The Bean Validation API (JSR-303) defines a meta-data model and API for bean validation based on annotations. The functionality is achieved through the resteasy-hibernatevalidator-provider component. In order to integrate, we need to add resteasy-hibernatevalidator-provider.jar and hibernate-validator.jar files to the classpath.
If you are using maven the, you need to add only below dependency. Change the version number of RESTEasy with yours.
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-hibernatevalidator-provider</artifactId>
<version>2.3.1.GA</version>
</dependency>
Step 1) Add the validation specific annotations in API parameters
You can use various annotations for validating the request parameters and form input. For example, I have used@NotNull and @Size annotations. Read about all available annotations in link. No not forget to add@ValidateRequest annotation on method API. @ValidateRequest enables the validation on method where it is applied.
package com.howtodoinjava.rest;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import javax.ws.rs.FormParam;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
import org.jboss.resteasy.spi.validation.ValidateRequest;
@Path("/rest")
public class UserService
{
@Path("/users")
@POST
@ValidateRequest
public Response addUser
(
@NotNull
@Size(min=1,max=50)
@FormParam("firstName") String firstName ,
@Size(max=50)
@FormParam("lastName") String lastName
)
{
return Response.ok().entity("User "" + firstName + " " + lastName + "" added through JAX-RS JavaScript API").build();
}
}
Step 2) Add validation exception handler
Well this is important step because hibernate validator plugin does not have any facility for exception handling, so you need to embed yous. Exception handlers in RESTEasy are used using the ExceptionMapperclass.
package com.howtodoinjava.exception;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
import org.hibernate.validator.method.MethodConstraintViolationException;
@Provider
public class ValidationExceptionHandler implements ExceptionMapper<MethodConstraintViolationException>
{
@Override
public Response toResponse(MethodConstraintViolationException exception)
{
return Response.status(Status.BAD_REQUEST).entity("Fill all fields").build();
}
}
Step 3) Build the client code and test application
I have written a normal HTML form which will send two parameters for firstName and lastName parameters using form submission. Parameter validation is performed automatically and if validation failed, exception handler is invoked to return correct status code.
<html>
<body>
<h1>RESTEasy Hibernate Validator Plugin</h1>
<div id="error"></div>
<form method="post" action="./rest/users">
<p>First Name : <input type="text" name="firstName" id="firstName"/></p>
<p>LastName : <input type="text" name="lastName" id="lastName"/></p>
<input type="submit" value="Add User" />
</form>
Demo by : <b>http://www.howtodoinjava.com</b>
</body>
</html>
You can test the above application either in browser window or you can use some REST testing tools like RESTClient firefox plugin.
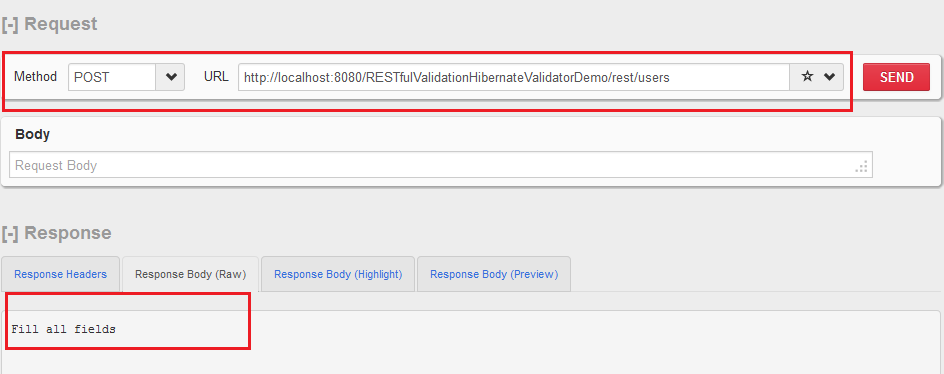
RESTEasy bean validation using hibernate validator
To Download the sourcecode of this demo, follow below link.
Sourcecode Download
Happy Learning !!