1、示例说明
本示例为基于行字符串的回显应用示例,客户端通过标准输入获取行字符串,并将字符串发送到服务端;服务端读取客户端行数据,解码之后将数据回写给客户端,同事服务端实现与客户端的读写心跳检测等;
2、服务端说明
服务端基于netty创建,解码器为LineBasedFrameDecoder和StringDecoder,主要功能是将ByteBuf类型数据解码转换为String类型数据;心跳机制基于IdleStateHandler实现;
2.1、服务端代码示例
SocketEchoServer 源码:
public class SocketEchoServer {
private static int PORT = 9999;
public static void main(String[] args){
SocketEchoServer.startServer(PORT);
}
private static void startServer(int port){
NioEventLoopGroup bossGroup = new NioEventLoopGroup(1);
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap sb = new ServerBootstrap();
sb.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.option(ChannelOption.SO_BACKLOG, 256)
.childOption(ChannelOption.SO_KEEPALIVE, true)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception {
socketChannel.pipeline().addLast("lineDecodeHandler", new LineBasedFrameDecoder(1024, false, false))
.addLast("stringDecoder", new StringDecoder())
.addLast("heartBeatHandler", new IdleStateHandler(0,0, 30))
.addLast("echoHandler", new SocketEchoServerHandler());
}
});
ChannelFuture cf = sb.bind(PORT).sync();
System.out.println("---- server bind success on port:" + PORT + " ----");
cf.channel().closeFuture().sync();
}catch (Exception e){
e.printStackTrace();
}finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
说明:
- 创建服务端Channel的事件处理线程组bossGroup,本处设置线程个数为1个;
- 创建客户端连接Channel的事件处理线程组workerGroup,线程个数为默认(处理器个数的2倍);
- 创建ServerBootstrap并设置相关参数,设置服务端Channel为NioServerSocketChannel,设置服务端的连接缓存为256,添加子连接的ChannelHandler为LineBasedFrameDecoder、StringDecoder、IdleStateHandler、SocketEchoServerHandler;
- 绑定本地端口9999,并同步等待绑定结果;
- 同步等待服务端监听连接的关闭结果;
2.2、SocketEchoServerHandler示例
SocketEchoServerHandler源码:
public class SocketEchoServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
InetSocketAddress address = (InetSocketAddress)ctx.channel().remoteAddress();
System.out.println("---- client active: host=" + address.getHostName() + ",port=" + address.getPort() + "----");
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
InetSocketAddress address = (InetSocketAddress)ctx.channel().remoteAddress();
System.out.println("---- client inactive: host=" + address.getHostName() + ",port=" + address.getPort() + "----");
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if(msg instanceof String){
String str = (String)msg;
ctx.writeAndFlush(Unpooled.copiedBuffer(str.getBytes()));
System.out.println("recv form client:" + str);
return;
}
}
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if(evt instanceof IdleStateEvent){
IdleStateEvent event = (IdleStateEvent) evt;
DateTimeFormatter format = DateTimeFormat.forPattern("yyyy-MM-dd HH:mm:ss");
String dt = DateTime.now().toString(format);
switch (event.state()){
case ALL_IDLE:
System.out.println(dt + ":服务端与客户端读写超时!");
break;
case READER_IDLE:
System.out.println(dt + ":服务端读超时!");
break;
case WRITER_IDLE:
System.out.println(dt + ":服务端写超时!");
