最近各手机厂商都在宣传万级调光,宣传数字越来越大,但是到底背光调节平滑度体验如何呢?
如最近realme 11 pro+ (MTK平台天玑7050),宣传20000级自动亮度调节。
发布会截图:
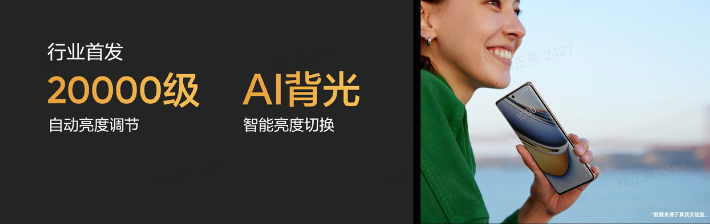
使用adb shell dumpsys SurfaceFlinger,发现 背光调节range[1~20479]
[LED state]
display_0: support[1]
brightness[0.393965] range[1~20479] current[8068]
display_1: support[0]
display_2: support[0]
测试验证了一下realme 11 pro+屏幕背光:
最小亮度1.7nit, 手动最大亮度500nit, HBM到800nit 。
从log看 最小dbv12, 手动最大18740,hbm 最大20479。
normal 区间:brightness[0.000049~0.915084] range[1~18740]
hbm 区间:brightness[0.915133~1.000000] range[18741~20479]
目前国内手机厂商竞争异常激烈,疯狂卷参数,但是这些参数对我们用户来说,具体有落实到实处吗?这是一个大大的问号
为了使用光学仪器410精准的测量屏幕背光情况,所以打写一个简单的背光测试apk。
一、获取背光控制最大最小值
frameworks/base/core/res/res/values/config.xml
<!-- Note: This setting is deprecated, please use
config_screenBrightnessSettingMinimumFloat instead -->
<integer name="config_screenBrightnessSettingMinimum">10</integer>
<!-- Note: This setting is deprecated, please use
config_screenBrightnessSettingMaximumFloat instead -->
<integer name="config_screenBrightnessSettingMaximum">255</integer>
<!-- Note: This setting is deprecated, please use
config_screenBrightnessSettingDefaultFloat instead -->
<integer name="config_screenBrightnessSettingDefault">102</integer>
<!-- Minimum screen brightness setting allowed by power manager.
-2 is invalid so setting will resort to int value specified above.
Set this to 0.0 to allow screen to go to minimal brightness.
The user is forbidden from setting the brightness below this level. -->
<item name="config_screenBrightnessSettingMinimumFloat" format="float" type="dimen">-2</item>
<!-- Maximum screen brightness allowed by the power manager.
-2 is invalid so setting will resort to int value specified above.
Set this to 1.0 for maximum brightness range.
The user is forbidden from setting the brightness above this level. -->
<item name="config_screenBrightnessSettingMaximumFloat" format="float" type="dimen">-2</item>
<!-- Default screen brightness setting set.
-2 is invalid so setting will resort to int value specified above.
Must be in the range specified by minimum and maximum. -->
<item name="config_screenBrightnessSettingDefaultFloat" format="float" type="dimen">-2</item>
直接通过代码获取config_screenBrightnessSettingMinimum和config_screenBrightnessSettingMaximum
//获取系统最小屏幕亮度:config_screenBrightnessSettingMinimum
private int getScreenBrightnessSettingMinimum(Context context) {
int screenBrightnessSettingMinimumId = getResources().getIdentifier("config_screenBrightnessSettingMinimum", "integer", "android");
int screenBrightnessSettingMinimum = getResources().getInteger(screenBrightnessSettingMinimumId);
return screenBrightnessSettingMinimum;
}
//获取系统最大屏幕亮度:config_screenBrightnessSettingMaximum
private int getscreenBrightnessSettingMaximum(Context context) {
int screenBrightnessSettingMaximumId = context.getResources().getIdentifier("config_screenBrightnessSettingMaximum", "integer", "android");
int screenBrightnessSettingMaximum = context.getResources().getInteger(screenBrightnessSettingMaximumId);
return screenBrightnessSettingMaximum;
}
二、设置屏幕背光
第三方app设置屏幕背光有两种方法:
