上一篇写了Liferay源代码的编译和二次开发环境的生成(ext工程),下面将介绍在二次开发环境上搭建自己的开发框架.
用Eclipse打开编译Liferay源代码生成的ext扩展开发环境,如下图
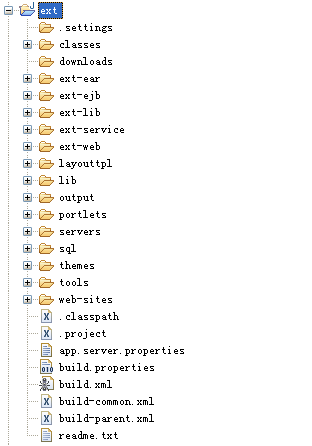
主要介绍ext-ejb和ext-web两个目录,利用Liferay的ant工具可以自动生成一套对于数据库表CURD的处理类,但是为符合自己的开发习惯,我在ext环境上搭建了自己的开发框架,主要包括两大块,第一是Hibernate数据访问层的封装,二是Struts的集成,集成了DispatchAction的多方法的功能.
下面介绍如何对Hibernate数据访问层进行封装ext-ejb/classes/META-INF 目录下面的ext-hbm.xml是Hibernate映射文件,ext-spring-professional.xml是Spring配置文件,对于ext-hbm.xml文件就不做解释了下面是Hibernate数据访问层的Spring的配置代码
<
bean id
=
"
cn.pisoft.portlet.hibernate.HibernateService.professional
"
class
=
"
cn.pisoft.portlet.hibernate.impl.HibernateServiceImpl
"
lazy
-
init
=
"
true
"
/>
<
bean id
=
"
cn.pisoft.portlet.hibernate.HibernateService.transaction
"
class
=
"
org.springframework.transaction.interceptor.TransactionProxyFactoryBean
"
lazy
-
init
=
"
true
"
>
<
property name
=
"
transactionManager
"
>
<
ref bean
=
"
liferayTransactionManager
"
/>
</
property
>
<
property name
=
"
target
"
>
<
ref bean
=
"
cn.pisoft.portlet.hibernate.HibernateService.professional
"
/>
</
property
>
<
property name
=
"
transactionAttributes
"
>
<
props
>
<
prop key
=
"
*
"
>
PROPAGATION_REQUIRED
</
prop
>
</
props
>
</
property
>
</
bean
>
<
bean id
=
"
cn.pisoft.portlet.hibernate.factory.HibernateServiceFactory
"
class
=
"
cn.pisoft.portlet.hibernate.factory.HibernateServiceFactory
"
lazy
-
init
=
"
true
"
>
<
property name
=
"
service
"
>
<
ref bean
=
"
cn.pisoft.portlet.hibernate.HibernateService.transaction
"
/>
</
property
>
</
bean
>
<
bean id
=
"
cn.pisoft.portlet.hibernate.impl.HibernateDaoImpl
"
class
=
"
cn.pisoft.portlet.hibernate.impl.HibernateDaoImpl
"
lazy
-
init
=
"
true
"
>
<
property name
=
"
dataSource
"
>
<
ref bean
=
"
liferayDataSource
"
/>
</
property
>
<
property name
=
"
sessionFactory
"
>
<
ref bean
=
"
liferaySessionFactory
"
/>
</
property
>
</
bean
>
<
bean id
=
"
cn.pisoft.portlet.hibernate.util.HibernateDaoUtil
"
class
=
"
cn.pisoft.portlet.hibernate.util.HibernateDaoUtil
"
lazy
-
init
=
"
true
"
>
<
property name
=
"
dao
"
>
<
ref bean
=
"
cn.pisoft.portlet.hibernate.impl.HibernateDaoImpl
"
/>
</
property
>
</
bean
>
下面是相关的JAVA类和接口
HibernateDao.java 接口
package
cn.pisoft.portlet.hibernate;

import
java.io.Serializable;
import
java.util.List;

import
cn.pisoft.portlet.common.Pager;





