中文液晶显示http协议网络读卡器是一款能利用现有的计算机网络,不需要独立布线就可以组成一个高性能低成本实时联网ID卡管理系统的端终设备,刷卡后即时向远程计算机传送卡号信息,电脑对刷卡信息处理后可即时向读卡器发送相应的显示及响应提示。HTTP网络读卡器已广泛用于计费,计件薪酬管理、人事考勤、打印监控、身份识别等场合。
设备特点
01、HTTP通讯协议,设备主动读取EM4100及兼容的低频RFID卡并即时将卡号发送到服务器;
02、122x32中文液晶双行显示,可显示任意中文、英文、数字及符号;
03、支持POE交换机网线供电;
04、支持WIFI无线热点连接;
05、支持两组继电器开关控制信号输出;
06、支持TTS中文合成语音播报;
07、公司自主研发、生产,性价比高,自主知识产权;
08、支持安卓Android系统、LINUX系统、WINDOWS系统开发使用。
09、支持x86、龙芯、兆芯、鲲鹏、麒麟、海光、飞腾、RK3288、imx6、全志、RK等CPU内核平台架构的设备使用。
HttpServer
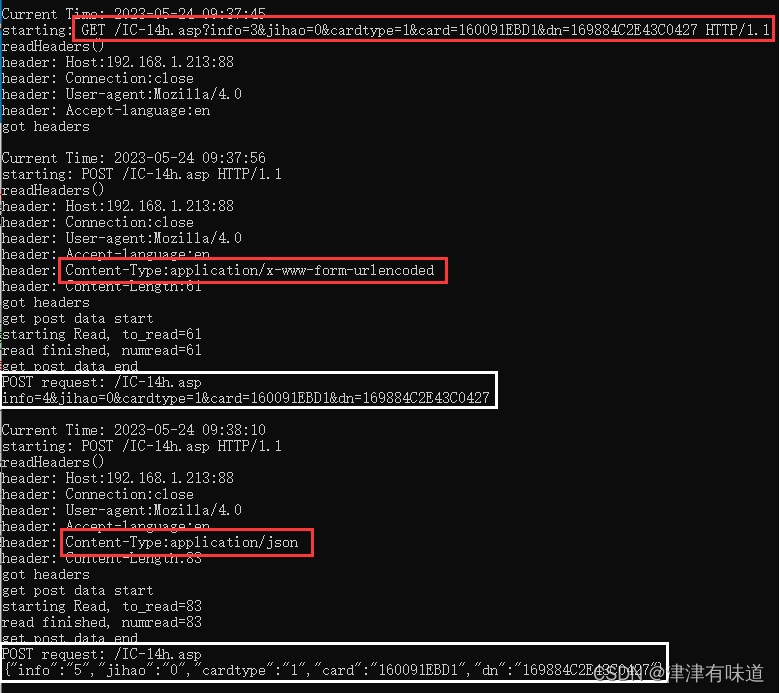
C#源码
using System;
using System.Collections;
using System.IO;
using System.Net;
using System.Net.Sockets;
using System.Threading;
using Newtonsoft.Json;
namespace Bend.Util
{
public class HttpProcessor
{
public TcpClient socket;
public HttpServer srv;
private Stream inputStream;
public StreamWriter outputStream;
public String http_method;
public String http_url;
public String http_protocol_versionstring;
public String http_content_type;
public Hashtable httpHeaders = new Hashtable();
private static int MAX_POST_SIZE = 10 * 1024 * 1024; // 10MB
public HttpProcessor(TcpClient s, HttpServer srv)
{
this.socket = s;
this.srv = srv;
}
private string streamReadLine(Stream inputStream)
{
int next_char;
string data = "";
while (true)
{
try
{
next_char = inputStream.ReadByte();
if (next_char == '\n') { break; }
if (next_char == '\r') { continue; }
if (next_char == -1) { Thread.Sleep(1); continue; };
data += Convert.ToChar(next_char);
}
catch
{
}
}
return data;
}
public void process()
{
inputStream = new BufferedStream(socket.GetStream());
outputStream = new StreamWriter(new BufferedStream(socket.GetStream()));
try
{
parseRequest();
readHeaders();
if (http_method.Equals("GET"))
{
handleGETRequest();
}
else if (http_method.Equals("POST"))
{
handlePOSTRequest();
}
outputStream.Flush();
}
catch (Exception e)
{
Console.WriteLine("Exception: " + e.ToString());
writeFailure();
}
inputStream = null; outputStream = null;
socket.Close();
}
public void parseRequest()
{
String request = streamReadLine(inputStream);
string[] tokens = request.Split(' ');
if (tokens.Length != 3)
{
throw new Exception("invalid http request line");
}
http_method = tokens[0].ToUpper();
http_url = tokens[1];
http_protocol_versionstring = tokens[2];
Console.WriteLine("Current Time: " + DateTime.Now.ToString());
Console.WriteLine("starting: " + request);
}
public void readHeaders()
{
try
{
Console.WriteLine("readHeaders()");
String line;
while ((line = streamReadLine(inputStream)) != null)
{
if (line.Equals(""))
{
Console.WriteLine("got headers");
return;
}
int separator = line.IndexOf(':');
if (separator == -1)
{
throw new Exception("invalid http header line: " + line);
}
String name = line.Substring(0, separator);
int pos = separator + 1;
