//线性表链式储存结构
#include<stdlib.h>
#include<stdio.h>
#define DATATYPE char
typedef struct node
{
DATATYPE data;
struct node *next;
} LINKLIST;
//头插法创立单链表
LINKLIST *hcreate()
{
LINKLIST *head,*p;
char ch;
head=( LINKLIST *)malloc( sizeof( LINKLIST));
head->next=NULL;
while(ch=getchar()!='\n')
{
p=(LINKLIST *)malloc(sizeof(LINKLIST));
p->data=ch;
p->next=head->next;
head->next=p;
}
return(head);
}
// 单链表的输出
void print(LINKLIST *head)
{
LINKLIST *p;
p=head->next;
while(p!=NULL)
{
printf("%c",p->data);
p=p->next;
}
}
int main()
{
LINKLIST *head,*p;
head=hcreate();
print(head);
}
#include<stdlib.h>
#include<stdio.h>
#define DATATYPE char
typedef struct node
{
DATATYPE data;
struct node *next;
} LINKLIST;
//头插法创立单链表
LINKLIST *hcreate()
{
LINKLIST *head,*p;
char ch;
head=( LINKLIST *)malloc( sizeof( LINKLIST));
head->next=NULL;
while(ch=getchar()!='\n')
{
p=(LINKLIST *)malloc(sizeof(LINKLIST));
p->data=ch;
p->next=head->next;
head->next=p;
}
return(head);
}
// 单链表的输出
void print(LINKLIST *head)
{
LINKLIST *p;
p=head->next;
while(p!=NULL)
{
printf("%c",p->data);
p=p->next;
}
}
int main()
{
LINKLIST *head,*p;
head=hcreate();
print(head);
}
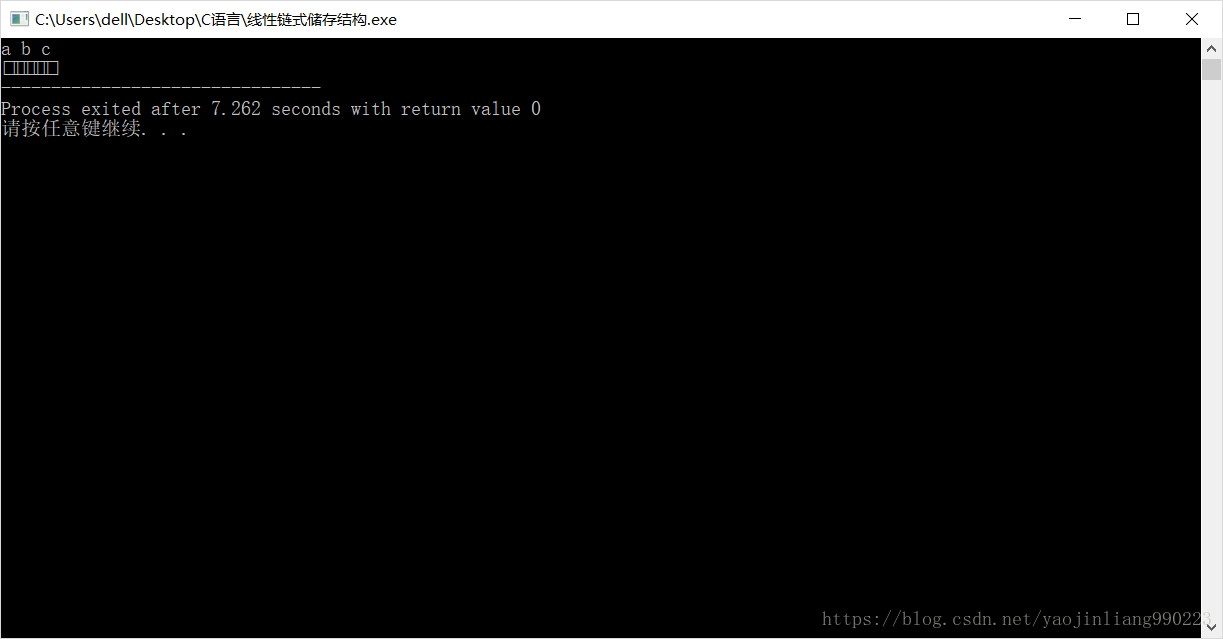