-
修改APP界面屏幕亮度,只影响本app,不会影响整个系统,退出app界面,恢复系统亮度
-
修改系统 Settings 中的屏幕亮度,影响所有APP
1.修改APP界面屏幕亮度
修改自身 APP 亮度比较简单,只需要在Activity 获取window的attributes,通过WindowManager.LayoutParams的screenBrightness变量调节
//修改当前Activity界面的窗口亮度
private void setWindowScreenBrightness(int brightness) {
Window window = getWindow();
WindowManager.LayoutParams lp = window.getAttributes();
lp.screenBrightness = (float) brightness / (float)mBrightnessSettingMaximum;
window.setAttributes(lp);
}
只影响当前app界面,退出app,恢复到系统背光。
2.修改系统 Settings 中的屏幕亮度
2.1 AndroidManifest.xml添加如下权限
<uses-permission android:name="android.permission.WRITE_SETTINGS"/>
2.2 非系统签名应用,检测是否有WRITE_SETTINGS,没有的话去请求用户授权
//检测是否有WRITE_SETTINGS,没有的话去请求用户授权
private boolean checkWriteSettingPermission(Activity context, int requestCode) {
boolean permission;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
permission = Settings.System.canWrite(context);
} else {
permission = ContextCompat.checkSelfPermission(context, Manifest.permission.WRITE_SETTINGS) == PackageManager.PERMISSION_GRANTED;
}
if (!permission) {
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.M) {
Intent intent = new Intent(Settings.ACTION_MANAGE_WRITE_SETTINGS);
intent.setData(Uri.parse("package:" + context.getPackageName()));
context.startActivityForResult(intent, requestCode);
} else {
ActivityCompat.requestPermissions(context, new String[]{Manifest.permission.WRITE_SETTINGS}, requestCode);
}
}
return permission;
}
2.3 关闭自动亮度,设置手动调节背光模式
//关闭光感,设置手动调节背光模式
//SCREEN_BRIGHTNESS_MODE_AUTOMATIC 自动调节屏幕亮度模式值为1
//SCREEN_BRIGHTNESS_MODE_MANUAL 手动调节屏幕亮度模式值为0
public void setScreenManualMode(Context context) {
ContentResolver contentResolver = context.getContentResolver();
try {
int mode = Settings.System.getInt(contentResolver,
Settings.System.SCREEN_BRIGHTNESS_MODE);
if (mode == Settings.System.SCREEN_BRIGHTNESS_MODE_AUTOMATIC) {
Settings.System.putInt(contentResolver,
Settings.System.SCREEN_BRIGHTNESS_MODE,
Settings.System.SCREEN_BRIGHTNESS_MODE_MANUAL);
}
} catch (Settings.SettingNotFoundException e) {
e.printStackTrace();
}
}
2.4 获取当前背光和设置背光的方法
//获取系统屏幕亮度
private int getScreenBrightness(Context context) {
int brightness = -1;
ContentResolver resolver = context.getContentResolver();
try {
brightness = Settings.System.getInt(resolver, Settings.System.SCREEN_BRIGHTNESS);
} catch (Settings.SettingNotFoundException e) {
e.printStackTrace();
}
return brightness;
}
// 修改系统的亮度值
private void setScreenLight(int brightness) {
Uri uri = Settings.System.getUriFor(Settings.System.SCREEN_BRIGHTNESS);
ContentResolver contentResolver = getContentResolver();
Settings.System.putInt(contentResolver, Settings.System.SCREEN_BRIGHTNESS, brightness);
contentResolver.notifyChange(uri, null);
}
三、测试程序
测试程序使用第一种方法:修改APP界面屏幕亮度,不会影响其他APP
java:
MainActivity.java
package com.example.screenbrightnesstest;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
import android.app.Activity;
import android.content.ContentResolver;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Color;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.provider.Settings;