/** */
/**
* <p>Title:</p>
*
* <p>Description:</p>
*
* <p>Copyright: Copyright (c) 2007</p>
*
* <p>Company: </p>
*
* <p>Author: 一个人的日子</p>
*
* <p>Email: zhjb2000@hotmail.com</p>
*
* <p>Version: 1.0</p>
*
* <p>Create 2007-7-29</p>
*
*/


public
interface
HibernateDao
...
{
public void save(Object po) throws Exception ;
public List query(String hql)throws Exception ;
public Object get(Class clas ,Serializable id)throws Exception;
public void update(Object po) throws Exception ;
public void delete(Object po) throws Exception ;
public Pager queryByPage(String hql,int totalElements,int pageNumber,int pageSize) throws Exception;
public Pager queryByPage(String hql,int pageNumber,int pageSize) throws Exception;
public void executeSql(String sql)throws Exception ;
public int getCountByExecuteSql(String sql)throws Exception ;
public List getTopN(String hql,int n)throws Exception ;
public List getListByExecuteSql(String sq,String columnNamel)throws Exception;
public List getListByExecuteSql(String sq, int fetchNubmer)throws Exception;
}
HibernateDaoImpl.java 上面接口的实现类
package
cn.pisoft.portlet.hibernate.impl;

import
java.io.Serializable;
import
java.sql.Connection;
import
java.sql.ResultSet;
import
java.sql.Statement;
import
java.util.ArrayList;
import
java.util.List;

import
org.hibernate.Query;
import
org.hibernate.Session;

import
cn.pisoft.portlet.common.Pager;
import
cn.pisoft.portlet.hibernate.HibernateDao;

import
com.liferay.portal.service.persistence.BasePersistence;



/** */
/**
* <p>Title:</p>
*
* <p>Description:</p>
*
* <p>Copyright: Copyright (c) 2007</p>
*
* <p>Company: </p>
*
* <p>Author: 一个人的日子</p>
*
* <p>Email: zhjb2000@hotmail.com</p>
*
* <p>Version: 1.0</p>
*
* <p>Create 2007-7-29</p>
*
*/


public
class
HibernateDaoImpl
extends
BasePersistence
implements
HibernateDao
...
{


public List query(String hql)throws Exception ...{
List list=null;
Session session = null;
session=this.openSession();
list=session.createQuery(hql).list();
this.closeSession(session);
return list;
}


public void save(Object po) throws Exception ...{
Session session = null;
session=this.openSession();
session.save(po);
this.closeSession(session);
}


public Object get(Class clas, Serializable id) throws Exception ...{
Session session = null;
session=this.openSession();
Object o=session.get(clas, id);
this.closeSession(session);
return o;
}


public void update(Object po) throws Exception ...{
Session session = null;
session=this.openSession();
session.update(po);
this.closeSession(session);
}


public void delete(Object po) throws Exception ...{
Session session = null;
session=this.openSession();
session.delete(po);
this.closeSession(session);
}


public Pager queryByPage(String hql, int totalElements, int pageNumber, int pageSize) throws Exception ...{
Session session = null;
session=this.openSession();
Pager page=new Pager();
page.setPageNumber(pageNumber);
page.setPageSize(pageSize);
page.setTotalElements(totalElements);
List list=null;
Query query=session.createQuery(hql);
query.setFirstResult((pageNumber - 1) * pageSize);
query.setMaxResults(pageSize);
list=query.list();
page.setElements(list);
this.closeSession(session);
return page;
}

public Pager queryByPage(String hql, int pageNumber, int pageSize) throws Exception ...{
Session session = null;
session=this.openSession();
Pager page=new Pager();
page.setPageNumber(pageNumber);
page.setPageSize(pageSize);
int totalElements = 0;
String staticSql = hql.trim();

if(staticSql.startsWith("select") || staticSql.startsWith("SELECT"))...{
int fromIndex = staticSql.indexOf("from");
int FROMIndex = staticSql.indexOf("FROM");

if(FROMIndex != -1 && fromIndex > FROMIndex)...{
fromIndex = FROMIndex;
}

if(fromIndex == -1)...{
fromIndex = 0;
}
staticSql = staticSql.substring(fromIndex);
}
int orderByIndex = staticSql.indexOf("order by");

if( orderByIndex != -1)...{
staticSql = staticSql.substring(0,orderByIndex);
}
Query query = session.createQuery("select count(*) " + staticSql);
List list = query.list();

if(list!=null && list.get(0)!=null)...{
totalElements=((Long)list.get(0)).intValue();
}
page.setTotalElements(totalElements);
query = session.createQuery(hql);
query.setFirstResult((pageNumber - 1) * pageSize);
query.setMaxResults(pageSize);
list = query.list();
page.setElements(list);
this.closeSession(session);
return page;
}


