class Commitent{
static PENDING='pending'
static FULFILLED='fulfilled'
static REJECTED='rejected'
constructor(func){
this.result=null
this.status=Commitent.PENDING
//保存函数
this.rejectCallbacks=[]
this.resolveCallbacks=[]
try{
// 绑定this,在新实例创建后再实现resolve,reject方法,和实例不是一起执行的
func(this.resolve.bind(this),this.reject.bind(this))
}catch(error){
// 立即执行,不是新实例创建后执行,不用绑定this
this.reject(error)
}
}
// reject和resolve是要在事件循环末尾执行的
reject(result){
setTimeout(() => {
if(this.status===Commitent.PENDING){
this.status=Commitent.REJECTED
this.result=result
this.rejectCallbacks.forEach(callback=>{
callback(result)
})
}
}, )
}
resolve(result){
setTimeout(() => {
if(this.status===Commitent.PENDING){
this.status=Commitent.FULFILLED
this.result=result
this.resolveCallbacks.forEach(callback=>{
callback(result)
})
}
}, )
}
// then是在resolve或reject执行完毕之后才执行的
then(OnFULFILLED,OnREJECTED){
// 实现链式调用
return new Commitent((resolve,reject)=>{
//解决传入参数不是一个函数的情况
OnFULFILLED =typeof OnFULFILLED==='function'? OnFULFILLED:()=>{}
OnREJECTED =typeof OnREJECTED==='function'? OnREJECTED:()=>{}
if(this.status===Commitent.FULFILLED){
setTimeout(() => {
OnFULFILLED(this.result)
}, )
}
if(this.status===Commitent.REJECTED){
setTimeout(() => {
OnREJECTED(this.result)
}, )
}
// 出现PENDING状态是因为then执行得过早了
if(this.status===Commitent.PENDING){
this.resolveCallbacks.push(OnFULFILLED)
this.rejectCallbacks.push(OnREJECTED)
}
})
}
}
const commitent=new Commitent((res,rej)=>{
res('123')
}).then(
result=>{
console.log(result)
}
)
手写promise
最新推荐文章于 2024-11-02 19:19:35 发布
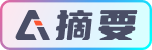