摘要
查询数据库,完成对查询数据的excel导出。
一、controller
@RequestMapping(value = "/export")
@Authorization(HrmPrivilegeConstants.EBS_MANAGE)
public void export(@ModelAttribute(value = "ebsChargingDetailQuery") EbsChargingDetailQuery ebsChargingDetailQuery,
HttpServletRequest request, HttpServletResponse response) {
try {
Map<String, Object> context = new HashMap<>(6);
logger.info("ebs模板导出开始");
PaginatedList<EbsChargingDetailVo> exportList = ebsChargingDetailService.pageSearch(ebsChargingDetailQuery);
context.put("templateName", "ebsChargingDetailExport");
context.put("moneyFormatUtil", new MoneyFormatUtil());
context.put("dateUtil", new DateUtil());
context.put("enumUtils", new EnumUtils());
context.put("payCourseMap", payCourseMap);
context.put("exportList", exportList);
ExportExcelUtil.exportExcel(request, response, "WEB-INF/exportTemplates/ebsChargingDetailExport.xls", "ebsChargingDetail_"+System.currentTimeMillis()+".xls", context);
logger.info("ebs模板导出结束");
} catch (Exception e) {
logger.error("EbsChargingDetailController.export.Exception", e);
}
}
二、EbsChargingDetailVo
package com.jd.fms.proxyinvoice.project.domain;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Date;
import com.jd.fms.proxyinvoice.project.custenum.domainTypeEnum.PlatFlagFeeWriteOffEnum;
import com.jd.fms.proxyinvoice.project.custenum.domainTypeEnum.TransferNaturePersonEnum;
public class EbsChargingDetailVo implements Serializable {
private static final long serialVersionUID = 1L;
private Long id;
private Long chargingDetailId;
private String invoiceOrderNo;
private Integer ou;
private Date chargingDate;
private String supplierId;
private String supplierName;
private String orgnizationCode;
private String feeDeptCode;
private String feeDeptAllPathName;
private String feeItemCode;
private String invoiceNo;
private Integer direaction;
private Integer feetypeId;
private String feetypeName;
private BigDecimal amount;
private String platformCode;
private String platformName;
private Integer supplierType;
private String industry;
private String category;
private Date invoiceCompleteDate;
private Date createdDate;
private Date updateDate;
private String ebsFlag;
private String currency;
private TransferNaturePersonEnum transferNaturePerson;
private PlatFlagFeeWriteOffEnum feeWriteOff;
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Long getChargingDetailId() {
return chargingDetailId;
}
public void setChargingDetailId(Long chargingDetailId) {
this.chargingDetailId = chargingDetailId;
}
public String getInvoiceOrderNo() {
return invoiceOrderNo;
}
public void setInvoiceOrderNo(String invoiceOrderNo) {
this.invoiceOrderNo = invoiceOrderNo;
}
public Integer getOu() {
return ou;
}
public void setOu(Integer ou) {
this.ou = ou;
}
public Date getChargingDate() {
return chargingDate;
}
public void setChargingDate(Date chargingDate) {
this.chargingDate = chargingDate;
}
public String getSupplierId() {
return supplierId;
}
public void setSupplierId(String supplierId) {
this.supplierId = supplierId;
}
public String getSupplierName() {
return supplierName;
}
public void setSupplierName(String supplierName) {
