LeetCode Everyday:坚持价值投资,做时间的朋友!!!
题目:
给你一个包含 n 个整数的数组nums
,判断nums
中是否存在三个元素a,b,c
,使得a + b + c = 0
?请你找出所有满足条件且不重复的三元组。
注意:答案中不可以包含重复的三元组。
示例:
- 示例 1:
给定数组 nums = [-1, 0, 1, 2, -1, -4], 满足要求的三元组集合为: [ [-1, 0, 1], [-1, -1, 2] ]
代码
方法一: 集合的思路
执行用时:676 ms, 在所有 Python3 提交中击败了93.05%的用户
内存消耗:17 MB, 在所有 Python3 提交中击败了6.02%的用户
class Solution:
def threeSum(self, nums):
"""
:type nums: List[int]
:rtype: List[List[int]]
"""
res = []
nums.sort()
for i in range(len(nums)):
if nums[i] > 0:
break
if i >= 1 and nums[i] == nums[i-1]:
continue
target = -nums[i]
cache = set()
for j in range(i+1, len(nums)):
if nums[j] in cache:
if len(res) == 0 or res[-1]!=[nums[i], target-nums[j], nums[j]]:
res.append([nums[i], target-nums[j], nums[j]])
cache.add(target-nums[j])
return res
"""
For Example: input: nums = [-1, 0, 1, 2, -1, -4]
output: [
[-1, 0, 1],
[-1, -1, 2]
]
"""
nums = [-1, 0, 1, 2, -1, -4]
solution = Solution()
result = solution.threeSum(nums)
print('输出为:', result)
方法二: 排序+双指针
执行用时 :44 ms, 在所有 Python3 提交中击败了59.87%的用户
内存消耗 :13.7 MB, 在所有 Python3 提交中击败了6.15%的用户
class Solution:
def threeSum(self, nums):
"""
:type nums: List[int]
:rtype: List[List[int]]
"""
n=len(nums)
res=[]
if(not nums or n<3):
return []
nums.sort()
res=[]
for i in range(n):
if(nums[i]>0):
return res
if(i>0 and nums[i]==nums[i-1]):
continue
L=i+1
R=n-1
while(L<R):
if(nums[i]+nums[L]+nums[R]==0):
res.append([nums[i],nums[L],nums[R]])
while(L<R and nums[L]==nums[L+1]):
L=L+1
while(L<R and nums[R]==nums[R-1]):
R=R-1
L=L+1
R=R-1
elif(nums[i]+nums[L]+nums[R]>0):
R=R-1
else:
L=L+1
return res
"""
For Example: input: nums = [-1, 0, 1, 2, -1, -4]
output: [
[-1, 0, 1],
[-1, -1, 2]
]
"""
nums = [-1, 0, 1, 2, -1, -4]
solution = Solution()
result = solution.threeSum(nums)
print('输出为:', result)
方法三: 今天回头刷的时候自己写出了这种版本,需要注意的是set里面必须得是不可哈希的,不能有列表类型,所以我换成了元组
执行用时 :44 ms, 在所有 Python3 提交中击败了59.87%的用户
内存消耗 :13.7 MB, 在所有 Python3 提交中击败了6.15%的用户
class Solution:
def threeSum(self, nums):
"""
:type nums: List[int]
:rtype: List[List[int]]
"""
res = []
nums.sort()
for i in range(len(nums)):
if nums[i] > 0:
break
if i >= 1 and nums[i] == nums[i-1]:
continue
lo, hi, target= i+1, len(nums)-1, -nums[i]
while lo < hi:
if nums[lo] + nums[hi] == target:
res.append((nums[i], nums[lo], nums[hi]))
lo += 1
hi -= 1
elif nums[lo] + nums[hi] > target:
hi -= 1
else:
lo += 1
res = [list(i) for i in set(res)]
return res
"""
For Example: input: nums = [-1, 0, 1, 2, -1, -4]
output: [
[-1, 0, 1],
[-1, -1, 2]
]
"""
nums = [-1, 0, 1, 2, -1, -4]
solution = Solution()
result = solution.threeSum(nums)
print('输出为:', result)
### 参考
1. https://www.bilibili.com/video/BV1ek4y1d7sw
2. https://leetcode-cn.com/problems/3sum/solution/pai-xu-shuang-zhi-zhen-zhu-xing-jie-shi-python3-by/
### 此外
- 原创内容转载请注明出处
- 请到我的[GitHub](https://github.com/xiaoxuebajie/LeetCode)点点 **star**
- 关注我的 [CSDN博客](https://mp.csdn.net/console/article)
- 关注我的[哔哩哔哩](https://space.bilibili.com/424394389/channel/detail?cid=132513)
- 关注公众号:CV伴读社
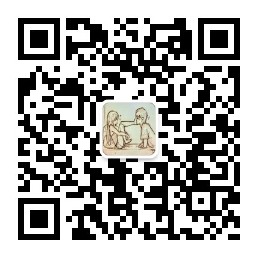