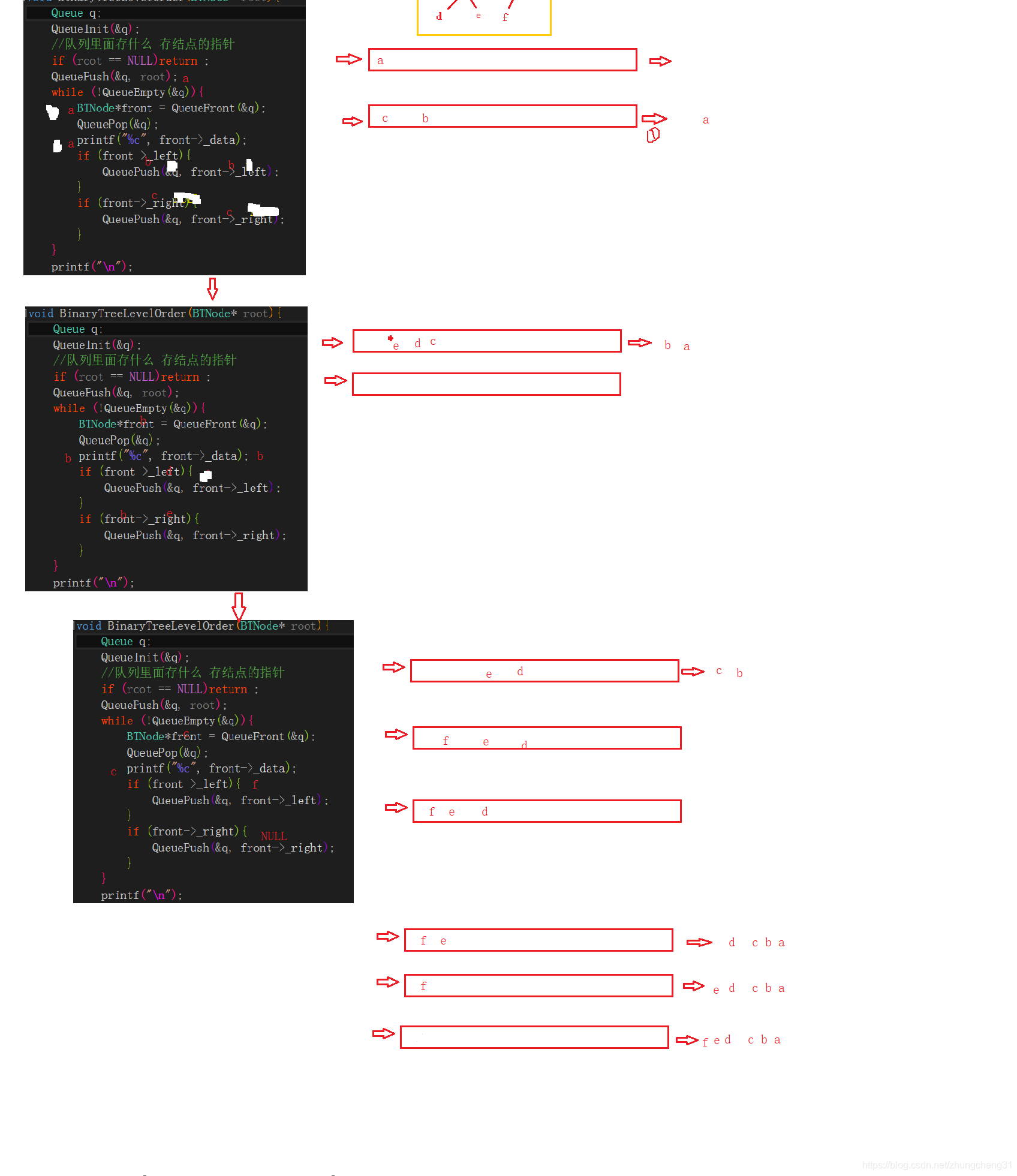
BTree.h 头文件
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
typedef char BTDataType;
typedef struct BinaryTreeNode
{
BTDataType data;
struct BinaryTreeNode* left;
struct BinaryTreeNode* right;
}BTNode;
BTNode* BinaryTreeCreate(BTDataType* a, int* pi);
void BinaryTreeDestory(BTNode* root);
int BinaryTreeSize(BTNode* root);
int BinaryTreeLeafSize(BTNode* root);
int BinaryTreeLevelKSize(BTNode* root, int k);
BTNode* BinaryTreeFind(BTNode* root, BTDataType x);
void BinaryTreePrevOrder(BTNode* root);
void BinaryTreeInOrder(BTNode* root);
void BinaryTreePostOrder(BTNode* root);
typedef struct QueueNode{
BTNode* data;
struct QueueNode* next;
}QueueNode;
typedef struct QueueList{
QueueNode*_front;
QueueNode*_tail;
}Queue;
BTNode* QueueFront(Queue*q);
void BinaryTreeLevelOrder(BTNode* root);
int BinaryTreeComplete(BTNode* root);
BTree.c
#include"BTree.h"
BTNode* BinaryTreeCreate(BTDataType* a, int* pi){
if (a[*pi] == '#'){
++(*pi);
return NULL;
}
BTNode*root = (BTNode*)malloc(sizeof(BTNode));
root->data = a[*pi];
++(*pi);
root->left = BinaryTreeCreate(a, pi);
root->right = BinaryTreeCreate(a, pi);
return root;
}
void BinaryTreeDestory(BTNode* root){
if (root == NULL)return;
BinaryTreeDestory(root->left);
BinaryTreeDestory(root->right);
free(root);
}
int BinaryTreeSize(BTNode* root){
if (root == NULL)return 0;
return 1 + BinaryTreeSize(root->left) + BinaryTreeSize(root->right);
}
int BinaryTreeLeafSize(BTNode* root){
if (root == NULL)return 0;
if (root->left == NULL&& root->right == NULL)return 1;
return BinaryTreeLeafSize(root->left) + BinaryTreeLeafSize(root->right);
}
int BinaryTreeLevelKSize(BTNode* root, int k){
if (root == NULL)return 0;
if (k == 1)return 1;
return BinaryTreeLevelKSize(root->left, k - 1)+BinaryTreeLevelKSize(root->right,k-1);
}
BTNode* BinaryTreeFind(BTNode* root, BTDataType x){
if (root == NULL)return NULL;
if (root->data == x)return root;
BTNode*node = BinaryTreeFind(root->left, x);
if (node)return node;
node = BinaryTreeFind(root->right, x);
if (node)return node;
return NULL;
}
void BinaryTreePrevOrder(BTNode* root){
if (root == NULL)return;
printf("前序遍历:%c ", root->data);
BinaryTreePrevOrder(root->left);
BinaryTreePrevOrder(root->right);
}
