1.导入EasyExcel依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>4.0.3</version>
</dependency>
2.以学生信息为例,编写一个实体类
package org.example.easyexcel;
import com.alibaba.excel.annotation.ExcelProperty;
public class Student {
@ExcelProperty("姓名")
private String name;
@ExcelProperty("年龄")
private int age;
@ExcelProperty("地址")
private String address;
public Student(String name, int age, String address) {
this.name = name;
this.age = age;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
3.内容以\n进行分行,根据\n来计算行数,来设置行高
package org.example.easyexcel;
import com.alibaba.excel.metadata.Head;
import com.alibaba.excel.metadata.data.WriteCellData;
import com.alibaba.excel.write.metadata.holder.WriteSheetHolder;
import com.alibaba.excel.write.style.column.AbstractColumnWidthStyleStrategy;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.poi.ss.usermodel.Cell;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class CellWeightWeightStrategy extends AbstractColumnWidthStyleStrategy {
private final Map<Integer, Integer> maxRowsMap = new HashMap<>();
@Override
protected void setColumnWidth(WriteSheetHolder writeSheetHolder, List<WriteCellData<?>> cellDataList, Cell cell,
Head head, Integer relativeRowIndex, Boolean isHead) {
boolean needSetWidth = isHead || CollectionUtils.isNotEmpty(cellDataList);
if (needSetWidth) {
// 包含\n的内容修改行高
String cellValue = getCellValue(cell);
boolean contains = StringUtils.contains(cellValue, "\n");
if (contains) {
int rows = cellValue.split("\n").length;
int rowIndex = cell.getRowIndex();
Integer maxRows = maxRowsMap.get(rowIndex);
if (maxRows == null || maxRows < rows) {
cell.getRow().setHeightInPoints(rows * 15);
maxRowsMap.put(rowIndex, rows);
}
}
}
}
public static String getCellValue(Cell cell) {
String cellValue;
switch (cell.getCellType()) {
case STRING:
cellValue = cell.getStringCellValue();
break;
case BOOLEAN:
cellValue = String.valueOf(cell.getBooleanCellValue());
break;
case NUMERIC:
cellValue = String.valueOf(cell.getNumericCellValue());
break;
default:
cellValue = "";
break;
}
return cellValue;
}
}
4.设置以\n自动换行
package org.example.easyexcel;
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.write.metadata.WriteSheet;
import com.alibaba.excel.write.metadata.style.WriteCellStyle;
import com.alibaba.excel.write.style.HorizontalCellStyleStrategy;
import java.util.ArrayList;
import java.util.List;
public class EasyExcelTest01 {
public static void main(String[] args) {
String fileName = "D:\\test.xls";
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 18, "北京\n廊坊"));
studentList.add(new Student("李四", 20, "北京\n天津"));
studentList.add(new Student("王六", 27, "上海\n苏州\n杭州"));
try (ExcelWriter excelWriter = EasyExcel.write(fileName, Student.class).registerWriteHandler(new CellWeightWeightStrategy()).build()) {
// 设置自动换行,前提内容中需要加「\n」才有效
WriteCellStyle writeCellStyle = new WriteCellStyle();
writeCellStyle.setWrapped(true);
HorizontalCellStyleStrategy cellStyleStrategy =
new HorizontalCellStyleStrategy(null, writeCellStyle);
WriteSheet writeSheet = EasyExcel.writerSheet("学生").registerWriteHandler(cellStyleStrategy).build();
excelWriter.write(studentList, writeSheet);
excelWriter.finish();
}
}
}
5.最终展示效果如下:
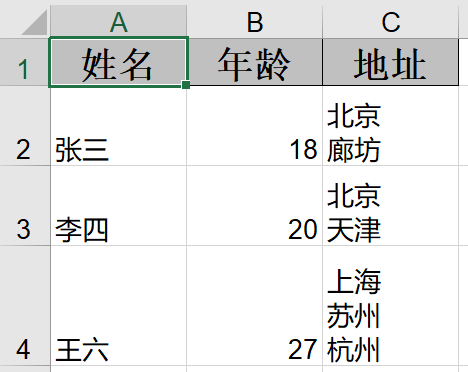