| | 图 1-1是一个用户、机构、角色管理的模块,使用的是RBAC模型(Role-Based Access Control,基于角色的访问控制), 不过这个接口设计的有问题,用户的属性和行为没有分开,这是一个严重的错误! 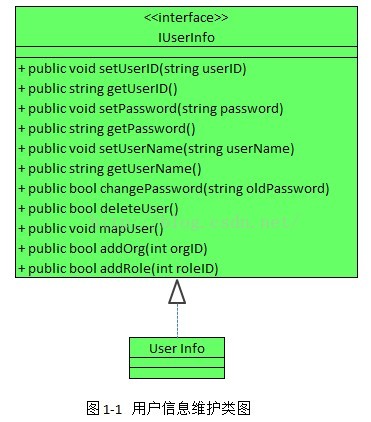
应该把用户信息抽取成一个BO(Business Object, 业务对象),IUserBO负责用户的属性,IUserBO 的职责就是收集和反馈 用户的属性信息;把行为抽取成一个Biz(Business Logic, 业务逻辑),IUserBiz负责用户的行为,完成用户信息的维护和 变更。按照这个思路对类图进行修正。 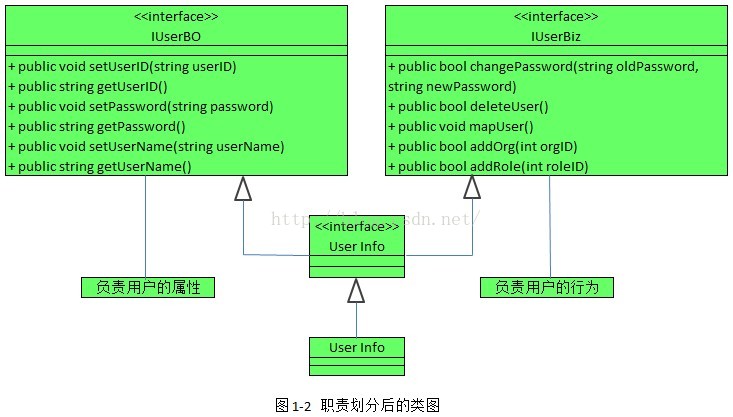
C++代码实现:
#*************************************************************************************#
#** @file : Makefile **#
#** @author : CC ^_^……!!! **#
#** @date : 2015.11.30 13:24:41 **#
#*************************************************************************************#
DIR_INC = ./inc
DIR_SRC = ./src
DIR_OBJ = ./obj
DIR_BIN = ./bin
SRC = $(wildcard ${DIR_SRC}/*.cpp)
OBJ = $(patsubst %.cpp, ${DIR_OBJ}/%.o, $(notdir ${SRC}))
TARGET = test_main
BIN_TARGET = ${DIR_BIN}/${TARGET}
CPP = g++
CFLAGS = -g -Wall -I ${DIR_INC}
${DIR_OBJ}/%.o:${DIR_SRC}/%.cpp
@echo $(SRC)
@echo $(OBJ)
${CPP} ${CFLAGS} -c $< -o $@
${BIN_TARGET}:${OBJ}
${CPP} ${OBJ} -o $@
.PHONY:clean
clean:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
clean_all:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
rm -rf ${DIR_BIN}/*
/*************************************************************************************/
/** @file : comm.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:18:03 **/
/*************************************************************************************/
#ifndef COMM_H
#define COMM_H
#include
#include
using namespace std;
#endif /** COMM_H **/
/*************************************************************************************/
/** @file : IUserBO.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:20:59 **/
/*************************************************************************************/
#ifndef IUSRE_BO_H
#define IUSRE_BO_H
#include "comm.h"
/*************************************************************************************/
/** @class : 用户属性接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IUserBO
{
public:
/** 设置用户ID **/
virtual void setUserID(string userID) = 0;
/** 获取用户ID **/
virtual string getUserID() = 0;
/** 设置用户姓名 **/
virtual void setUserName(string userName) = 0;
/** 获取用户姓名 **/
virtual string getUserName() = 0;
/** 设置用户密码 **/
virtual void setPassword(string password) = 0;
/** 获取用户密码 **/
virtual string getPassword() = 0;
};
#endif /** IUSRE_BO_H **/
/*************************************************************************************/
/** @file : IUserBiz.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:21:25 **/
/** : 2015年12月1日 10:07:13 **/
/*************************************************************************************/
#ifndef IUSRE_BIZ_H
#define IUSRE_BIZ_H
#include "comm.h"
/*************************************************************************************/
/** @class : 用户逻辑接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IUserBiz
{
public:
/** 修改密码 **/
virtual bool changePassword(string oldPassword, string newPassword) = 0;
/** 删除用户 **/
virtual bool deleteUser() = 0;
/** 映射用户 **/
virtual void mapUser() = 0;
/** 添加组织 **/
virtual bool addOrg(int orgID) = 0;
/** 添加角色 **/
virtual bool addRole(int roleID) = 0;
};
#endif /** IUSRE_BIZ_H **/
/*************************************************************************************/
/** @file : IUserInfo.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:21:43 **/
/*************************************************************************************/
#ifndef IUSRE_INFO_H
#define IUSRE_INFO_H
#include "comm.h"
#include "IUserBO.h"
#include "IUserBiz.h"
/*************************************************************************************/
/** @class : 用户信息接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IUserInfo : public IUserBO, public IUserBiz
{
};
#endif /** IUSRE_INFO_H **/
/*************************************************************************************/
/** @file : UserInfo.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:21:59 **/
/** : 2015年12月1日 10:06:54 **/
/*************************************************************************************/
#ifndef USRE_INFO_H
#define USRE_INFO_H
#include "comm.h"
#include "IUserInfo.h"
/*************************************************************************************/
/** @class : 用户信息类(class) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class UserInfo : public IUserInfo
{
private:
/** 用户ID **/