import android.util.Log;
import android.view.View;
import android.view.Window;
import android.view.WindowManager;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import android.Manifest;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private static final String TAG = "lzl-test";
private Context mContext;
private int mRoScreenBrightnessSettingMinimum;
private int mRoScreenBrightnessSettingMaximum;
private TextView mTextViewScreenBrightnessRange;
private EditText mEditTextInputIntBrightnessMin;
private int mInputIntIntBrightnessMin;
private EditText mEditTextInputIntBrightnessMax;
private int mInputIntIntBrightnessMax;
private EditText mEditTextInputIntBrightness;
private int mInputIntBrightness;
private EditText mEditTextInputIntBrightnessAddStep;
private int mInputIntBrightnessAddStep = 1;
private TextView mTextViewOutputInfo;
private int mColor = Color.RED;
private int onClickCount = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mContext = getApplicationContext();
//获取系统最小屏幕亮度:config_screenBrightnessSettingMinimum
mRoScreenBrightnessSettingMinimum = getScreenBrightnessSettingMinimum(mContext);
Log.d(TAG, "config_screenBrightnessSettingMinimum:" + mRoScreenBrightnessSettingMinimum);
//获取系统最大屏幕亮度:config_screenBrightnessSettingMaximum
mRoScreenBrightnessSettingMaximum = getscreenBrightnessSettingMaximum(mContext);
Log.d(TAG, "config_screenBrightnessSettingMaximum:" + mRoScreenBrightnessSettingMaximum);
TextView textView = (TextView)findViewById(R.id.tv_screenBrightnessSettingMinimum_val);
textView.setText(String.format("%d",mRoScreenBrightnessSettingMinimum));
textView = (TextView)findViewById(R.id.tv_screenBrightnessSettingMaximum_val);
textView.setText(String.format("%d",mRoScreenBrightnessSettingMaximum));
mInputIntIntBrightnessMin = mRoScreenBrightnessSettingMinimum;
mInputIntIntBrightnessMax = mRoScreenBrightnessSettingMaximum;
mEditTextInputIntBrightnessMin = (EditText)findViewById(R.id.editTextNumber_screenBrightnessMinimum);
mEditTextInputIntBrightnessMin.setText(String.format("%d",mInputIntIntBrightnessMin));
mEditTextInputIntBrightnessMax = (EditText)findViewById(R.id.editTextNumber_screenBrightnessMaximum);
mEditTextInputIntBrightnessMax.setText(String.format("%d",mInputIntIntBrightnessMax));
mTextViewScreenBrightnessRange = (TextView)findViewById(R.id.tv_ScreenBrightness_range);
mTextViewScreenBrightnessRange.setText(String.format("ScreenBrightness 测试,输入范围[%d~%d]",mInputIntIntBrightnessMin, mInputIntIntBrightnessMax));
mEditTextInputIntBrightness = (EditText)findViewById(R.id.editTextNumber_screenBrightness);
mInputIntBrightness = mInputIntIntBrightnessMax/2;
mEditTextInputIntBrightness.setText(String.format("%d",mInputIntBrightness));
mEditTextInputIntBrightnessAddStep = (EditText)findViewById(R.id.editTextNumber_screenBrightnessAddStep);
mInputIntBrightnessAddStep = 1;
mEditTextInputIntBrightnessAddStep.setText(String.format("%d",mInputIntBrightnessAddStep));
findViewById(R.id.button_set_screenBrightnessMinimum).setOnClickListener(this);
findViewById(R.id.button_set_screenBrightnessMaximum).setOnClickListener(this);
