Description
Nemo is a naughty boy. One day he went into the deep sea all by himself. Unfortunately, he became lost and couldn't find his way home. Therefore, he sent a signal to his father, Marlin, to ask for help.
After checking the map, Marlin found that the sea is like a labyrinth with walls and doors. All the walls are parallel to the X-axis or to the Y-axis. The thickness of the walls are assumed to be zero.
All the doors are opened on the walls and have a length of 1. Marlin cannot go through a wall unless there is a door on the wall. Because going through a door is dangerous (there may be some virulent medusas near the doors), Marlin wants to go through as few doors as he could to find Nemo.
Figure-1 shows an example of the labyrinth and the path Marlin went through to find Nemo.
We assume Marlin's initial position is at (0, 0). Given the position of Nemo and the configuration of walls and doors, please write a program to calculate the minimum number of doors Marlin has to go through in order to reach Nemo.
After checking the map, Marlin found that the sea is like a labyrinth with walls and doors. All the walls are parallel to the X-axis or to the Y-axis. The thickness of the walls are assumed to be zero.
All the doors are opened on the walls and have a length of 1. Marlin cannot go through a wall unless there is a door on the wall. Because going through a door is dangerous (there may be some virulent medusas near the doors), Marlin wants to go through as few doors as he could to find Nemo.
Figure-1 shows an example of the labyrinth and the path Marlin went through to find Nemo.
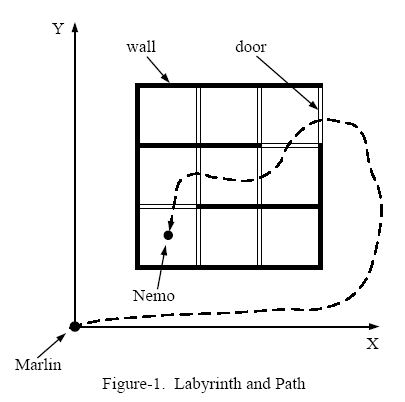
We assume Marlin's initial position is at (0, 0). Given the position of Nemo and the configuration of walls and doors, please write a program to calculate the minimum number of doors Marlin has to go through in order to reach Nemo.
Input
The input consists of several test cases. Each test case is started by two non-negative integers M and N. M represents the number of walls in the labyrinth and N represents the number of doors.
Then follow M lines, each containing four integers that describe a wall in the following format:
x y d t
(x, y) indicates the lower-left point of the wall, d is the direction of the wall -- 0 means it's parallel to the X-axis and 1 means that it's parallel to the Y-axis, and t gives the length of the wall.
The coordinates of two ends of any wall will be in the range of [1,199].
Then there are N lines that give the description of the doors:
x y d
x, y, d have the same meaning as the walls. As the doors have fixed length of 1, t is omitted.
The last line of each case contains two positive float numbers:
f1 f2
(f1, f2) gives the position of Nemo. And it will not lie within any wall or door.
A test case of M = -1 and N = -1 indicates the end of input, and should not be processed.
Then follow M lines, each containing four integers that describe a wall in the following format:
x y d t
(x, y) indicates the lower-left point of the wall, d is the direction of the wall -- 0 means it's parallel to the X-axis and 1 means that it's parallel to the Y-axis, and t gives the length of the wall.
The coordinates of two ends of any wall will be in the range of [1,199].
Then there are N lines that give the description of the doors:
x y d
x, y, d have the same meaning as the walls. As the doors have fixed length of 1, t is omitted.
The last line of each case contains two positive float numbers:
f1 f2
(f1, f2) gives the position of Nemo. And it will not lie within any wall or door.
A test case of M = -1 and N = -1 indicates the end of input, and should not be processed.
Output
For each test case, in a separate line, please output the minimum number of doors Marlin has to go through in order to rescue his son. If he can't reach Nemo, output -1.