string userID;
/** 用户姓名 **/
string userName;
/** 用户密码 **/
string password;
public:
/** 用户信息类构造函数 **/
UserInfo();
/** 用户信息类构造函数(带参数) **/
UserInfo(string userID, string userName, string password);
/** 用户信息类析构函数 **/
~UserInfo();
/** 设置用户ID **/
void setUserID(string userID);
/** 获取用户ID **/
string getUserID();
/** 设置用户姓名 **/
void setUserName(string userName);
/** 获取用户姓名 **/
string getUserName();
/** 设置用户密码 **/
void setPassword(string password);
/** 获取用户密码 **/
string getPassword();
/** 修改密码 **/
bool changePassword(string oldPassword, string newPassword);
/** 删除用户 **/
bool deleteUser();
/** 映射用户 **/
void mapUser();
/** 添加组织 **/
bool addOrg(int orgID);
/** 添加角色 **/
bool addRole(int roleID);
};
#endif /** USRE_INFO_H **/
/*************************************************************************************/
/** @file : UserInfo.cpp **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:22:23 **/
/** : 2015年12月1日 10:06:40 **/
/*************************************************************************************/
#include "UserInfo.h"
/*************************************************************************************/
/** @function : 用户信息类构造函数 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserInfo::UserInfo()
{
this->userID = "";
this->userName = "";
this->password = "";
}
/*************************************************************************************/
/** @function : 用户信息类构造函数(带参数) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserInfo::UserInfo(string userID, string userName, string password)
{
this->userID = userID;
this->userName = userName;
this->password = password;
}
/*************************************************************************************/
/** @function : 用户信息类析构造函数 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserInfo::~UserInfo()
{
this->userID = "";
this->userName = "";
this->password = "";
}
/*************************************************************************************/
/** @function : 设置用户ID **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserInfo::setUserID(string userID)
{
this->userID = userID;
}
/*************************************************************************************/
/** @function : 获取用户ID **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserInfo::getUserID()
{
return this->userID;
}
/*************************************************************************************/
/** @function : 设置用户姓名 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserInfo::setUserName(string userName)
{
this->userName = userName;
}
/*************************************************************************************/
/** @function : 获取用户姓名 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserInfo::getUserName()
{
return this->userName;
}
/*************************************************************************************/
/** @function : 设置用户密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserInfo::setPassword(string password)
{
this->password = password;
}
/*************************************************************************************/
/** @function : 获取用户密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserInfo::getPassword()
{
return this->password;
}
/*************************************************************************************/
/** @function : 修改密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
bool UserInfo::changePassword(string oldPassword, string newPassword)
{
if (this->password == oldPassword)
{
this->password = newPassword;
cout<<"Change password successfully."<
不过在实际是使用中,我们更倾向于使用两个不同的类或接口,一个是IUserBO,一个是IUserBiz,类图如1-3所示: 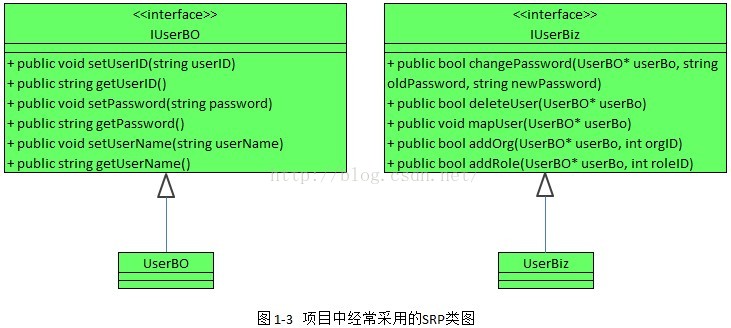
C++代码实现:
#*************************************************************************************#
#** @file : Makefile **#
#** @author : CC ^_^……!!! **#
#** @date : 2015.11.30 13:24:41 **#
#*************************************************************************************#
DIR_INC = ./inc
DIR_SRC = ./src
DIR_OBJ = ./obj
DIR_BIN = ./bin
SRC = $(wildcard ${DIR_SRC}/*.cpp)
OBJ = $(patsubst %.cpp, ${DIR_OBJ}/%.o, $(notdir ${SRC}))
TARGET = test_main
BIN_TARGET = ${DIR_BIN}/${TARGET}
CPP = g++
CFLAGS = -g -Wall -I ${DIR_INC}
${DIR_OBJ}/%.o:${DIR_SRC}/%.cpp
@echo $(SRC)
@echo $(OBJ)
${CPP} ${CFLAGS} -c $< -o $@
${BIN_TARGET}:${OBJ}
${CPP} ${OBJ} -o $@
.PHONY:clean
clean:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
clean_all:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
