1、入门实例
Compartor<Integer> com = new Compartor<Integer>() {
public int compare(Interger o1,Integer o2) {
return Integer.compare(o1,o2);
}
};
lamdbd表达式的写法
(o1,o2)->Integer.compare(o1,o2);
方法引用
Integer::compare;
2、语法
-> lambda操作符 或者箭头操作符
->左边:lambda形参列表其实就是接口中抽象方法的形参列表
->右边:lambds体 就是重写抽象方法的方法体
3、lambda使用分为6中情况
3.1、无参,无返回值
Runnable r1 = new Runnable() {
@Override
public void run() {
System.out.println("lambda");
}
}
() -> System.out.println("lambda");
3.2、lambda需要一个参数,但是没有返回值
@Test
public void consumerTest(){
Consumer<String> str = new Consumer<String>() {
@Override
public void accept(String s) {
System.out.println(s);
}
};
Consumer<String> str_lambda = (String s) -> {
System.out.println(s);
};
Consumer<String> str_lambda2 = System.out::println;
}
3.3、数据类型的省略
@Test
public void consumerTest(){
Consumer<String> str = new Consumer<String>() {
@Override
public void accept(String s) {
System.out.println(s);
}
};
Consumer<String> str_lambda3 = (s)-> System.out.println(s);
}
3.4、lambda只有一个参数,小括号也可以省略
@Test
public void consumerTest(){
Consumer<String> str = new Consumer<String>() {
@Override
public void accept(String s) {
System.out.println(s);
}
};
Consumer<String> str_lambda3 = s-> System.out.println(s);
}
3.5、lambda需要两个或以上的参数,多条语句执行,并且可以有返回值
@Test
public void comparatorTest(){
Comparator<Integer> comparator = new Comparator<>() {
@Override
public int compare(Integer o1, Integer o2) {
System.out.println(o1);
System.out.println(o2);
return o1.compareTo(o2);
}
};
Comparator<Integer> lambda_comparator = (o1,o2) -> {
System.out.println(o1);
System.out.println(o2);
return o1.compareTo(o2);
};
3.6、当lambda体,只有一条语句,{}可以省略
Consumer<String> str_lambda3 = s-> System.out.println(s);
4、lambda总结
- 左边:可以省略参数类型
- 左边:当只有一个参数时,可以省略小括号
- 右边:当只有一行lambda体是可以省略{}
5、函数式接口
5.1、第一种Consumer 消费性
参数类型:T
返回值:void
描述:对类型T的对象操作,包含方法 void accept(T t)
5.2、第二种Supplier 供给性
参数类型:无
返回值:T
描述:对类型T的对象操作,包含方法 T get();
5.3、第三种
参数类型:T
返回值:R
描述:对类型T的对象操作,包含方法 R Apply(T t)
5.4、第四种
参数类型:T
返回值:boolean
描述:对类型T的对象操作,包含方法 boolean test(T t)
5.5 第五种 其它
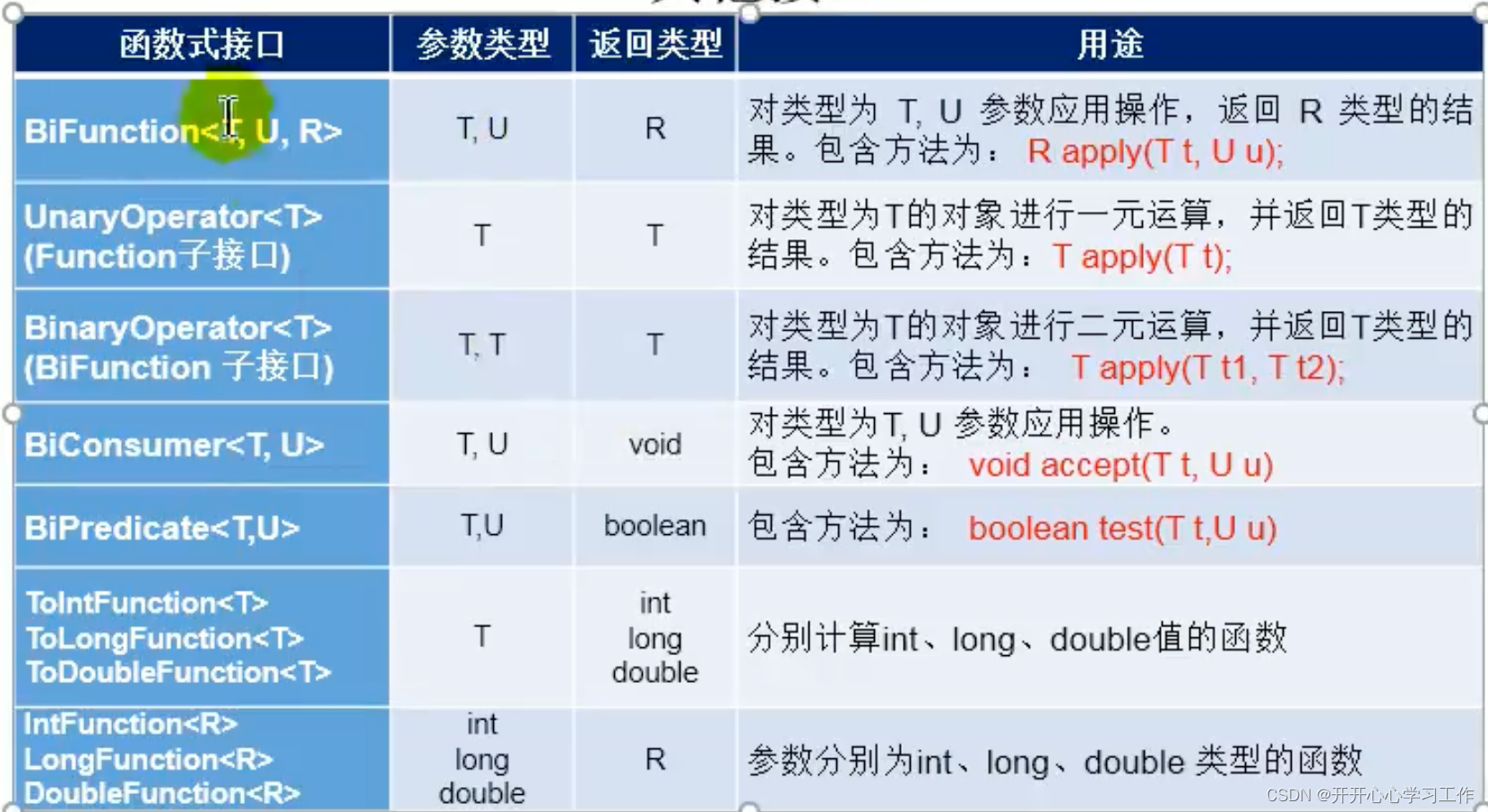
6、进阶语法
- 对象::实例方法名
- 类::静态方法名
- 类::实列方法名
6.1、 第一种 对象::实列方法名
@Test
public void test1(){
Consumer<String> consumer = str -> System.out.println(str);
consumer.accept("测试");
Consumer<String> consumer1 = System.out::println;
consumer1.accept("测试2");
}
@Test
public void test2(){
Employee employee = new Employee(1,"Tom",18,666.66);
