目录
3.面向对象思想的体现一:类和对象的创建和执行操作有哪三步?
5. 类的方法内是否可以定义变量?是否可以调用属性?是否可以定义方法?是否可以调用方法?
1.判 断: 与void show(int a,char b,double c){}构成重载的有:
8.成员变量和局部变量在声明的位置上、是否有默认初始化值上、是否能有权限修饰符修饰上、内存分配的位置上有何不同?
12.关于类的属性的赋值,有几种赋值的方式。谈谈赋值的先后顺序
13.this关键字可以用来调用哪些结构,简单说明一下其使用。
14.Java中目前学习涉及到的四种权限修饰符都有什么?并说明各自的权限范围
15.创建Circle类,提供私有的radius属性,提供相应的get和set方法,提供求圆面积的方法。
2.利用面向对象的编程方法,设计类Circle计算圆的面积。
9.定义一个int型的数组:int[] arr = new int[]{12,3,3,34,56,77,432};
让数组的每个位置上的值去除以首位置的元素,得到的结果,作为该位置上的 新值。遍历新的数组。
例3.已知有一个数列:f(0) = 1,f(1) = 4,f(n+2)=2*f(n+1) + f(n),其中n是大于0的整数,求f(10)的值。
例4:斐波那契数列:500阶台阶问走完这些台阶一共有几种方法
一、理论
1.面向对象思想编程内容的三条主线分别是什么
① 类及类的成员:属性、方法、构造器;代码块、内部类
② 面向对象的三大特征:封装、继承、多态
③ 其它关键字:this,super,abstract,interface,static,final,package,import
面向对象的编程思想?
(类、对象;面向对象的三大特征;。。。)
2.谈谈你对面向对象中类和对象的理解,并指出二者的关系?
类:抽象的、概念上的内容
对象:实实在在存在的一个个体。
对象是由类派生出来的。
3.面向对象思想的体现一:类和对象的创建和执行操作有哪三步?
① 创建类
② 类的实例化
③ 调用对象的结构:”对象.属性” “对象.方法”
4. 画出如下代码在执行时的内存分配情况
class Car{
String color = "red";
int num = 4;
void show(){
int a = 10;
System.out.println("color="+color+",num="+num);
}
}
class CarTest {
public static void main(String[] args) {
Car c1 = new Car();
Car c2 = new Car();
c1.color = "blue";
c1.show(); //color:blue num:4
c2.show(); //color:red num:4
} }
5. 类的方法内是否可以定义变量?是否可以调用属性?是否可以定义方法?是否可以调用方法?
是;是;否;是
6.方法的重载
1.判 断: 与void show(int a,char b,double c){}构成重载的有:
2.什么是方法的重载?
“两同一不同”:同一个类、相同方法名;参数列表不同。
如何调用确定的方法:方法名---->参数列表
7.方法的参数传递:值传递
1.画出以下程序的内存分布情况:
提供如下代码的内存解析
1. 内存结构:栈(局部变量)、堆(new出来的结构:对象(非static成员变量)、数组)
2. 变量:成员变量 vs 局部变量(方法内、方法形参、构造器内、构造器形参、代码块内)
3.内存分布图如下:
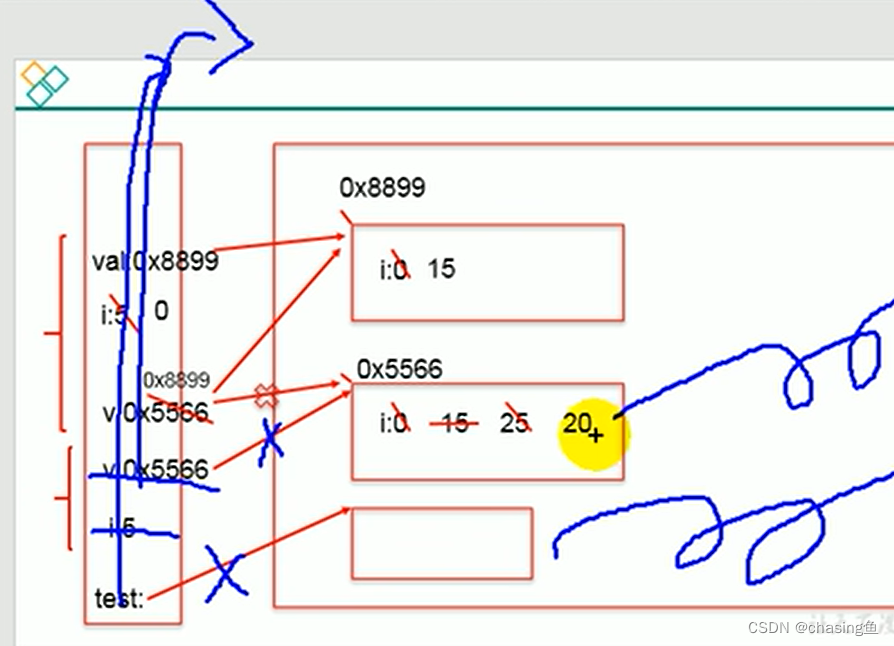
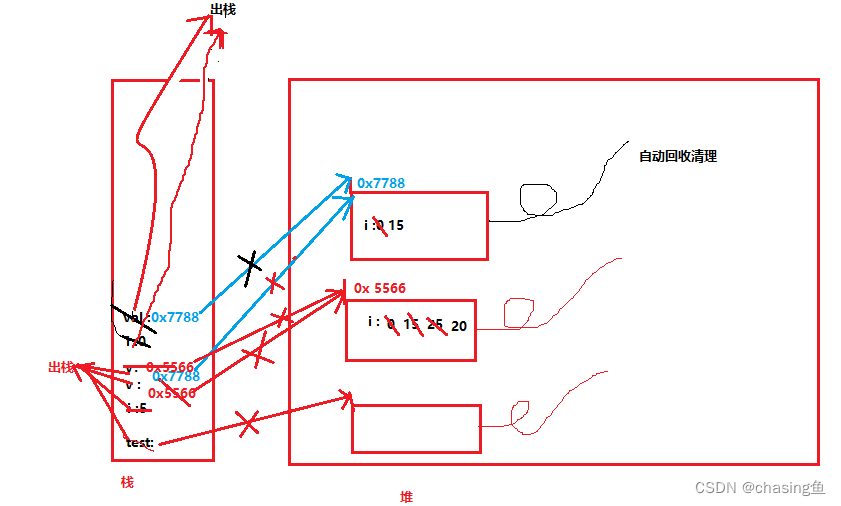
2.说明Java方法中的参数传递机制的具体体现?
