map = new AMap.Map('map-container', {
zoom: 18,
center: [116.39, 39.9],
showLabel: false,
mapStyle: 'amap://styles/whitesmoke',
viewMode: '3D',
pitch: 40,
})
map.getCenter()
map.getZoom()
- marker创建,增加标注,增加事件,非dom事件
const marker = new AMap.Marker({
position: [location.lng, location.lat],
content: '<div class="custom-icon"></div>',
})
marker.on('mouseover', () => {))
.on('mouseout', () => {))
.on('click', () => {))
marker.setLabel({
offset: new AMap.Pixel(10, 10),
content: `<div class='info'>${location.address}</div>`,
direction: 'right',
})
marker.setLabel({
content: null,
direction: null,
})
AMap.Event.trigger(marker, 'mouseover')
map.setZoomAndCenter(18, currentMarker.getPosition(), true)
var polyline = new AMap.Polyline({
path: [[v.lng, v.lat],[v1.lng, v1.lat]],
strokeColor: '#FF0000',
strokeWeight: 1,
strokeOpacity: 0.9
})
map.add(polyline)
map.on('complete', () => {
console.log('map complete')
emit('onMapInit')
}).on('click', () => {
}).on('dragend', () => {
}).on('touchstart', () => {
})
- 3D行政区图层AmbientLight,DirectionLight,Object3DLayer
这是效果图,可以基于此在上面添加图层,绘制轨迹和其它覆盖物(基于canvas)
官方提示: JS API v1.4.5版本开始,提供在3D视图模式下创建和添加三维立体图形的能力,这些立体图形包括网格(Mesh)类型、线类型、点类型等
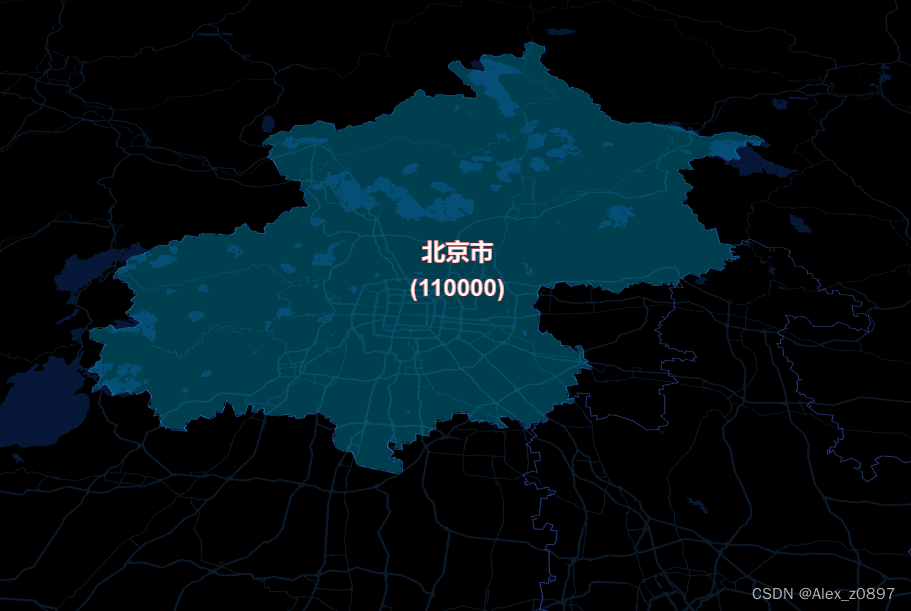
vue相关加载map和Object3D的部分
const loadMap = () => {
console.log('initMap')
const AMap = window.AMap
console.log(window.AMap)
map.value = new AMap.Map('map', {
resizeEnable: true,
mapStyle: 'amap://styles/darkblue',
viewMode: '3D',
pitch: 40,
zoom: 12,
showLabel: false
// labelzIndex: -1,
})
map.value.on('complete', () => {
console.log('map complete')
emit('onMapInit')
ambientLight()
})
}
const ambientLight = () => {
const AMap = window.AMap
const _map = map.value
_map.AmbientLight = new AMap.Lights.AmbientLight([1, 1, 1], 0.5)
_map.DirectionLight = new AMap.Lights.DirectionLight([0, 0, 1], [1, 1, 1], 1)
var object3Dlayer = new AMap.Object3DLayer()
_map.add(object3Dlayer)
new AMap.DistrictSearch({
subdistrict: 0, // 返回下一级行政区
extensions: 'all', // 返回行政区边界坐标组等具体信息
level: 'city' // 查询行政级别为 市
}).search('北京市', function (status, result) {
var bounds = result.districtList[0].boundaries
var height = 1000
var color = '#0088ff4f'
var prism = new AMap.Object3D.Prism({
path: bounds,
height: height,
color: color
})
prism.transparent = true
object3Dlayer.add(prism)
var text = new AMap.Text({
text: result.districtList[0].name + '</br>(' + result.districtList[0].adcode + ')',
verticalAlign: 'bottom',
position: [116.528261, 39.934313],
height: 5000,
style: {
'background-color': 'transparent',
'-webkit-text-stroke': 'red',
'-webkit-text-stroke-width': '0.5px',
'text-align': 'center',
border: 'none',
color: 'white',
'font-size': '24px',
'font-weight': 600
}
})
text.setMap(_map)
})
}
- 以下是官方找的demo, 然后再增加一些随机轨迹图层的渲染
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="initial-scale=1.0, user-scalable=no, width=device-width">
<title>英文、中英文地图</title>
<link rel="stylesheet" href="https://a.amap.com/jsapi_demos/static/demo-center/css/demo-center.css" />
<style>
html,
body,
width: 100%;
height: 100%;
}
height: 2rem;
}
display: inline-block;
width: 4rem;
}
color:
margin-left: 1rem;
margin-right: 0rem;
width: 6rem;
}
</style>
</head>
<body>
<div id="container"></div>
<script src="https://webapi.amap.com/maps?v=1.4.10&key=xxx&plugin=Map3D,AMap.DistrictSearch"></script>
<!--script crossorigin="anonymous" id="amap_plus_js" src="https://webapi.amap.com/maps/modules?v=1.4.22&key=bb01f498549fe82c328c9dc1c2280d6c&vrs=1671592305593&m=mouse,vectorlayer,overlay,wgl,vectorlayer,wgl,AMap.CustomLayer,rbush,Map3D,AMap.DistrictSearch,sync" type="text/javascript"></script-->
