一.物流信息管理
import java.io.*;
import java.util.Vector;
class Transactions
{
FileReader tra_fileReader;
BufferedReader tra_in;
String goodinformationA[] = new String[250];
String goodinformationR[] = new String[250];
String goodinformationO[] = new String[250];
String goodinformationD[] = new String[250];
int Acount = 0;
int Rcount = 0;
int Ocount = 0;
int Dcount = 0;
String inv[];
int invcount;
String errormessage[] = new String[1000];
int errorcount = 0;
public Transactions(String inv[], int invcount)
{
this.inv = inv;
this.invcount = invcount;
try
{
tra_fileReader = new FileReader("C:\\Users\\dell\\Desktop\\2019215053 杨雨润 实验三\\Inventory.java\\Transactions.txt");
tra_in = new BufferedReader(tra_fileReader);
String inf;
while((inf = tra_in.readLine()) != null)
{
String trag[] = inf.split("\t");
if(trag[0].equals( "A" ))
goodinformationA[Acount++] = inf;
else if(trag[0].equals( "R" ))
goodinformationR[Rcount++] = inf;
else if(trag[0].equals( "O" ))
goodinformationO[Ocount++] = inf;
else if(trag[0].equals( "D" ))
goodinformationD[Dcount++] = inf;
}
tra_in.close();
}
catch (IOException TraIOE)
{
System.out.println(TraIOE.getMessage());
}
}
public void DealA()
{
for(int i = 0;i < Acount;i++)
{
String mes[] = goodinformationA[i].split("\t");
String itemnumber = mes[1];
int quantity = 0;
String supplier = mes[2];
String description = mes[3];
inv[invcount++] = itemnumber + "\t" + quantity + "\t" + supplier + "\t" + description;
}
}
public void DealR()
{
for(int i = 0;i < Rcount;i++)
{
String Rmes[] = goodinformationR[i].split("\t");
String Rnum = Rmes[1];
int Rgoodcount = Integer.valueOf(Rmes[2]);
for(int j = 0;j < invcount;j++)
{
String invmess[] = inv[j].split("\t");
String invnum = invmess[0];
int invgoodcount = Integer.valueOf(invmess[1]);
if(Rnum.equals(invnum))
{
int newgoodcount = Rgoodcount + invgoodcount;
inv[j] = invnum + "\t" + newgoodcount + "\t" + invmess[2] + "\t" + invmess[3];
}
}
}
}
public void DealO()
{
String reg = "\t";
String shoppingmessage[] = new String[1000];
int shoppingmessagecount = 0;
for(int i = 0; i < Ocount;i++)
{
for(int j = 0;j < Ocount-1;j++)
{
String z;
String n1[] = goodinformationO[j].split(reg);
String n2[] = goodinformationO[j + 1].split(reg);
int num1 = Integer.valueOf(n1[2]);
int num2 = Integer.valueOf(n2[2]);
if(num1 > num2)
{
z = goodinformationO[j];
goodinformationO[j] = goodinformationO[j + 1];
goodinformationO[j + 1] = z;
}
}
}
for(int i = 0;i < Ocount;i++)
{
String Omes[] = goodinformationO[i].split(reg);
String Onum = Omes[1];
int Ogoodcount = Integer.valueOf(Omes[2]);
for(int j = 0;j < invcount;j++)
{
String invmess[] = inv[j].split("\t");
String invnum = invmess[0];
int invgoodcount = Integer.valueOf(invmess[1]);
if(Onum.equals(invnum))
{
if(invgoodcount < Ogoodcount)
{
int shortage = Ogoodcount - invgoodcount;
errormessage[errorcount++] = (invmess[0] + " 号货物暂时缺货 " + shortage + " 件,买家是 " + Omes[3] + "\t" + "\n");
}
else
{
invgoodcount = invgoodcount - Ogoodcount;
inv[j] = invmess[0] + "\t" + invgoodcount + "\t" + invmess[2] + "\t" + invmess[3] ;
shoppingmessage[shoppingmessagecount++] = Onum + "\t" + Ogoodcount + "\t" + Omes[3] + "\n";
}
}
}
}
try
{
Vector<String> shopmessage = new Vector<>();
for(int i = 0;i < shoppingmessagecount;i++)
shopmessage.add(shoppingmessage[i]) ;
for(int i = 0; i < shopmessage.size() - 1; i++)
{
for(int j = i + 1; j < shopmessage.size(); j++)
{
String shop1[] = ( shopmessage.get(i).split("\t") );
String shop2[] = ( shopmessage.get(j).split("\t") );
String goodnum1 = shop1[0];
String goodnum2 = shop2[0];
int goodcount1 = Integer.valueOf(shop1[1]);
