计算长方体、四棱锥的表面积和体积
Time Limit: 1000MS
Memory Limit: 65536KB
Problem Description
计算如下立体图形的表面积和体积。
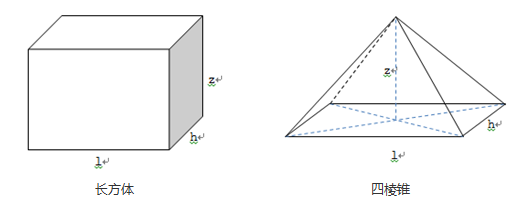
从图中观察,可抽取其共同属性到父类Rect中:长度:l 宽度:h 高度:z
在父类Rect中,定义求底面周长的方法length( )和底面积的方法area( )。
定义父类Rect的子类立方体类Cubic,计算立方体的表面积和体积。其中表面积area( )重写父类的方法。
定义父类Rect的子类四棱锥类Pyramid,计算四棱锥的表面积和体积。其中表面积area( )重写父类的方法。
输入立体图形的长(l)、宽(h)、高(z)数据,分别输出长方体的表面积、体积、四棱锥的表面积和体积。
Input
输入多行数值型数据(double);
每行三个数值,分别表示l h z
若输入数据中有负数,则不表示任何图形,表面积和体积均为0。
Output
行数与输入相对应,数值为长方体表面积 长方体体积 四棱锥表面积 四棱锥体积(中间有一个空格作为间隔,数值保留两位小数)
Example Input
1 2 3 0 2 3 -1 2 3 3 4 5
Example Output
22.00 6.00 11.25 2.00 0.00 0.00 0.00 0.00 0.00 0.00 0.00 0.00 94.00 60.00 49.04 20.00
Hint
四棱锥体公式:V=1/3Sh,S——底面积 h——高
Author
zhouxq
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
double l = sc.nextDouble();
double h = sc.nextDouble();
double z = sc.nextDouble();
// 构造长方体对象;
Cubic cubic = new Cubic(l, h, z);
// 构造四棱锥对象
Pyramid pyramid = new Pyramid(l, h, z);
// 格式化数据
String area1 = String.format("%.2f", cubic.area());
String volumn1 = String.format("%.2f", cubic.volumn());
String area2 = String.format("%.2f", pyramid.area());
String volumn2 = String.format("%.2f", pyramid.volumn());
// 输出数据
System.out.println(area1 + " " + volumn1 + " " + area2 + " "
+ volumn2);
}
sc.close();
}
}
/**
* 父类
*
* @author student
*
*/
class Rect {
double l;
double h;
double z;
public Rect(double l, double h, double z) {
if (l > 0 && h > 0 && z > 0) {
this.l = l;
this.h = h;
this.z = z;
}
}
public Rect(int l) {
this(l, l, l);
}
public Rect() {
}
/**
* 计算长方体底面周长
*/
public double length() {
return 2 * (l + h);
}
/**
* 长方体底面面积
*/
public double area() {
return l * h;
}
}
/**
* 长方体类
*/
class Cubic extends Rect {
public Cubic(double l, double h, double z) {
super(l, h, z);
}
/**
* 长方体表面积,重写父类方法
*/
public double area() {
return 2 * super.area() + length() * z;
}
/**
* 长方体体积,子类新增方法
*/
public double volumn() {
return super.area() * z;
}
}
/**
* 四椎体类
*
* @author student
*
*/
class Pyramid extends Rect {
public Pyramid(double l, double h, double z) {
super(l, h, z);
}
/**
* 四椎体表面积,重写父类方法;
*/
public double area() {
return super.area() + l * Math.sqrt(z * z + h * h / 4) + h
* Math.sqrt(z * z + l * l / 4);
}
/**
* 四棱锥体积,子类新增方法
*/
public double volumn() {
return super.area() * z / 3;
}
}