rm -rf ${DIR_BIN}/*
/*************************************************************************************/
/** @file : comm.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:18:03 **/
/*************************************************************************************/
#ifndef COMM_H
#define COMM_H
#include
#include
using namespace std;
#endif /** COMM_H **/
/*************************************************************************************/
/** @file : IUserBO.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:48:16 **/
/*************************************************************************************/
#ifndef IUSRE_BO_H
#define IUSRE_BO_H
#include "comm.h"
/*************************************************************************************/
/** @class : 用户属性接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IUserBO
{
public:
/** 设置用户ID **/
virtual void setUserID(string userID) = 0;
/** 获取用户ID **/
virtual string getUserID() = 0;
/** 设置用户姓名 **/
virtual void setUserName(string userName) = 0;
/** 获取用户姓名 **/
virtual string getUserName() = 0;
/** 设置用户密码 **/
virtual void setPassword(string password) = 0;
/** 获取用户密码 **/
virtual string getPassword() = 0;
};
#endif /** IUSRE_BO_H **/
/*************************************************************************************/
/** @file : IUserBiz.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:48:38 **/
/** : 2015年12月1日 10:05:32 **/
/*************************************************************************************/
#ifndef IUSRE_BIZ_H
#define IUSRE_BIZ_H
#include "comm.h"
#include "UserBO.h"
/*************************************************************************************/
/** @class : 用户逻辑接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IUserBiz
{
public:
/** 修改密码 **/
virtual bool changePassword(UserBO* userBo, string oldPassword, string newPassword) = 0;
/** 删除用户 **/
virtual bool deleteUser(UserBO* userBo) = 0;
/** 映射用户 **/
virtual void mapUser(UserBO* userBo) = 0;
/** 添加组织 **/
virtual bool addOrg(UserBO* userBo, int orgID) = 0;
/** 添加角色 **/
virtual bool addRole(UserBO* userBo, int roleID) = 0;
};
#endif /** IUSRE_BIZ_H **/
/*************************************************************************************/
/** @file : UserBO.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:41:14 **/
/** : 2015年12月1日 10:08:53 **/
/*************************************************************************************/
#ifndef USRE_BO_H
#define USRE_BO_H
#include "comm.h"
#include "IUserBO.h"
/*************************************************************************************/
/** @class : 用户属性类(class) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class UserBO : public IUserBO
{
private:
/** 用户ID **/
string userID;
/** 用户姓名 **/
string userName;
/** 用户密码 **/
string password;
public:
/** 用户信息类构造函数 **/
UserBO();
/** 用户信息类构造函数(带参数) **/
UserBO(string userID, string userName, string password);
/** 用户信息类析构函数 **/
~UserBO();
/** 设置用户ID **/
void setUserID(string userID);
/** 获取用户ID **/
string getUserID();
/** 设置用户姓名 **/
void setUserName(string userName);
/** 获取用户姓名 **/
string getUserName();
/** 设置用户密码 **/
void setPassword(string password);
/** 获取用户密码 **/
string getPassword();
};
#endif /** USRE_BO_H **/
/*************************************************************************************/
/** @file : UserBiz.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:48:47 **/
/** : 2015年12月1日 10:05:14 **/
/*************************************************************************************/
#ifndef USRE_BIZ_H
#define USRE_BIZ_H
#include "comm.h"
#include "IUserBiz.h"
/*************************************************************************************/
/** @class : 用户逻辑类(class) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class UserBiz : public IUserBiz
{
public:
/** 修改密码 **/
bool changePassword(UserBO* userBo, string oldPassword, string newPassword);
/** 删除用户 **/
bool deleteUser(UserBO* userBo);
/** 映射用户 **/
void mapUser(UserBO* userBo);
/** 添加组织 **/
bool addOrg(UserBO* userBo, int orgID);
/** 添加角色 **/
bool addRole(UserBO* userBo, int roleID);
};
#endif /** USRE_BIZ_H **/
/*************************************************************************************/
/** @file : UserBO.cpp **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:48:56 **/
/*************************************************************************************/
