Description
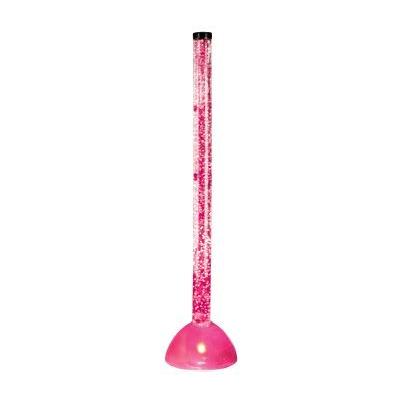
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
题意:多组输入,每组输入n个数,如果用冒泡排序对这n个数排序求交换的次数。
思路:如果数据小的话是可以直接用冒泡排序然后用计数器记录交换次数,可现在数据比较大所以再用冒泡直接排肯定会超时,则此时可以用归并排序去求解。
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
#include <cstdio>
#include <cstring>
#include <algorithm>
using namespace std;
long long int a[500020],b[500020],sum;
void mergesort(long long int *sett,long long int *t,int l,int r) //归并排序
{
if(l<r)
{
int m=(r+l)/2; //只要还没有分到最小块就继续往下分
mergesort(sett,t,l,m);
mergesort(sett,t,m+1,r);
int i=l,j=m+1,k=l; //i是指向左块的指针,j是指向右块的指针,k是指向暂时数组的指针
while(i<=m&&j<=r)
{
if(sett[i]<=sett[j]) //如果左边的数小于右边的数,就将左边的数存入暂时数组中
t[k++]=sett[i++];
else //如果有右边的数小于左边的数,就将右边的数存入暂时数组中
{
t[k++]=sett[j++];
sum+=m+1-i; //此时就说明sett[j]前边的逆序数有m+1-i个
}
}
while(i<=m)
t[k++]=sett[i++];
while(j<=r)
t[k++]=sett[j++];
for(int p=l; p<=r; p++) //将暂时数组的数复制到原数组中
{
sett[p]=t[p];
}
}
return ;
}
int main()
{
//freopen("oo.text","r",stdin);
int n;
while(~scanf("%d",&n)&&n)
{
sum=0;
for(int i=1; i<=n; i++)
scanf("%lld",&a[i]);
memset(b,0,sizeof(b));
mergesort(a,b,1,n);
printf("%lld\n",sum);
}
return 0;
}