findViewById(R.id.button_set_screenBrightness).setOnClickListener(this);
findViewById(R.id.button_set_screenBrightnessAddStep).setOnClickListener(this);
findViewById(R.id.button_add).setOnClickListener(this);
findViewById(R.id.button_add).setOnClickListener(this);
mTextViewOutputInfo = (TextView) findViewById(R.id.tv_output_info);
}
//获取系统最小屏幕亮度:config_screenBrightnessSettingMinimum
private int getScreenBrightnessSettingMinimum(Context context) {
int screenBrightnessSettingMinimumId = getResources().getIdentifier("config_screenBrightnessSettingMinimum", "integer", "android");
int screenBrightnessSettingMinimum = getResources().getInteger(screenBrightnessSettingMinimumId);
return screenBrightnessSettingMinimum;
}
//获取系统最大屏幕亮度:config_screenBrightnessSettingMaximum
private int getscreenBrightnessSettingMaximum(Context context) {
int screenBrightnessSettingMaximumId = context.getResources().getIdentifier("config_screenBrightnessSettingMaximum", "integer", "android");
int screenBrightnessSettingMaximum = context.getResources().getInteger(screenBrightnessSettingMaximumId);
return screenBrightnessSettingMaximum;
}
//修改当前Activity界面的窗口亮度
private void setWindowScreenBrightness(int brightness) {
Window window = getWindow();
WindowManager.LayoutParams lp = window.getAttributes();
Log.d(TAG, "Brightness:" + brightness + ", Max Brightness:" + mInputIntIntBrightnessMax);
lp.screenBrightness = (float) brightness / (float)mInputIntIntBrightnessMax;
window.setAttributes(lp);
}
private void showTextViewOutputInfo() {
mColor = (onClickCount % 2 == 0) ? Color.RED : Color.BLUE;
String str = String.format("范围:%d~%d\nint brightness[%d], float brightness[%f]\n自增步长: %d\n",
mInputIntIntBrightnessMin, mInputIntIntBrightnessMax, mInputIntBrightness,
(float) mInputIntBrightness / (float)mInputIntIntBrightnessMax, mInputIntBrightnessAddStep);
mTextViewOutputInfo.setText(str);
mTextViewOutputInfo.setTextColor(mColor);
}
@Override
public void onClick(View v) {
onClickCount++;
if (v.getId() == R.id.button_set_screenBrightnessMinimum) {
if (!mEditTextInputIntBrightnessMin.getText().toString().isEmpty()) {
mInputIntIntBrightnessMin = Integer.parseInt(mEditTextInputIntBrightnessMin.getText().toString());
} else {
mInputIntIntBrightnessMin = mRoScreenBrightnessSettingMaximum;
}
mTextViewScreenBrightnessRange.setText(String.format("ScreenBrightness 测试,输入范围[%d~%d]",mInputIntIntBrightnessMin, mInputIntIntBrightnessMax));
showTextViewOutputInfo();
} else if (v.getId() == R.id.button_set_screenBrightnessMaximum) {
if (!mEditTextInputIntBrightnessMax.getText().toString().isEmpty()) {
mInputIntIntBrightnessMax = Integer.parseInt(mEditTextInputIntBrightnessMax.getText().toString());
} else {
mInputIntIntBrightnessMax = mRoScreenBrightnessSettingMaximum;
}
mTextViewScreenBrightnessRange.setText(String.format("ScreenBrightness 测试,输入范围[%d~%d]",mInputIntIntBrightnessMin, mInputIntIntBrightnessMax));
showTextViewOutputInfo();
} else if (v.getId() == R.id.button_set_screenBrightness) {
if (!mEditTextInputIntBrightness.getText().toString().isEmpty()) {