#include "UserBO.h"
/*************************************************************************************/
/** @function : 用户属性类构造函数 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserBO::UserBO()
{
this->userID = "";
this->userName = "";
this->password = "";
}
/*************************************************************************************/
/** @function : 用户属性类构造函数(带参数) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserBO::UserBO(string userID, string userName, string password)
{
this->userID = userID;
this->userName = userName;
this->password = password;
}
/*************************************************************************************/
/** @function : 用户属性类析构造函数 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
UserBO::~UserBO()
{
this->userID = "";
this->userName = "";
this->password = "";
}
/*************************************************************************************/
/** @function : 设置用户ID **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserBO::setUserID(string userID)
{
this->userID = userID;
}
/*************************************************************************************/
/** @function : 获取用户ID **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserBO::getUserID()
{
return this->userID;
}
/*************************************************************************************/
/** @function : 设置用户姓名 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserBO::setUserName(string userName)
{
this->userName = userName;
}
/*************************************************************************************/
/** @function : 获取用户姓名 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserBO::getUserName()
{
return this->userName;
}
/*************************************************************************************/
/** @function : 设置用户密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void UserBO::setPassword(string password)
{
this->password = password;
}
/*************************************************************************************/
/** @function : 获取用户密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string UserBO::getPassword()
{
return this->password;
}
/*************************************************************************************/
/** @file : UserBiz.cpp **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 16:53:49 **/
/** : 2015年12月1日 10:04:42 **/
/*************************************************************************************/
#include "UserBiz.h"
/*************************************************************************************/
/** @function : 修改密码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
bool UserBiz::changePassword(UserBO* userBo, string oldPassword, string newPassword)
{
if (userBo->getPassword() == oldPassword)
{
userBo->setPassword(newPassword);
cout<<"Change password successfully."<
getUserName()<<" Add a organization:"<
<
getUserName()<<" Add a role:"<
<
图2-1是电话管理的模块,Iphone这个接口包含了两个职责:一个是协议管理,一个是数据传送,dial()和hangup() 两个方法实现的是协议管理,分别负责拨号接通和挂机;call()和answer()实现了数据传送(通话)。 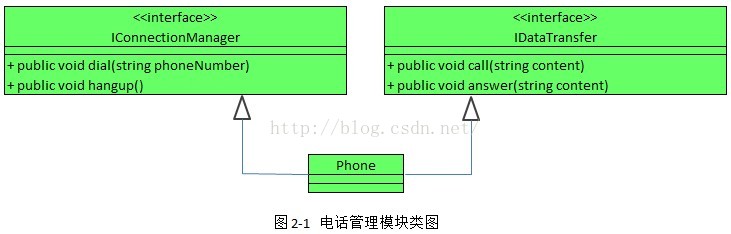 C++代码实现:
#*************************************************************************************#
#** @file : Makefile **#
#** @author : CC ^_^……!!! **#
#** @date : 2015.11.30 13:24:41 **#
#*************************************************************************************#
DIR_INC = ./inc
DIR_SRC = ./src
DIR_OBJ = ./obj
DIR_BIN = ./bin
SRC = $(wildcard ${DIR_SRC}/*.cpp)
OBJ = $(patsubst %.cpp, ${DIR_OBJ}/%.o, $(notdir ${SRC}))
TARGET = test_main
BIN_TARGET = ${DIR_BIN}/${TARGET}
CPP = g++
CFLAGS = -g -Wall -I ${DIR_INC}
${DIR_OBJ}/%.o:${DIR_SRC}/%.cpp
@echo $(SRC)
@echo $(OBJ)
${CPP} ${CFLAGS} -c $< -o $@
${BIN_TARGET}:${OBJ}
${CPP} ${OBJ} -o $@
.PHONY:clean
clean:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
clean_all:
find ${DIR_OBJ} -name *.o -exec rm -rf {} \;
rm -rf ${DIR_BIN}/*
/*************************************************************************************/
/** @file : comm.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 13:18:03 **/
/*************************************************************************************/
#ifndef COMM_H
#define COMM_H
#include
#include
using namespace std;
#endif /** COMM_H **/
/*************************************************************************************/
/** @file : IConnectionManager.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 15:12:12 **/
