二叉树:
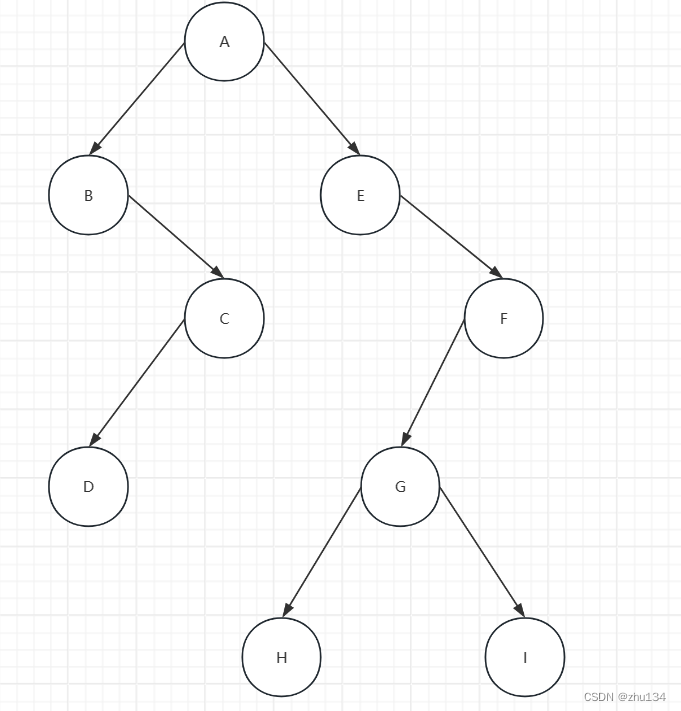
代码:
#include <iostream>
#include <vector>
using namespace std;
// 树节点结构体
struct TreeNode {
char data; // 节点数据
int parentIndex; // 父节点在数组中的下标
};
// 父亲数组表示法
void parentArrayRepresentation(TreeNode* tree[], int n) {
cout << "父亲数组表示法:" << endl;
cout << "节点数据 父节点下标" << endl;
for (int i = 0; i < n; i++) {
cout << tree[i]->data << " " << tree[i]->parentIndex << endl;
}
cout << endl;
}
// 子表表示法
void childListRepresentation(vector<vector<int>>& childList) {
cout << "子表表示法:" << endl;
cout << "节点数据 子节点列表" << endl;
for (int i = 0; i < childList.size(); i++) {
cout << char('A' + i) << " ";
for (int j = 0; j < childList[i].size(); j++) {
cout << char('A' + childList[i][j]) << " ";
}
cout << endl;
}
cout << endl;
}
// 长子-兄弟表示法
void childSiblingRepresentation(TreeNode* tree[], int n) {