public void executeSql(String sql) throws Exception ...{
Session session = null;
session=this.openSession();
Connection con= session.connection();
con.createStatement().execute(sql);
con.close();
this.closeSession(session);
}

public List getTopN(String hql, int n) throws Exception ...{
Session session = null;
session=this.openSession();
Query query=session.createQuery(hql);
query.setMaxResults(n);
List list=query.list();
this.closeSession(session);
return list;
}


public int getCountByExecuteSql(String sql) throws Exception ...{
Session session = null;
session=this.openSession();
Connection con= session.connection();
Statement stmt = con.createStatement(ResultSet.TYPE_FORWARD_ONLY,ResultSet.CONCUR_READ_ONLY);
ResultSet rs = stmt.executeQuery(sql);
int returnNumber =0;

while (rs.next())...{
returnNumber = rs.getInt("count");
break;
}
this.closeSession(session);
return returnNumber;
}


public List getListByExecuteSql(String sql,String columnName ) throws Exception ...{
Session session = null;
session=this.openSession();
Connection con= session.connection();
Statement stmt = con.createStatement(ResultSet.TYPE_FORWARD_ONLY,ResultSet.CONCUR_READ_ONLY);
ResultSet rs = stmt.executeQuery(sql);
List<String> list = new ArrayList<String>();

while(rs.next())...{
list.add(rs.getString(columnName));
}
this.closeSession(session);
return list;
}



public List<Object[]> getListByExecuteSql(String sql,int fetchNubmer) throws Exception ...{
Session session = null;
session=this.openSession();
Connection con= session.connection();
Statement stmt = con.createStatement(ResultSet.TYPE_FORWARD_ONLY,ResultSet.CONCUR_READ_ONLY);
ResultSet rs = stmt.executeQuery(sql);
List<Object[]> list = new ArrayList<Object[]>();
Object [] objs = null;

while(rs.next())...{
objs = new Object[fetchNubmer];

for(int i=0;i<fetchNubmer;i++)...{
objs[i] = rs.getObject(i+1);
}
list.add(objs);
}
this.closeSession(session);
return list;
}

}
HibernateService.java 接口
package
cn.pisoft.portlet.hibernate;

import
java.io.Serializable;
import
java.util.List;

import
cn.pisoft.portlet.common.Pager;





/** */
/**
* <p>Title:</p>
*
* <p>Description:</p>
*
* <p>Copyright: Copyright (c) 2007</p>
*
* <p>Company: </p>
*
* <p>Author: 一个人的日子</p>
*
* <p>Email: zhjb2000@hotmail.com</p>
*
* <p>Version: 1.0</p>
*
* <p>Create 2007-7-29</p>
*
*/


public
interface
HibernateService
...
{
public void save(Object po) throws Exception ;
public List query(String hql)throws Exception ;
public Object get(Class clas ,Serializable id)throws Exception;
public void update(Object po) throws Exception ;
public void delete(Object po) throws Exception ;
public Pager queryByPage(String hql,int totalElements,int pageNumber,int pageSize) throws Exception;
public Pager queryByPage(String hql,int pageNumber,int pageSize) throws Exception;
public void executeSql(String sql)throws Exception ;
public int getCountByExecuteSql(String sql)throws Exception ;
public List getTopN(String hql,int n)throws Exception ;
public List getListByExecuteSql(String sql,String columnName)throws Exception;
public List getListByExecuteSql(String sql, int fetchNubmer)throws Exception;
}
HibernateServiceImpl.java 上面接口的实现类
package
cn.pisoft.portlet.hibernate.impl;

import
java.io.Serializable;
import
java.util.List;

import
cn.pisoft.portlet.common.Pager;
import
cn.pisoft.portlet.hibernate.HibernateService;
import
cn.pisoft.portlet.hibernate.util.HibernateDaoUtil;