<script>
//初始化地图
var map = new AMap.Map('container', {
resizeEnable: true, //是否监控地图容器尺寸变化
mapStyle: "amap://styles/darkblue",
viewMode:'3D',
pitch: 70,
zoom: 12,
labelzIndex: -1,
});
//隐藏地图上的所有地名
//map.setFeatures(['bg', 'point', 'building']);
//绑定radio点击事件
var radios = document.querySelectorAll("#map-styles input");
radios.forEach(function(ratio) {
ratio.onclick = setMapStyle;
});
function setMapStyle() {
var styleName = "amap://styles/" + this.value;
map.setMapStyle(styleName);
}
// 设置光照
map.AmbientLight = new AMap.Lights.AmbientLight([1, 1, 1], 0.5);
map.DirectionLight = new AMap.Lights.DirectionLight([0, 0, 1], [1, 1, 1], 1);
var object3Dlayer = new AMap.Object3DLayer();
map.add(object3Dlayer);
new AMap.DistrictSearch({
subdistrict: 0, //返回下一级行政区
extensions: 'all', //返回行政区边界坐标组等具体信息
level: 'city' //查询行政级别为 市
}).search('朝阳区', function (status, result) {
console.log(status)
var bounds = result.districtList[0].boundaries;
var height = 1000;
var color = '#0088ff4f'; // rgba
var prism = new AMap.Object3D.Prism({
path: bounds,
height: height,
color: color
});
prism.transparent = true;
object3Dlayer.add(prism);
var text = new AMap.Text({
text: result.districtList[0].name + '</br>(' + result.districtList[0].adcode + ')',
verticalAlign: 'bottom',
position: [116.528261, 39.934313],
height: 5000,
style: {
'background-color': 'transparent',
'-webkit-text-stroke': 'red',
'-webkit-text-stroke-width': '0.5px',
'text-align': 'center',
'border': 'none',
'color': 'white',
'font-size': '24px',
'font-weight': 600
}
});
text.setMap(map);
});
function getData(callback){
AMap.plugin('AMap.DistrictSearch', function() {
var search = new AMap.DistrictSearch();
search.search('朝阳区', function(status, data) {
if (status === 'complete') {
var positions = []
var provinces = data['districtList'][0]['districtList']
for (var i = 0; i < provinces.length; i += 1) {
positions.push({
center: provinces[i].center,
radius:Math.max(2, Math.floor(Math.random() * 10))
})
}
callback(positions)
}
});
});
}
//绘制多段的轨迹
function addLayerV2() {
AMap.plugin('AMap.CustomLayer', function() {
var canvas = document.createElement('canvas');
var customLayer = new AMap.CustomLayer(canvas, {
zooms: [10, 18],
alwaysRender:true,//缩放过程中是否重绘,复杂绘制建议设为false
zIndex: 120 //显示高度
});
var segments = [];
var positions = [];
var lat_min = 39.86, lat_max = 40.05, lng_min = 116.38, lng_max = 116.55;
for (var i = 0; i < 5; i++) {
var positions = [];
var count = Math.floor(Math.random() * 10) + 10;
for (var j = 0; j < count; j++) {
var lat = Math.random() * (lat_max - lat_min) + lat_min;
var lng = Math.random() * (lng_max - lng_min) + lng_min;
positions.push([lng, lat]);
}
segments.push(positions);
}
var onRender = function(){
var retina = AMap.Browser.retina;
var size = map.getSize();//resize
var width = size.width;
var height = size.height;
canvas.style.width = width+'px'
canvas.style.height = height+'px'
if(retina){//高清适配
width*=2;
height*=2;
}
canvas.width = width;
canvas.height= height;//清除画布
var ctx = canvas.getContext("2d");
for (var i = 0; i < segments.length; i++) {
var positions = segments[i];
ctx.strokeStyle = "#" + ((1 << 24) * Math.random() | 0).toString(16);
ctx.lineWidth = 5;
ctx.beginPath();
for (var j = 0; j < positions.length; j++) {
var pixel = map.lngLatToContainer(positions[j]);
if (j == 0) {
ctx.moveTo(pixel.x, pixel.y);
} else {
ctx.lineTo(pixel.x, pixel.y);
}
}
ctx.stroke();
}
};
customLayer.render = onRender;
customLayer.setMap(map);
});
}
getData(addLayerV2);
</script>
</body>
</html>