void BinaryTreeInOrder(BTNode* root){
if (root == NULL)return;
BinaryTreeInOrder(root->left);
printf("中序遍历:%c ", root->data);
BinaryTreeInOrder(root->right);
}
void BinaryTreePostOrder(BTNode* root){
if (root == NULL)return;
BinaryTreeInOrder(root->left);
BinaryTreeInOrder(root->right);
printf("后序遍历:%c ", root->data);
}
void QueueInit(Queue*q){
assert(q);
q->_front = NULL;
q->_tail = NULL;
}
void QueuePush(Queue*q, BTNode*data){
assert(q);
QueueNode*newNode = (QueueNode*)malloc(sizeof(QueueNode));
if (newNode == NULL){
printf("内存不足!");
exit(-1);
}
newNode->data = data;
newNode->next = NULL;
if (q->_front == NULL)q->_front = q->_tail = newNode;
else{
q->_tail->next = newNode;
q->_tail = newNode;
}
}
int QueueEmpty(Queue*q){
assert(q);
if (q->_front == q->_tail&&q->_front==NULL)return 1;
else return 0;
}
void QueuePop(Queue*q){
assert(q);
assert(q->_front);
QueueNode*next = q->_front->next;
free(q->_front);
q->_front = next;
if (next == NULL){
q->_tail = NULL;
}
}
BTNode* QueueFront(Queue*q){
assert(q);
assert(q->_front);
return q->_front->data;
}
void BinaryTreeLevelOrder(BTNode* root){
Queue q;
QueueInit(&q);
if (root == NULL)return;
QueuePush(&q, root);
while (!QueueEmpty(&q)){
BTNode*front= QueueFront(&q);
printf("%c ", front->data);
QueuePop(&q);
if (front->left) QueuePush(&q, front->left);
if (front->right)QueuePush(&q, front->right);
}
printf("\n");
}
int BinaryTreeComplete(BTNode* root){
Queue q;
QueueInit(&q);
if (root == NULL)return 1;
QueuePush(&q,root);
while (!QueueEmpty(&q)){
BTNode*front = QueueFront(&q);
if (front == NULL)break;
QueuePop(&q);
QueuePush(&q, front->left);
QueuePush(&q, front->right);
}
while (!QueueEmpty(&q)){
BTNode*front = QueueFront(&q);
if (front)return 0;
QueuePop(&q);
}
return 1;
}
test.c
#include"BTree.h"
void PreOrder(BTNode*root){
if (root == NULL)return ;
printf("%c ", root->data);
PreOrder(root->left);
PreOrder(root->right);
}
void TestBinaryTreeCreate(){
char str[] = { 'a', 'b', 'c', '#', '#', 'd', 'e', '#', 'g', '#', '#', 'f', '#', '#', '#' };
int i = 0;
BTNode*root = BinaryTreeCreate(str, &i);
int size=BinaryTreeLeafSize(root);
printf("叶子结点个数:%d\n ", size);
int nums=BinaryTreeLevelKSize(root, 4);
printf("第k层结点个数:%d\n", nums);
BTNode*ret= BinaryTreeFind(root, 'g');
if (ret == NULL) printf("没有该结点!");