基本数据类型:数据值
引用数据类型:地址值 (含变量的数据类型)
Person p1 = new Person();
Person(){eat();age}
/*
一维数组: int[] x 或者 int x[]
二维数组: int[][] y 或者 int[] y[] 或者 int y[][]
int[] x,y[];
x = y;//编译错误
二者的类型不同,一个是Person类型,一个是User类型都是引用数据类型不能使用强制类型转换
*/
User u1 = p1;//编译错误 (逆向思维、反证法) :u1.eat() u1.age
8.成员变量和局部变量在声明的位置上、是否有默认初始化值上、是否能有权限修饰符修饰上、内存分配的位置上有何不同?
1.在类中声明的位置不同
属性(成员变量):直接定义在{}内
局部变量:声名在方法内、方法形参、代码块内、构造器形参、构造器内部的变量
2. 关于权限修饰符的不同
属性:可以在声名属性时,指明其权限,使用权限修饰符
常用的权限修饰符:private、public、缺省、protected ---->封装性
目前,大家声名属性时,都使用缺省(啥也不写)
局部变量:不可以使用曲线修饰符。
3.默认初始化值的情况:
属性:类的属性,根据其类型,都有默认值初始化值。
整型(byte、short、int、long):0
浮点型(float、double) :0.0
字符型(char) :0
布尔型(boolean):false
引用数据类型(类、接口、数组):null
局部变量:没有默认初始化值。
意味着,我们在调用局部变量之前,一定要显示赋值
特别的:形参在调用时,我们赋值即可。
4. 在内存中加载的位置
属性:加载在堆空间中(非static)
局部变量:加载到栈空间
9.谈谈return关键字的使用
① 结束方法 ② 针对于有返回值的方法,return + 返回数据
10.递归
11.构造器的作用是什么?使用中有哪些注意点(>=3条)
作用:①创建对象 ②初始化对象结构
注意点:构造器没有返回值
1.如果没有显示的定义类的构造器的话,则系统默认提供一个空参的构造器。
2.定义构造器的格式:权限修饰符 类名 ( 形参列表 ) { }
3.一个类中定义的多个构造器,彼此构成重载。
4.一旦我们显式的定义了类的构造器之后,系统就不再提供默认的空参构造器。
5.一个类中,至少会有一个构造器。
12.关于类的属性的赋值,有几种赋值的方式。谈谈赋值的先后顺序
默认初始化-显式初始化-构造器中初始化-对象.方法 或 对象.属性 给属性赋值
①默认初始化值
②显式初始化
③构造器中初始化(赋值)
④通过“对象.方法”或"对象.属性",赋值
以上操作的先后顺序:① - ② - ③ - ④ ① - ③ - ② - ④
最终值为:最后一个操作的赋值
13.this关键字可以用来调用哪些结构,简单说明一下其使用。
this可以调用:属性、方法、构造器
this:理解为当前对象,当前正在创建的对象
14.Java中目前学习涉及到的四种权限修饰符都有什么?并说明各自的权限范围
private、缺省(英文标识:default)、protected、public
private int age;
private void eat(){};
15.创建Circle类,提供私有的radius属性,提供相应的get和set方法,提供求圆面积的方法。
public Circle{
public static void main(String[] args){
Circle circle = new Circle();
circle.setRadius(3);
System.out.println(circle.findArea());
}
private double radius;
public void setRadius(double radius){
this.radius = radius;
}
public double getRadius(){
return radius;
}
public double findArea(){
return 3.14 * getRadius() * radius;
}
}
16.拓展,方法的调用
int[] arr = new int[] {1,2,3,4,5};
System.out.println(arr);
char[] arr1 = new char[] {'a','b','c'};
System.out.println(arr1);
最后的输出结果是多少?原因?
