<?php
namespace Personnelsystem\Controller;
header("content-type:text/html;charset=utf-8");
class UploadFileController extends LoginTrueController {
public function __construct()
{
parent::__construct();
$this->LoginTrue();
}
/**
* 批量上传文件
*/
public function index(){
$this->display();
}
/**
* 开始下载
*/
public function uploadAction(){
$type = I('upload_type');
if(!$type){
$this->error('请选择上传类型');
}
$zip_file = !empty($_FILES['zip_file']) ? $_FILES['zip_file'] : '';
if(!$zip_file){
$this->error('请选择上传的文件');
}
$arr = explode('.',$zip_file['name']);
if($arr[count($arr)-1] != 'zip'){
$this->error('文件类型错误,请上传zip文件');
}
$file_path = '';
$upload = new \Think\Upload();// 实例化上传类
$upload->maxSize = 3145728000 ;// 设置附件上传大小
$upload->exts = array('zip');// 设置附件上传类型
$upload->rootPath = $_SERVER['DOCUMENT_ROOT'] . '/'; // 设置附件上传根目录
$upload->savePath = '/Uploads/temp/'; // 设置附件上传(子)目录
$upload->subName = array('date','Ymd');
// 上传文件
$info = $upload->upload();
if(!$info) {// 上传错误提示错误信息
$this->error($upload->getError());
}
foreach($info as $file){
$file_path = $_SERVER['DOCUMENT_ROOT'] . $file['savepath'].$file['savename'];
$path = $_SERVER['DOCUMENT_ROOT'] . $file['savepath'];
}
if(!$file_path){
$this->error('上传文件失败');
}
if($type == 1){ // 毕业证书
$this->uploadBiye($file_path, $path);
}elseif ($type == 2){// 保密协议
$this->uploadBiye($file_path,$path);
}elseif($type == 3){// 劳动合同
$this->uploadBiye($file_path,$path);
}elseif($type == 4){//个人
$this->uploadBiye($file_path,$path);
}elseif($type == 5) {//公司
$this->uploadCompany($file_path, $path);
}else{
$this->error('下载类型错误');
}
}
/**
* 批量上传
* @param $file_path
*/
private function uploadBiye($zipfile, $filepath) {
// dump($filepath);die();
$zip = new \ZipArchive();
if ($zip->open($zipfile) === TRUE) {//中文文件名要使用ANSI编码的文件格式
// 加入此段↓
$fileNum = $zip->numFiles;
for ($i = 0; $i < $fileNum; $i++) {
$statInfo = $zip->statIndex($i, \ZipArchive::FL_ENC_RAW);
$zip->renameIndex($i, iconv('GBK', 'utf-8//IGNORE', $statInfo['name']));
}
$zip->open($zipfile);
$zip->extractTo($filepath);//提取全部文件
//$zip->extractTo('/my/destination/dir/', array('pear_item.gif', 'testfromfile.php'));//提取部分文件
$zip->close();
$output = 1;
} else {
$output = 0;
}
if($output != '1'){
$this->error('解压文件出错');
}
$arr = scandir($filepath);
$end = end($arr);
if (is_dir($filepath.$end)){
$temp = scandir($filepath.$end);
}else{
$temp = scandir($filepath);
}
//dump($arr);die();
foreach ($temp as $k => $value) {
if($value == '.' or $value == '..'){
continue;
}
$temp_arr = explode('.', $value);
if($temp_arr[1] == 'zip'){
continue;
}
$name = time() . rand_str(6, 0, '123456789') . '.' . $temp_arr[1];
$staff_name = substr($temp_arr[0],0,strrpos($temp_arr[0],"-"));//员工名
$type = substr($temp_arr[0],strripos($temp_arr[0],"-")+1);
//获取公司名字
$staff_company_id = M('staff')->where("stName = '$staff_name'")->getField('stCompanyId');
$staff_id = M('staff')->where("stName = '$staff_name'")->getField('stId');
$staff_company = M('company')->where("id='$staff_company_id'")->getField('name');