cout << "长子-兄弟表示法:" << endl;
cout << "节点数据 第一个子节点 兄弟节点" << endl;
for (int i = 0; i < n; i++) {
cout << tree[i]->data << " ";
int firstChild = -1;
// 寻找当前节点的第一个子节点
for (int j = 0; j < n; j++) {
if (tree[j]->parentIndex == i) {
firstChild = j; // 找到第一个子节点
break; // 找到第一个子节点后,停止寻找
}
}
if (firstChild != -1) { // 如果当前节点有子节点
cout << firstChild << " ";
int sibling = firstChild;
while (sibling < n) {
// 如果找到下一个兄弟节点,则输出兄弟节点的索引
if (tree[sibling]->parentIndex == tree[firstChild]->parentIndex && sibling != firstChild) {
cout << sibling << " ";
}
sibling++; // 继续查找下一个节点
}
}
cout << endl;
}
cout << endl;
}
// 初始化树
void initBTree(TreeNode* tree[], int n) {
tree[0] = new TreeNode{'A', -1};
tree[1] = new TreeNode{'B', 0};
tree[2] = new TreeNode{'C', 0};
tree[3] = new TreeNode{'D', 2};
tree[4] = new TreeNode{'E', 0};
tree[5] = new TreeNode{'F', 4};
