package com.jarvis.base.util;
/**
*
*
* @Title: ReflectHelper.java
* @Package com.jarvis.base.util
* @Description: 反射工具类
* @version V1.0
*/
public class ReflectHelper {
/**
* 提指定的类载入以系统中
*
* @param name
* 类名称
* @return 类对象
* @throws ClassNotFoundException
*/
public static Class<?> classForName(String name) throws ClassNotFoundException {
try {
return Thread.currentThread().getContextClassLoader().loadClass(name);
}
catch (ClassNotFoundException e) {
e.printStackTrace();
System.err.println("类[" + name + "]加载出错");
} catch (SecurityException e) {
e.printStackTrace();
System.err.println("类[" + name + "]加载出错");
}
return Class.forName(name);
}
/**
* 根据名称生成指定的对象
*
* @param name
* 类名称
* @return 具体的对象,若发生异常,则返回null
*/
public static Object objectForName(String name) {
try {
return Class.forName(name).newInstance();
} catch (Exception ex) {
ex.printStackTrace();
System.err.println("类[" + name + "]获取对象实例出错");
}
return null;
}
}
package com.jarvis.base.util;
import java.io.UnsupportedEncodingException;
import java.text.DecimalFormat;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.StringTokenizer;
import java.util.UUID;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
*
*
* @Title: StringHelper.java
* @Package com.jarvis.base.utils
* @Description:
* @version V1.0 字符串处理工具类。
*/
public final class StringHelper {
/**
* 描述: 构造方法
*/
private StringHelper() {
}
/**
* 空字符串
*/
public static final String EMPTY_STRING = "";
/**
* 点
*/
public static final char DOT = '.';
/**
* 下划线
*/
public static final char UNDERSCORE = '_';
/**
* 逗点及空格
*/
public static final String COMMA_SPACE = ", ";
/**
* 逗点
*/
public static final String COMMA = ",";
/**
* 开始括号
*/
public static final String OPEN_PAREN = "(";
/**
* 结束括号
*/
public static final String CLOSE_PAREN = ")";
/**
* 单引号
*/
public static final char SINGLE_QUOTE = '\'';
/**
* 回车
*/
public static final String CRLF = "\r\n";
/**
* 常量 12
*/
public static final int FIANL_TWELVE = 12;
/**
* 十六进制常量 0x80
*/
public static final int HEX_80 = 0x80;
/**
* 十六进制常量 0xff
*/
public static final int HEX_FF = 0xff;
/**
* 把字符数组,转化为一个字符
*
* @param seperator
* 字符分隔符
* @param strings
* 数组对象
* @return 字符串
*/
public static String join(String seperator, String[] strings) {
int length = strings.length;
if (length == 0) {
return EMPTY_STRING;
}
StringBuffer buf = new StringBuffer(length * strings[0].length()).append(strings[0]);
for (int i = 1; i < length; i++) {
buf.append(seperator).append(strings[i]);
}
return buf.toString();
}
/**
* 把迭代对象转化为一个字符串
*
* @param seperator
* 分隔符
* @param objects
* 迭代器对象
* @return 字符串
*/
public static String join(String seperator, Iterator<?> objects) {
StringBuffer buf = new StringBuffer();
if (objects.hasNext()) {
buf.append(objects.next());
}
while (objects.hasNext()) {
buf.append(seperator).append(objects.next());
}
return buf.toString();
}
/**
* 把两个字符串数组的元素用分隔符连接,生成新的数组,生成的数组以第一个字符串数组为参照,与其长度相同。
*
* @param x
* 字符串数组
* @param seperator
* 分隔符
* @param y
* 字符串数组
* @return 组合后的字符串数组
*/
public static String[] add(String[] x, String seperator, String[] y) {
String[] result = new String[x.length];
for (int i = 0; i < x.length; i++) {
result[i] = x[i] + seperator + y[i];
}
return result;
}
/**
* 生成一个重复的字符串,如需要重复*10次,则生成:**********。
