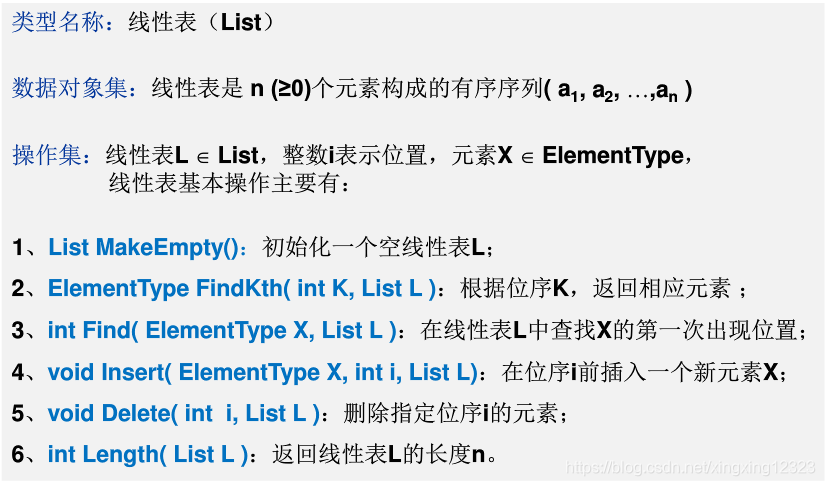
数组实现线性表
typedef int Position;
typedef struct LNode *List;
struct LNode{
ElementType Data[MAXSIZE];
Position Last;
};
typedef int bool;
#define TRUE 1
#define FALSE 0
#define ERROR -1
#define MAXSIZE 1000
List makeEmpty(){
List L;
L = (List)malloc(sizeof(struct LNode));
L->Last = -1;
return L;
}
ElementType FindKth(int k,List L){
if((k+1) > L->Last || k<1){
return NULL;
}
return Data[k-1];
}
int Find(ELementType x,List L){
for(int i=0;i<=L->Last;i++){
if(L->Data[i]==x){
return i;
}
}
return ERROR;
}
bool Insert(ElementType X,int i,List L){
if(L->Last==MAXSIZE-1){
return FALSE;
}
if(i < 0 || i > L->Last+1 ){
return FALSE;
}
for(int j=L->Last;j>=i-1;j--){
L->Data[j+1] =L->Data[j];
}
L->Data[i-1] = x;
L->Last++;
return TRUE;
}
bool Delete(int i,List L){
if(i<0 || i>L->Last || L->Last < 0){
return FALSE;
}
for(int j=i-1;j<L->Last;j++){
L->Data[j] = L->Data[j+1];
}
L->Last--;
return TRUE;
}
int Length(List L){
return L->Last+1;
}
链表实现线性表
typedef struct LNode *PtrToLNode;
struct LNode {
ElementType Data;
PtrToLNode Next;
};
typedef PtrToLNode Position;
typedef PtrToLNode List;
typedef int bool;
#define TRUE 1
#define FALSE 0
#define ERROR -1
#define MAXSIZE 1000
List makeEmpty(){
List L;
L = (List)malloc(sizeof(struct LNode));
L->Next = NULL;
return L;
}
ElementType FindKth(int k,List L){
Position p=L;
int i=0;
while(p && i <= k-1 ){
p=p->Next;
i++;
}
return p->Data;
}
Position Find(ELementType x,List L){
Position p=L;
while(p && p -> Data != x)
p = p -> Next;
return p;
}
bool Insert(ElementType X,Position p,List L){
Position pre,temp;
for(pre=L;pre&&pre->Data !=p;pre=pre->Next);
if(pre==NULL){
return FALSE;
}else{
temp = (Position)malloc(sizeof(struct LNode));
temp->Next = pre->Next;
temp ->Data = X;
pre->Next = temp;
return TRUE;
}
}
bool Delete(Position p,List L){
Position pre,temp;
for(pre=L;pre&&pre->Data !=p;pre=pre->Next);
if(pre == NULL || p==NULL){
return FALSE;
}else{
pre->Next = pre->Next->Next;
free(p);
return TRUE;
}
}
int Length(List L){
int i=0;
for(L){
i++;
L=L->Next;
}
return i;
}