package com.atguigu.exer;
public class ArrayPrintTest {
public static void main(String[] args) {
int[] arr = new int[] {1,2,3};
/**
* cril+左键点击println
*
*/
System.out.println(arr);//地址值:调用的是println(Object x) 方法
char[] arr1 = new char[] {'a','b','c'};
System.out.println(arr1);//abc:调用的是println(char x[])方法
}
}
原因:
System.out.println(arr): System.out.println(arr1);
二、编程
1.创建一个Person类
package com.atguigu.exer;
public class Person {
String name;
int age;
/**
* sex:1 表示男性
* sec:0 表示女性
*/
int sex;
public void study() {
System.out.println("studying");
}
public void showAge() {
System.out.println("age:"+age);
}
public int addAge(int i) {
age += i;
return age;
}
}
package com.atguigu.exer;
/*
* 创建一个Person类,其定义如下:
* (1)创建Person类的对象,设置该对象的name、age和sex属性,
* 调用study方法,输出字符串“studying”,
* 调用showAge()方法显示age值,
* 调用addAge()方法给对象的age属性值增加2岁。
*
*
* (2)创建第二个对象,执行上述操作,体会同一个类的不同对象之间的关系。
*
*
*/
public class PersonTest {
public static void main(String[] args) {
Person p1 = new Person();//创建类的对象
p1.name = "Tom";
p1.age = 18;
p1.sex = 1;
p1.study();
p1.showAge();
int newAge = p1.addAge(2);
System.out.println(p1.name+"的新年龄为:"+newAge);
System.out.println(p1.age);//20
//***************************
//每一个不同的对象,所包含的值也不一样,不为一个存储空间
Person p2 = new Person();
p2.showAge();//0
p2.addAge(10);
p2.showAge();//10
p1.showAge();//20
}
}
2.利用面向对象的编程方法,设计类Circle计算圆的面积。
package com.atguigu.exer;
/*
* 2.利用面向对象的编程方法,设计类Circle计算圆的面积。
* 注:方式一方式二,在Circle类中,都有属性。方式三中没有属性,将半径设置成了,形参,不为错误但是半径是圆的属性,最好是用方式一和方式二
*
* 该题目中,将半径定义为属性,就不能再在findArea()方法的形参中出现,定义为形参就不能再当作属性进行定义,同一个参数只能定义一次
*/
//老师讲解
public class CircleTest {
public static void main(String[] args) {
Circle c1 = new Circle();
//方式一,方式二
//c1.radius = 2.1;
//方式一
//double area = c1.findArea();
//System.out.println("圆的面积为:"+area);
//方式二:
//c1.findArea();
//方式三:
double value = c1.findArea(2.1);
System.out.println("圆的面积为:"+value);//圆的面积为:13.854423602330987
}
}
class Circle {
//属性
//方式一,方式二
// double radius;
//求圆的面积
//方式一:无参数有返回值
//public double findArea() {
// double area = Math.PI*radius*radius;
// return area;
//}
//方式二:无参数,无返回值
// public void findArea() {
// double area = Math.PI*radius*radius;
// System.out.println("圆的面积为:"+area);
// }
//方式三:有参数,有返回值
public double findArea(double r) {
double area = Math.PI*r*r;
return area;
}
}
3.声明一个method方法,打印" * "型矩形
package com.atguigu.exer;
/*
*
* 3.1编写程序,声明一个method方法,在方法中打印一个10*8 的*型矩形,在main方法中调用该方法。
*
* 3.2修改上一个程序,在method方法中,除打印一个10*8的*型矩形外,
* 再计算该矩形的面积,并将其作为方法返回值。在main方法中调用该方法,
* 接收返回的面积值并打印。
*
* 3.3修改上一个程序,在method方法提供m和n两个参数,
* 方法中打印一个m*n的*型矩形,并计算该矩形的面积, 将其作为方法返回值。
* 在main方法中调用该方法,接收返回的面积值并打印。
*
*
*/
public class Exer3Test {
public static void main(String[] args) {
Exer3Test e1 = new Exer3Test();
//3.1测试
// e1.method();
//3.2测试
//第一种方式:
//int area = e1.method();
//System.out.println("面积为:"+area);
//第二种方式:
//System.out.println("面积为:"+e1.method());
//3.3测试
//int area = e1.method(12, 10);
//System.out.println("面积为:"+area);
System.out.println("面积为:"+e1.method(12, 10));
}
/*3.1
* public int method() {
for(int i=1;i<=10;i++) {
for(int j=1;j<=8;j++) {
System.out.print("*"+" ");
}
System.out.println();
}
}
*
*/
/*3.2
* public int method() {
for(int i=1;i<=10;i++) {
for(int j=1;j<=8;j++) {
System.out.print("*"+" ");
}
System.out.println();
}
return 10*8;
}
*
*
*/
//3.3
public int method(int m,int n) {
for(int i=1;i<=m;i++) {
for(int j=1;j<=n;j++) {
System.out.print("*"+" ");
}
System.out.println();
}
return m*n;
}
}
4. 对象数组题目:
定义类Student,包含三个属性:学号number(int),年级state(int),成绩score(int)。
创建20个学生对象,学号为1到20,年级和成绩都由随机数确定。
问题一:打印出3年级(state值为3)的学生信息。
问题二:使用冒泡排序按学生成绩排序,并遍历所有学生信息
提示:
1) 生成随机数:Math.random(),返回值类型double;
2) 四舍五入取整:Math.round(double d),返回值类型long。
package com.atguigu.exer;
import java.util.Arrays;
/*
* 4. 对象数组题目:
* 定义类Student,包含三个属性:学号number(int),年级state(int),成绩score(int)。
* 创建20个学生对象,学号为1到20,年级和成绩都由随机数确定。
* 问题一:打印出3年级(state值为3)的学生信息。
* 问题二:使用冒泡排序按学生成绩排序,并遍历所有学生信息
* 提示:
* 1) 生成随机数:Math.random(),返回值类型double;
* 2) 四舍五入取整:Math.round(double d),返回值类型long。
* 5.声明一个日期类型MyDate:有属性:年year,月month,日day。创建2个日期
* 对象,分别赋值为:你的出生日期,你对象的出生日期,并显示信息。
*
*
*/
public class StudentTest {
public static void main(String[] args) {
// Student s1 = new Student();
// Student s2 = new Student();
// Student s3 = new Student();
// Student s4 = new Student();
// Student s5 = new Student();
// ......