this.supplierName = supplierName;
}
public String getOrgnizationCode() {
return orgnizationCode;
}
public void setOrgnizationCode(String orgnizationCode) {
this.orgnizationCode = orgnizationCode;
}
public String getFeeDeptCode() {
return feeDeptCode;
}
public void setFeeDeptCode(String feeDeptCode) {
this.feeDeptCode = feeDeptCode;
}
public String getFeeDeptAllPathName() {
return feeDeptAllPathName;
}
public void setFeeDeptAllPathName(String feeDeptAllPathName) {
this.feeDeptAllPathName = feeDeptAllPathName;
}
public String getFeeItemCode() {
return feeItemCode;
}
public void setFeeItemCode(String feeItemCode) {
this.feeItemCode = feeItemCode;
}
public String getInvoiceNo() {
return invoiceNo;
}
public void setInvoiceNo(String invoiceNo) {
this.invoiceNo = invoiceNo;
}
public Integer getDireaction() {
return direaction;
}
public void setDireaction(Integer direaction) {
this.direaction = direaction;
}
public Integer getFeetypeId() {
return feetypeId;
}
public void setFeetypeId(Integer feetypeId) {
this.feetypeId = feetypeId;
}
public String getFeetypeName() {
return feetypeName;
}
public void setFeetypeName(String feetypeName) {
this.feetypeName = feetypeName;
}
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public String getPlatformCode() {
return platformCode;
}
public void setPlatformCode(String platformCode) {
this.platformCode = platformCode;
}
public String getPlatformName() {
return platformName;
}
public void setPlatformName(String platformName) {
this.platformName = platformName;
}
public Integer getSupplierType() {
return supplierType;
}
public void setSupplierType(Integer supplierType) {
this.supplierType = supplierType;
}
public String getIndustry() {
return industry;
}
public void setIndustry(String industry) {
this.industry = industry;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public Date getInvoiceCompleteDate() {
return invoiceCompleteDate;
}
public void setInvoiceCompleteDate(Date invoiceCompleteDate) {
this.invoiceCompleteDate = invoiceCompleteDate;
}
public Date getCreatedDate() {
return createdDate;
}
public void setCreatedDate(Date createdDate) {
this.createdDate = createdDate;
}
public Date getUpdateDate() {
return updateDate;
}
public void setUpdateDate(Date updateDate) {
this.updateDate = updateDate;
}
public String getEbsFlag() {
return ebsFlag;
}
public void setEbsFlag(String ebsFlag) {
this.ebsFlag = ebsFlag;
}
public TransferNaturePersonEnum getTransferNaturePerson() {
return transferNaturePerson;
}
public void setTransferNaturePerson(TransferNaturePersonEnum transferNaturePerson) {
this.transferNaturePerson = transferNaturePerson;
}
public PlatFlagFeeWriteOffEnum getFeeWriteOff() {
return feeWriteOff;
}
public void setFeeWriteOff(PlatFlagFeeWriteOffEnum feeWriteOff) {
this.feeWriteOff = feeWriteOff;
}
}
三、ExportExcelUtil
package com.jd.fms.proxyinvoice.common.util;
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import net.sf.jxls.transformer.XLSTransformer;
public class ExportExcelUtil {
public static String exportExcel(HttpServletRequest request, HttpServletResponse response, String templateFilePath,
String outputName, Map beans) {