mInputIntBrightness = Integer.parseInt(mEditTextInputIntBrightness.getText().toString());
Log.d(TAG, "mIntBrightness:" + mInputIntBrightness);
if (mInputIntBrightness < mInputIntIntBrightnessMin || mInputIntBrightness > mInputIntIntBrightnessMax) {
Toast.makeText(getApplicationContext(), "请输入有效int型Brightness,range[" + mInputIntIntBrightnessMin +"~" + mInputIntIntBrightnessMax +"]", 1000).show();
} else {
setWindowScreenBrightness(mInputIntBrightness);
showTextViewOutputInfo();
}
} else {
Toast.makeText(getApplicationContext(), "请输入有效的参数", 1000).show();
}
} else if (v.getId() == R.id.button_set_screenBrightnessAddStep) {
if (!mEditTextInputIntBrightnessAddStep.getText().toString().isEmpty()) {
mInputIntBrightnessAddStep = Integer.parseInt(mEditTextInputIntBrightnessAddStep.getText().toString());
Log.d(TAG, "mIntBrightnessAddStep:" + mInputIntBrightnessAddStep);
if ((mInputIntBrightnessAddStep + mInputIntBrightness) > mInputIntIntBrightnessMax) {
mInputIntBrightnessAddStep = 1;
Toast.makeText(getApplicationContext(), "请输入有效的参数", 1000).show();
}
} else {
Toast.makeText(getApplicationContext(), "请输入有效的参数", 1000).show();
}
showTextViewOutputInfo();
} else if (v.getId() == R.id.button_add) {
mInputIntBrightness = mInputIntBrightness + mInputIntBrightnessAddStep;
Log.d(TAG, "mIntBrightness:" + mInputIntBrightness);
if (mInputIntBrightness > mInputIntIntBrightnessMax) {
Toast.makeText(getApplicationContext(), "Brightness超过了[" + mInputIntIntBrightnessMin +"~" + mInputIntIntBrightnessMax +"]范围", 1000).show();
} else {
setWindowScreenBrightness(mInputIntBrightness);
showTextViewOutputInfo();
}
} else {
Log.e(TAG, "未知按键");
}
}
}
xml:
activity_main.xml layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:layout_margin="5dp">
<TextView
android:id="@+id/tv_screenBrightnessSetting_info"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="30dp"
android:layout_gravity="center"
android:text="获取 screenBrightness 配置信息" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_screenBrightnessSettingMinimum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="config_screenBrightnessSettingMinimum:" />
<TextView
android:id="@+id/tv_screenBrightnessSettingMinimum_val"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_screenBrightnessSettingMaximum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="config_screenBrightnessSettingMaximum:" />
<TextView
android:id="@+id/tv_screenBrightnessSettingMaximum_val"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="" />
</LinearLayout>
<TextView
android:id="@+id/textView_ScreenBrightness_min_max_info"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="30dp"
android:layout_gravity="center"
android:text="ScreenBrightness 测试,设置最小/最大亮度值" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_screenBrightnessSettingMinimum_input"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="输入:" />
<EditText
android:id="@+id/editTextNumber_screenBrightnessMinimum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:layout_weight="1"
android:inputType="number" />
<Button