// Student s20 = new Student();
//声名Student类型的数组
Student[] stus = new Student[20];//类似于String[] str = new String[20];
for(int i = 0;i<stus.length;i++) {
//给数组元素赋值
stus[i] = new Student();
//给Student对象的属性赋值
stus[i].number = (i+1);
//年级:【1,6】
stus[i].state = (int)(Math.random()*(6-1+1)+1);
//成绩:【0,100】
stus[i].score = (int)(Math.random()*(100-0+1));
}
//遍历学生数组
for(int i = 0;i < stus.length;i++) {
System.out.println("学号"+" "+"年级"+" "+"成绩"+"\n"+
stus[i].number+" "+stus[i].state+" "+stus[i].score+"\n");
}
System.out.println("------------------------------");
//问题一:打印出3年级(state值为3)的学生信息。
for(int i = 0;i<stus.length;i++) {
if(stus[i].state == 3) {
System.out.println("学号"+" "+"年级"+" "+"成绩"+"\n"+
stus[i].number+" "+stus[i].state+" "+stus[i].score+"\n");
}
}
System.out.println("**********************************");
//问题二:使用冒泡排序按学生成绩排序,并遍历所有学生信息
for(int i = 0;i<stus.length-1;i++) {
for(int j = 0;j<stus.length-1-i;j++) {
if(stus[j].score > stus[j+1].score ) {
Student temp = stus[j];
stus[j] = stus[j+1];
stus[j+1] = temp;
}
}
}
for(int i = 0;i < stus.length;i++) {
// System.out.println("学号"+" "+"年级"+" "+"成绩"+"\n"+
// stus[i].number+" "+stus[i].state+" "+stus[i].score+"\n");
System.out.println(stus[i].info());
}
}
}
class Student{
int number;//学号
int state;//年级
int score;//成绩
public String info() {
return "学号"+" "+"年级"+" "+"成绩"+"\n"+
number+" "+state+" "+score+"\n";
}
}
5.声明一个日期类型MyDate:
有属性:年year,月month,日day。
创建2个日期 对象,分别赋值为:你的出生日期,你对象的出生日期,并显示信息。
package com.atguigu.exer;
/*
*
* 5.声明一个日期类型MyDate:有属性:年year,月month,日day。
* 创建2个日期对象,分别赋值为:你的出生日期,你对象的出生日期,并显示信息。
*
*
*/
public class MyDateTest {
public static void main(String[] args) {
//对数组进行赋值;实例化类
MyDate date = new MyDate();//自己
MyDate objectDate = new MyDate();//自己的对象
date.year = 1998;
date.month = 12;
date.day =20;
System.out.println(date.info());
objectDate.year=1998;
objectDate.month = 10;
objectDate.day = 28;
System.out.println(objectDate.info());
}
}
class MyDate{
int year;
int month;
int day;
public String info() {
return year+"年"+month+"月"+day+"日";
}
}
6.自定义一个Arrays工具类
类名为ArrayUtil,实现数组,最大值、最小值、总和、平均值、复制、反转、遍历、查找指定元素、排序。新建类ArrayUtilTest,进行测试
package com.atguigu.java;
/*
*
* 自定义数组的工具类
*
*
*/
public class ArrayUtil {
//求数组的最大值
public int getMax(int[] arr) {
int max = arr[0];
for(int i=1;i<arr.length;i++) {
if(arr[i]>max) {
max = arr[i];
}
}
/*以下程序也可以找出最大值,但是它侧重于数值交换,把最大值与0交换,数组改变了,故不可取!