*
* @param string
* 重复元素
* @param times
* 重复次数
* @return 生成后的字符串
*/
public static String repeat(String string, int times) {
StringBuffer buf = new StringBuffer(string.length() * times);
for (int i = 0; i < times; i++) {
buf.append(string);
}
return buf.toString();
}
/**
* 字符串替换处理,把旧的字符串替换为新的字符串,主要是通过字符串查找进行处理
*
* @param source
* 需要进行替换的字符串
* @param old
* 需要进行替换的字符串
* @param replace
* 替换成的字符串
* @return 替换处理后的字符串
*/
public static String replace(String source, String old, String replace) {
StringBuffer output = new StringBuffer();
int sourceLen = source.length();
int oldLen = old.length();
int posStart = 0;
int pos;
// 通过截取字符串的方式,替换字符串
while ((pos = source.indexOf(old, posStart)) >= 0) {
output.append(source.substring(posStart, pos));
output.append(replace);
posStart = pos + oldLen;
}
// 如果还有没有处理的字符串,则都添加到新字符串后面
if (posStart < sourceLen) {
output.append(source.substring(posStart));
}
return output.toString();
}
/**
* 替换字符,如果指定进行全替换,必须设wholeWords=true,否则只替换最后出现的字符。
*
* @param template
* 字符模板
* @param placeholder
* 需要替换的字符
* @param replacement
* 新的字符
* @param wholeWords
* 是否需要全替换,true为需要,false为不需要。如果不需要,则只替换最后出现的字符。
* @return 替换后的新字符
*/
public static String replace(String template, String placeholder, String replacement, boolean wholeWords) {
int loc = template.indexOf(placeholder);
if (loc < 0) {
return template;
} else {
final boolean actuallyReplace = wholeWords || loc + placeholder.length() == template.length()
|| !Character.isJavaIdentifierPart(template.charAt(loc + placeholder.length()));
String actualReplacement = actuallyReplace ? replacement : placeholder;
return new StringBuffer(template.substring(0, loc)).append(actualReplacement).append(
replace(template.substring(loc + placeholder.length()), placeholder, replacement, wholeWords))
.toString();
}
}
/**
* 替换字符,只替换第一次出现的字符串。
*
* @param template
* 字符模板
* @param placeholder
* 需要替换的字符串
* @param replacement
* 新字符串
* @return 替换后的字符串
*/
public static String replaceOnce(String template, String placeholder, String replacement) {
int loc = template.indexOf(placeholder);
if (loc < 0) {
return template;
} else {
return new StringBuffer(template.substring(0, loc)).append(replacement)
.append(template.substring(loc + placeholder.length())).toString();
}
}
/**
* 把字符串,按指字的分隔符分隔为字符串数组
*
* @param seperators
* 分隔符
* @param list
* 字符串
* @return 字符串数组
*/
public static String[] split(String list, String seperators) {
return split(list, seperators, false);
}
/**
* 把字符串,按指字的分隔符分隔为字符串数组
*
* @param seperators
* 分隔符
* @param list
* 字符串
* @param include
* 是否需要把分隔符也返回
* @return 字符串数组
*/
public static String[] split(String list, String seperators, boolean include) {
StringTokenizer tokens = new StringTokenizer(list, seperators, include);
String[] result = new String[tokens.countTokens()];
int i = 0;
while (tokens.hasMoreTokens()) {
result[i++] = tokens.nextToken();
}
return result;
}
/**
* 提取字符串中,以.为分隔符后的所有字符,如string.exe,将返回exe。
*
* @param qualifiedName
* 字符串
* @return 提取后的字符串
*/
public static String unqualify(String qualifiedName) {
return unqualify(qualifiedName, ".");
}
/**
* 提取字符串中,以指定分隔符后的所有字符,如string.exe,将返回exe。
*
* @p
Java常用工具类---Reflect反射工具类、String字符串工具类
最新推荐文章于 2023-05-10 14:46:26 发布
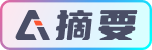