, args);
}
}
3、application.properties中配置Redis连接信息
如下:
# Redis数据库索引(默认为0)
spring.redis.database=0
# Redis服务器地址
spring.redis.host=172.31.19.222
# Redis服务器连接端口
spring.redis.port=6379
# Redis服务器连接密码(默认为空)
spring.redis.password=
# 连接池最大连接数(使用负值表示没有限制)
spring.redis.pool.max-active=8
# 连接池最大阻塞等待时间(使用负值表示没有限制)
spring.redis.pool.max-wait=-1
# 连接池中的最大空闲连接
spring.redis.pool.max-idle=8
# 连接池中的最小空闲连接
spring.redis.pool.min-idle=0
# 连接超时时间(毫秒)
spring.redis.timeout=0
4、新建Redis缓存配置类RedisConfig
如下:
package springboot.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.cache.RedisCacheManager;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* Redis缓存配置类
* @author szekinwin
*
*/
@Configuration
@EnableCaching
public class RedisConfig extends CachingConfigurerSupport{
@Value("${spring.redis.host}")
private String host;
@Value("${spring.redis.port}")
private int port;
@Value("${spring.redis.timeout}")
private int timeout;
//自定义缓存key生成策略
// @Bean
// public KeyGenerator keyGenerator() {
// return new KeyGenerator(){
// @Override
// public Object generate(Object target, java.lang.reflect.Method method, Object... params) {
// StringBuffer sb = new StringBuffer();
// sb.append(target.getClass().getName());
// sb.append(method.getName());
// for(Object obj:params){
// sb.append(obj.toString());
// }
// return sb.toString();
// }
// };
// }
//缓存管理器
@Bean
public CacheManager cacheManager(@SuppressWarnings("rawtypes") RedisTemplate redisTemplate) {
RedisCacheManager cacheManager = new RedisCacheManager(redisTemplate);
//设置缓存过期时间
cacheManager.setDefaultExpiration(10000);
return cacheManager;
}
@Bean
public RedisTemplate<String, String> redisTemplate(RedisConnectionFactory factory){
StringRedisTemplate template = new StringRedisTemplate(factory);
setSerializer(template);//设置序列化工具
template.afterPropertiesSet();
return template;
}
private void setSerializer(StringRedisTemplate template){
@SuppressWarnings({ "rawtypes", "unchecked" })
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setValueSerializer(jackson2JsonRedisSerializer);
}
}
5、新建UserMapper
如下:
package springboot.dao;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import org.springframework.cache.annotation.CacheConfig;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import springboot.domain.User;
@Mapper
@CacheConfig(cacheNames = "users")
public interface UserMapper {
@Insert("insert into user(name,age) values(#{name},#{age})")
int addUser(@Param("name")String name,@Param("age")String age);
@Select("select * from user where id =#{id}")
@Cacheable(key ="#p0")
User findById(@Param("id") String id);
@CachePut(key = "#p0")
@Update("update user set name=#{name} where id=#{id}")
void updataById(@Param("id")String id,@Param("name")String name);
//如果指定为 true,则方法调用后将立即清空所有缓存
@CacheEvict(key ="#p0",allEntries=true)
@Delete("delete from user where id=#{id}")
void deleteById(@Param("id")String id);
}
@Cacheable将查询结果缓存到redis中,(key="#p0")指定传入的第一个参数作为redis的key。
@CachePut,指定key,将更新的结果同步到redis中
@CacheEvict,指定key,删除缓存数据,allEntries=true,方法调用后将立即清除缓存
6、service层与controller层
Service层代码如下:
package springboot.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import springboot.dao.UserMapper;
import springboot.domain.User;
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User findById(String id){
return userMapper.findById(id);
}
public int addUser(String name,String age){
return userMapper.addUser(name,age);
}
public void updataById(String id,String name){
userMapper.updataById(id,name);
}
public void deleteById(String id){
userMapper.deleteById(id);
}
}
Controller层,代码如下:
package springboot.web;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import springboot.domain.User;
import springboot.service.UserService;
@RestController
public class HelloController {
@Autowired
private UserService userService;
@RequestMapping("/adduser")
public int addUser(@RequestParam("name")String name,@RequestParam("age")String age){
return userService.addUser(name, age);
}
@RequestMapping("/findUser")
public User findUser(@RequestParam("id") String id){
return userService.findById(id);
}
@RequestMapping("/updataById")
public String updataById(@RequestParam("id") String id,@RequestParam("name") String name){
try {
userService.updataById(id, name);
} catch (Exception e) {
return "error";
}
return "success";
}
@RequestMapping("/deleteById")
public String deleteById(@RequestParam("id") String id){
try {
userService.deleteById(id);
} catch (Exception e) {
return "error";
}
return "success";
}
}
启动redis服务器,redis服务器的安装与启动可以参考博客,地址如下:
http://www.cnblogs.com/gdpuzxs/p/6623171.html
7、配置log4j日志信息
如下:
8、验证redis缓存
首先我们向user表总插入一条数据,数据库显示如下:
现在,我们查询一下user表中id=24的数据,观擦控制台输出的信息,如下:
通过控制台输出信息我们可以知道,这次执行了数据库查询,并开启了Redis缓存查询结果。接下来我们再次查询user表中id=24的数据,观察控制台,如下:
通过控制台输出信息我们可以知道,这次并没有执行数据库查询,而是从Redis缓存中查询,并返回查询结果。我们查看redis中的信息,如下:
方法finduser方法使用了注解@Cacheable(key="#p0"),即将id作为redis中的key值。当我们更新数据的时候,应该使用@CachePut(key="#p0")进行缓存数据的更新,否则将查询到脏数据。
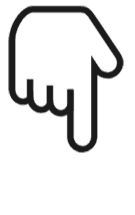