* int max = 0;
* for(int i = 0;i<arr.length;i++){
* if(arr[i]>max){
* int temp = arr[i];
* arr[i] = max;//arr[i]的值成0了,数组发生了改变
* max = temp;
* }
*
* }
*
*
*/
return max;
}
//求数组的最小值
public int getMin(int[] arr) {
int min = arr[0];
for(int i=1;i<arr.length;i++) {
if(min>arr[i]) {
min = arr[i];
}
}
return min;
}
//求数组的最总和值
public int getSum(int[] arr) {
int sum = 0;
for(int i=0;i<arr.length;i++) {
sum += arr[i];
}
return sum;
}
//求数组的最平均数
public double getAvg(int[] arr) {
int sum = getSum(arr);
double avg = (double)sum/arr.length;
return avg;
}
//反转数组
//如下两个方法为方法的重载
public void reverse(int[] arr) {
for(int i=0;i<arr.length/2;i++) {
int temp = arr[i];
arr[i] = arr[arr.length-1-i];
arr[arr.length-1-i] = temp;
}
//方式二:
//for(int i = 0,j = arr.length-1;i<j;i++,j--) {
// String temp = arr[i];
// arr[i] = arr[j];
// arr[j] = temp;
//}
}
public void reverse(String[] arr) {
}
//复制数组
public int[] copy(int[] arr) {
int[] arr1 = new int[arr.length];
for(int i=0;i<arr.length;i++) {
arr1[i] = arr[i];
}
return arr1;
}
//数组排序
public void sort(int[] arr) {
//冒泡排序
for(int i = 0;i<arr.length-1;i++) {//控制一共需要多少次的对比排序才能把数组元素排成有序队列
for(int j = 0;j<arr.length-1-i;j++) {//每一大轮,相邻两个元素需要进行几次比较选出最大值
if(arr[j] > arr[j+1]) {//获取两个数的最大值
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
print(arr);
}
//遍历数组
public void print(int[] arr) {
for(int i=0;i<arr.length;i++) {
System.out.print(arr[i]+" ");
}
System.out.println();
}
//查找指定元素
public int getIndex(int[] arr,int dest) {
/**
* 返回值为负数时没有找到指定元素
*
*/
/*
for(int i=0;i<arr.length;i++) {
if(arr[i] == dest) {
return i;
}
}
return -1;
*/
sort(arr);
int head = 0;//初始的首索引
int end = arr.length-1;//初始的末索引
while(head <= end) {//②循环条件
//③循环体
int middle = (head + end)/2;
if(dest == arr[middle]) {
return middle;
}else if(arr[middle] > dest) {
end = middle -1;//④迭代条件
}else {//arr2[middle] < dest1
head = middle +1;//④迭代条件
}
}
return -1;
}
}
package com.atguigu.java;
public class ArrayUtilTest {
public static void main(String[] args) {
ArrayUtil util = new ArrayUtil();
int[] arr = new int[] {32,34,5,3,54,654,-98,0,-53,5};//636
int max = util.getMax(arr);//最大值
System.out.println("最大值max = "+max);
int min = util.getMin(arr);//最小值
System.out.println("最小值min = "+min);
int sum = util.getSum(arr);//总和
System.out.println("数组总和sum = "+sum);
double avg = util.getAvg(arr);//平均数
System.out.println("数组平均值avg = "+avg);
System.out.println(new ArrayUtil().getAvg(arr));
System.out.print("数组arr:");
util.print(arr);
util.reverse(arr);//反转数组
System.out.print("反转后数组arr:");
util.print(arr);
int[] arr1=util.copy(arr);//复制数组
System.out.print("复制的数组arr1:");
util.print(arr1);
arr1[0] = 777;
System.out.print("修改后的arr1数组:");
util.print(arr1);
System.out.print("数组arr:");
util.print(arr);
int index = util.getIndex(arr, 0);//指定元素的位置
if(index<0) {
System.out.println("没有找到!");
}else {
System.out.println("指定元素的位置index是:"+index);
}
System.out.print("排序后的arr数组:");
util.sort(arr);//数组的排序
}
}
7.方法重载
1.编写程序,定义三个重载方法并调用。方法名为mOL。
>三个方法分别接收一个int参数、两个int参数、一个字符串参数。
分别执行平方运算并输出结果,相乘并输出结果,输出字符串信息。
>在主类的main ()方法中分别用参数区别调用三个方法。
2.定义三个重载方法max()
第一个方法求两个int值中的最大值
第二个方法求两个double值中的最大值,
第三个方法求三个double值中的最大值
并分别调用三个方法。
package com.atguigu.exer;
/*
* 1.编写程序,定义三个重载方法并调用。方法名为mOL。
* >三个方法分别接收一个int参数、两个int参数、一个字符串参数。
* 分别执行平方运算并输出结果,相乘并输出结果,输出字符串信息。
* >在主类的main ()方法中分别用参数区别调用三个方法。
*
* 2.定义三个重载方法max()
* 第一个方法求两个int值中的最大值
* 第二个方法求两个double值中的最大值,
* 第三个方法求三个double值中的最大值
* 并分别调用三个方法。
*
*/
public class OverLoadExer {
//1.如下三个方法构成重载
public void mOL(int i) {
System.out.println(i*i);
}
public void mOL(int i,int j) {
System.out.println(i*j);
}
public void mOL(String s) {
System.out.println(s);
}
//2.如下三个方法构成重载
public int max(int i,int j) {
return (i > j)? i:j;
}
public double max(double d1,double d2) {