InputStream is = null;
OutputStream os = null;
try {
response.setContentType("application/vnd.ms-excel");
outputName = new String(outputName.getBytes("gbk"), "iso8859-1");
response.setHeader("Content-Disposition", "attachment; filename=" + outputName);
XLSTransformer transformer = new XLSTransformer();
is = new BufferedInputStream(new FileInputStream(request.getSession().getServletContext()
.getRealPath(templateFilePath)));
HSSFWorkbook workbook = (HSSFWorkbook) transformer.transformXLS(is, beans);
os = response.getOutputStream();
workbook.write(os);
} catch (Exception e) {
throw new RuntimeException("exportExcel error", e);
} finally {
if (is != null)
try {
is.close();
} catch (IOException e) {
throw new RuntimeException("exportExcel 关闭inputStream error", e);
}
if (os != null)
try {
os.close();
} catch (IOException e) {
throw new RuntimeException("exportExcel 关闭outputStream error", e);
}
}
return null;
}
}
四、EnumUtils
package com.jd.fms.proxyinvoice.project.util;
import com.jd.fms.proxyinvoice.project.custenum.domainTypeEnum.*;
public class EnumUtils {
public static String getPaperInvoiceApplyStatus(Integer status) {
PaperInvoiceApplyStatusEnum[] s = PaperInvoiceApplyStatusEnum.values();
for (PaperInvoiceApplyStatusEnum se : s) {
if (se.getValue().equals(status)) {
return se.getText();
}
}
return null;
}
public static String getCustomerType(Integer status) {
CustomerTypeEnum[] s = CustomerTypeEnum.values();
for (CustomerTypeEnum se : s) {
if (se.getValue().equals(status)) {
return se.getText();
}
}
return null;
}
public static String getPlatformConnectWay(Integer status) {
PlatformConnectWayEnum[] s = PlatformConnectWayEnum.values();
for (PlatformConnectWayEnum se : s) {
if (se.getValue().equals(status)) {
return se.getText();
}
}
return null;
}
public static String getPdfFlag(Integer status) {
PdfFlagEnum[] s = PdfFlagEnum.values();
for (PdfFlagEnum se : s) {
if (se.getCode().equals(status)) {
return se.getDescription();
}
}
return null;
}
public static String getFirstInvoice(Integer value){
FirstInvoiceEnum[] s = FirstInvoiceEnum.values();
for (FirstInvoiceEnum se : s) {
if (se.value().equals(value)) {
return se.getMsg();
}
}
return null;
}
public static String getPaymentDirection(Integer status) {
PaymentDirectionEnum[] s = PaymentDirectionEnum.values();
for (PaymentDirectionEnum se : s) {
if (se.getValue().equals(status)) {
return se.getText();
}
}
return null;
}
public static String getOu(Integer status) {
OuEnum[] s = OuEnum.values();
for (OuEnum se : s) {
if (se.value().equals(status)) {
return se.getText();
}
}
return null;
}
}
五、DateUtil
package com.jd.fms.proxyinvoice.common.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
import java.util.TimeZone;
public class DateUtil {
public static final String FORMATTEXT01 = "yyyy-MM-dd";
public static final String FORMATTEXT02 = "yyyy-MM-dd HH:mm:ss";
public static final String FORMATTEXT03 = "yyyyMMddHHmmss";
public static final String FORMATTEXT04 = "yyyyMMdd";
public static final String FORMATTEXT05 = "yyyyMMdd_HHmmss";
public static final String FORMATTEXT06 = "yyyy-MM";
