一、项目目录结构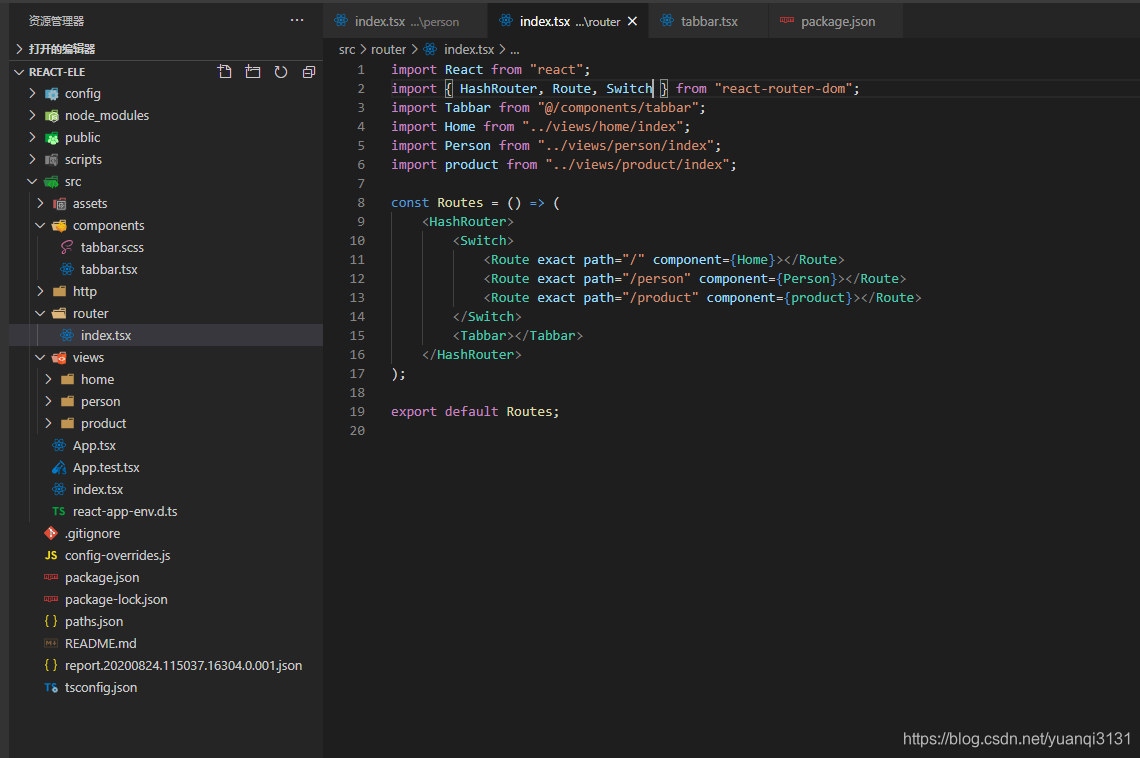
二、App.tsx
import React from "react";
import Routes from "./router/index";
function App() {
return <Routes></Routes>;
}
export default App;
三、tabbar.tsx
import React, { Component } from "react";
import { TabBar } from "antd-mobile";
import { withRouter, RouteComponentProps } from "react-router-dom";
import "./tabbar.scss";
interface IProps extends RouteComponentProps {}
interface IState {}
class Tabbar extends Component<IProps, IState> {
constructor(prop: IProps) {
super(prop);
}
state: IState = {};
render(): any {
const pathname = this.props.location.pathname;
return (
<div className="tabbar">
<TabBar
unselectedTintColor="#949494"
tintColor="#33A3F4"
barTintColor="white"
>
<TabBar.Item
icon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/home.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
selectedIcon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/home_active.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
title="首页"
key="Home"
selected={pathname === "/"}
onPress={() => {
this.props.history.replace("/");
}}
></TabBar.Item>
<TabBar.Item
icon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/discover.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
selectedIcon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/discover_active.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
title="发现"
key="Product"
selected={pathname === "/product"}
onPress={() => {
this.props.history.replace("/product");
}}
></TabBar.Item>
<TabBar.Item
icon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/my.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
selectedIcon={
<div
style={{
width: "22px",
height: "22px",
background: `url(${require("@/assets/image/my_active.png")}) center center / 21px 21px no-repeat`,
}}
/>
}
title="我的"
key="Person"
selected={pathname === "/person"}
onPress={() => {
this.props.history.replace("/person");
}}
></TabBar.Item>
</TabBar>
</div>
);
}
}
export default withRouter(Tabbar);