return (d1 > d2)? d1:d2;
}
public double max(double d1,double d2,double d3) {
double max = (d1 > d2)? d1:d2;
return (max > d3)? max:d3;
}
}
package com.atguigu.exer;
/*
* 1.编写程序,定义三个重载方法并调用。方法名为mOL。
* >三个方法分别接收一个int参数、两个int参数、一个字符串参数。
* 分别执行平方运算并输出结果,相乘并输出结果,输出字符串信息。
* >在主类的main ()方法中分别用参数区别调用三个方法。
*
* 2.定义三个重载方法max()
* 第一个方法求两个int值中的最大值
* 第二个方法求两个double值中的最大值,
* 第三个方法求三个double值中的最大值
* 并分别调用三个方法。
*
*/
public class OverLoadTest {
public static void main(String[] args) {
OverLoadExer test = new OverLoadExer();
test.mOL(3);
test.mOL(4, 30);
test.mOL("方法重载");
int imax = test.max(2, 6);
System.out.println("最大值:"+imax);
double dmax = test.max(5.1, 6.8);
System.out.println("最大值:"+dmax);
double smax = test.max(6.4, -4, 88);
System.out.println("最大值:"+smax);
}
}
8.编写一个method方法
public static void method(int a,int b) {
a = a*10;
b = b*20;
System.out.println(a);
System.out.println(b);
System.exit(0);//退出程序。main方法中在此之后的命令行不会再执行。
}
9.定义一个int型的数组:int[] arr = new int[]{12,3,3,34,56,77,432};
让数组的每个位置上的值去除以首位置的元素,得到的结果,作为该位置上的 新值。遍历新的数组。
public class WeiRuanTest {
public static void main(String[] args){
int[] arr = new int[]{12,3,3,34,56,77,432};
Util test = new Util();
test.print(arr);
test.division(arr);
test.print(arr);
}
}
class Util{
public void print(int[] arr) {
for(int i=0;i<arr.length;i++) {
System.out.print(arr[i]+" ");
}
System.out.println();
}
public void division(int[] arr) {
/*错误写法:arr[0]变为1,之后的每一位除的数都变成了1
for(int i= 0;i < arr.length;i++){
arr[i] = arr[i] / arr[0];
}
*/
//正确写法1:从后往前,arr[0]最后改变
for(int i = arr.length - 1;i >= 0;i--){
arr[i] = arr[i] / arr[0];
}
//正确写法2
/*int temp = arr[0];//将arr[0]的值赋给一个变量,arr[0]变为1,变量值不改变
for(int i= 0;i < arr.length;i++){
arr[i] = arr[i] / temp;
}*/
}
}
10、值传递练习
package com.atguigu.exer1;
/*
*
* 定义一个Circle类,包含一个double型的radius属性代表圆的半径,
* 一个findArea()方法返回圆的面积。
*
*
*
*
*/
public class Circle {
double radius;//半径
//求圆的面积
public double findArea(){
//double area = Math.PI*radius*radius;
return Math.PI*radius*radius;
}
}
package com.atguigu.exer1;
/*
*
* 定义一个类PassObject,在类中定义一个方法printAreas(),
* 该方法的定义如下:public void printAreas(Circle c, int time)
* 在printAreas方法中打印输出1到time之间的每个整数半径值,以及对应的面积。
* 例如,times为5,则输出半径1,2,3,4,5,以及对应的圆面积。
*
* 在main方法中调用printAreas()方法,调用完毕后输出当前半径值。程序运行结果如图所示。
*
*/
public class PassObject {
public static void main(String[] args) {
Circle c = new Circle();
PassObject pass = new PassObject();
pass.printAreas(c,5);
}
public void printAreas(Circle c,int time) {
System.out.println("Radius"+"\t"+"\t"+"Area");
for(int i=1;i<=time;i++) {
//设置圆的半径
c.radius = i;
double area = c.findArea();
System.out.println(((double)i)+"\t"+"\t"+area);
}
c.radius = time+1;
System.out.println("now radius is:"+c.radius);
}
}
11.递归
例1.实现1~100自然数求和
例2:计算1~n之间所有自然数的乘积:n!
例3.已知有一个数列:f(0) = 1,f(1) = 4,f(n+2)=2*f(n+1) + f(n),其中n是大于0的整数,求f(10)的值。
例4:斐波那契数列:500阶台阶问走完这些台阶一共有几种方法
package com.atguigu.java2;
/*
* 递归方法的使用
* 1.定义:递归方法:一个方法体内调用它自身。
* 2.方法递归包含了一种隐式的循环,它会重复执行某段代码,但这种重复执行无须循环控制。
* 递归一定要向已知方向递归,否则这种递归就变成了无穷递归,类似于死循环。
*
*
*/
public class RecursionTest {
public static void main(String[] args) {
//例1:计算1~100之间所有自然数的和
//方式一
int sum = 0;
for(int i=0;i<=100;i++) {
sum += i;
}
System.out.println(sum);
//方式二
RecursionTest test = new RecursionTest();
int sum1 = test.getSum(100);
System.out.println(sum1);
//例2:计算1~n之间所有自然数的乘积:n!
//乘积:product
int product = test.getProduct(100);
System.out.println(product);
//例3.已知有一个数列:f(0) = 1,f(1) = 4,f(n+2)=2*f(n+1) + f(n),
//其中n是大于0的整数,求f(10)的值。
int value = test.f(10);
System.out.println(value);
//例4:斐波那契数列
//500阶台阶问走完这些台阶一共有几种方法
int value1 = test.fibonacc(10);
System.out.println(value1);
}
//例1:计算1~100之间所有自然数的和
public int getSum(int n) {
if(n == 1) {//递归终止
return 1;
}else {
return n + getSum(n-1);
}
}
//例2:计算1~n之间所有自然数的乘积:n!
