C语言小游戏——4*8拼图游戏(有源码)
视频介绍
用入门C语言编写4*8拼图游戏
图片展示
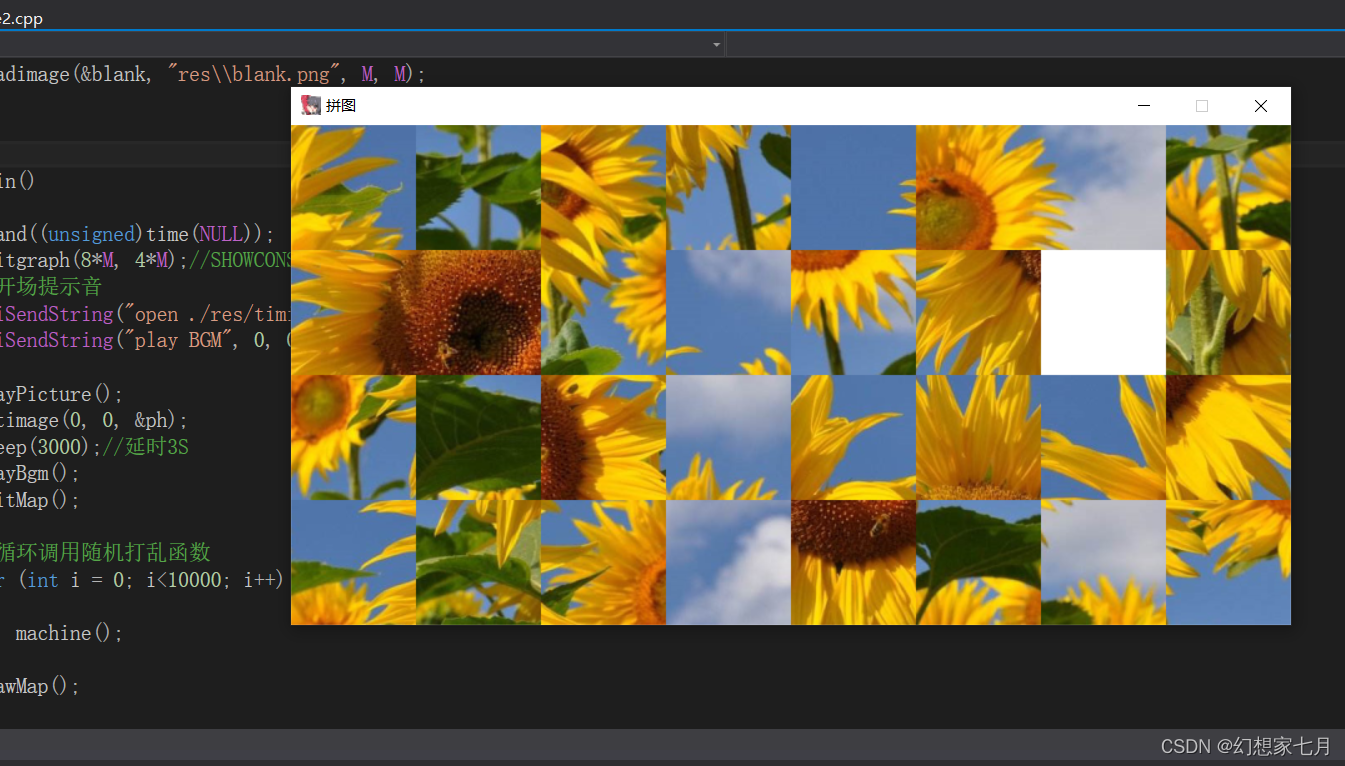
功能介绍
- 原图反向随机打乱,百分之百有解
- 鼠标右键查看原图
- 随机加载背景音乐、移动音效等
- 随机加载图片
- 源码如下,所用到的资源包及成品exe见上面视频链接
源码(编译环境:vs2013)
#include <stdio.h>
#include <stdlib.h>
#include<graphics.h>
#include<time.h>
#include<windows.h>
#include<mmsystem.h>
#pragma comment(lib,"winmm.lib")
#define M 100
IMAGE ph, blank, ph1;
int arr[31] = { 0 };
int map[4][8] = { 0 };
void initMap()
{
int i, j, m = 0;
int pos;
for (i = 0; i<32; i++)
{
arr[i] = i;
}
for (i = 0; i<4; i++)
{
for (j = 0; j<8; j++)
{
pos = m;
map[i][j] = arr[pos];
m++;
}
}
}
void drawMap()
{
for (int i = 0; i<4; i++)
{
for (int j = 0; j<8; j++)
{
int x = j * M;
int y = i * M;
switch (map[i][j])
{
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
case 7:
putimage(x, y, M, M, &ph, map[i][j] * M, 0);
break;
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
putimage(x, y, M, M, &ph, (map[i][j] - 8) * M, M);
break;
case 16:
case 17:
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
putimage(x, y, M, M, &ph, (map[i][j] - 16) * M, 2*M);
break;
case 24:
case 25:
case 26:
case 27:
case 28:
case 29:
case 30:
putimage(x, y, M, M, &ph, (map[i][j] - 24) * M, 3*M);
break;
case 31:
putimage(x, y, &blank);
break;
}
}
}
}
int searchPos_i()
{
for (int i = 0; i<4; i++)
{
for (int j = 0; j<8; j++)
{
if (map[i][j] == 31)
return i;
}
}
return -1;
}
int searchPos_j()
{
for (int i = 0; i<4; i++)
{
for (int j = 0; j<8; j++)
{
if (map[i][j] == 31)
return j;
}
}
return -1;
}
void machine()
{
int m, n;
int pos_i = searchPos_i();
int pos_j = searchPos_j();
int mouse_i = 0;
int mouse_j = 0;
m = rand() % 4*M;
n = rand() % 8*M;
mouse_i = m / M;
mouse_j = n / M;
if (mouse_i == pos_i - 1 && mouse_j == pos_j)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
}
if (mouse_i == pos_i + 1 && mouse_j == pos_j)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
}
if (mouse_i == pos_i && mouse_j == pos_j - 1)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
}
if (mouse_i == pos_i && mouse_j == pos_j + 1)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
}
}
void palyGame()
{
ExMessage m;
while (map[0][0] != 0 || map[0][1] != 1 || map[0][2] != 2 || map[0][3] != 3 || map[0][4] != 4 || map[0][5] != 5 || map[0][6] != 6 || map[0][7] != 7 || map[1][0] != 8 || map[1][1] != 9 || map[1][2] != 10 || map[1][3] != 11 || map[1][4] != 12 || map[1][5] != 13 || map[1][6] != 14 || map[1][7] != 15 || map[2][0] != 16 || map[2][1] != 17 || map[2][2] != 18 || map[2][3] != 19 || map[2][4] != 20 || map[2][5] != 21 || map[2][6] != 22 || map[2][7] != 23 || map[3][0] != 24 || map[3][1] != 25 || map[3][2] != 26 || map[3][3] != 27 || map[3][4] != 28 || map[3][5] != 29 || map[3][6] != 30 || map[3][7] != 31)