else printf("找到该结点,是:%c\n", ret->data);
BinaryTreeLevelOrder(root);
int isBTC=BinaryTreeComplete(root);
printf("是否是完全二叉树:%d ", isBTC);
}
int main(){
TestBinaryTreeCreate();
system("pause");
return 0;
}
复习版本
BTree.h
#include<iostream>
#include<assert.h>
typedef char DataType;
typedef struct BinaryTreeNode
{
DataType _data;
struct BinaryTreeNode* _left;
struct BinaryTreeNode* _right;
}BTNode;
BTNode* BinaryTreeCreate(DataType* a, int *pi)
{
if (a[*pi] == '#')
{
++(*pi);
return NULL;
}
BTNode* root = (BTNode*)malloc(sizeof(BTNode));
root->_data = a[*pi];
++(*pi);
root->_left = BinaryTreeCreate(a, pi);
root->_right = BinaryTreeCreate(a, pi);
return root;
}
void PrevOrder(BTNode*root)
{
if (root == NULL)
{
printf("# ");
return;
}
printf("%c ", root->_data);
PrevOrder(root->_left);
PrevOrder(root->_right);
}
void InOrder(BTNode*root)
{
if (root == NULL)
{
printf("# ");
return;
}
InOrder(root->_left);
printf("%c ", root->_data);
InOrder(root->_right);
}
void PostOrder(BTNode* root)
{
if (root == NULL)
{
printf("# ");
return;
}
PostOrder(root->_left);
PostOrder(root->_right);
printf("%c ", root->_data);
}
int TreeSize1(BTNode* root)
{
if (root == NULL)
{
return 0;
}
static int size = 0;
++size;
TreeSize1(root->_left);
TreeSize1(root->_right);
return size;
}
void TreeSize2(BTNode* root, int *psize)
{
if (root == NULL)
{
return;
}
else
{
++(*psize);
}
TreeSize2(root->_left,psize);
TreeSize2(root->_right,psize);
}
int TreeSize(BTNode* root)
{
if (root == NULL)
{
return 0;
}
return 1+TreeSize(root->_left) + TreeSize(root->_right);
}
int BinaryTreeLevelKSize(BTNode*root, int k)
{
if (k == 0)
{
return 0;
}
if (k == 1)
{
return 1;
}
return BinaryTreeLevelKSize(root->_left,k-1) + BinaryTreeLevelKSize(root->_right,k-1);
}
typedef struct QueueNode
{
BTNode* node;
struct QueueNode* next;
}QueueNode;
typedef struct QueueList
{
QueueNode* _front;
QueueNode* _tail;
}Queue;
void QueueInit(Queue* q)
{
assert(q);
q->_front = NULL;
q->_tail = NULL;
}
bool QueueEmpty(Queue* q)
{
assert(q);
if (q->_front == q->_tail&&q->_front == NULL)return 1;
else return 0;
}
BTNode* QueueFront(Queue* q)
{
assert(q);
assert(q->_front);
return q->_front->node;
}
void QueuePop(Queue* q)
{
assert(q);
assert(q->_front);
QueueNode* next = q->_front->next;