public int getProduct(int n) {
if(n == 1) {//递归终止
return 1;
}else {
return n * getSum(n-1);
}
}
//例3:已知有一个数列:f(0) = 1,f(1) = 4,f(n+2)=2*f(n+1) + f(n),
//其中n是大于0的整数,求f(10)的值。
public int f(int n) {
if(n == 0) {
return 1;
}else if(n == 1) {
return 4;
}else {
//错误:return f(n+2)-2*f(n+1);
return 2*f(n-1) + f(n-2);
}
}
//例4:斐波那契数列(Fibonacc)
//500阶台阶问走完这些台阶一共有几种方法
public int fibonacc(int n) {
if(n == 1) {
return 1;
}else if(n == 2) {
return 1;
}else {
return fibonacc(n - 1)+fibonacc(n - 2);
}
}
}
12.封装与隐藏
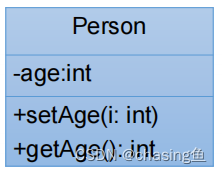
package com.atguigu.exer;
public class Person {
private int age;
public void setAge(int a) {//set(设置)::赋值
/*方式一
* if(a >0 && a<13.) {
age = a;
}else {
//throw new RuntimeException("传入非法数据");
System.out.println("传入非法数据");
}*/
/*方式二
* if(a < 0 || a > 130) {
* //throw new RuntimeException("传入非法数据");
System.out.println("传入非法数据");
}else {
age = a;
}*/
//方式三
if(a < 0 || a > 130) {
System.out.println("传入非法数据");
return;
}
age = a;
}
public int getAge() {//返回,数据(值):看看现在的值是多少
return age;
}
//绝对不能这样写:功能不明确:在Java中get就是get,set就是set
public int doAge(int a) {
age = a;
return age;
}
}
package com.atguigu.exer;
public class PersonTest {
public static void main(String[] args) {
Person b = new Person();
// b.age = 1;//编译不通过
b.setAge(12);
System.out.println("年龄为:"+b.getAge());
// b.doAge(122);//功能混乱
}
}
13.构造器
package com.atguigu.exer1;
/*
* 编写两个类,TriAngle和TriAngleTest,其中TriAngle类中声明私有的底边长base和高height,
* 同时声明公共方法访问私有变量。此外,提供类必要的构造器。另一个类中使用这些公共方法,计算三角形的面积。
*
*
*/
public class TriAngle {//Angle:角 angel:天使
//属性
private double base;//底边长
private double height;//高
//构造器
public TriAngle() {//开发中习惯性定义一个空参的构造器
}
public TriAngle(double b,double h) {
base = b;
height = h;
}
//方法
public void setBase(double b) {
base = b;
}
public double getBase() {
return base;
}
public void setHeight(double h) {
height = h;
}
public double getHerght() {
return height;
}
}
package com.atguigu.exer1;
public class TriAngleTest {
public static void main(String[] args) {
TriAngle angle = new TriAngle(4, 6);
System.out.println("三角形面积为:"+(angle.getBase()*angle.getHerght())/2);
TriAngle t = new TriAngle();
t.setBase(2.3);
t.setHeight(5.4);
System.out.println("base:"+t.getBase()+",height"+t.getHerght());
System.out.println("三角形面积为:"+(t.getBase()*t.getHerght())/2);
}
}
14.关键字—this
package com.atguigu.exer2;
public class Boy {
private String name;
private int age;
//构造器
public Boy() {
}
public Boy(String name, int age) {
this.name = name;
this.age = age;
}
//方法
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public void marry(Girl girl) {
System.out.println("我想娶"+girl.getName());
}
public void shout() {
if(age >= 22) {
System.out.println("你可以合法登记结婚了!");
}else {
System.out.println("先多谈谈恋爱~~");
}
}
}
package com.atguigu.exer2;
public class Girl {
private String name;
private int age;
//构造器
public Girl() {
}
public Girl(String name, int age) {
this.name = name;
this.age = age;
}
//方法
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void marry(Boy boy) {
System.out.println("我想嫁给"+boy.getName());
boy.marry(this);//this:当前对象,谁调用marry,谁就是this
}
/**
* @Description 比较两个对象的大小
* @author Administrator
* @param girl
* @return 正数:当前对象大;负数:当前对象小; 0:当前对象与形参对象相等
*/
public int compare(Girl girl) {//compare:比较
// if(this.age > girl.age) {
// return 1;
// }else if(this.age < girl.age) {
// return -1;
// }else {
// return 0;
// }
return this.age - girl.age;
}
}
package com.atguigu.exer2;
public class BoyGirlTest {
public static void main(String[] args) {
Boy boy = new Boy("罗密欧", 21);
boy.shout();
Girl girl = new Girl("朱丽叶", 18);
girl.marry(boy);
Girl girl1 = new Girl("祝英台",19);
int compare = girl.compare(girl1);
if(compare > 0) {
System.out.println(girl.getName()+"大");
}else if(compare < 0) {
System.out.println(girl1.getName()+"大");
}else {
System.out.println("一样大");
}
}
}
三、实验
1.实验一
package com.atguigu.exer3;
/*
* 实验一
*
*/
import java.util.Scanner;
public class Account {
//属性
private int id;//账号
private double balance;//余额
private double annuallnteresrRate;//年利率
//构造器
public Account() {
}
public Account(int id,double balance,double annuallnterestRate) {
this.balance = balance;
this.annuallnteresrRate = annuallnterestRate;
while(true) {
if(id >=1000 && id<100000000) {
this.id = id;
break;
}else {
System.out.println("请重新输入账号!");
Scanner scan = new Scanner(System.in);