while ((pos < line.Length) && (line[pos] == ' '))
{
pos++;
}
string value = line.Substring(pos, line.Length - pos);
Console.WriteLine("header: {0}:{1}", name, value);
httpHeaders[name] = value;
if (value == "application/x-www-form-urlencoded")
{
http_content_type = "application/x-www-form-urlencoded";
}
else if( value == "application/json"){
http_content_type = "application/json";
}
}
}
catch (Exception e)
{
Console.WriteLine("Exception: " + e.ToString());
}
}
public void handleGETRequest()
{
srv.handleGETRequest(this);
}
private const int BUF_SIZE = 4096;
public void handlePOSTRequest()
{
// this post data processing just reads everything into a memory stream. post上来的数据处理过程只是把所有东西都读入记忆流
// this is fine for smallish things, but for large stuff we should really 这对于小东西来说没问题,但是对于大的东西,我们真的应该把一个输入流交给请求处理器,然而,
// hand an input stream to the request processor. However, the input stream
// we hand him needs to let him see the "end of the stream" at this content 我们递给他的输入流需要让他看到这个内容长度的“流的结束”
// length, because otherwise he won't know when he's seen it all!
try
{
Console.WriteLine("get post data start");
int content_len = 0;
MemoryStream ms = new MemoryStream();
if (this.httpHeaders.ContainsKey("Content-Length"))
{
content_len = Convert.ToInt32(this.httpHeaders["Content-Length"]);
if (content_len > MAX_POST_SIZE)
{
throw new Exception(String.Format("POST Content-Length({0}) too big for this simple server",content_len));
}
byte[] buf = new byte[BUF_SIZE];
int to_read = content_len;
while (to_read > 0)
{
Console.WriteLine("starting Read, to_read={0}", to_read);
int numread = this.inputStream.Read(buf, 0, Math.Min(BUF_SIZE, to_read));
Console.WriteLine("read finished, numread={0}", numread);
if (numread == 0)
{
if (to_read == 0)
{
break;
}
else
{
throw new Exception("client disconnected during post");
}
}
to_read -= numread;
ms.Write(buf, 0, numread);
}
ms.Seek(0, SeekOrigin.Begin);
}
Console.WriteLine("get post data end");
srv.handlePOSTRequest(this, new StreamReader(ms), http_content_type);
}
catch (Exception e)
{
Console.WriteLine("Exception: " + e.ToString());
}
}
public void writeSuccess()
{
outputStream.WriteLine("HTTP/1.0 200 OK");
outputStream.WriteLine("Content-Type: text/html;charset=utf-8");
outputStream.WriteLine("Connection: close");
outputStream.WriteLine("");
}
public void writeFailure()
{
outputStream.WriteLine("HTTP/1.0 404 File not found");
outputStream.WriteLine("Connection: close");
outputStream.WriteLine("");
}
}
public abstract class HttpServer
{
protected int port;
TcpListener listener;
bool is_active = true;
public HttpServer(int port)
{
this.port = port;
}
public void listen()
{
listener = new TcpListener(port);
listener.Start();
while (is_active)
{
TcpClient s = listener.AcceptTcpClient();