free(q->_front);
q->_front = next;
if (next == NULL)
{
q->_tail = NULL;
}
}
void QueuePush(Queue*q, BTNode* node)
{
assert(q);
QueueNode* newQueueNode = (QueueNode*)malloc(sizeof(QueueNode));
if (newQueueNode == NULL)
{
printf("内存不足");
exit(-1);
}
newQueueNode->node = node;
newQueueNode->next = NULL;
if (q->_front == NULL)
{
q->_front = q->_tail = newQueueNode;
}
else
{
q->_tail->next = newQueueNode;
q->_tail = newQueueNode;
}
}
void BinaryTreeLevelOrder(BTNode* root)
{
Queue q;
QueueInit(&q);
if (root == NULL)return;
QueuePush(&q, root);
while (!QueueEmpty(&q))
{
BTNode* front = QueueFront(&q);
printf("%c ", front->_data);
QueuePop(&q);
if (front->_left)
QueuePush(&q, front->_left);
if (front->_right)
QueuePush(&q, front->_right);
}
printf("\n");
}
#include<queue>
using namespace std;
void BinaryTreeLevelOrder3(BTNode* root)
{
BTNode* temp[100];
int in = 0;
int out = 0;
temp[in++] = root;
while (in > out)
{
if (temp[out])
{
cout << temp[out]->_data << " ";
temp[in++] = temp[out]->_left;
temp[in++] = temp[out]->_right;
}
out++;
}
}
int BinaryTreeComplete(BTNode* root){
Queue q;
QueueInit(&q);
if (root == NULL){
return 1;
}
QueuePush(&q, root);
while (!QueueEmpty(&q)){
BTNode*front = QueueFront(&q);
QueuePop(&q);
if (front == NULL) break;
QueuePush(&q, front->_left);
QueuePush(&q, front->_right);
}
while (!QueueEmpty(&q)){
BTNode*front = QueueFront(&q);
QueuePop(&q);
if (front) return 0;
}
return 1;
}
BTree_test.cc
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
#include"BTree.h"
void test4()
{
char arr[] = { 'A', 'B', 'D', '#', '#', 'E', '#', 'H', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#' };
int a = 0;
BTNode* root = BinaryTreeCreate(arr, &a);
PrevOrder(root);
printf("\n");
InOrder(root);
printf("\n");
PostOrder(root);
printf("\n");
int Tree_size = 0;
TreeSize2(root,&Tree_size);
printf("结点个数:%d\n", Tree_size);
int K_size = BinaryTreeLevelKSize(root, 3);
printf("第k层 结点个数:%d\n", K_size);
}
void test5()
{
char arr[] = { 'A', 'B', 'D', '#', '#', 'E', '#', 'H', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#' };
int a = 0;
BTNode* root = BinaryTreeCreate(arr, &a);
BinaryTreeLevelOrder(root);
int ret = BinaryTreeComplete(root);
printf("%d\n", ret);
}
int main()
{
test4();
test5();
system("pause");
return 0;
}