android:id="@+id/button_set_screenBrightnessMinimum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="设置" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_screenBrightnessSettingMaximum_input"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="输入:" />
<EditText
android:id="@+id/editTextNumber_screenBrightnessMaximum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:layout_weight="1"
android:inputType="number" />
<Button
android:id="@+id/button_set_screenBrightnessMaximum"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="设置" />
</LinearLayout>
<TextView
android:id="@+id/tv_ScreenBrightness_range"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="30dp"
android:layout_gravity="center"
android:text="ScreenBrightness 测试,输入范围" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_screenBrightness_input"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="输入:" />
<EditText
android:id="@+id/editTextNumber_screenBrightness"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:layout_weight="1"
android:inputType="number" />
<Button
android:id="@+id/button_set_screenBrightness"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="设置" />
</LinearLayout>
<TextView
android:id="@+id/tv_screenBrightness_add_input"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="30dp"
android:layout_gravity="center"
android:text="screenBrightness自增步长设置" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_add_setp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="输入:" />
<EditText
android:id="@+id/editTextNumber_screenBrightnessAddStep"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:layout_weight="1"
android:inputType="number" />
<Button
android:id="@+id/button_set_screenBrightnessAddStep"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="设置" />
</LinearLayout>
<Button
android:id="@+id/button_add"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_weight="1"
android:text="自增" />
<TextView
android:id="@+id/tv_output"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="30dp"
android:layout_gravity="center"
android:text="输出信息" />
<TextView
android:id="@+id/tv_output_info"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="5dp"
android:layout_gravity="center" />
</LinearLayout>
</LinearLayout>
运行
我自己主力机器刚好是小米13Ultra,对比验证了一下:
-
realme 11 pro+直接通过代码获取config_screenBrightnessSettingMinimum和config_screenBrightnessSettingMaximum分别是1和255
-
小米13Ultra直接通过代码获取config_screenBrightnessSettingMinimum和config_screenBrightnessSettingMaximum分别是15和16383
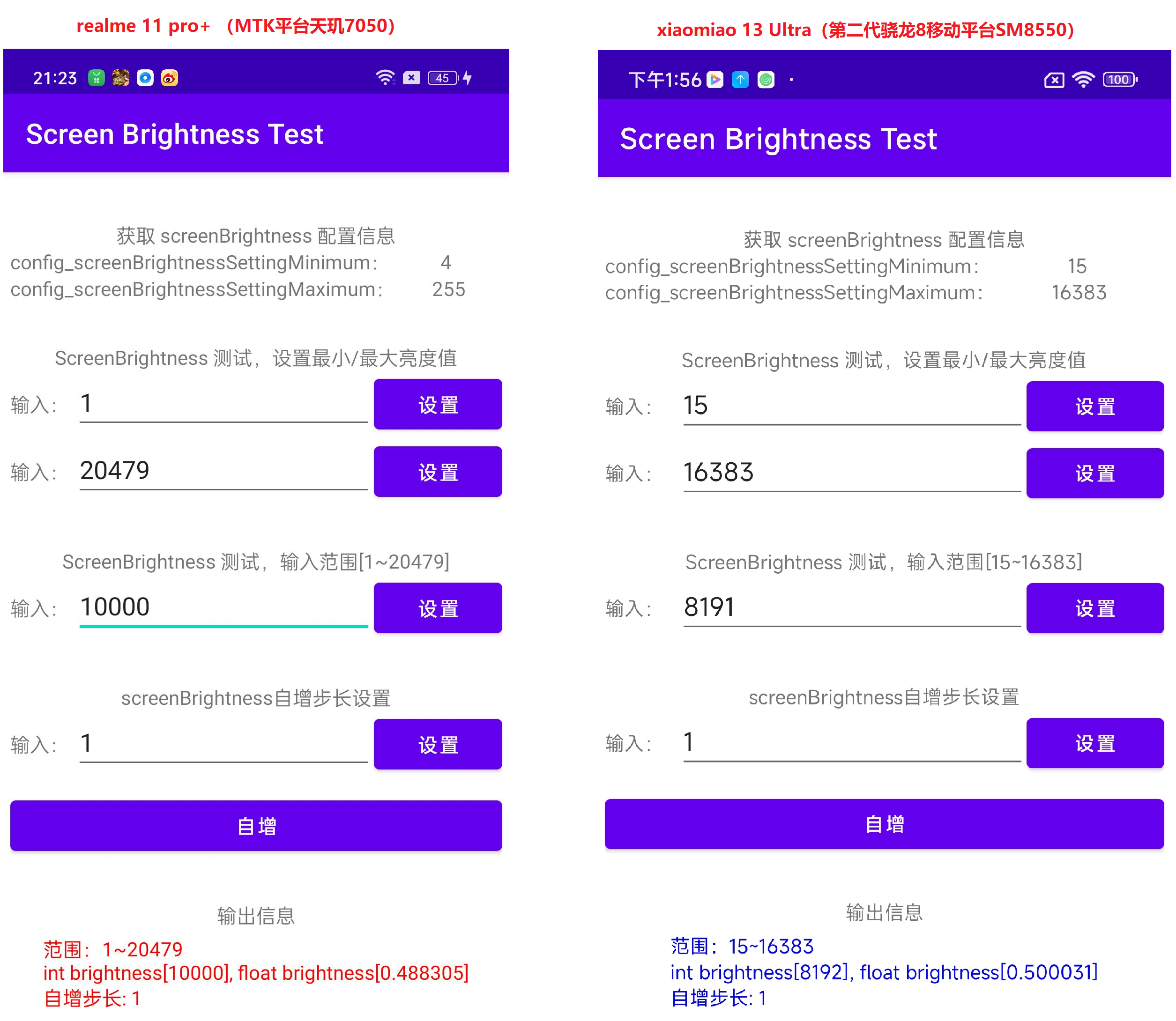
背光验证:
apk可以给手机准确的调背光,测试了下realme 11 pro+的亮度情况,手机整体 brightness 12-18740 对应1.74 -456nit的 2.2gamma形亮度曲线。 这个形状的亮度曲线,在低亮度的时候,亮度变化会比较小。
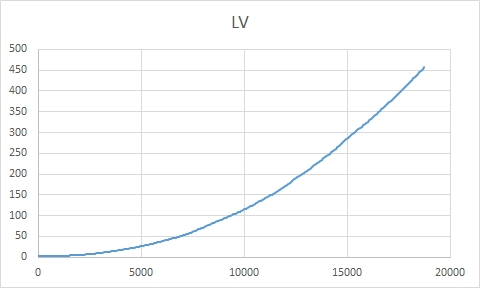
从理论最大500nit计算,最小的亮度差应该是0.0025nit,410光学仪器在1-10nit的测试精度是2%,人眼全黑的状态下可以识别的亮度差大约是0.025nit。所以这个精度,应该是没有必要。
而具体测试了前1000个亮度,发现整体表现并不好,连最起码的单调递增都没有做到,亮度曲线有轻微的反复。这也很像MTK平台万级背光方案调节亮度跟内容修改参数没有匹配很好的现象。
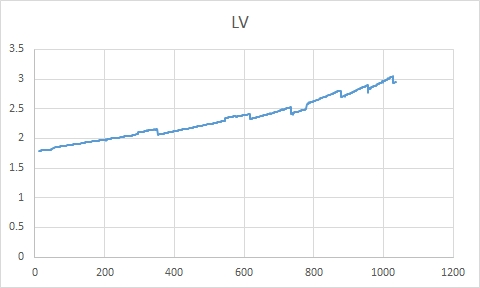
主观看,在低亮部分,也能看到一些轻微抖动,所以这个2w级背光调节,对我们用户的实际体验,应该是没有特别的优化效果。
源码
百度网盘链接:百度网盘 请输入提取码 提取码:test
github下载地址:
GitHub - liuzhengliang1102/AndroidStudio-LearnAppDevelopment
ScreenBrightnessTest目录