int goodcount2 = Integer.valueOf(shop2[1]);
String goodbuyer1 = shop1[2];
String goodbuyer2 = shop2[2];
if( (goodnum1.equals(goodnum2)) && (goodbuyer1.equals(goodbuyer2)) )
{
int newgoodcount = goodcount1 + goodcount2;
String newmessag = goodnum1 + "\t" + newgoodcount + "\t" + goodbuyer1;
shopmessage.remove(i);
shopmessage.remove(i);
shopmessage.add(0, newmessag);
j--;
}
}
}
FileWriter shop_filewrite = new FileWriter("C:\\Users\\dell\\Desktop\\2019215053 杨雨润 实验三\\Inventory.java\\Shopping.txt");
BufferedWriter shop_out = new BufferedWriter(shop_filewrite);
String s = "";
for(int i = 0; i < shopmessage.size();i++)
{
s = s + shopmessage.get(i);
}
shop_out.write(s);
shop_out.close();
}
catch (IOException SIOE)
{
System.out.println(SIOE.getMessage());
}
}
public void DealD()
{
for (int i = 0; i < Dcount; i++)
{
String Dmes[] = goodinformationD[i].split("\t");
String Dnum = Dmes[1];
for (int j = 0; j < invcount; j++)
{
if(inv[j] != null)
{
String invmess[] = inv[j].split("\t");
String invnum = invmess[0];
int invgoodcount = Integer.valueOf(invmess[1]);
if( Dnum.equals(invnum) && invgoodcount == 0)
{
inv[j] = null;
}
else if( Dnum.equals(invnum) && invgoodcount != 0 )
{
errormessage[errorcount++] = (invnum + " 号货物库存不为0,不能被删除" + "\n");
}
}
}
}
}
public void invsort()
{
String newinv[] = new String[1000];
int newinvcount = 0;
for(int i = 0; i < invcount;i++)
{
if(inv[i] != null)
newinv[newinvcount++] = inv[i];
}
for(int i = 0; i < newinvcount;i++)
{
for(int j = 0;j < newinvcount-1;j++)
{
String z;
String n1[] = newinv[j].split("\t");
String n2[] = newinv[j + 1].split("\t");
int num1 = Integer.valueOf(n1[0]);
int num2 = Integer.valueOf(n2[0]);
if(num1 > num2)
{
z = newinv[j];
newinv[j] = newinv[j + 1];
newinv[j + 1] = z;
}
}
}
try
{
FileWriter newinv_filewrite = new FileWriter("C:\\Users\\dell\\Desktop\\2019215053 杨雨润 实验三\\Inventory.java\\NewInventory.txt");
BufferedWriter newinv_out = new BufferedWriter(newinv_filewrite);
String s = "";
for(int i = 0; i < newinvcount;i++)
{
s = s + newinv[i] + "\n";
}
newinv_out.write(s);
newinv_out.close();
}
catch (IOException NIOE)
{
System.out.println(NIOE.getMessage());
}
}
public void showerror()
{
String s = "";
for(int i = 0; i < errorcount;i++)
s = s + errormessage[i];
try
{
FileWriter error_filewrite = new FileWriter("C:\\Users\\dell\\Desktop\\2019215053 杨雨润 实验三\\Inventory.java\\Errors.txt");
BufferedWriter error_out = new BufferedWriter(error_filewrite);
error_out.write(s);
error_out.close();
}
catch (IOException EIOE)
{
System.out.println(EIOE.getMessage());
}
}
}
public class Inventory
{
public static void main(String args[])
{
String inventory[] = new String[1000];
int inv_count = 0;
try
{
FileReader inv_fileReader = new FileReader("C:\\Users\\dell\\Desktop\\2019215053 杨雨润 实验三\\Inventory.java\\Inventory.txt");
BufferedReader inv_in = new BufferedReader(inv_fileReader);
String message;
while((message = inv_in.readLine()) != null)
inventory[inv_count++] = message;
Transactions transactions = new Transactions(inventory, inv_count);
transactions.DealA();
transactions.DealR();
transactions.DealO();
transactions.DealD();
transactions.invsort();
transactions.showerror();
inv_in.close();
}
catch (IOException IOE)
{
System.out.println(IOE.getMessage());
}
}
}
二.map的简单使用
import java.io.*;
import java.util.HashMap;
import java.util.Map;
public class test
{
public static void main(String args[])throws IOException
{
Map<String, String> map = new HashMap< String, String>();
map.put("17","40");
System.out.println(map.get("17"));
map.put("17", "52");
System.out.println(map.get("17"));
}
}
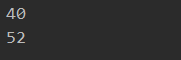