在linux系统里面,我们有时候需要获取当前时间,用它来统计程序运行的时间,或者显示标准格式的日期和时间等等。那么怎么获取时间呢?下面就来了解一下。
本文讲解linux中时间相关的函数和时间转换相关函数:
获取标准时间
#include <time.h>
time_t time(time_t *t);
time_t 是什么类型? 秒数,为long正整型;
获取函数内部的值有几种方式?
1、返回值
2、把地址传进去
将标准时间转换成字符串
char *ctime(const time_t *timep);
用到了 time_t 类型 返回类型 char * 可当成字符串 %s
asctime函数
原型如下:
char *asctime(const struct tm *tm);
这个函数可以把任意一个tm结构体转换为标准格式的日期和时间。
将标准时间转换成本地时间
struct tm *localtime(const time_t *timep);
返回的结构体
使用时间函数的示例:
/***************************
* 每隔 1 秒钟向文件写入一条时间信息 sleep(1)
* Tue Jan 5 16:24:06 2021
* Tue Jan 5 16:24:06 2021
* Tue Jan 5 16:24:06 2021
*
****************************/
#include <time.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#define FONT_SIZE 128
int main(int argc,char ** argv){
char result[FONT_SIZE] = {0};
char * weeks[] = {"星期一","星期二","星期三","星期四","星期五","星期六","星期日",};
struct tm * t;
time_t a = 0;
time_t now = time(&a);
int fdno = open("2.txt",O_WRONLY|O_CREAT|O_TRUNC,0664);
while(1){
t = localtime(&now);
//printf("asc=%s",asctime(t));
snprintf(result, FONT_SIZE, "%d-%d-%d %d:%d %s\n",\
t->tm_year+1900, t->tm_mon+1, \
t->tm_mday, t->tm_hour, t->tm_min, weeks[t->tm_wday-1]);
write(fdno, result, strlen(result));
memset(result,0,FONT_SIZE);
sleep(3);
}
close(fdno);
return 0;
}
目录操作函数
1、打开目录
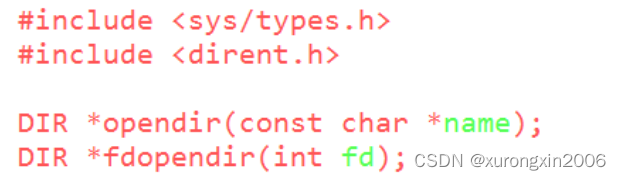
打开一个目录流
,
用
name
目录名打开;
返回
成功
:
返回一个目录流指针
,
指向目录入口
失败
: NULL
设置
errno
2、读目录

将打开的目录
DIR
流传入该函数
,
读目录会返回
struct dirent;
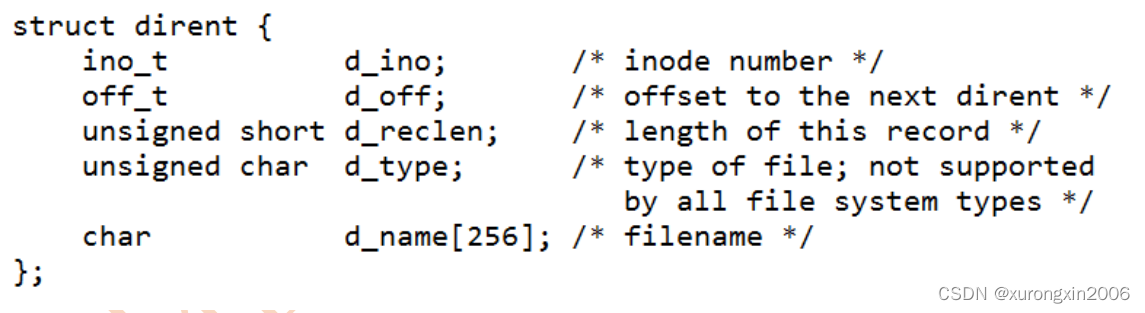
多次调用会依次返回目录下面的文件或子目录;
所有的目录下的文件或子目录列举完毕
,
返回
NULL;
返回:
成功
:
得到一个
dirrent
对象
失败
: NULL
设置
errno
3、关闭目录

实现一个
ls –l
的功能
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <sys/types.h>
#include <pwd.h>
#include <grp.h>
#include <time.h>
#include <dirent.h>
char dirnames[1024][128];
void get_file_meta(const char * name, const char * path);
void get_dirname(const char * path,char (*ret)[1024][128]);
/*****************************
* 名称:自定义ls函数
* 参数:例如 mls [-l] [path]
******************************/
int main(int argc,char ** argv){
//不带参数时,默认当前目录
if(argv[1] == NULL){
argv[1] = "./";
}
int flag = 0;
char * path = argv[1];
//判断有没有选项
if(argv[1][0] == '-'){
if( strstr(argv[1],"l") != NULL){
flag = 1;
path = "./";
}
}
//在flag=1也就是说有选项的情况下,判断第二个参数
if(flag == 1 && argv[2] != NULL){
path = argv[2];
}
//获取目录下的文件集合
get_dirname(path,&dirnames);
//遍历出每个文件的属性
for(int j=0;j<1024;j++)
{
if(strlen(dirnames[j])>0){
if(flag == 0){
printf("%s\n",dirnames[j]);
}else{
get_file_meta(dirnames[j],path);
}
}
}
return 0;
}
//获取指定目录下的文件列表
void get_dirname(const char * path,char (*ret)[1024][128]){
DIR *dir;
struct dirent *dp;
//打开目录流
if ((dir = opendir(path)) == NULL) {
perror("opendir");
exit(1);
}
//读取目录流中dp对象
int i=0;
while ((dp = readdir(dir)) != NULL) {
if( strcmp(dp->d_name,".") == 0 || strcmp(dp->d_name,"..") == 0){
continue;
}
strcpy((char *)(*ret)[i], dp->d_name);
//printf("%s\n",dp->d_name);
i++;
}
//关闭目录流
closedir(dir);
}
//获取文件属性
void get_file_meta(const char * name , const char * path){
char cmd[128] = {'\0'};
struct stat buf;
strcat(cmd,path);
strcat(cmd,name);
//获取文件信息
lstat(cmd, &buf);
//打印文件类型
if (S_ISREG(buf.st_mode))
printf("-");
else if (S_ISDIR(buf.st_mode))
printf("d");
else if (S_ISCHR(buf.st_mode))
printf("c");
else if (S_ISBLK(buf.st_mode))
printf("b");
else if (S_ISFIFO(buf.st_mode))
printf("p");
else if (S_ISLNK(buf.st_mode))
printf("l");
else if (S_ISSOCK(buf.st_mode))
printf("s");
//打印权限
char str[]="xwr-";
for (int i=8;i>=0;i--) {
if(buf.st_mode & 1<<i)
printf("%c", str[i%3]);
else
printf("%c", str[3]);
}
//打印链接数
printf(" %lu ", buf.st_nlink);
//打印用户名和组名
printf("%s ",getpwuid(buf.st_uid)->pw_name);
printf("%s ",getgrgid(buf.st_gid)->gr_name);
//打印文件大小
printf("%ld ", buf.st_size);
//打印日期时间
struct tm *pt;
pt = localtime(&buf.st_ctime);
printf(" %d月 %d %d:%d ", pt->tm_mon+1, pt->tm_mday, pt->tm_hour, pt->tm_min);
printf("%s", name);
printf("\n");
memset(cmd,'\0',128);
}