public static final String FORMATTEXT07 = "yyyy_MM_dd";
public static final String FORMATTEXT08 = "yyyy";
public static final String FORMATTEXT09 = "MM";
public static final String FORMATTEXT10 = "dd";
public static final String FORMATTEXT11 = "yyyyMMddHHmmssSSS";
public static final String FORMATTEXT12 = "yyyy-MM-dd HH:mm:ss.SSS";
public static final String FORMATTEXT13 = "yyyy/MM/dd";
public static final String FORMATTEXT14 = "yyyyMM";
public static final String FORMATTEXT15 = "yyyyMMdd";
public static final String FORMATTEXT16 = "MM/dd/yyyy";
public static final String FORMATTEXT17 = "yyyy-MM-dd HH:mm";
public static final String FORMATTEXT18 = "yyyy-M";
public static final int getWeek(Date date) {
Calendar c = Calendar.getInstance();
c.setTime(date);
int week = c.get(Calendar.DAY_OF_WEEK) - 1;
if (week == 0) {
return week = 7;
} else {
return week;
}
}
public static final String formatDate(long time, String formatText) {
SimpleDateFormat format = new SimpleDateFormat(formatText);
String result = format.format(new Date(time));
return result;
}
public static final String formatDate(Date date, String formatText) {
if (CheckUtil.isNull(date) || CheckUtil.isNull(formatText)) {
return "";
}
SimpleDateFormat formats = new SimpleDateFormat(formatText);
return formats.format(date);
}
public static final String formatDate(String date, String formatText) {
SimpleDateFormat formats = new SimpleDateFormat(formatText);
return formats.format(parseDate(date, formatText));
}
public static final int getCurrentYear() {
Calendar calendar = Calendar.getInstance();
return calendar.get(calendar.YEAR);
}
public static final int getCurrentMonth() {
Calendar calendar = Calendar.getInstance();
return calendar.get(calendar.MONTH) +1;
}
public static final int getCurrentDay() {
Calendar calendar = Calendar.getInstance();
return calendar.get(calendar.DATE);
}
public static final String getCurrentTime(String formatText) {
SimpleDateFormat format = new SimpleDateFormat(formatText);
String result = format.format(new Date());
return result;
}
public static final Date parseDate(String strDate, String formatText) {
SimpleDateFormat df = null;
Date date = null;
df = new SimpleDateFormat(formatText);
try {
date = df.parse(strDate);
} catch (ParseException e) {
e.printStackTrace();
}
return (date);
}
public static final Date addMonth(Date date, int value){
if (CheckUtil.isNull(date)){
date = new Date();
}
Calendar c = Calendar.getInstance();
c.setTime(date);
c.add(Calendar.MONTH, value);
return c.getTime();
}
public static final int getDateYear(Date date){
Calendar c = Calendar.getInstance();
c.setTime(date);
return c.get(Calendar.YEAR);
}
public static final int getDateMonth(Date date){
Calendar c = Calendar.getInstance();
c.setTime(date);
return c.get(Calendar.MONTH)+1;
}
public static final Date addDay(Date date, int value){
if (CheckUtil.isNull(date)){
return null;
}
Calendar c = Calendar.getInstance();
c.setTime(date);
c.add(Calendar.DAY_OF_MONTH, value);
return c.getTime();
}
public static final Date getFirstDayOfMonth(Date date){
if (CheckUtil.isNull(date)){
return null;
}
Calendar c = Calendar.getInstance();