tree[6] = new TreeNode{'G', 5};
tree[7] = new TreeNode{'H', 6};
tree[8] = new TreeNode{'I', 6};
}
// 父亲数组表示法
void parentArrayMethod() {
TreeNode* tree[9];
initBTree(tree, 9);
parentArrayRepresentation(tree, 9);
}
// 子表表示法
void childListMethod() {
vector<vector<int>> childList(9);
childList[0].push_back(1);
childList[0].push_back(4);
childList[1].push_back(2);
childList[2].push_back(3);
childList[4].push_back(5);
childList[5].push_back(6);
childList[6].push_back(7);
childList[6].push_back(8);
childListRepresentation(childList);
}
// 长子-兄弟表示法
void childSiblingMethod() {
TreeNode* tree[9];
initBTree(tree, 9);
childSiblingRepresentation(tree, 9);
}
int main() {
parentArrayMethod();
childListMethod();
childSiblingMethod();
return 0;
}
运行结果:
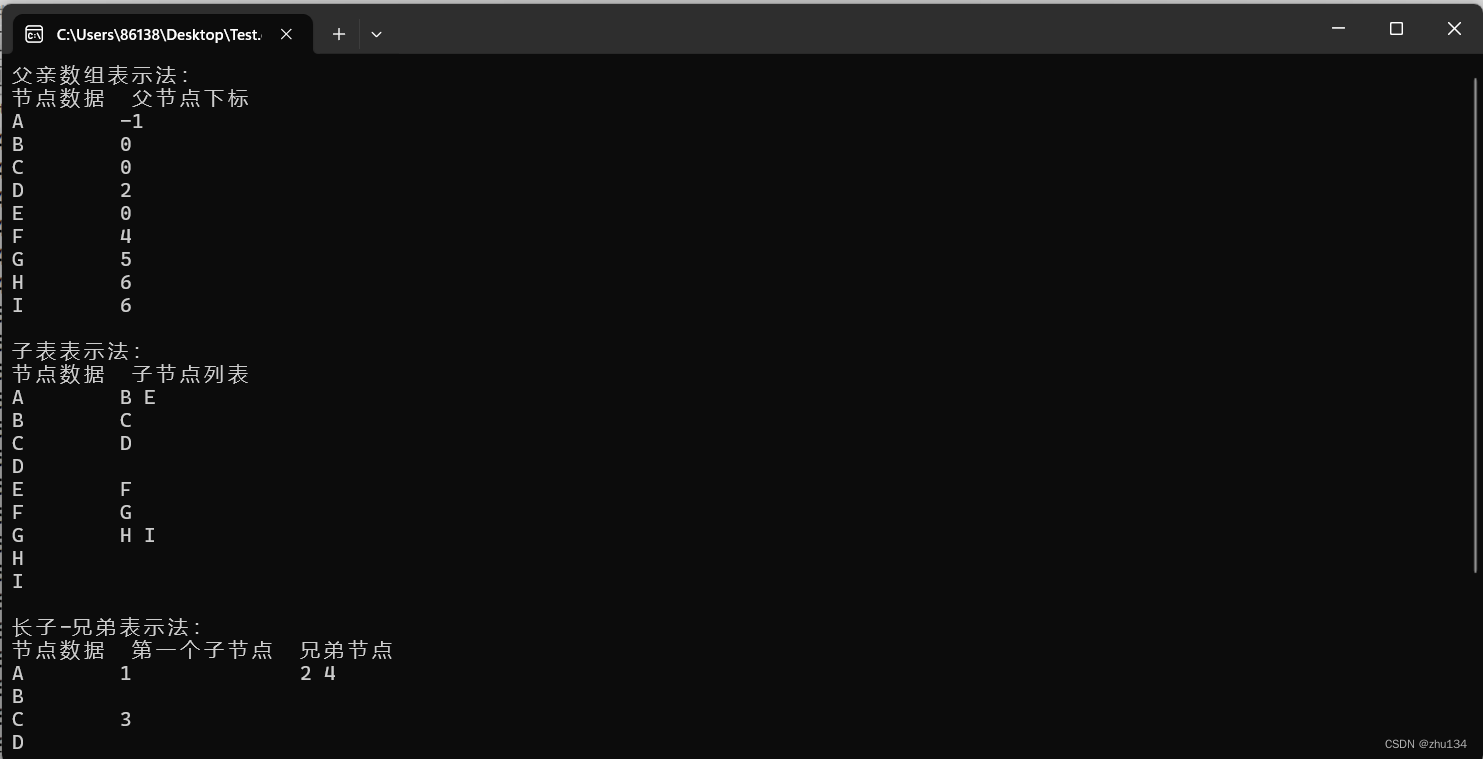
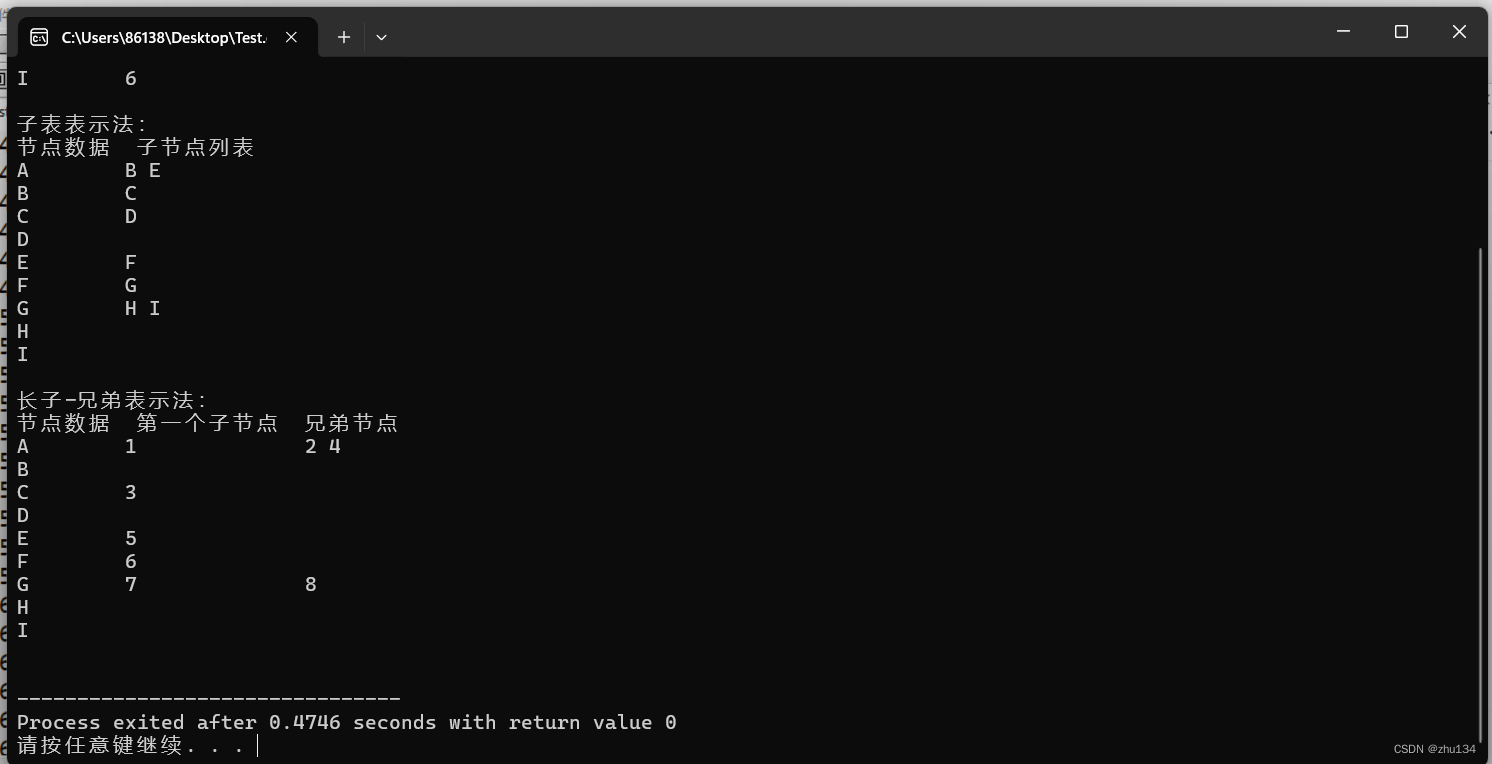