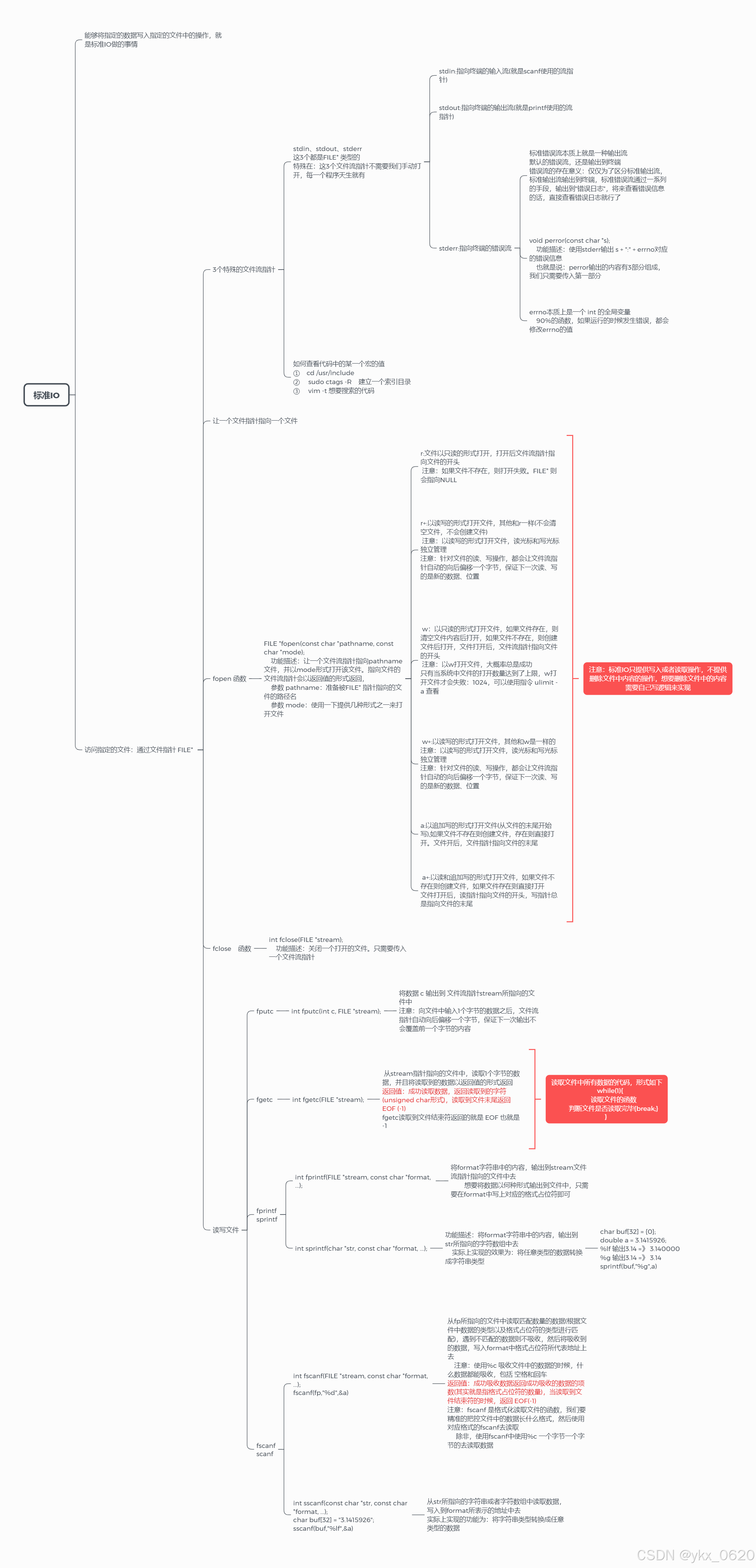
//save.c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
#include <wait.h>
#include <signal.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <sys/msg.h>
#include <sys/shm.h>
#include <sys/un.h>
typedef struct sockaddr_in addr_in_t;
typedef struct sockaddr addr_t;
typedef struct sockaddr_un addr_un_t;
typedef struct Student{
char name[20];
int chinese;
int math;
int english;
int physical;
int chemical;
int biological;
}stu;
typedef struct node
{
union
{
int len;
stu data;
};
struct node *next;
}linklist,*linklistPtr;
linklistPtr create()
{
linklistPtr H = (linklistPtr)malloc(sizeof(linklist));
if (NULL == H)
return NULL;
H->len = 0;
H->next = NULL;
return H;
}
linklistPtr create_node(stu arr)
{
linklistPtr p = (linklistPtr)malloc(sizeof(linklist));
if (NULL == p)
return NULL;
p->data=arr;
return p;
}
int head_add(linklistPtr H, stu arr)
{
if (NULL == H)
return 0;
linklistPtr p = create_node(arr);
p->next = H->next;
H->next = p;
H->len++;
return 1;
}
int head_del(linklistPtr H)
{
if (NULL == H || H->len == 0)
return 0;
linklistPtr p = H->next;
H->next = p->next;
free(p);
p = NULL;
H->len--;
return 1;
}
void my_free(linklistPtr H)
{
if (NULL == H)
return;
while (H->next != NULL)
head_del(H);
free(H);
H = NULL;
return;
}
void filewrite(FILE* wfp, linklistPtr H)
{
linklistPtr p = H->next;
while (p != NULL)
{
printf("a\n");
fprintf(wfp, "%s %d %d %d %d %d %d\n", p->data.name, p->data.chinese, p->data.math, p->data.english, p->data.physical, p->data.chemical, p->data.biological);
p = p->next;
}
}
int main(int argc, const char *argv[])
{
linklistPtr H = create();
stu arr[5] = {
{"aaa", 78, 22, 45, 48, 66, 15},
{"bbb", 45, 68, 88, 99, 47, 68},
{"ccc", 44, 25, 68, 58, 66, 95},
{"ddd", 10, 30, 98, 90, 47, 36},
{"eee", 21, 45, 45, 32, 53, 90}
};
for (int i = 0; i < 5; i++)
{
head_add(H, arr[i]);
}
FILE* wfp = fopen("./linkliststu.txt", "w");
if (wfp == NULL)
{
perror("fopen");
return 1;
}
filewrite(wfp, H);
fclose(wfp);
my_free(H);
H = NULL;
return 0;
}
//load.c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
#include <wait.h>
#include <signal.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#include <sys/msg.h>
#include <sys/shm.h>
#include <sys/un.h>
typedef struct sockaddr_in addr_in_t;
typedef struct sockaddr addr_t;
typedef struct sockaddr_un addr_un_t;
typedef struct Student{
char name[20];
int chinese;
int math;
int english;
int physical;
int chemical;
int biological;
}stu;
int main(int argc, const char *argv[])
{
FILE* rfp = fopen("./linkliststu.txt", "r");
stu arr[20] = {0};
int i = 0;
while (1)
{
int a = fscanf(rfp,"%s %d %d %d %d %d %d", arr[i].name,&arr[i].chinese,&arr[i].math,&arr[i].english,&arr[i].physical,&arr[i].chemical,&arr[i].biological);
if (EOF == a)
break;
i++;
}
fclose(rfp);
for (int j =0; j < i; j++)
{
printf("%s %d %d %d %d %d %d\n", arr[j].name,arr[j].chinese,arr[j].math,arr[j].english,arr[j].physical,arr[j].chemical,arr[j].biological);
}
return 0;
}