1.完成四个默认成员函数
构造函数,拷贝构造,析构,赋值运算符重载
2.前置后置++和+/+=以及--/-/-=乘除的实现#ifndef __COMPLEX_H__
#define __COMPLEX_H__
#include <iostream>
using namespace std;
class Complex //定义Complex类
{
public:
//构造、析构、拷贝构造、赋值运算符重载
Complex(double real = 0.0, double image = 0.0)
:_real(real),
_image(image)
{}
~Complex()
{
cout << "~Complex" << endl;
}
Complex(Complex& c)
:_real(c._real)
, _image(c._image)
{}
Complex& operator=(Complex& c)
{
if (this != &c)
{
_real = c._real;
_image = c._image;
}
return *this;
}
//等于、不等于
bool operator==(const Complex& c)
{
if (_real == c._real && _image == c._image)
return true;
return false;
}
bool operator!=(const Complex& c)
{
if (_real != c._real || _image != c._image)
return true;
return false;
}
//自增自减
//前置
Complex& operator++()
{
_real++;
_image++;
return *this;
}
//后置
Complex operator++(int)
{
Complex ret = *this;
_real++, _image++;
return ret;
}
//前置
Complex& operator--()
{
_real--;
_image--;
return *this;
}
//后置
Complex operator--(int)
{
Complex ret = *this;
_real--, _image--;
return ret;
}
//+、+=、-、-=、*、*=、/、/=
Complex operator+(Complex& c)const
{
Complex ret(c);
ret._real += _real;
ret._image += _image;
return ret;
}
Complex& operator+=(const Complex& c)
{
_real += c._real;
_image += c._image;
return *this;
}
Complex operator-(Complex& c)const
{
Complex ret;
ret._real = _real - c._real;
ret._image = _image - c._image;
return ret;
}
Complex& operator-=(const Complex& c)
{
_real -= c._real;
_image -= c._image;
return *this;
}
Complex operator*(const Complex& c)const
{
Complex ret;
ret._real = _real*c._real - _image*c._image;
ret._image = _image*c._real + _real*c._image;
return ret;
}
Complex& operator*=(const Complex& c)
{
*this = (*this)*c;
return *this;
}
Complex operator/(const Complex&c)const
{
//= [(a + bi)(c - di)] / [(c + di)(c - di)] = [(ac + bd) + (bc - ad)i] / (c ^ 2 + d ^ 2).;
Complex ret;
ret._real = (_real*c._real + _image*c._image) / (c._real*c._real + c._image*c._image);
ret._image = (_image*c._real - _real*c._image) / (c._real*c._real + c._image*c._image);
return ret;
}
Complex& operator/=(const Complex&c)
{
*this = (*this) / c;
return *this;
}
//求模
double abs()
{
return sqrt(_real*_real + _image*_image);
}
//打印
void display()const
{
cout << "(" << _real << " + " << _image << "i" << ")" << endl;
}
private:
double _real;
double _image;
};
#endif //__COMPLEX_H__
int main()
{
Complex com1;
com1.display();
Complex com2(3, 5);
com2.display();
com1 = com2;
com1.display();
cout << (com1 == com2) << endl;
cout << (com1 != com2) << endl;
cout << com1.abs() << endl;
com1++.display();
(++com1).display();
cout << (com1 == com2) << endl;
cout << (com1 != com2) << endl;
com2--.display();
(--com2).display();
}
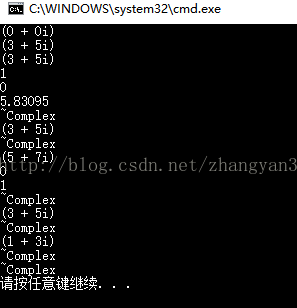
#ifndef __COMPLEX_H__
#define __COMPLEX_H__
#include <iostream>
using namespace std;
class Complex //定义Complex类
{
public:
//构造、析构、拷贝构造、赋值运算符重载
Complex(double real = 0.0, double image = 0.0)
:_real(real),
_image(image)
{}
~Complex()
{
cout << "~Complex" << endl;
}
Complex(Complex& c)
:_real(c._real)
, _image(c._image)
{}
Complex& operator=(Complex& c)
{
if (this != &c)
{
_real = c._real;
_image = c._image;
}
return *this;
}
//等于、不等于
bool operator==(const Complex& c)
{
if (_real == c._real && _image == c._image)
return true;
return false;
}
bool operator!=(const Complex& c)
{
if (_real != c._real || _image != c._image)
return true;
return false;
}
//自增自减
//前置
Complex& operator++()
{
_real++;
_image++;
return *this;
}
//后置
Complex operator++(int)
{
Complex ret = *this;
_real++, _image++;
return ret;
}
//前置
Complex& operator--()
{
_real--;
_image--;
return *this;
}
//后置
Complex operator--(int)
{
Complex ret = *this;
_real--, _image--;
return ret;
}
//+、+=、-、-=、*、*=、/、/=
Complex operator+(Complex& c)const
{
Complex ret(c);
ret._real += _real;
ret._image += _image;
return ret;
}
Complex& operator+=(const Complex& c)
{
_real += c._real;
_image += c._image;
return *this;
}
Complex operator-(Complex& c)const
{
Complex ret;
ret._real = _real - c._real;
ret._image = _image - c._image;
return ret;
}
Complex& operator-=(const Complex& c)
{
_real -= c._real;
_image -= c._image;
return *this;
}
Complex operator*(const Complex& c)const
{
Complex ret;
ret._real = _real*c._real - _image*c._image;
ret._image = _image*c._real + _real*c._image;
return ret;
}
Complex& operator*=(const Complex& c)
{
*this = (*this)*c;
return *this;
}
Complex operator/(const Complex&c)const
{
//= [(a + bi)(c - di)] / [(c + di)(c - di)] = [(ac + bd) + (bc - ad)i] / (c ^ 2 + d ^ 2).;
Complex ret;
ret._real = (_real*c._real + _image*c._image) / (c._real*c._real + c._image*c._image);
ret._image = (_image*c._real - _real*c._image) / (c._real*c._real + c._image*c._image);
return ret;
}
Complex& operator/=(const Complex&c)
{
*this = (*this) / c;
return *this;
}
//求模
double abs()
{
return sqrt(_real*_real + _image*_image);
}
//打印
void display()const
{
cout << "(" << _real << " + " << _image << "i" << ")" << endl;
}
private:
double _real;
double _image;
};
#endif //__COMPLEX_H__
int main()
{
Complex com1;
com1.display();
Complex com2(3, 5);
com2.display();
com1 = com2;
com1.display();
cout << (com1 == com2) << endl;
cout << (com1 != com2) << endl;
cout << com1.abs() << endl;
com1++.display();
(++com1).display();
cout << (com1 == com2) << endl;
cout << (com1 != com2) << endl;
com2--.display();
(--com2).display();
}
int main()
{
Complex com1(4,4);
Complex com2(2, 2);
Complex com3 = com1 + com2;
com3.display();
com3 += com2;
com3.display();
com3 -= com2;
com3.display();
com3 *= com2;
com3.display();
com3 /= com2;
com3.display();
}
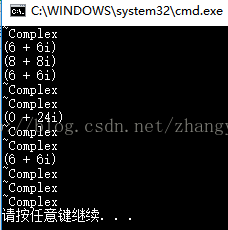
int main()
{
Complex com1(4,4);
Complex com2(2, 2);
Complex com3 = com1 + com2;
com3.display();
com3 += com2;
com3.display();
com3 -= com2;
com3.display();
com3 *= com2;
com3.display();
com3 /= com2;
com3.display();
}