HttpProcessor processor = new HttpProcessor(s, this);
Thread thread = new Thread(new ThreadStart(processor.process));
thread.Start();
Thread.Sleep(1);
}
}
public abstract void handleGETRequest(HttpProcessor p);
public abstract void handlePOSTRequest(HttpProcessor p, StreamReader inputData, string http_content_type);
}
public class MyHttpServer : HttpServer
{
public MyHttpServer(int port) : base(port)
{
}
public override void handleGETRequest(HttpProcessor p)
{
//Console.WriteLine("request: {0}", p.http_url);
string[] dataArray0 = p.http_url.Split(new char[2] { '?', '?' });
int t = dataArray0.GetUpperBound(0);
if (t > 0)
{
Console.WriteLine("\r");
string[] dataArray1 = dataArray0[1].Split(new char[2] { '&', '&' });
if (dataArray1.GetUpperBound(0) > 0)
{
string framenumber = "";
string cardnumber = "";
for (int i = 0; i < dataArray1.Length; i++)
{
string[] dataArray2 = dataArray1[i].Split(new char[2] { '=', '=' });
if (dataArray2[0] == "info") { framenumber = dataArray2[1]; } //接收到的数据包号,需回应该包号
if (dataArray2[0] == "jihao") { string jihao = dataArray2[1]; } //设备机号(可自编)
if (dataArray2[0] == "cardtype") { string cardtype = dataArray2[1]; } //卡类型有IC、ID、ISO15693等
if (dataArray2[0] == "card") { cardnumber = dataArray2[1]; } //接收到的原始16进制卡号,可根据需要自行转换成其他卡号
if (dataArray2[0] == "data") { string blockdata = dataArray2[1]; } //扇区内容
if (dataArray2[0] == "dn") { string Serialnumber = dataArray2[1]; } //设备硬件序列号,出厂时已固化,全球唯一
if (dataArray2[0] == "status") { string status = dataArray2[1]; } //读卡状态,如密码认证失败为12
}
string ResponseStr = "Response=1";
ResponseStr = ResponseStr + "," + framenumber;
ResponseStr = ResponseStr + "," + getChinesecode("卡号") + ":" + cardnumber + " " + DateTime.Now.ToString("yy-MM-dd HH:mm:ss"); //显示文字,注意中文汉字一定要转换为设备能显示的编码,其它字母数字符号不需要转换
ResponseStr = ResponseStr + ",20"; //显示时间,单位秒,最大取值255
ResponseStr = ResponseStr + ",2"; //蜂鸣响声代码,代码取值请参看文档说明
ResponseStr = ResponseStr + ",0"; //固定组合语音代码,代码取值请参看文档说明
p.outputStream.WriteLine(ResponseStr);
}
}
}
public override void handlePOSTRequest(HttpProcessor p, StreamReader inputData, string http_content_type)
{
Console.WriteLine("POST request: {0}", p.http_url);
string data = inputData.ReadToEnd();
if (data.Length > 0)
{
Console.WriteLine(data + "\r\n");
if (http_content_type == "application/x-www-form-urlencoded")
{
string[] dataArray1 = data.Split(new char[2] { '&', '&' });
if (dataArray1.GetUpperBound(0) > 0)
{
string framenumber="";
string cardnumber="";
for (int i = 0; i < dataArray1.Length; i++)
{
string[] dataArray2 = dataArray1[i].Split(new char[2] { '=', '=' });
if (dataArray2[0] == "info") { framenumber = dataArray2[1]; } //接收到的数据包号,需回应该包号
if (dataArray2[0] == "jihao") { string jihao = dataArray2[1]; } //设备机号(可自编)
if (dataArray2[0] == "cardtype") { string cardtype = dataArray2[1]; } //卡类型有IC、ID、ISO15693等
