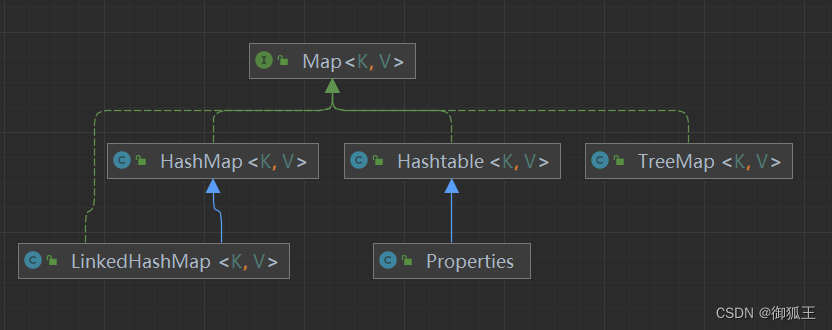
java学习Day09 Map
Map 和 Collection
public class Map_ {
@SuppressWarnings({"all"})
public static void main(String[] args) {
Map map = new HashMap();
map.put("no1","arthur");
map.put("no2","dutch");
map.put("no2","alice");
map.put("no3","alice");
map.put(null,null);
map.put(null,"john");
map.put("no4",null);
map.put("no5",null);
map.put(new Object(),"sadie");
System.out.println(map);
System.out.println(map.get("no3"));
}
}
Map源码
public class MapSource {
@SuppressWarnings({"all"})
public static void main(String[] args) {
Map map = new HashMap();
map.put("no1","arthur");
map.put("no2","dutch");
Set set = map.entrySet();
System.out.println(set.getClass());
for (Object o :set) {
Map.Entry entry = (Map.Entry) o;
System.out.println(entry.getKey()+" "+entry.getValue());
}
}
Map常用方法
public class MapMethod {
@SuppressWarnings({"all"})
public static void main(String[] args) {
Map map = new HashMap();
map.put("john",new book("",120));
map.put("john","dutch");
map.put("baoq","mr");
map.put("songz","mr");
map.put("arthur",null);
map.put(null,"yifei");
map.put("mm","xt");
System.out.println(map);
map.remove(null);
System.out.println(map);
System.out.println(map.get("mm"));
System.out.println(map.size());
System.out.println(map.isEmpty());
map.clear();
System.out.println(map.containsKey("mr"));
}
}
class book{
private String name;
private int num;
public book(String name, int num) {
this.name = name;
this.num = num;
}
}
Map循环
public class MapFor {
@SuppressWarnings({"all"})
public static void main(String[] args) {
Map map = new HashMap();
map.put("john",new book("",120));
map.put("john","dutch");
map.put("baoq","mr");
map.put("songz","mr");
map.put("arthur",null);
map.put(null,"yifei");
map.put("mm","xt");
Set keySet = map.keySet();
for (Object key :keySet) {
System.out.println(key+" "+map.get(key));
}
System.out.println("==================");
Iterator iterator = keySet.iterator();
while (iterator.hasNext()) {
Object key = iterator.next();
System.out.println(key+" "+map.get(key));
}
Collection values = map.values();
for (Object value :values) {
System.out.println(value);
}
Iterator iterator1 = values.iterator();
while (iterator1.hasNext()) {
Object key = iterator1.next();
System.out.println(key);
}
System.out.println("==================");
Set entrySet = map.entrySet();
for (Object entry :entrySet) {
Map.Entry m = (Map.Entry) entry;
System.out.println(m.getKey()+" "+m.getValue());
}
Iterator iterator2 = entrySet.iterator();
while (iterator2.hasNext()) {
Object entry = iterator2.next();
Map.Entry m =(Map.Entry) entry;
System.out.println(m.getKey()+" "+m.getValue());
}
}
}
MapExercise
public class MapExercise {
public static void main(String[] args) {
Map hashMap = new HashMap();
hashMap.put(1,new employee("alice",10,50000,1));
hashMap.put(2,new employee("sadie",9,6000,2));
hashMap.put(3,new employee("violet",12,70000,3));
Set set = hashMap.keySet();
for (Object key :set) {
employee employee= (com.yuhuw.collection.map.employee) hashMap.get(key);
if (employee.getSar() >18000) {
System.out.println(employee);
}
}
Set entrySet = hashMap.entrySet();
for (Object entry :entrySet) {
Map.Entry map = (Map.Entry) entry;
employee employee = (com.yuhuw.collection.map.employee) map.getValue();
if (employee.getSar() >18000) {
System.out.println(employee);
}
}
System.out.println("====================");
Iterator iterator = entrySet.iterator();
while (iterator.hasNext()) {
Object entry= iterator.next();
Map.Entry map = (Map.Entry) entry;
employee employee = (com.yuhuw.collection.map.employee) map.getValue();
if (employee.getSar() >18000){
System.out.println(employee);
}
}
}
}
class employee{
private String name;
private int age;
private double sar;
private int id;
public employee(String name, int age, double sar, int id) {
this.name = name;
this.age = age;
this.sar = sar;
this.id = id;
}
public double getSar() {
return sar;
}
public void setSar(double sar) {
this.sar = sar;
}
@Override
public String toString() {
return "employee{" +
"name='" + name + '\'' +
", age=" + age +
", sar=" + sar +
", id=" + id +
'}';
}
}