{
int pos_i = searchPos_i();
int pos_j = searchPos_j();
int mouse_i = 0;
int mouse_j = 0;
m = getmessage();
if (m.message==WM_RBUTTONDOWN)
{
putimage(0, 0, &ph);
}
if (m.message==WM_RBUTTONUP)
drawMap();
if (m.message==WM_LBUTTONDOWN)
{
mouse_i = m.y / M;
mouse_j = m.x / M;
if (mouse_i == pos_i - 1 && mouse_j == pos_j)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
drawMap();
}
if (mouse_i == pos_i + 1 && mouse_j == pos_j)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
drawMap();
}
if (mouse_i == pos_i && mouse_j == pos_j - 1)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
drawMap();
}
if (mouse_i == pos_i && mouse_j == pos_j + 1)
{
map[pos_i][pos_j] = map[mouse_i][mouse_j];
map[mouse_i][mouse_j] = 31;
drawMap();
}
}
}
putimage(0, 0, &ph);
}
void playBgm()
{
int y;
y = rand() % 8;
switch (y)
{
case 0:mciSendString("open ./res/beijingyinyue0.mp3 alias BGM1", 0, 0, 0);
break;
case 1:mciSendString("open ./res/beijingyinyue1.mp3 alias BGM1", 0, 0, 0);
break;
case 2:mciSendString("open ./res/beijingyinyue2.mp3 alias BGM1", 0, 0, 0);
break;
case 3:mciSendString("open ./res/beijingyinyue3.mp3 alias BGM1", 0, 0, 0);
break;
case 4:mciSendString("open ./res/beijingyinyue4.mp3 alias BGM1", 0, 0, 0);
break;
case 5:mciSendString("open ./res/beijingyinyue5.mp3 alias BGM1", 0, 0, 0);
break;
case 6:mciSendString("open ./res/beijingyinyue6.mp3 alias BGM1", 0, 0, 0);
break;
case 7:mciSendString("open ./res/beijingyinyue7.mp3 alias BGM1", 0, 0, 0);
break;
}
mciSendString("play BGM1", 0, 0, 0);
}
void playPicture()
{
int x;
x = rand() % 8;
switch (x)
{
case 0:loadimage(&ph, "res\\0.png", 8*M, 4*M);
break;
case 1:loadimage(&ph, "res\\1.png", 8*M, 4*M);
break;
case 2:loadimage(&ph, "res\\2.png", 8*M, 4*M);
break;
case 3:loadimage(&ph, "res\\3.png", 8*M, 4*M);
break;
case 4:loadimage(&ph, "res\\4.png", 8*M, 4*M);
break;
case 5:loadimage(&ph, "res\\5.png", 8*M, 4*M);
break;
case 6:loadimage(&ph, "res\\6.png", 8*M, 4*M);
break;
case 7:loadimage(&ph, "res\\7.jpg", 8*M, 4*M);
break;
}
loadimage(&blank, "res\\blank.png", M, M);
}
int main()
{
srand((unsigned)time(NULL));
initgraph(8*M, 4*M);
mciSendString("open ./res/timi.mp3 alias BGM", 0, 0, 0);
mciSendString("play BGM", 0, 0, 0);
playPicture();
putimage(0, 0, &ph);
Sleep(3000);
playBgm();
initMap();
for (int i = 0; i<10000; i++)
{
machine();
}
drawMap();
palyGame();
while (1);
closegraph();
return 0;
}