c.setTime(date);
c.set(Calendar.DATE,1);
c.set(Calendar.HOUR_OF_DAY, 0);
c.set(Calendar.MINUTE, 0);
c.set(Calendar.SECOND, 0);
return c.getTime();
}
public static final List<Integer> getIntervalYear(int startYear, int endYear){
if (CheckUtil.isNull(startYear) || CheckUtil.isNull(endYear)){
return null;
}
if(startYear > endYear){
return null;
}
List<Integer> intervalYear = new ArrayList<Integer>();
int interval = endYear - startYear;
for(int i = 0; i <= interval; i++){
intervalYear.add(startYear+i);
}
return intervalYear;
}
public static String getESTTime(String formatText){
TimeZone tz = TimeZone.getTimeZone("EST");
Calendar calendar = Calendar.getInstance();
SimpleDateFormat sdf = new SimpleDateFormat(formatText);
sdf.setTimeZone(tz);
return sdf.format(calendar.getTime());
}
public static final Date getMonthEnd(String date, String formatText) {
SimpleDateFormat dateFormat = new SimpleDateFormat(formatText);
Calendar calendar = Calendar.getInstance();
try{
calendar.setTime(dateFormat.parse(date));
} catch (Exception e){
e.printStackTrace();
}
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMaximum(Calendar.DAY_OF_MONTH));
return calendar.getTime();
}
public static final Date getMonthStart(String date, String formatText) {
SimpleDateFormat dateFormat = new SimpleDateFormat(formatText);
Calendar calendar = Calendar.getInstance();
try{
calendar.setTime(dateFormat.parse(date));
} catch (Exception e){
e.printStackTrace();
}
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMinimum(Calendar.DAY_OF_MONTH));
return calendar.getTime();
}
public static void main(String[] args) throws ParseException {
Calendar calendar = Calendar.getInstance();
System.out.println(formatDate(getMonthEnd("2016-10", FORMATTEXT06), FORMATTEXT01));
System.out.println(getDateYear(new Date()));
System.out.println(getDateMonth(new Date()));
System.out.println(formatDate(addMonth(new Date(), -9), FORMATTEXT06));
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMinimum(Calendar.DAY_OF_MONTH));
Date date = calendar.getTime();
System.out.println();
System.out.println(DateUtil.formatDate(DateUtil.addMonth(new Date(), +2), "yyyy-M"));
}
}
六、MoneyFormatUtil
package com.jd.fms.proxyinvoice.common.util;
import java.math.BigDecimal;
public class MoneyFormatUtil {
private final static String [] PATTERN ={"零","壹","贰","叁","肆","伍","陆","柒","捌","玖"};
private final static String [] C_PATTERN ={"","拾","佰","仟","万","拾","佰","仟","亿"};
private final static String [] CF_PATTERN = {"","角","分"};
private final static String ZEOR = "零";
public static String toCn(String moneyString) {
int dotPoint = moneyString.indexOf(".");
String moneyStr;
if (dotPoint != -1) {
moneyStr = moneyString.substring(0, moneyString.indexOf("."));
} else {
moneyStr = moneyString;
}
StringBuffer fraction = null;
StringBuffer ms = new StringBuffer();
for (int i = 0; i < moneyStr.length(); i++) {
ms.append(PATTERN[moneyStr.charAt(i) - 48]);
}
int cpCursor = 1;
for (int j = moneyStr.length() - 1; j > 0; j--) {
ms.insert(j, C_PATTERN[cpCursor]);
cpCursor = cpCursor == 8 ? 1 : cpCursor + 1;
}
while (ms.indexOf("零拾") != -1) {
