Spring注解驱动开发学习总结11:AOP功能使用
1、AOP功能使用
AOP:是指在程序运行期间动态地将某段代码切入到指定方法指定位置进行运行的编程方式。
以下建立一个日志的AOP功能进行举例:
1、导入AOP依赖:spring-aspects,4.3.12.RELEASE
2、构建业务类:比如构建一个数学计算器的业务类(MathCalculator),该业务类添加一个除法方法;
3、构建日志切面类:LogAspects。
3.1 给切面类(LogAspects)添加@Aspect注解,即告诉ioc容器这是一个切面类
3.2 添加通知方法:
3.2.1 前置通知(@Before):在目标方法运行之前运行
3.2.2 后置通知(@After):在目标方法运行结束之后运行
3.2.3 返回通知(@AfterReturning):在目标方法正常返回之后运行
3.2.4 异常通知(@AfterThrowing):在目标方法出现异常以后运行
3.2.5 环绕通知(@Around):基于动态代理,可以手动执行目标方法(joinPoint.proceed()),并且
可以在执行目标方法的前后执行对应的通知功能(前置、后置、返回、异常)。
3.3 给切面类(LogAspects)的目标方法标注在哪些方法上运行,即添加切入点pointCut。
3.4 通知方法中,可以使用JoinPoint类的参数获取对应切入点方法的信息
4、将切面类和业务逻辑类都加入到容器中。
5、给配置类上添加@EnableAspectJAutoProxy注解,即告诉ioc容器开启基于注解的aop模式。
1.1 导入AOP依赖
pom文件新增AOP依赖:spring-aspects,4.3.12.RELEASE
1.2 构建业务类
构建一个数学计算器的业务类(MathCalculator),该业务类添加一个除法方法。
package com.example.bean;
public class MathCalculator {
public int div(int i, int j) {
System.out.println("MathCalculator_div方法开始执行");
return i/j;
}
}
1.3 构建切面类
构建切面类:com/example/aop/LogAspects.java
1、添加通知方法
1)添加前置通知@Before方法:logStart,打印目标方法的名称和参数列表;
2)添加后置通知@After方法:logEnd,打印目标方法结束
3)添加返回通知@AfterReturning方法:logReturn,打印目标方法的返回值
4)添加异常通知@AfterThrowing方法:logException,打印目标方法的异常信息
2、添加切入点表达式:@Pointcut(“execution(public int com.example.bean.MathCalculator.*(…))”),表示bean.MathCalculator类下的所有返回值为int的方法,都会调用切面中的通知方法。
3、添加获取目标方法的参数类JoinPoint,同时也可以获取到返回值和异常信息的参数值。
package com.example.aop;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.*;
import java.util.Arrays;
@Aspect
public class LogAspects {
// 抽取公共的切入点表达式
// 1、本类引用
// 2、其他的切面引用
@Pointcut("execution(public int com.example.bean.MathCalculator.*(..))")
public void pointCut() {
}
@Before("pointCut()")
public void logStart(JoinPoint joinPoint) {
Object[] args = joinPoint.getArgs();
System.out.println("日志切面工具,前置通知方法@Before运行: " + joinPoint.getSignature().getName() + "方法运行前,参数列表是:" + Arrays.asList(args));
}
@After("pointCut()")
public void logEnd(JoinPoint joinPoint) {
System.out.println("日志切面工具,后置通知方法@After运行: " + joinPoint.getSignature().getName() + "方法运行结束");
}
@AfterReturning(value = "pointCut()", returning = "result")
public void logReturn(JoinPoint joinPoint, Object result) {
System.out.println("日志切面工具,返回通知方法@AfterReturning运行: " + joinPoint.getSignature().getName() + "方法运行成功,返回结果是: " + result);
}
@AfterThrowing(value = "pointCut()", throwing = "exception")
public void logException(JoinPoint joinPoint, Exception exception) {
System.out.println("日志切面工具,异常通知方法@AfterThrowing运行: " + joinPoint.getSignature().getName() + "方法运行异常,异常消息是: " + exception);
}
}
1.4 构建配置类
构建配置类:com/example/config/MainConfigOfAOP.java
需要在配置类上开启基于注解的aop模式:@EnableAspectJAutoProxy
package com.example.config;
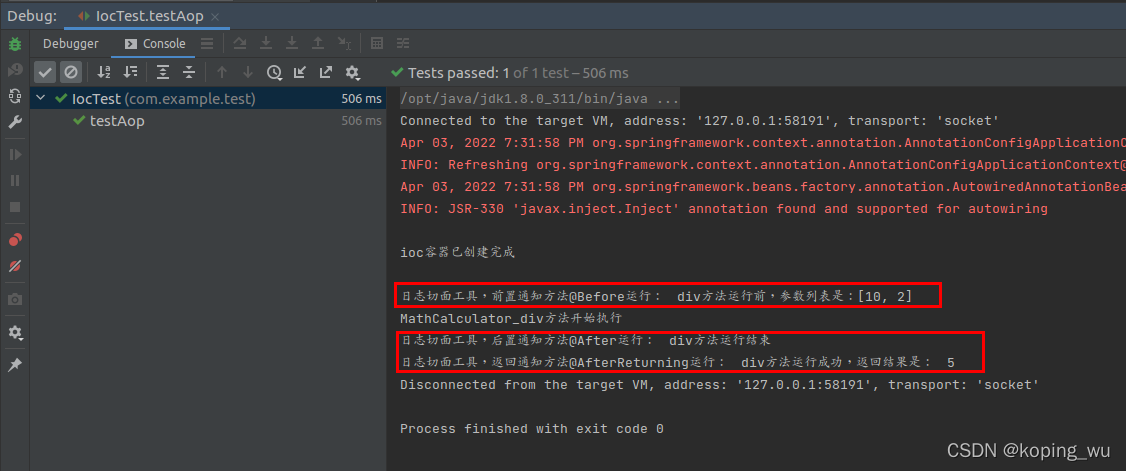
import com.example.aop.LogAspects;
import com.example.bean.MathCalculator;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@EnableAspectJAutoProxy
@Configuration
public class MainConfigOfAOP {
@Bean
public MathCalculator mathCalculator() {
return new MathCalculator();
}
@Bean
public LogAspects logAspects() {
return new LogAspects();
}
}
1.5 测试运行方法成功
添加运行成功的测试方法:testAop
@Test
public void testAop() {
AnnotationConfigApplicationContext applicationContext = new AnnotationConfigApplicationContext(MainConfigOfAOP.class);
System.out.println("\nioc容器已创建完成\n");
MathCalculator bean = applicationContext.getBean(MathCalculator.class);
bean.div(10, 2);
}
运行结果如下图。
1)在除法运行之前会执行前置通知方法@Before,并且打印方法名和参数列表
2)在除法运行之后会执行后置通知方法@After
3)在除法返回结果之后会执行返回方法@AfterReturning,并成功打印返回值
1.5 测试运行方法异常
添加运行成功的测试方法:testAop
@Test
public void testAop() {
AnnotationConfigApplicationContext applicationContext = new AnnotationConfigApplicationContext(MainConfigOfAOP.class);
System.out.println("\nioc容器已创建完成\n");
MathCalculator bean = applicationContext.getBean(MathCalculator.class);
bean.div(10, 0);
}
运行结果如下图。
1)由于将除数改为了0,运行时发生了异常。因此在除法运行异常之后会执行异常方法@AfterThrowing,并打印异常信息