SpringBoot-dubbo-zookeeper整合项目
服务端 demo-server-zoo
项目服务端(provider)架构
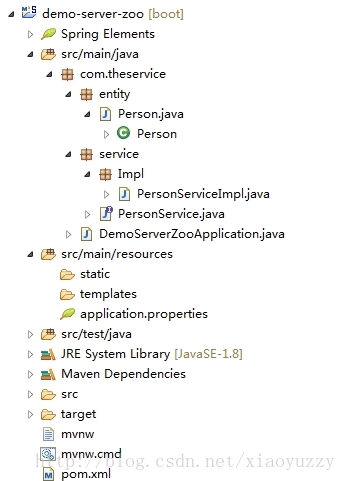
pom.xml配置
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo-server-zoo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>demo-server-zoo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/>
</parent>
<properties>
<dubbo-spring-boot>1.0.0</dubbo-spring-boot>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>io.dubbo.springboot</groupId>
<artifactId>spring-boot-starter-dubbo</artifactId>
<version>${dubbo-spring-boot}</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties配置
##服务提供者名称
spring.dubbo.application.name=provider
##zookeeper注册中心的地址
spring.dubbo.registry.address=zookeeper://127.0.0.1:2181
##
spring.dubbo.protocol.name=dubbo
##端口号
spring.dubbo.protocol.port=20880
##扫描包名
spring.dubbo.scan=com.theservice.service
实体类配置
package com.theservice.entity;
import java.io.Serializable;
/** 实现序列化接口
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午10:58:01
* 类说明
*/
public class Person implements Serializable{
private static final long serialVersionUID = 1L;
private String id;
private String name;
private String tel;
private String addr;
public Person(){
}
public Person(String id, String name, String tel, String addr) {
this.id=id;
this.name=name;
this.tel=tel;
this.addr=addr;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
}
service配置
package com.theservice.service;
import com.theservice.entity.Person;
/**
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午10:42:53
* 类说明
*/
public interface PersonService {
public Person getPerson(String id);
}
serviceImpl实现类配置
package com.theservice.service.Impl;
import com.alibaba.dubbo.config.annotation.Service;
import com.theservice.entity.Person;
import com.theservice.service.PersonService;
/**
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午11:02:15
* 类说明
*/
@Service(version = "1.0.0")
public class PersonServiceImpl implements PersonService{
public Person getPerson(String id) {
return new Person("1","张三","135","北京");
}
}
启动类配置
package com.theservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoServerZooApplication {
public static void main(String[] args) {
SpringApplication.run(DemoServerZooApplication.class, args);
}
}
消费端 demo-client-zoo
项目消费端(consumer)架构
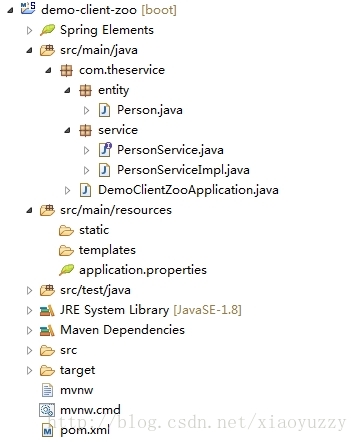
pom.xml文件配置
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo-client-zoo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>demo-client-zoo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/>
</parent>
<properties>
<dubbo-spring-boot>1.0.0</dubbo-spring-boot>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>io.dubbo.springboot</groupId>
<artifactId>spring-boot-starter-dubbo</artifactId>
<version>${dubbo-spring-boot}</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties配置
##端口号
server.port=8081
##消费者名称
spring.dubbo.application.name=consumer
##注册zookeeper的地址
spring.dubbo.registry.address=zookeeper://127.0.0.1:2181
##服务包名
spring.dubbo.scan=com.theservice.service
实体类配置(注:这个实体类名称需要和服务器提供的实体类名称相同,并且属性相同)
package com.theservice.entity;
import java.io.Serializable;
/**
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午10:39:17
* 类说明 :实现序列化接口
*/
public class Person implements Serializable{
private static final long serialVersionUID = 1L;
private String id;
private String name;
private String tel;
private String addr;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
@Override
public String toString() {
return "Person [id=" + id + ", name=" + name + ", tel=" + tel + ", addr=" + addr + "]";
}
}
PersonService配置
package com.theservice.service;
import com.theservice.entity.Person;
/**
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午10:42:53
* 类说明
*/
public interface PersonService {
/**
* 根据id,查询这个人的信息
* @param id
*/
public Person getPerson(String id);
}
PersonServiceImpl
package com.theservice.service;
import org.springframework.stereotype.Component;
import com.alibaba.dubbo.config.annotation.Reference;
import com.theservice.entity.Person;
/**
* @author 作者 :MR zhou
* @version 创建时间:2018年1月25日 上午10:43:50
* 类说明
*/
@Component
public class PersonServiceImpl{
/**
*dubbo服务注册到zookeeper的版本号
*/
@Reference(version = "1.0.0")
PersonService service;
public void getPerson(){
String id="1";
Person p = service.getPerson(id);
System.out.println(p.toString());
}
}
client启动类
package com.theservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
import com.theservice.service.PersonServiceImpl;
@SpringBootApplication
public class DemoClientZooApplication {
public static void main(String[] args) {
ConfigurableApplicationContext run = SpringApplication.run(DemoClientZooApplication.class, args);
PersonServiceImpl serviceImpl = run.getBean(PersonServiceImpl.class);
serviceImpl.getPerson();
}
}
注意事项:
0.服务端与消费端的包名需要一致(com.theservice.service/com.theservice.entity)
1.需要先启动zookeeper注册中心服务
2.然后启动服务端(server)
3.启动消费端(client)
4.成功后控制台会打印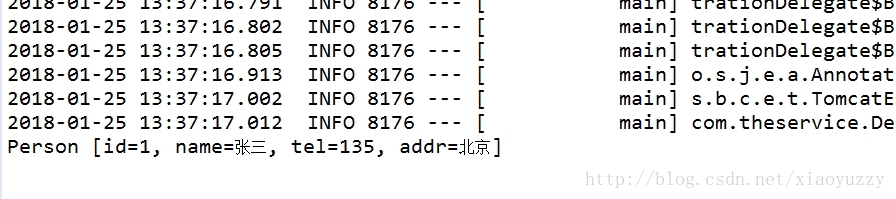