break;
}
}
}
}
channelRead:读取解码器解码转换后的String对象,将对象回写到对应的客户端;
userEventTriggered:若事件类型为IdleStateEvent表明为心跳检测事件,获取对应事件类型并打印;
3、客户端说明
客户端主要功能为连接服务器,从标准输入中读取行字符串,将行数据发送给服务端,同时接收服务端回写的数据;
3.1、客户端示例
SocketEchoClient源码:
public class SocketEchoClient {
private static String LOCAL_HOST = "127.0.0.1";
private static int SERVER_PORT = 9999;
public static void main(String[] args){
SocketEchoClient.startClient(SERVER_PORT);
}
private static void startClient(int serverPort){
NioEventLoopGroup group = new NioEventLoopGroup(1);
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new ChannelInitializer<NioSocketChannel>() {
@Override
protected void initChannel(NioSocketChannel nioSocketChannel) throws Exception {
nioSocketChannel.pipeline().addLast("lineDecodeHandler", new LineBasedFrameDecoder(1024, false, false))
.addLast("stringDecoder",new StringDecoder())
.addLast("echoClientHandler", new SocketEchoClientHandler());
}
});
ChannelFuture cf = b.connect(LOCAL_HOST, SERVER_PORT).sync();
System.out.println("client connect to server success! host:" + LOCAL_HOST + ":" + SERVER_PORT);
cf.channel().closeFuture().sync();
}catch (Exception e){
e.printStackTrace();
}finally {
group.shutdownGracefully();
}
}
}
说明:
- 创建一个线程的EventLoopGroup;
- 设置客户端Channel类型为NioSocketChannel;
- 设置客户端ChannelHandler的为LineBasedFrameDecoder、StringDecoder及SocketEchoClientHandler;
- 同步连接服务器;
3.2、SocketEchoClientHandler示例
SocketEchoClientHandler源码:
public class SocketEchoClientHandler extends ChannelInboundHandlerAdapter{
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if(msg instanceof String){
String str = (String)msg;
System.out.println("recv form server:" + str);
return;
}
}
@Override
public void channelActive(final ChannelHandlerContext ctx) throws Exception {
try {
new Thread(new Runnable() {
public void run() {
Scanner scanner = new Scanner(System.in);
while (scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("##stop")){
ctx.close();
break;
}
line += System.getProperty("line.separator");
System.out.println(line);
ctx.writeAndFlush(Unpooled.copiedBuffer(line.getBytes()));
}
}
}).start();
}catch (Exception e){
e.printStackTrace();
ctx.close();
}
}
}
channelRead:读取服务端回写数据;
channelActive:客户端连接成功后,创建并启动读取标准输入处理的线程,线程中读取行数据并将数据发送给服务端;
4、应用演示示例
以下为服务端及客户端的实例输入输出,序号为时序。
服务端:
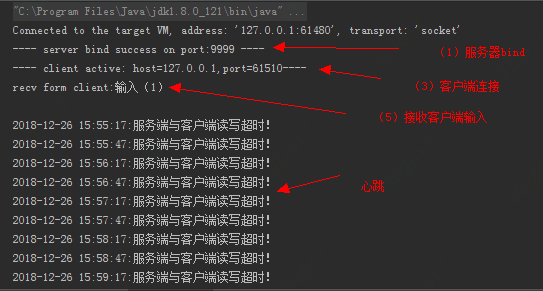
客户端:
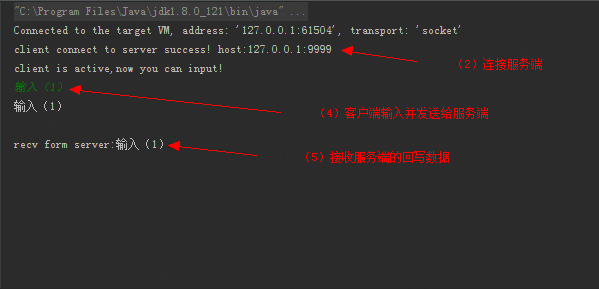
应用示例源码:https://github.com/zhaozhou11/netty-demo.git
Netty源码愫读:
Netty源码愫读(一)ByteBuf相关源码学习 【https://www.jianshu.com/p/016daa404957】
Netty源码愫读(二)Channel相关源码学习【https://www.jianshu.com/p/02eac974258e】
Netty源码愫读(三)ChannelPipeline、ChannelHandlerContext相关源码学习【https://www.jianshu.com/p/be82d0fcdbcc】
Netty源码愫读(四)ChannelHandler相关源码学习【https://www.jianshu.com/p/6ee0a3b9d73a】
Netty源码愫读(五)EventLoop与EventLoopGroup相关源码学习【https://www.jianshu.com/p/05096995d296】
Netty源码愫读(六)ServerBootstrap相关源码学习【https://www.jianshu.com/p/a71a9a0291f3】
Netty应用实战: