所需工具 composer2:官网下载下来安装即可
2.所需依赖 在thinkphp5的项目根目录下运行安装命令:
composer require phpoffice/phpword
然后再package.json的require中即可看到:"phpoffice/phpword": "^1.0"
3.参考网站 phpword的官方手册:https://phpword.readthedocs.io/en/latest/templates-processing.html#setimagevalue 4.准备测试模板.word,如下图所示,分为三个区域,普通文字区域、表格区域、图片区域用${}来标识
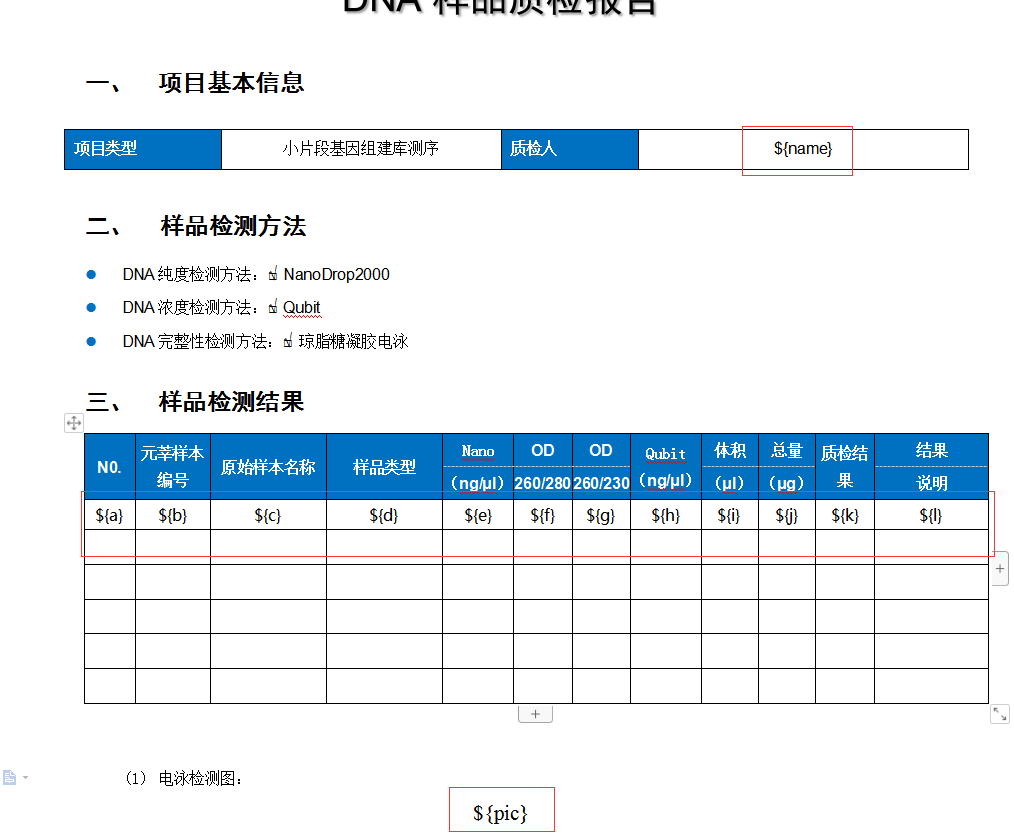
5.封装phpword测试代码:
<?php
namespace Custom;
use PhpOffice\PhpWord\IOFactory;
use PhpOffice\PhpWord\PhpWord;
use PhpOffice\PhpWord\TemplateProcessor;
class Pword
{
private $_save_path;
/**
* 初始化构造函数
*
**/
public function __construct($params = array())
{
if (!empty($params['save_path'])) {
$this->_save_path = $params['save_path'];
}
}
public function generateWordByTemplate($template_path, $params)
{
try {
$phpword = new PhpWord();
$document = new TemplateProcessor($template_path);
$document->setValue('name', $params['name']);
$replacements = array(
array('a' => 'Batman', 'b' => 'Gotham City', 'c' => 'c', 'd' => 'd', 'e' => 'e', 'f' => 'f', 'g' => 'g', 'h' => 'h', 'i' => 'i', 'j' => 'j', 'k' => 'k', 'l' => 'l'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
array('a' => 'Superman1', 'b' => 'Metropolis', 'c' => 'cc', 'd' => 'dd', 'e' => 'ee', 'f' => 'ff', 'g' => 'gg', 'h' => 'hh', 'i' => 'ii', 'j' => 'jj', 'k' => 'kk', 'l' => 'll'),
);
//$document->cloneBlock('blockname', 0, true, false, $replacements);
$document->cloneRowAndSetValues('a', $replacements);
$document->setImageValue('pic', $params['img']);
$document->saveAs($this->_save_path);
} catch (\Exception $e) {
throw new \Exception($e->getMessage());
}
}
}
调用Pword代码:
public function test_generate()
{
try {
$root_path = $_SERVER['DOCUMENT_ROOT'];
$relative_path = '/static/download/files/test_generate/'.date('Ymd').'/';
if (!is_dir($root_path.$relative_path)) {
mkdir($root_path.$relative_path, 0777, true);
}
$relative_path .= 'report_test_'.date('Ymd').'.docx';
$word = new \Custom\Pword(['save_path' => $root_path.$relative_path]);
$image_path = $root_path.'/static/upload/images/extract/20221121/f1ce8b962b50d49251e935cd2678f7dd.png';
$image_size = getimagesize($image_path);
//表格的数据库直接放在了Pword中进行了测试,后续真中用的时候需要在逻辑层进行传参
//插入的图片进行了宽度的约束,防止图片插入过大而显示不全,高度会自动缩放,所以不用指定
$word->generateWordByTemplate($root_path.'/static/dna_template.docx',
['name' => '测试123',
'img' => [ 'path' => $image_path,'width'=> $image_size[0] < 600 ? $image_size[0] : 600, 'height' => $image_size[1]],
]
);
echo $relative_path;
} catch (\Exception $e) {
throw new \Exception($e->getMessage());
}
}
6.最终的生成word文档为,截图两部分,图片为黑色的,请见谅:
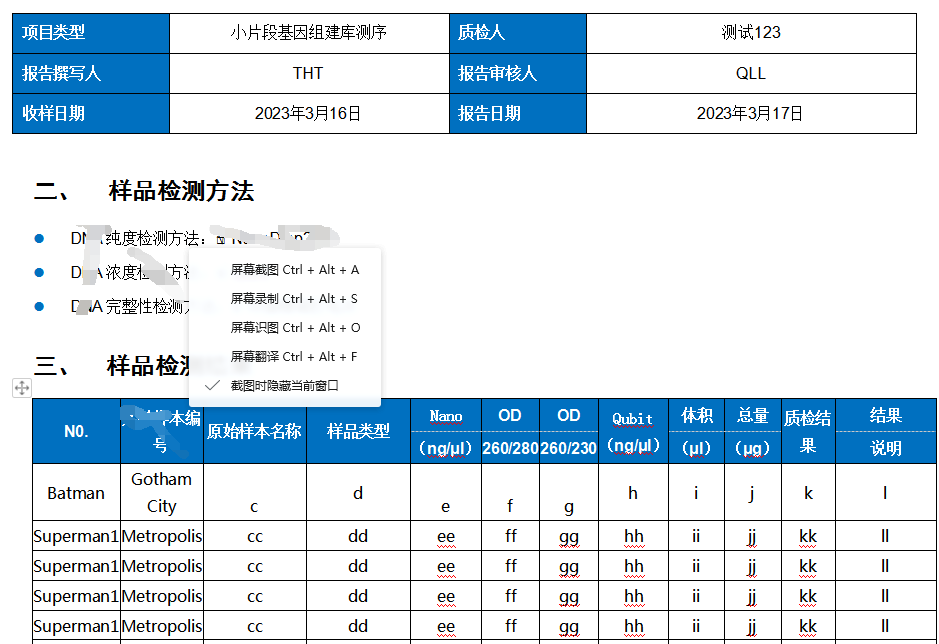
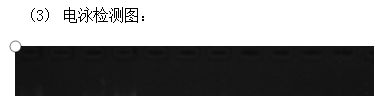
如果在安装phpword中存在问题,可以看我相关文章查看