import React, { Component } from 'react';
class Debounce extends Component{
constructor(props) {
super(props);
}
inputValue = (content) => {
console.log(content);
}
debounce = (fun, wait) => {
return (...rest) => {
let args = rest;
console.log(this.timerId);
if(this.timerId) clearTimeout(this.timerId);
this.timerId = setTimeout(() => {
fun(args);
}, wait);
}
}
throttle = (fun, wait=3000) => {
return (...rest) => {
let args = rest;
if(!this.canRun){
this.canRun = setTimeout(() => {
fun(args);
this.canRun = null;
}, wait);
}
}
}
onUndebounceClick = (e) => {
console.log(e.target.value);
}
onDebounceClick = (e) => {
let debounceKeyup = this.debounce(this.inputValue, 3000);
debounceKeyup(e.target.value);
}
onThrottleClick = () => {
let throttleKeyup = this.throttle(this.inputValue, 3000);
throttleKeyup('a');
}
render() {
return(
<div>
正常的input: <input onKeyUp={this.onUndebounceClick}/><br/>
防抖的input: <input onKeyUp={this.onDebounceClick}/><br/>
节流的button: <button onClick={this.onThrottleClick}>click</button>
</div>
)
}
}
export default Debounce;
在React中实现防抖节流
最新推荐文章于 2024-07-25 17:14:51 发布
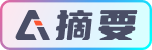