/** */
/**
* <p>Title:</p>
*
* <p>Description:</p>
*
* <p>Copyright: Copyright (c) 2007</p>
*
* <p>Company: </p>
*
* <p>Author: 一个人的日子</p>
*
* <p>Email: zhjb2000@hotmail.com</p>
*
* <p>Version: 1.0</p>
*
* <p>Create 2007-7-29</p>
*
*/


public
class
HibernateServiceImpl
implements
HibernateService
...
{


public List query(String hql) throws Exception ...{
return HibernateDaoUtil.query(hql);
}


public void save(Object po) throws Exception ...{
HibernateDaoUtil.save(po);
}


public Object get(Class clas, Serializable id) throws Exception ...{
return HibernateDaoUtil.get(clas, id);
}


public void update(Object po) throws Exception ...{
HibernateDaoUtil.update(po);
}


public void delete(Object po) throws Exception ...{
HibernateDaoUtil.delete(po);
}


public Pager queryByPage(String hql, int totalElements, int pageNumber, int pageSize) throws Exception ...{
return HibernateDaoUtil.queryByPage(hql, totalElements, pageNumber, pageSize);
}

public Pager queryByPage(String hql, int pageNumber, int pageSize) throws Exception ...{
return HibernateDaoUtil.queryByPage(hql, pageNumber, pageSize);
}


public void executeSql(String sql) throws Exception ...{
HibernateDaoUtil.executeSql(sql);
}


public List getTopN(String hql, int n) throws Exception ...{
return HibernateDaoUtil.getTopN(hql,n);
}


public int getCountByExecuteSql(String sql) throws Exception ...{
// TODO Auto-generated method stub
return HibernateDaoUtil.getCountByExecuteSql(sql);
}

public List getListByExecuteSql(String sql,String columnName)throws Exception...{
return HibernateDaoUtil.getListByExecuteSql(sql,columnName);
}

public List getListByExecuteSql(String sql, int fetchNubmer)throws Exception...{
return HibernateDaoUtil.getListByExecuteSql(sql,fetchNubmer);
}

}
HibernateDaoUtil.java
package
cn.pisoft.portlet.hibernate.util;

import
java.io.Serializable;
import
java.util.List;

import
cn.pisoft.portlet.common.Pager;
import
cn.pisoft.portlet.hibernate.HibernateDao;




/** */
/**
* <p>Title:</p>
*
* <p>Description:</p>
*
* <p>Copyright: Copyright (c) 2007</p>
*
* <p>Company: </p>
*
* <p>Author: 一个人的日子</p>
*
* <p>Email: zhjb2000@hotmail.com</p>
*
* <p>Version: 1.0</p>
*
* <p>Create 2007-7-29</p>
*
*/


public
class
HibernateDaoUtil
...
{
private static final String _UTIL = HibernateDaoUtil.class.getName();
private static HibernateDaoUtil _util;
private HibernateDao dao;

public static void save(Object po) throws Exception ...{
getDao().save(po);
}

public static List query(String hql)throws Exception ...{
return getDao().query(hql);
}

public static Object get(Class clas ,Serializable id)throws Exception...{
return getDao().get(clas, id);
}

public static void update(Object po) throws Exception ...{
getDao().update(po);
}

public static void delete(Object po) throws Exception ...{
getDao().delete(po);
}

public static Pager queryByPage(String hql,int totalElements,int pageNumber,int pageSize) throws Exception ...{
return getDao().queryByPage(hql, totalElements, pageNumber, pageSize);
}

public static Pager queryByPage(String hql,int pageNumber,int pageSize) throws Exception ...{
return getDao().queryByPage(hql, pageNumber, pageSize);
}

public static void executeSql(String sql)throws Exception ...{
getDao().executeSql(sql);
}

public static List getListByExecuteSql(String sql,String columnName)throws Exception...{
return getDao().getListByExecuteSql(sql,columnName);
}

public static List getListByExecuteSql(String sql, int fetchNubmer)throws Exception...{
return getDao().getListByExecuteSql(sql,fetchNubmer);
}

public static int getCountByExecuteSql(String sql) throws Exception...{
return getDao().getCountByExecuteSql(sql);
}

public static List getTopN(String hql,int n)throws Exception ...{
return getDao().getTopN(hql, n);
}

public static HibernateDao getDao() ...{
return _getUtil().dao;
}


public void setDao(HibernateDao dao) ...{
this.dao = dao;
}


private static HibernateDaoUtil _getUtil() ...{

if (_util == null) ...{
_util = (HibernateDaoUtil)com.liferay.portal.kernel.bean.BeanLocatorUtil.locate(_UTIL);
}
return _util;
}
}