ms.replace(ms.indexOf("零拾"), ms.indexOf("零拾") + 2, ZEOR);
}
while (ms.indexOf("零佰") != -1) {
ms.replace(ms.indexOf("零佰"), ms.indexOf("零佰") + 2, ZEOR);
}
while (ms.indexOf("零仟") != -1) {
ms.replace(ms.indexOf("零仟"), ms.indexOf("零仟") + 2, ZEOR);
}
while (ms.indexOf("零万") != -1) {
ms.replace(ms.indexOf("零万"), ms.indexOf("零万") + 2, "万");
}
while (ms.indexOf("零亿") != -1) {
ms.replace(ms.indexOf("零亿"), ms.indexOf("零亿") + 2, "亿");
}
while (ms.indexOf("零零") != -1) {
ms.replace(ms.indexOf("零零"), ms.indexOf("零零") + 2, ZEOR);
}
while (ms.indexOf("亿万") != -1) {
ms.replace(ms.indexOf("亿万"), ms.indexOf("亿万") + 2, "亿");
}
while (ms.lastIndexOf("零") == ms.length() - 1) {
ms.delete(ms.lastIndexOf("零"), ms.lastIndexOf("零") + 1);
}
int end;
if ((dotPoint = moneyString.indexOf(".")) != -1) {
String fs = moneyString.substring(dotPoint + 1,
moneyString.length());
if (fs.indexOf("00") == -1 || fs.indexOf("00") >= 2) {
end = fs.length() > 2 ? 2 : fs.length();
fraction = new StringBuffer(fs.substring(0, end));
for (int j = 0; j < fraction.length(); j++) {
fraction.replace(j, j + 1,
PATTERN[fraction.charAt(j) - 48]);
}
for (int i = fraction.length(); i > 0; i--) {
fraction.insert(i, CF_PATTERN[i]);
}
fraction.insert(0, "元");
} else {
fraction = new StringBuffer("元整");
}
} else {
fraction = new StringBuffer("元整");
}
ms.append(fraction);
return ms.toString();
}
public static String fromatDecimal(String value) {
if (CheckUtil.isNull(value)) {
return "";
} else if (value.equals("-")) {
return value;
}
return Arith.thousandCharacterformat(
new BigDecimal(value).doubleValue(), 2);
}
public static String formatDecimal(double value) {
return Arith.thousandCharacterformat(value, 2);
}
public static String fromatDecimal(BigDecimal value) {
if (null == value) {
return "";
}
return Arith.thousandCharacterformat(value.doubleValue(), 2);
}
public static String fromatDecimalNoScale(BigDecimal value) {
if (null == value) {
return "";
}
return String.valueOf(value.longValue());
}
public static String money2String(String value) {
if (null == value || "".equals(value.trim())) {
return null;
} else {
return value.replace(" ", "").replace(",", "");
}
}
public static String formartPercent(BigDecimal v)
{
return Arith.thousandCharacterformat(v.multiply(new BigDecimal(100)), 1) + "%";
}
public static void main(String[] args) {
System.out.println(fromatDecimal(new BigDecimal("10000000.4567")));
}
}
七、excel代码公式
订单号 财务机构 商家名称 费用承担部门编码 费用承担部门名称 费用科目编码 费用科目名称 发票号码 费用名称 服务费是否转嫁自然人 服务费是否内部核销 税费是否内部核销 收付款方向 金额 平台简码 平台名称 客户类型 开票日期
<jx:forEach items="${exportList}" var="line">
${line.invoiceOrderNo} ${enumUtils.getOu(line.ou)} ${line.supplierName} ${line.feeDeptCode} ${line.feeDeptAllPathName} ${line.feeItemCode} ${payCourseMap.get(line.feeItemCode)} ${line.invoiceNo} ${line.feetypeName} ${line.transferNaturePerson} ${line.fwfWriteOff} ${line.taxWriteOff} ${enumUtils.getPaymentDirection(line.direaction)} ${line.amount} ${line.platformCode} ${line.platformName} ${enumUtils.getCustomerType(line.supplierType)} ${dateUtil.formatDate(line.invoiceCompleteDate, dateUtil.FORMATTEXT01)}
</jx:forEach>
八、excel截图
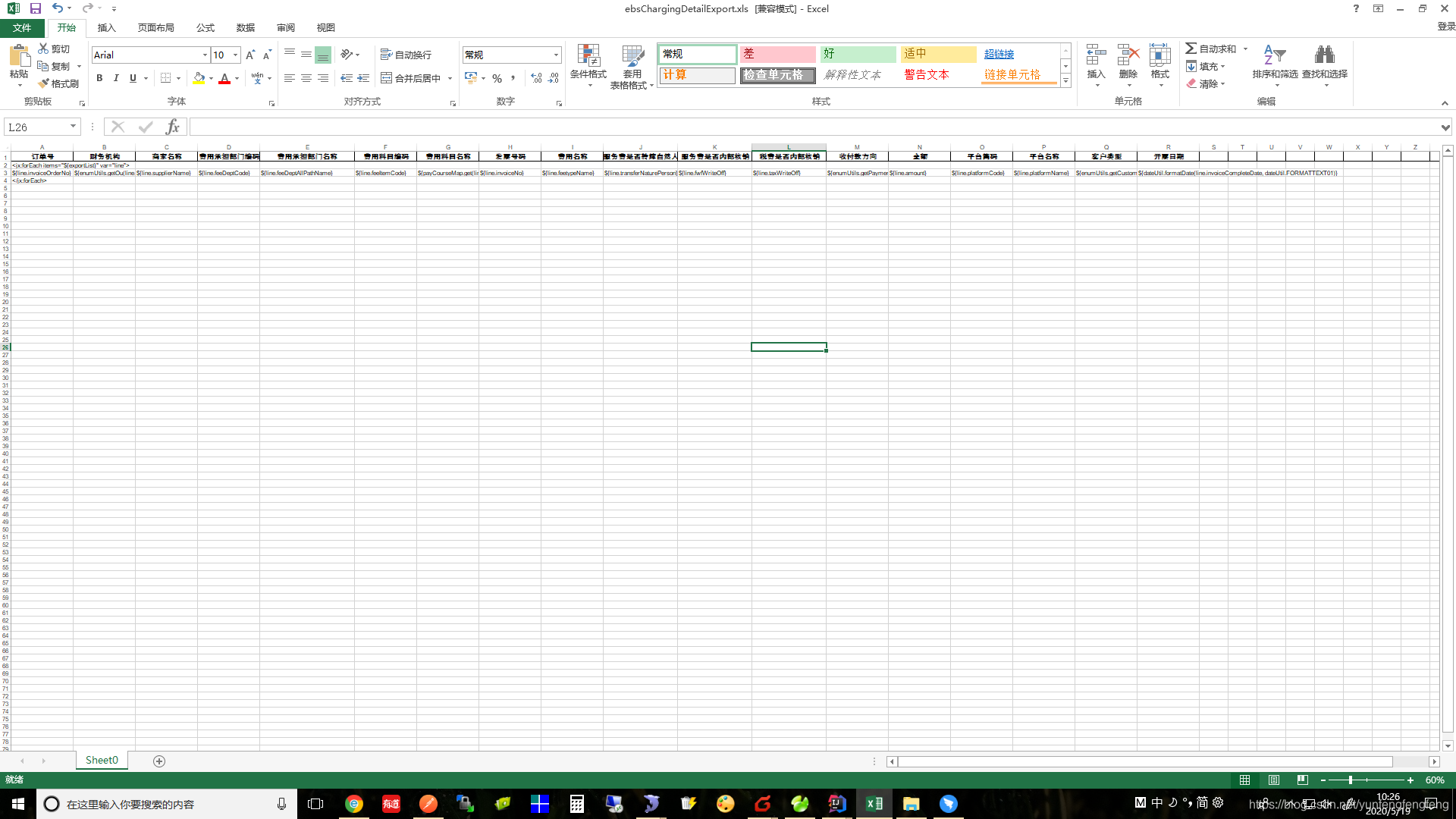