if (dataArray2[0] == "card") { cardnumber = dataArray2[1]; } //接收到的原始16进制卡号,可根据需要自行转换成其他卡号
if (dataArray2[0] == "data") { string blockdata = dataArray2[1]; } //扇区内容
if (dataArray2[0] == "dn") { string Serialnumber = dataArray2[1]; } //设备硬件序列号,出厂时已固化,全球唯一
if (dataArray2[0] == "status") { string status = dataArray2[1]; } //读卡状态,如密码认证失败为12
}
string ResponseStr = "Response=1";
ResponseStr = ResponseStr + "," + framenumber;
ResponseStr = ResponseStr + "," + getChinesecode("卡号") + ":" + cardnumber + " " + DateTime.Now.ToString("yy-MM-dd HH:mm:ss"); //显示文字,注意中文汉字一定要转换为设备能显示的编码,其它字母数字符号不需要转换
ResponseStr = ResponseStr + ",20"; //显示时间,单位秒,最大取值255
ResponseStr = ResponseStr + ",2"; //蜂鸣响声代码,代码取值请参看文档说明
ResponseStr = ResponseStr + ",0"; //固定组合语音代码,代码取值请参看文档说明
p.outputStream.WriteLine(ResponseStr);
}
}
else
{
RootObject rb = JsonConvert.DeserializeObject<RootObject>(data);
string framenumber = rb.info; //接收到的数据包号,需回应该包号
string jihao = rb.jihao; //设备机号(可自编)
string cardtype = rb.cardtype; //卡类型有IC、ID、ISO15693等
string cardnumber = rb.card; //接收到的原始16进制卡号,可根据需要自行转换成其他卡号
string blockdata = rb.data; //扇区内容
string Serialnumber = rb.dn; //设备硬件序列号,出厂时已固化,全球唯一
string status = rb.status; //读卡状态,如密码认证失败为12
string ResponseStr = "Response=1";
ResponseStr = ResponseStr + "," + framenumber;
ResponseStr = ResponseStr + "," + getChinesecode("卡号") + ":" + cardnumber + " " + DateTime.Now.ToString("yy-MM-dd HH:mm:ss"); //显示文字,注意中文汉字一定要转换为设备能显示的编码,其它字母数字符号不需要转换
ResponseStr = ResponseStr + ",20"; //显示时间,单位秒,最大取值255
ResponseStr = ResponseStr + ",2"; //蜂鸣响声代码,代码取值请参看文档说明
ResponseStr = ResponseStr + ",0"; //固定组合语音代码,代码取值请参看文档说明
p.outputStream.WriteLine(ResponseStr);
}
}
}
public static string getChinesecode(string inputip) //获取中文编码
{
byte[] Chinesecodearry = System.Text.Encoding.GetEncoding(936).GetBytes(inputip);
int codelen = (byte)Chinesecodearry.Length;
string hexcode = "";
for (int i = 0; i < codelen;i++ ){
if (i % 2 == 0) { hexcode = hexcode + "\\x"; }
hexcode = hexcode + Chinesecodearry[i].ToString("X2");
}
return hexcode;
}
public class RootObject
{
public string info { get; set; }
public string jihao { get; set; }
public string cardtype { get; set; }
public string card { get; set; }
public string data { get; set; }
public string dn { get; set; }
public string status { get; set; }
}
}
public class TestMain
{
public static int Main(String[] args)
{
Int16 listprot;
HttpServer httpServer;
if (args.GetLength(0) > 0)
{
listprot = Convert.ToInt16(args[0]);
}
else
{
listprot = 88;
}
httpServer = new MyHttpServer(listprot);
Thread thread = new Thread(new ThreadStart(httpServer.listen));
thread.Start();
Console.WriteLine("The system is listening on port " + Convert.ToString(listprot));
return 0;
}
}
}