目录
工程目录:
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
<display-name>SpringMvc-01</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd ">
<!-- 使用注解的包,包括子集 -->
<context:component-scan base-package="com.java1234"/>
<!-- 支持对象与json的转换。 -->
<mvc:annotation-driven />
<!-- 视图解析器 -->
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/" />
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
Student.java
package com.java1234.model;
public class Student {
private int id;
private String name;
private int age;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Student() {
super();
// TODO Auto-generated constructor stub
}
public Student(int id, String name, int age) {
super();
this.id = id;
this.name = name;
this.age = age;
}
}
StudentController.java
package com.java1234.controller;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import com.java1234.model.Student;
@Controller
@RequestMapping("/student")
public class StudentController {
private static List<Student> studentList=new ArrayList<Student>();
static {
studentList.add(new Student(1,"张飞",88));
studentList.add(new Student(2,"关羽",67));
studentList.add(new Student(3,"赵云",44));
}
@RequestMapping("/list")
public ModelAndView list() {
ModelAndView mav=new ModelAndView();
mav.addObject("studentList", studentList);
mav.setViewName("student/list");
return mav;
}
@RequestMapping("/preSave")
public ModelAndView preSave(@RequestParam(value="id",required=false) String hh) {
ModelAndView mav=new ModelAndView();
if(hh!=null) {
mav.addObject("stu",studentList.get(Integer.parseInt(hh)-1));
mav.setViewName("student/update");
}else {
mav.setViewName("student/add");
}
return mav;
}
}
add.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/student/save.do" method="post">
<table>
<tr>
<td>姓名:<input type="text" name="name"/></td>
</tr>
<tr>
<td>年龄:<input type="text" name="age"/></td>
</tr>
<tr>
<td><input type="submit" value="提交"/></td>
</tr>
</table>
</form>
</body>
</html>
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<a href="${pageContext.request.contextPath}/student/preSave.do">学生添加</a>
<table>
<tr><th>编号</th><th>姓名</th><th>年龄</th><th colspan="2">操作</th></tr>
<c:forEach var="student" items="${studentList }">
<tr>
<td>${student.id }</td>
<td>${student.name }</td>
<td>${student.age }</td>
<td><a href="${pageContext.request.contextPath}/student/preSave.do?id=${student.id}">修改</a></td>
</tr>
</c:forEach>
</table>
</body>
</html>
update.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/student/save.do" method="post">
<table>
<tr>
<td>姓名:<input type="text" name="name" value="${stu.name }"></td>
</tr>
<tr>
<td>年龄:<input type="text" name="age" value="${stu.age }"/></td>
</tr>
<tr>
<td><input type="hidden" name="id" value="${stu.id }"/></td>
</tr>
<tr>
<td><input type="submit" value="提交"/></td>
</tr>
</table>
</form>
</body>
</html>
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<a href="student/list.do">列表</a>
</body>
</html>
运行:
点击学生添加:
点击修改:
改进StudentController.java
package com.java1234.controller;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import com.java1234.model.Student;
@Controller
@RequestMapping("/student")
public class StudentController {
private static List<Student> studentList=new ArrayList<Student>();
static {
studentList.add(new Student(1,"张飞",88));
studentList.add(new Student(2,"关羽",67));
studentList.add(new Student(3,"赵云",44));
}
@RequestMapping("/list")
public ModelAndView list() {
ModelAndView mav=new ModelAndView();
mav.addObject("studentList", studentList);
mav.setViewName("student/list");
return mav;
}
@RequestMapping("/preSave")
public ModelAndView preSave(@RequestParam(value="id",required=false) String hh) {
ModelAndView mav=new ModelAndView();
if(hh!=null) {
mav.addObject("stu",studentList.get(Integer.parseInt(hh)-1));
mav.setViewName("student/update");
}else {
mav.setViewName("student/add");
}
return mav;
}
@RequestMapping("/save")
public String save(Student student){
if(student.getId()!=0){
Student s=studentList.get(student.getId()-1);
s.setName(student.getName());
s.setAge(student.getAge());
}else{
studentList.add(student);
}
// return "redirect:/student/list.do";
return "forward:/student/list.do";
}
@RequestMapping("/delete")
public String delete(@RequestParam("id") int id){
studentList.remove(id-1);
return "redirect:/student/list.do";
}
}
SpringMvc的自动封装对象功能: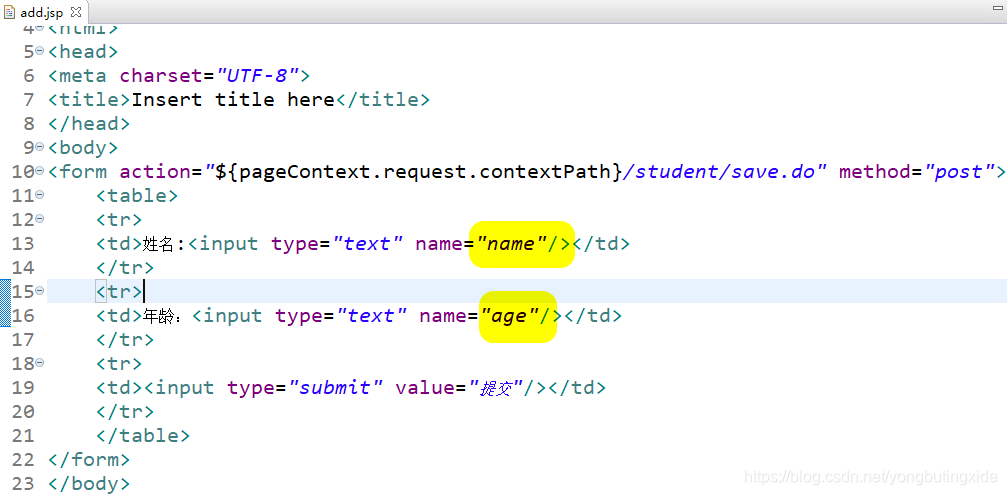
乱码问题:
在web.xml中添加编码相关的过滤器,解决乱码问题
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
<display-name>SpringMvc-01</display-name>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
</web-app>
SpringMvc对servlet API的支持:
SpringMvc可以直接引用servelet中的相关对象,比如request,response,session等