/*************************************************************************************/
#ifndef ICONNECTION_MANAGER_H
#define ICONNECTION_MANAGER_H
#include "comm.h"
/*************************************************************************************/
/** @class : 手机协议管理接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IConnectionManager
{
public:
/** 拨打电话 **/
virtual void dial(string dialPhoneNumber) = 0;
/** 挂断电话 **/
virtual void hangup() = 0;
};
#endif /** ICONNECTION_MANAGER_H **/
/*************************************************************************************/
/** @file : IDataTransfer.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 15:15:09 **/
/*************************************************************************************/
#ifndef IDATATRANSFER_H
#define IDATATRANSFER_H
#include "comm.h"
/*************************************************************************************/
/** @class : 数据传输接口(interface) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class IDataTransfer
{
public:
/** 通话 **/
virtual void call(string content) = 0;
/** 回应 **/
virtual void answer(string content) = 0;
};
#endif /** IDATATRANSFER_H **/
/*************************************************************************************/
/** @file : Phone.h **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 15:32:31 **/
/** : 2015年12月1日 16:37:16 **/
/*************************************************************************************/
#ifndef PHONE_H
#define PHONE_H
#include "comm.h"
#include "IConnectionManager.h"
#include "IDataTransfer.h"
/*************************************************************************************/
/** @class : 手机类(class) **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
class Phone : public IConnectionManager, public IDataTransfer
{
private:
string myPhoneNumber;
string dialPhoneNumber;
public:
/** 手机类构造函数 **/
Phone(string myPhoneNumber);
/** 设置我的电话号码 **/
void setMyPhoneNumber(string myPhoneNumber);
/** 获取我的电话号码 **/
string getMyPhoneNumber();
/** 设置我拨打电话号码 **/
void setDialPhoneNumber(string dialPhoneNumber);
/** 获取我拨打电话号码 **/
string getDialPhoneNumber();
/** 拨打电话 **/
void dial(string dialPhoneNumber);
/** 挂断电话 **/
void hangup();
/** 通话 **/
void call(string content);
/** 回应 **/
void answer(string content);
};
#endif /** PHONE_H **/
/*************************************************************************************/
/** @file : Phone.cpp **/
/** @author : CC ^_^……!!! **/
/** @date : 2015年11月30日 15:35:50 **/
/** : 2015年12月1日 16:37:25 **/
/*************************************************************************************/
#include "Phone.h"
/*************************************************************************************/
/** @function : 手机类构造函数 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
Phone::Phone(string myPhoneNumber)
{
this->myPhoneNumber = myPhoneNumber;
}
/*************************************************************************************/
/** @function : 设置我的电话号码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void Phone::setMyPhoneNumber(string myPhoneNumber)
{
this->myPhoneNumber = myPhoneNumber;
}
/*************************************************************************************/
/** @function : 获取我的电话号码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string Phone::getMyPhoneNumber()
{
return this->myPhoneNumber;
}
/*************************************************************************************/
/** @function : 设置我拨打电话号码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void Phone::setDialPhoneNumber(string dialPhoneNumber)
{
this->dialPhoneNumber = dialPhoneNumber;
}
/*************************************************************************************/
/** @function : 获取我拨打电话号码 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
string Phone::getDialPhoneNumber()
{
return this->dialPhoneNumber;
}
/*************************************************************************************/
/** @function : 拨打电话 **/
/** @author : CC ^_^……!!! **/
/*************************************************************************************/
void Phone::dial(string dialPhoneNumber)
{
this->dialPhoneNumber = dialPhoneNumber;
cout<<"You dialed number is "<
dialPhoneNumber<
dialPhoneNumber = ""; cout<<"Phone is hang up!"<
myPhoneNumber<<" Say :"<
dialPhoneNumber<<" Answer :"<
| |
| |
| | |
|
| | |