$root = $_SERVER['DOCUMENT_ROOT'];
$path = "/Uploads/Staff/$staff_company/$staff_name" . '/';
if(!is_dir($root . $path)){
mkdir($root . $path, 0777,true);
}
// 判断类型单文件还是多文件
//dump($filepath.$end.'/'.$value);dump($root.$path.$name);dump($type);die('321');
if (is_dir($filepath.$end)){
$copy = $filepath.$end.'/'.$value;
}else{
$copy = $filepath.$value;
}
if(copy($copy, $root . $path . $name)){
if ($type == '毕业证'){
$res = M('staff')->where("stId = '$staff_id'")->save(['biye_img' => $path . $name]);
}elseif ($type == '学位证'){
$res = M('staff')->where("stId = '$staff_id'")->save(['degree_img' => $path . $name]);
}elseif (strstr($type,'员工简历') !== false ){
$jianli = M('staff')->where("stId = '$staff_id'")->getField('jianli_img');
$res = M('staff')->where("stId = '$staff_id'")->save(['jianli_img' => $path . $name.','.$jianli]);
}elseif (strstr($type,'劳动合同') !== false){
$laodong = M('contract')->where("user_id = '$staff_id'")->getField('picture_hetong');
$res = M('contract')->where("user_id = '$staff_id'")->save(['picture_hetong' => $path . $name.','.$laodong]);
}elseif (strstr($type,'保密协议') !== false){
$baomi = M('contract')->where("user_id = '$staff_id'")->getField('baomi_img');
$res = M('contract')->where("user_id = '$staff_id'")->save(['baomi_img' => $path . $name.','.$baomi]);
}elseif ($type == '离职证明'){
$res = M('staff')->where("stId = '$staff_id'")->save(['lizhi_img' => $path . $name]);
}else{
$this->error('文件类型有误');
}
}else{
continue;
}
}
// 删掉临时文件
$this->deldir($filepath);
$this->success("上传成功");
}
//删除指定文件夹以及文件夹下的所有文件
private function deldir($dir) {
//先删除目录下的文件:
$dh = opendir($dir);
while ($file=readdir($dh)) {
if($file !="." && $file!="..") {
$fullpath=$dir."/".$file;
if(!is_dir($fullpath)) {
unlink($fullpath);
} else {
$this->deldir($fullpath);
}
}
}
closedir($dh);
//删除当前文件夹:
if(rmdir($dir)) {
return true;
} else {
return false;
}
}
}
//多文件夹上传
private function newUpload($zipfile, $filepath) {
$zip = new \ZipArchive();
if ($zip->open($zipfile) === TRUE) {
if(!is_dir($filepath)) mkdir($filepath,0775,true);
$docnum = $zip->numFiles;
for($i = 0; $i < $docnum; $i++) {
$statInfo = $zip->statIndex($i,\ZipArchive::FL_ENC_RAW);
$filename = $this->transcoding($statInfo['name']);
if($statInfo['crc'] == 0) {
//新建目录
if(!is_dir($filepath.'/'.substr($filename, 0,-1)))
mkdir($filepath.'/'.substr($filename, 0,-1),0775,true);
// copy('zip://'.$zipfile.'#'.$zip->getNameIndex($i), $filepath.'/'.$filename);
} else {
//拷贝文件
copy('zip://'.$zipfile.'#'.$zip->getNameIndex($i), $filepath.'/'.$filename);
}
}
$zip->close();
$output = 1;
}else{
$output = 0;
}
if($output != '1'){
$this->error('解压文件出错');
}
$path = scandir($filepath);
}
function transcoding($fileName){
$encoding = mb_detect_encoding($fileName,['UTF-8','GBK','BIG5','CP936']);
if (DIRECTORY_SEPARATOR == '/'){ //linux
$filename = iconv($encoding,'UTF-8',$fileName);
}else{ //win
$filename = iconv($encoding,'GBK',$fileName);
}
return $filename;
}
PHP 上传压缩文件
最新推荐文章于 2024-04-10 17:52:50 发布
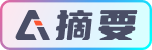