题意:输入m和n,接下来m行每行输入x,y,d,t,如果d=1,则以y轴正方向在(x,y)的上方垒t个墙(墙与y轴平行);如果d=0,则以x轴正方向在(x,y)的右方垒t个墙(墙与x轴平行)。接下来n行每行输入x,y,d,如果d=1,则在(x,y)的上方的墙上有一个门;如果d=0,则在(x,y)的右方的墙上有一个门。Nemo就被困在了这个由墙和门构建的迷宫里,他的父亲在(0,0)处,求他父亲要救到他至少要穿过几扇门。
思路:让Nemo直接从他所在位置走出来,他穿过的门数即他父亲救他穿过的门数,如果他本身就在迷宫外,则所穿门数为0。为了便于搜索,把整个图整体向右上方移了0.5个单位,这样,墙和门所在的位置是小数,而Nemo的坐标即变成了整数,具体怎么移的请看代码。
Sample Input
8 9 1 1 1 3 2 1 1 3 3 1 1 3 4 1 1 3 1 1 0 3 1 2 0 3 1 3 0 3 1 4 0 3 2 1 1 2 2 1 2 3 1 3 1 1 3 2 1 3 3 1 1 2 0 3 3 0 4 3 1 1.5 1.5 4 0 1 1 0 1 1 1 1 1 2 1 1 1 1 2 0 1 1.5 1.7 -1 -1
Sample Output
5 -1
<span style="color:#0000ff;">#include <cstdio>
#include <queue>
#include <cstring>
#define MAX 100002
using namespace std;
struct node
{
int d[4]; //d[0]代表上,d[1]代表下,d[2]代表左,d[3]代表右
int sum; //标记该点的步数
};
node mapp[205][205];
bool vis[205][205];
bool ff[205][205];
int n,m;
int dx[]= {0,0,-1,1};
int dy[]= {1,-1,0,0};
void Setwall() //开始垒墙
{
int x,y,d,t;
while(m--)
{
scanf("%d %d %d %d",&x,&y,&d,&t);
while(t--)
{
if(d) //如果是竖着垒墙
{
y++;
mapp[x][y].d[3]=1; //(x,y)的右边有墙
mapp[x+1][y].d[2]=1; //(x+1,y)的左边有墙
if(!ff[x][y])
{
ff[x][y]=1; //标记(x,y)房间已经建好
mapp[x][y].d[0]=mapp[x][y].d[1]=mapp[x][y].d[2]=-1;//除了有墙的另外三个方向什么都没有,标记为-1
}
if(!ff[x+1][y])
{
ff[x+1][y]=1;
mapp[x+1][y].d[0]=mapp[x+1][y].d[1]=mapp[x+1][y].d[3]=-1;
}
}
else //同理,此时是横着垒墙
{
x++;
mapp[x][y].d[0]=1;
mapp[x][y+1].d[1]=1;
if(!ff[x][y])
{
ff[x][y]=1;
mapp[x][y].d[1]=mapp[x][y].d[2]=mapp[x][y].d[3]=-1;
}
if(!ff[x][y+1])
{
ff[x][y+1]=1;
mapp[x][y+1].d[0]=mapp[x][y+1].d[2]=mapp[x][y+1].d[3]=-1;
}
}
}
}
}
void Setdoor() //开始在墙上安门
{
int x,y,d;
while(n--)
{
scanf("%d %d %d",&x,&y,&d);
if(d)
{
mapp[x][y+1].d[3]=0; //如果是门标记为0
mapp[x+1][y+1].d[2]=0;
}
else
{
mapp[x+1][y].d[0]=0;
mapp[x+1][y+1].d[1]=0;
}
}
}
int BFS(int x,int y)
{
int xx,yy;
queue<pair<int,int> >qq; //一次要存两个数的队列要用pair<类型,类型>
vis[x][y]=true;
mapp[x][y].sum=0;
qq.push(pair<int,int>(x,y));
if(!ff[x][y]) //如果此点未被标记说明</span><span style="color: rgb(51, 102, 255); font-family: 'Times New Roman', Times, serif;font-size:18px;">Nemo已在迷宫外,直接返回0</span><span style="color:#0000ff;">
return 0;
while(!qq.empty())
{
x=qq.front().first;
y=qq.front().second;
qq.pop();
for(int i=0; i<4; i++) //朝此房间的四个方向走
{
xx=x+dx[i];
yy=y+dy[i];
if(mapp[x][y].d[i]==-1) //如果此房间的某个方向是-1,说明</span><span style="font-family: 'Times New Roman', Times, serif;font-size:18px;"><span style="color:#3333ff;">Nemo已走出迷宫</span></span><span style="color:#0000ff;">
return mapp[x][y].sum;
else if(!mapp[x][y].d[i]&&!vis[xx][yy])
{
vis[xx][yy]=1; //如果此房间的此方向是门且未访问过则接着进队列
mapp[xx][yy].sum=mapp[x][y].sum+1;
qq.push(pair<int,int>(xx,yy));
}
}
}
return -1; //如果走不出直接返回-1
}
int main()
{
//freopen("oo.text","r",stdin);
double x,y;
while(~scanf("%d %d",&m,&n))
{
if(m==-1&&n==-1)
break;
memset(vis,0,sizeof(vis));
memset(ff,0,sizeof(ff));
Setwall();
Setdoor();
scanf("%lf %lf",&x,&y);
if(x<1||x>199||y<1||y>199||m==0)
printf("0\n");
else
printf("%d\n",BFS((int)(x+1),(int)(y+1)));
}
return 0;
}
</span>