1.问题描述
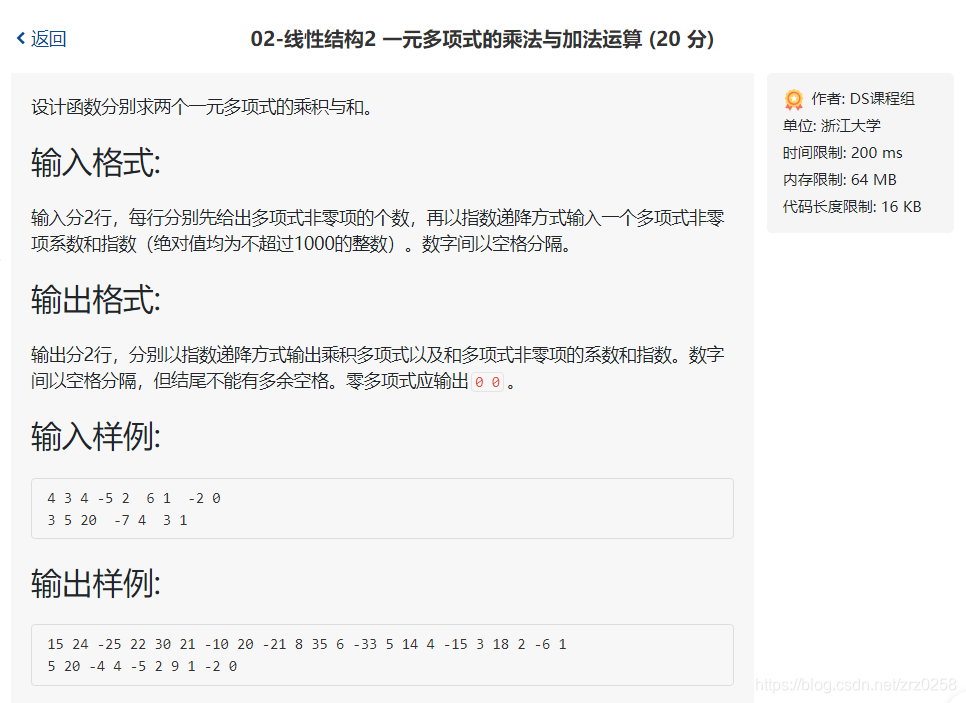
2.注意事项
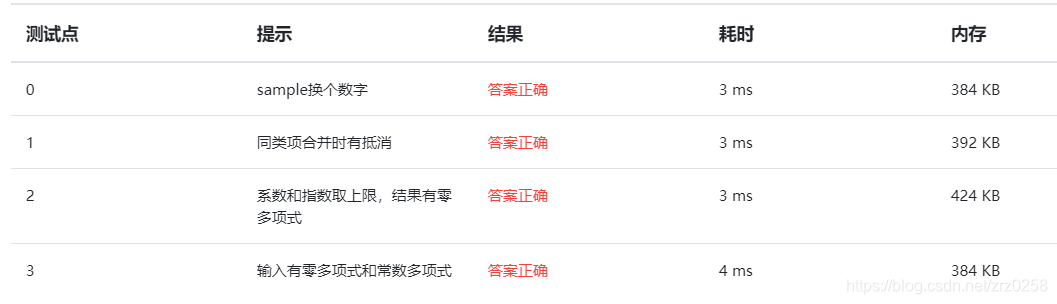
3.代码(C++实现)
#include<iostream>
using namespace std;
typedef struct NODE {
int xi;
int zhi;
struct NODE* next;
}Node;
Node* Get() {
int num;
cin >> num;
Node* node;
Node* head;
Node* temp;
node = new Node;
node->next = NULL;
head = node;
if (num > 0) {
for (; num > 0; num--) {
temp = new Node;
cin >> temp->xi;
cin >> temp->zhi;
temp->next = NULL;
head->next = temp;
head = head->next;
}
}
return node;
}
void Print(Node* node) {
Node* p = node->next;
if (!p) {
cout << '0' << ' ' << '0' << endl;
}
else
for (; p; p = p->next) {
if(p->next != NULL)
cout << p->xi << ' ' << p->zhi << ' ';
else
cout << p->xi << ' ' << p->zhi << endl;
}
}
Node* Add(Node* n1, Node* n2) {
Node* add = new Node;
add->next = NULL;
Node* t1 = n1->next;
Node* t2 = n2->next;
Node* head = add;
Node* temp;
while (t1 != NULL && t2 != NULL) {
if (t1->zhi > t2->zhi) {
temp = new Node;
temp->next = NULL;
temp->zhi = t1->zhi;
temp->xi = t1->xi;
add->next = temp;
add = add->next;
t1 = t1->next;
}
else if (t1->zhi < t2->zhi) {
temp = new Node;
temp->next = NULL;
temp->zhi = t2->zhi;
temp->xi = t2->xi;
add->next = temp;
add = add->next;
t2 = t2->next;
}
else if (t1->zhi == t2->zhi) {
if (t1->xi + t2->xi == 0) {
}
else {
temp = new Node;
temp->next = NULL;
temp->zhi = t1->zhi;
temp->xi = t1->xi + t2->xi;
add->next = temp;
add = add->next;
}
t1 = t1->next;
t2 = t2->next;
}
}
if (t2 != NULL)
add->next = t2;
else if (t1 != NULL)
add->next = t1;
return head;
}
Node* Mul(Node* n1, Node* n2) {
Node* t1 = n1->next;
Node* t2 = n2->next;
Node* mul = new Node;
mul->next = NULL;
int i;
if (t1 == NULL || t2 == NULL) {
return mul;
}
else {
for (i=1; t1; t1 = t1->next,i++) {
Node* hang = new Node;
hang->next = NULL;
Node* headhang = hang;
for (t2 = n2->next; t2; t2 = t2->next) {
Node* temp = new Node;
temp->next = NULL;
temp->xi = t1->xi * t2->xi;
temp->zhi = t1->zhi + t2->zhi;
hang->next = temp;
hang = hang->next;
}
mul = Add(mul, headhang);
}
return mul;
}
}
int main() {
Node* n1;
n1 = Get();
Node* n2;
n2 = Get();
Node* mul;
mul = Mul(n1, n2);
Print(mul);
Node* add;
add = Add(n1, n2);
Print(add);
return 0;
}