System.out.print("id为:");
id = scan.nextInt();
}
}
}
//方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
public double getAnnuallnteresrRate() {
return annuallnteresrRate;
}
public void setAnnuallnteresrRate(double annuallnteresrRate) {
this.annuallnteresrRate = annuallnteresrRate;
}
public void withdraw(double amount) {//取钱 amount:数量金额
if(amount > this.balance) {
System.out.println("余额不足,取款失败");
}else {
this.balance -= amount;
System.out.println("成功取出:"+amount);
}
}
public void deposit(double amount) {
if(amount > 0) {
balance += amount;
System.out.println("成功存入:"+amount);
}
}
public String accUser() {
annuallnteresrRate *= 100;
return "id is"+" "+id+",annuallnterestRate is"+" "+annuallnteresrRate+"%"
+",balance is "+balance;
}
}
package com.atguigu.exer3;
public class Customer {
//属性
private String firstName;
private String lastName;
private Account account;
//构造器
public Customer() {
}
public Customer(String f,String l) {
firstName = f;
lastName = l;
}
//方法
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public void getUser() {
System.out.println("Customer"+"["+lastName+","+firstName+"]"+" "+
"has a account: "+account.accUser());
}
}
package com.atguigu.exer3;
public class CustomerTest {
public static void main(String[] args) {
Customer customer = new Customer("Jane", "Smith");
//customer.setAccount(new Account(1000, 2000,0.0123));
Account acct = new Account(1000, 2000,0.0123);
customer.setAccount(acct);
//System.out.println(customer.getAccount().getAnnuallnteresrRate());
customer.getAccount().deposit(100);//存款100
customer.getAccount().withdraw(960);//取款960
customer.getAccount().withdraw(2000);
customer.getUser();
}
}
2.实验二
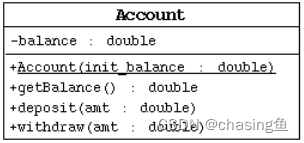
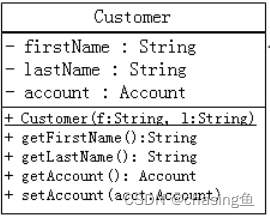
>addCustomer 方法必须依照参数(姓,名)构造一个新的 Customer 对象,然后把
package com.atguigu.shiyan2;
public class Account {
//属性
private double balance;
//构造器
public Account() {
}
public Account(double init_balance) {
this.balance = init_balance;
}
//方法
public double getBalance() {
return balance;
}
public void deposit(double amt) {//存款
if(amt > 0) {
balance += amt;
System.out.println("成功存入"+amt);
}
}
public void withdraw(double amt) {//取款
if(balance >= amt) {
balance -= amt;
System.out.println("成功取出"+amt);
}else {
System.out.println("余额不足,取款失败");
}
}
}
package com.atguigu.shiyan2;
import com.atguigu.shiyan2 .Account;
public class Customer {
//属性
private String firstName;
private String lastName;
private Account account;
//构造器
public Customer() {
}
public Customer(String f,String l) {
firstName = f;
lastName = l;
}
//方法
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
}
package com.atguigu.shiyan2;
public class Bank {
//属性
private Customer[] customer;//对象数组,存放多个客户。
private int numberOfCustomer ;//记录客户的个数,默认初始化为0
//构造器
public Bank() {
customer = new Customer[10];//数组的动态初始化
}
//方法
//添加客户
该操作相当于数组元素赋值:在之前要进行数组初始化,否则会报空指针异常
public void addCustomer(String f,String l) {
//customer[numberOfCustomer] = new Customer(f, l);
Customer cust = new Customer(f, l);
/*customer[numberOfCustomer] = cust;
numberOfCustomer++;
或*/
customer[numberOfCustomer++] = cust;
}
//获取客户指定位置上的客户
public Customer getCustomer(int index) {
// return customer[index];可能报异常
if(index >= 0 && index <= numberOfCustomer) {
return customer[index];
}
return null;
}
//获取客户的个数
public int getNumberOfCustomer() {
return numberOfCustomer;
}
}
package com.atguigu.shiyan2;
public class BankTest {
public static void main(String[] args) {
Bank bank = new Bank();
bank.addCustomer("Jane", "Smith");
//连续操作
bank.getCustomer(0).setAccount(new Account(2000));
bank.getCustomer(0).getAccount().deposit(100);//存款
bank.getCustomer(0).getAccount().withdraw(960);//取款
bank.getCustomer(0).getAccount().withdraw(2000);//取款
double balance = bank.getCustomer(0).getAccount().getBalance();
//客户+账户余额
System.out.println("客户:"+bank.getCustomer(0).getFirstName()+bank.getCustomer(0).getLastName()+" 的账户余额:"+balance);
System.out.println("*****************");
bank.addCustomer("杨", "幂");
bank.getCustomer(1).setAccount(new Account(2000));
bank.getCustomer(1).getAccount().withdraw(960);//取款
double balance1 = bank.getCustomer(1).getAccount().getBalance();
System.out.println("客户:"+bank.getCustomer(1).getFirstName()+bank.getCustomer(1).getLastName()+" 的账户余额:"+balance1);
System.out.println("银行客户的个数为:"+bank.getNumberOfCustomer());
}
}