Supplier<String> supplier1 = () -> {
return employee.getName();
};
Supplier<String> supplier2 = () -> employee.getName();
supplier2.get();
supplier1.get();
System.out.println("-------------------------");
Supplier<String> supplier3 = employee::getName;
}
6.2、第二种 类::静态方法
@Test
public void test3(){
Comparator<Integer> comparator1 = (o1,o2)->Integer.compare(o1,o2);
Comparator<Integer> comparator2 = Integer::compare;
}
@Test
public void test4() {
Function<Double,Long> function = new Function<>(){
@Override
public Long apply(Double o) {
return Math.round(o);
}
};
Long apply = function.apply(3.4);
long function1 = Math.round(2.2);
Function<Double,Long> = Math::round;
long result = function2.apply(6.6);
}
6.3、第三种 类::实例方法
@Test
public void test5() {
Comparator<String> com1 = (s1,s2)->s1.compareTo(s2);
Comparator<String> com2 = String::compareTo;
System.out.println(com1.compare("1","2"));
System.out.println(com2.compare("1","2"));
System.out.println("=====================");
Comparator<Integer> com3 = (i1,i2) -> i1.compareTo(i2);
Comparator<Integer> com4 = Integer::compareTo;
}
public void test6() {
BiPredicate<String,String> biPredicate1 = (s1,s2) -> s1.equals(s2);
BiPredicate<String,String> biPredicate2 = String::equals;
BiPredicate<Integer,Integer> biPredicate3 = (i1,i2) -> i1.equals(i2);
BiPredicate<Integer,Integer> biPredicate4 = Integer::equals;
}
@Test
public void test7(){
Function<Employee,String> function1 = employee -> employee.getName();
Function<Employee,String> function2 = Employee::getName;
}
6.4、构造器引用
@Test
public void test1(){
Supplier<Employee> supplier1 = ()->new Employee();
Supplier<Employee> supplier2 = Employee::new;
}
public Employee(Integer id) {
this.id = id;
System.out.println("id构造器");
}
@Test
public void test2() {
Function<Integer,Employee> function1 = id->new Employee(id);
Employee apply = function1.apply(1);
System.out.println(JSON.toJSONString(apply));
Function<Integer,Employee> function2 = Employee::new;
Employee employee1 = function1.apply(2);
System.out.println(JSON.toJSONString(employee1));
}
@Test
public void test3() {
BiFunction<Integer,String,Employee> biFunction1 = (id,name)-> new Employee(id,name);
BiFunction<Integer,String,Employee> biFunction2 = Employee::new;
}
6.5、数组引用
@Test
public void test4(){
Function<Integer, String[]> function1 = len -> new String[len];
Function<Integer, String[]> function2 = String[]::new;
}